Display a list or grid Collection
Display and arrange collections of items efficiently with lists and grids.
@Composable private fun ScrollBoxes() { Column( modifier = Modifier .background(Color.LightGray) .size(100.dp) .verticalScroll(rememberScrollState()) ) { repeat(10) { Text("Item $it", modifier = Modifier.padding(2.dp)) } } }
Create a finite scrollable list
Display a finite set of items in a scrollable list to manage datasets, create responsive designs, and facilitate navigation.
val itemsList = (0..5).toList() val itemModifier = Modifier.border(1.dp, Color.Blue).width(80.dp).wrapContentSize() LazyHorizontalGrid( rows = GridCells.Fixed(3), horizontalArrangement = Arrangement.spacedBy(16.dp), verticalArrangement = Arrangement.spacedBy(16.dp) ) { items(itemsList) { Text("Item is $it", itemModifier) } item { Text("Single item", itemModifier) } }
Create a scrollable grid
Create a scrolling grid using lazy grids to manage large datasets and dynamic content.
LazyColumn { items( messages, key = { it.id }, contentType = { it.type } ) { message -> when (message) { is Audio -> AudioMessge(message) is Text -> TextMessage(message) }
Build a list using multiple item types
Display mixed content types, such as text, images, and interactive elements, using a list with multiple item types.
val lazyPagingItems = pager.flow.collectAsLazyPagingItems() LazyColumn { items( lazyPagingItems.itemCount, key = lazyPagingItems.itemKey { it.id } ) { index -> val message = lazyPagingItems[index] if (message != null) { MessageRow(message) } else { MessagePlaceholder() } } } @Composable fun MessagePlaceholder() { Box(Modifier.fillMaxWidth().height(48.dp)) { CircularProgressIndicator() } } @Composable fun TextMessage(message: Text) { Card(modifier = Modifier.padding(8.dp)) { Column( modifier = Modifier.padding(8.dp), verticalArrangement = Arrangement.Center ) { Text(message.sender) Text(message.text) } } }
Lazily load data with lists and Paging
Load and display large lists incrementally, reducing initial load times and optimizing memory ussge.
val scrollState = rememberScrollState() Column( modifier = Modifier .fillMaxWidth() .verticalScroll(scrollState), ) { Box( modifier = Modifier .fillMaxWidth() .height(500.dp) .background(Color.White) .graphicsLayer { translationY = 0.5f * scrollState.value }, contentAlignment = Alignment.Center ) { Image( painterResource(id = R.drawable.android_logo_vector), contentDescription = "Android logo", contentScale = ContentScale.FillWidth, modifier = Modifier.fillMaxSize() ) } Text( text = stringResource(R.string.lorem_ipsum), modifier = Modifier .background(Color.White) .padding(horizontal = 8.dp), style = TextStyle(fontSize = 24.sp), color = Color.Black, textAlign = TextAlign.Justify ) }
Create a parallax scrolling effect
Implement parallax scrolling in your app's UI to create a more dynamic user experience.
LazyColumn { items(verticalListItems) { Column { Text( text = it.title, style = MaterialTheme.typography.titleLarge, modifier = Modifier.padding(horizontal = 8.dp) ) LazyRow( contentPadding = PaddingValues(horizontal = 16.dp, vertical = 8.dp), horizontalArrangement = Arrangement.spacedBy(4.dp), ) { items(it.pictures) { Card { AsyncImage( model = it.url, contentDescription = “A remote image”, ) } } } } } }
Display nested scrolling items in a list
Display nested items within a scrolling list to present complex layouts.
@Composable fun MessageList() { val listState = rememberLazyListState() // Remember a CoroutineScope to be able to launch val coroutineScope = rememberCoroutineScope() LazyColumn(state = listState, modifier = Modifier.height(120.dp)) { items(10, { index -> index }) { Text( modifier = Modifier.height(40.dp), text = "Item $it" ) } } Button(onClick = { coroutineScope.launch { // Animate scroll to the first item listState.animateScrollToItem(index = 0) } }) { Text(text = "Go top") } }
Create a button to enable snap scrolling
Snap scrolling lets users scroll to a certain point on a list, saving time and increasing user engagement with content.
// Display 10 items val pagerState = rememberPagerState(pageCount = { 10 }) HorizontalPager(state = pagerState) { page -> // Our page content. Text( text = "Page: $page", modifier = Modifier.fillMaxWidth() ) }
Display a paging list
Create a horizontal or vertical paging list to display content that users can access by scrolling.
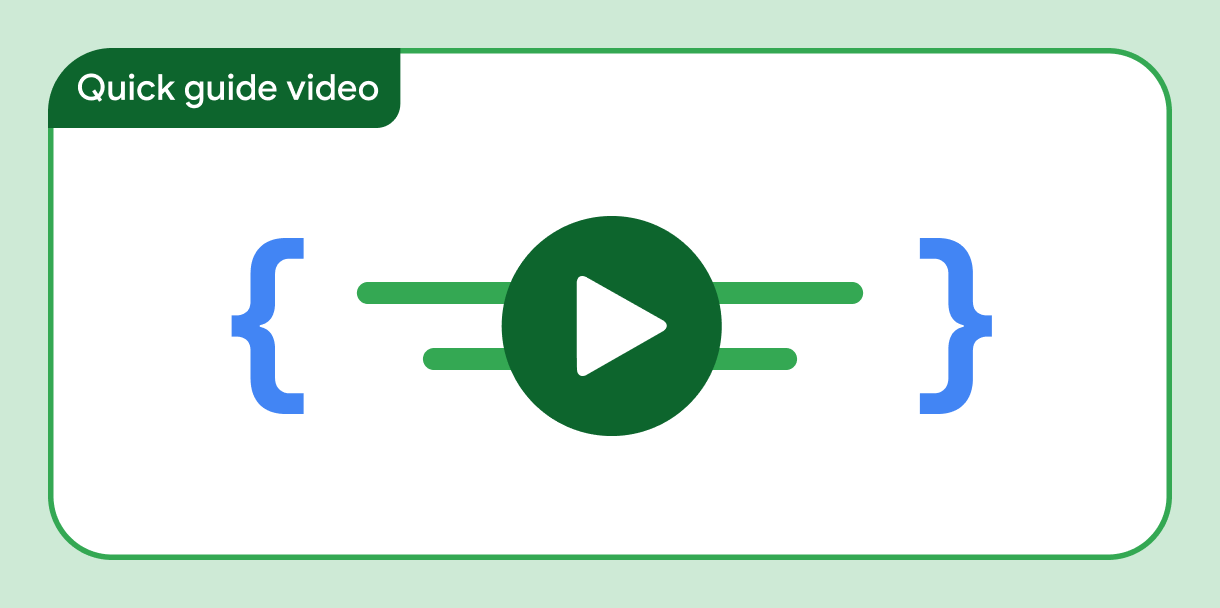
Lists in Compose
5 minutes
Explore Compose's Lazy components that make it easy to display lists of items. Learn how to show different item types and even how to implement sticky headers. See how to programmatically control or react to the scroll-position changes.
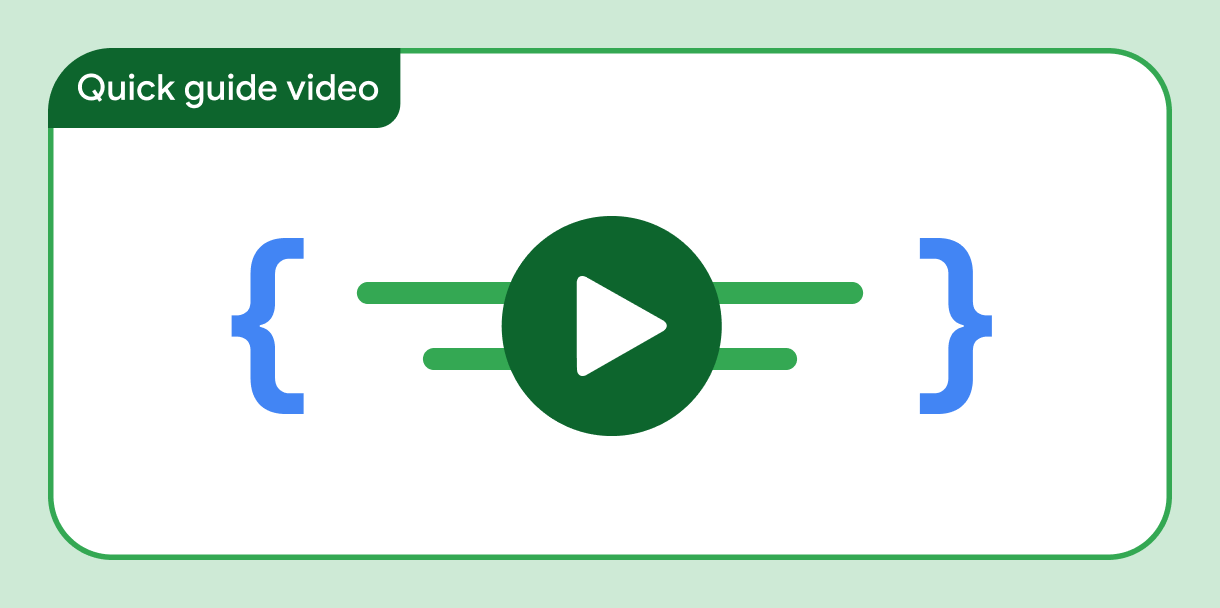
Lazy Lists
5 minutes
Compose gives you a simpler and more-performant way of creating scrolling lists, using fewer lines of code than RecyclerView. Learn how to use lazy layouts to create lists that let add content to lists, on demand.
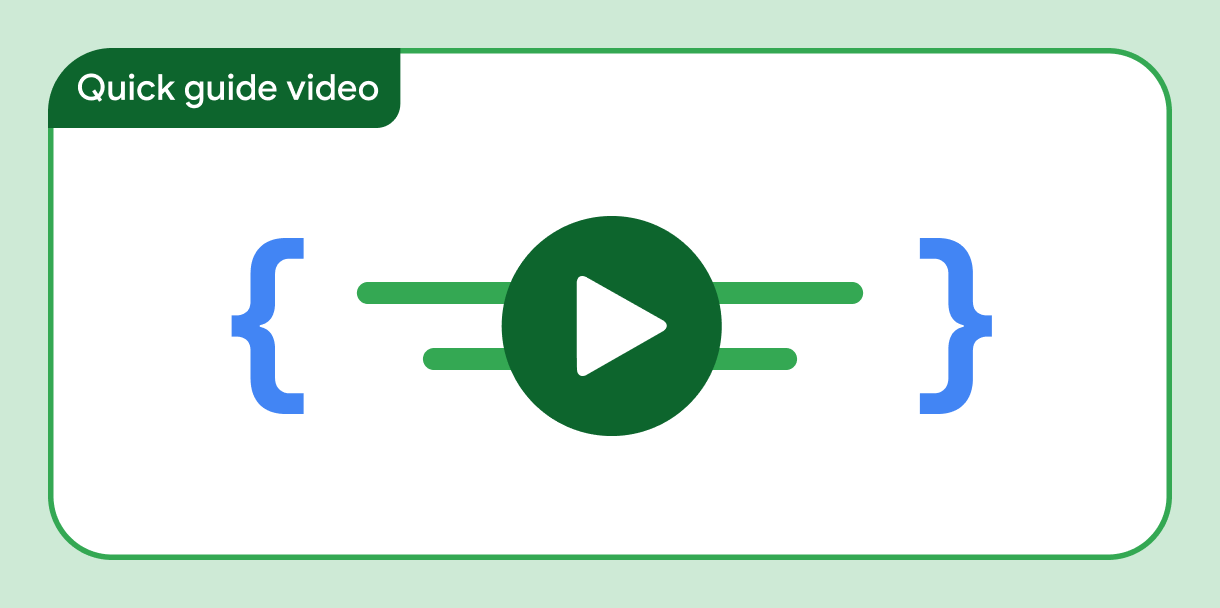
Advanced layout concepts
13 minutes
See how to build complex designs for your Compose layouts, focusing on layout phase and constraints, subcompose layouts, and intrinsic measurements.
Have questions or feedback
Go to our frequently asked questions page and learn about quick guides or reach out and let us know your thoughts.