“快速指南”集合
显示图片
使用矢量、位图或利用屏幕上的画布直接绘制,在屏幕上处理图片。
加载和显示图片
使用 Coil 或 Glide 从磁盘或互联网将图片加载到应用中。
显示动画图片
显示动画图片,用于显示加载动画和在用户执行操作后提供反馈的图标。
在画布上显示分层图片
混合或叠加源图片以显示分层图片。
显示裁剪为某个形状的图片
将图片裁剪为某个形状,以自定义图片在应用中的显示方式。
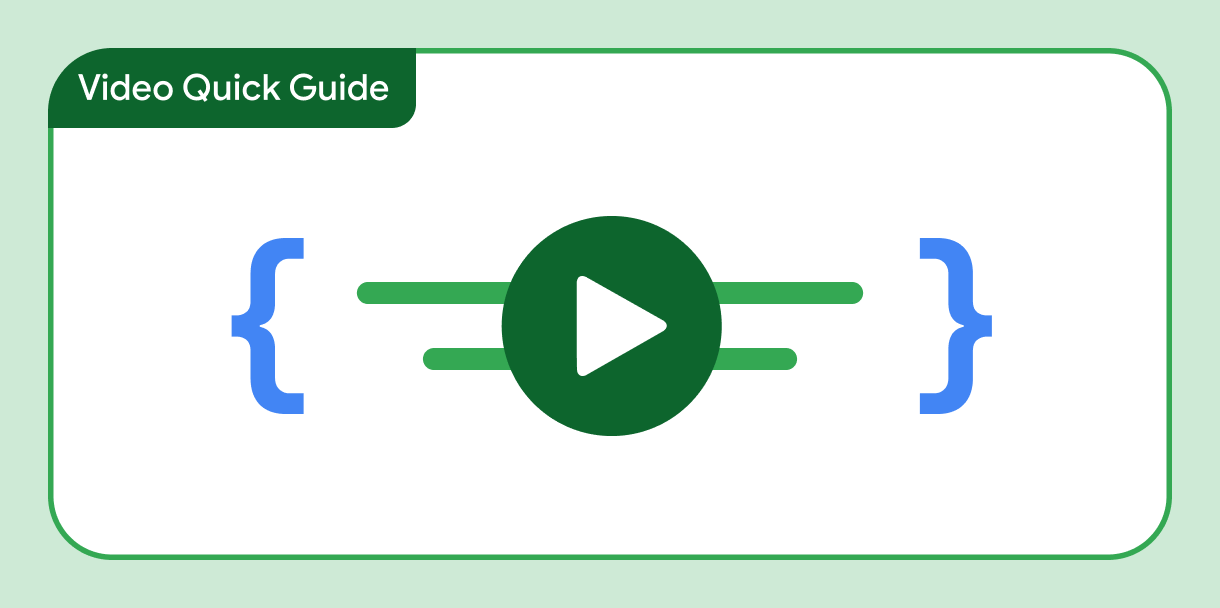
5 个简短动画
7 分钟
这 5 个简单易用的动画可帮助您在短短几分钟内让应用生动起来。即使您没有时间了解有关动画的所有知识,也能让您的 Compose 应用脱颖而出。
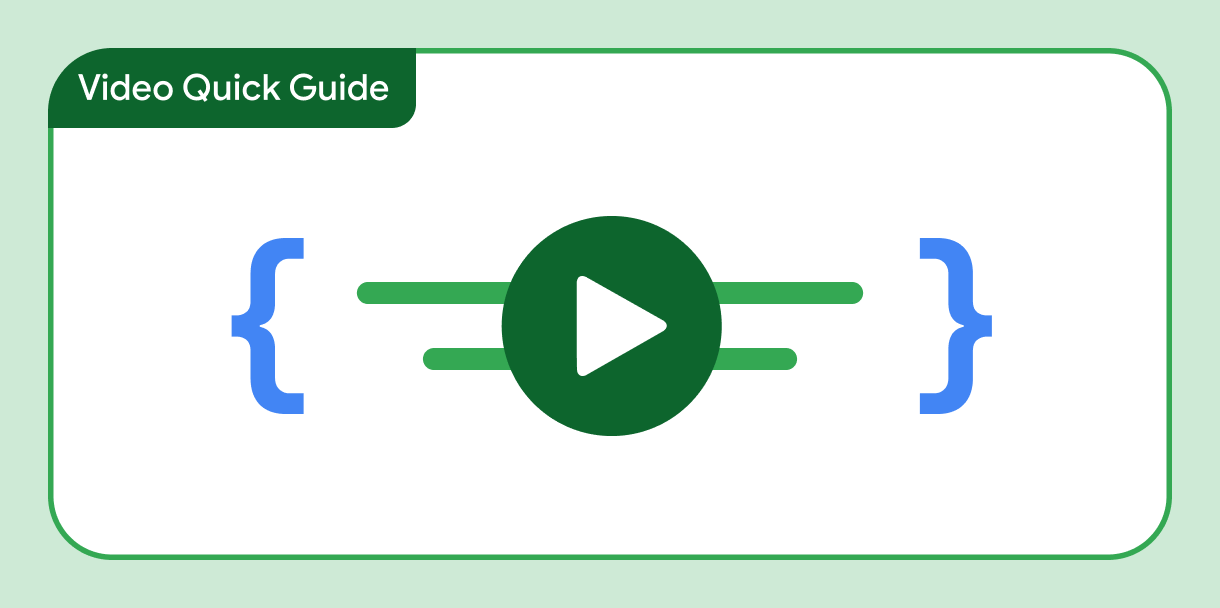
绘制简介
9 分钟
在 Compose 中熟练工作后,您可能需要开始绘制自己的自定义组件。本视频介绍了如何开始使用自定义绘制。
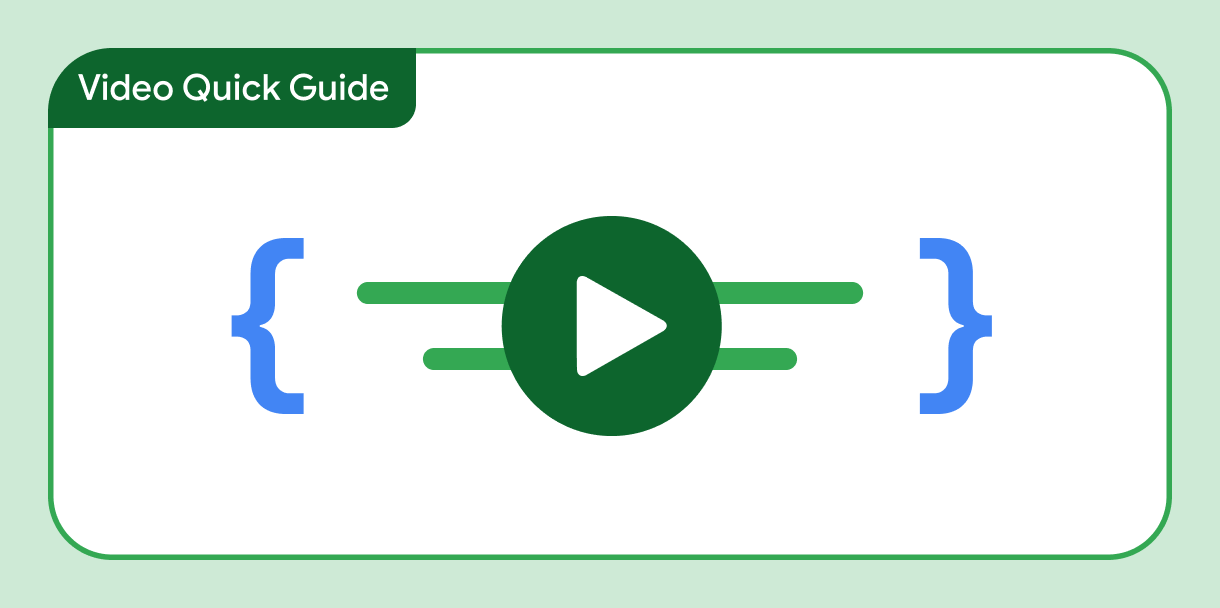
Compose 中的动画
5 分钟
了解如何使用 Compose Animation API 为状态值添加动画效果、使用转场效果、为可见性或大小变化添加动画效果,以及添加简单的淡化效果。
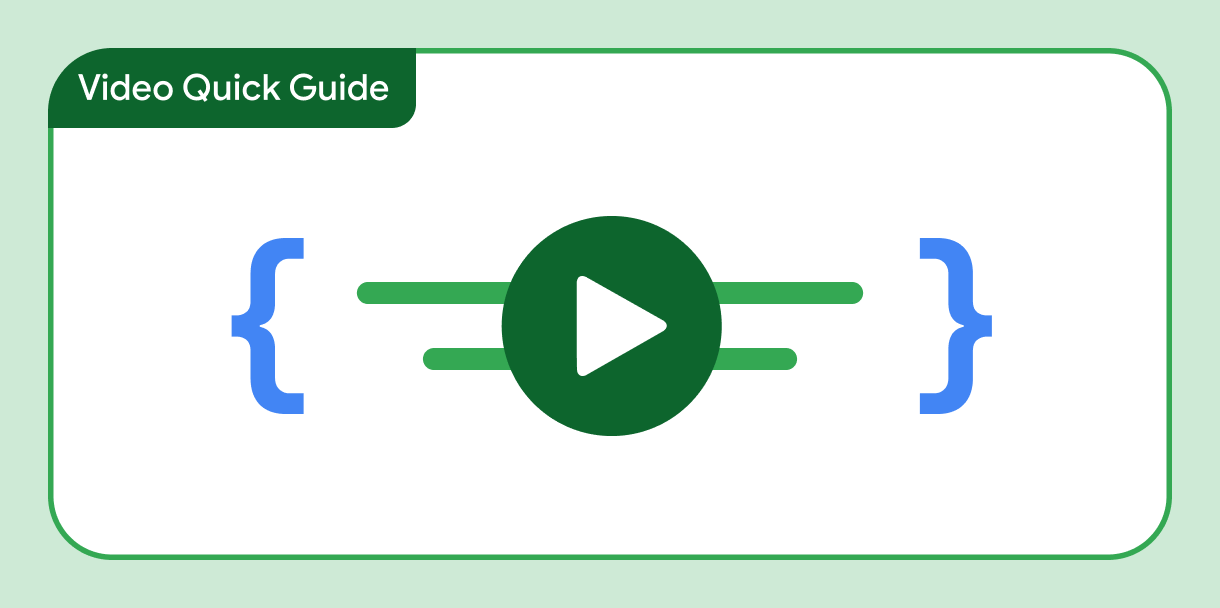
在 Compose 中绘制 ClickableText
5 分钟
了解如何使用专为在画布上绘制文本而设计的 Compose API。此代码段显示了用于在圆角矩形中绘制表情符号字体的代码。
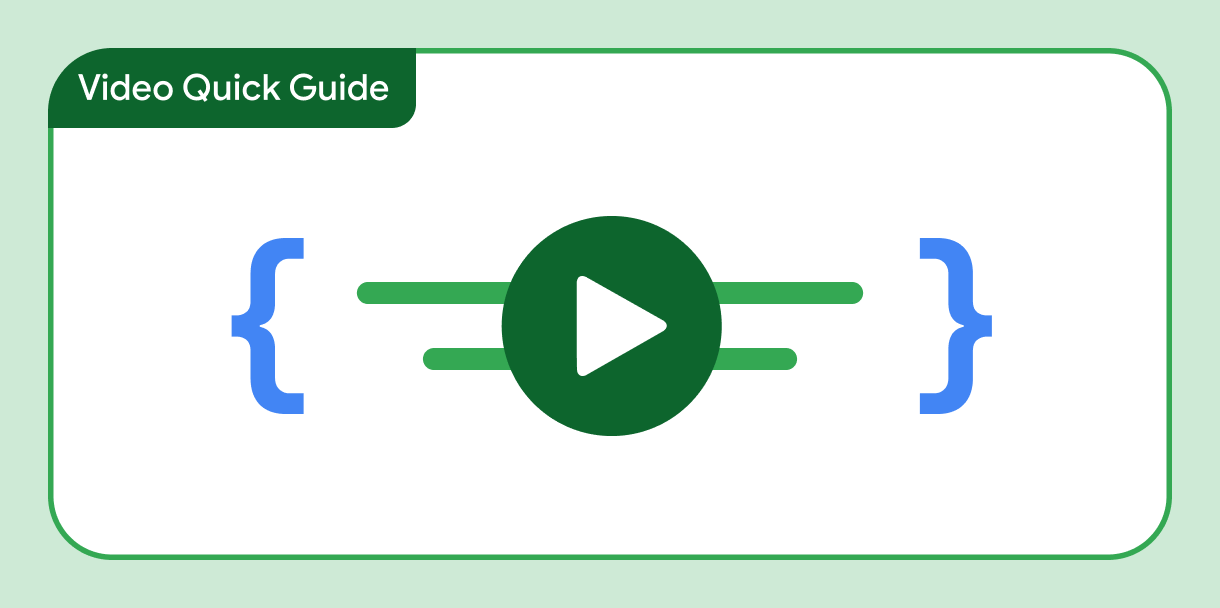
在 Compose 中绘制文本
2 分钟
了解如何使用专为在画布上绘制文本而设计的 Compose API。此代码段显示了用于在圆角矩形中绘制表情符号字体的代码。
有问题或反馈
请访问我们的常见问题解答页面,了解简短指南,或与我们联系,告诉我们您的想法。