Display text Collection
Enhance your app's usability and aesthetic appeal by customizing the way it displays text.
@Composable fun MultipleStylesInText() { Text( buildAnnotatedString { withStyle(style = SpanStyle(color = Color.Blue)) { append("H") } append("ello ") withStyle(style = SpanStyle(fontWeight = FontWeight.Bold, color = Color.Red)) { append("W") } append("orld") } ) }
Style parts of text
Style parts of text to increase readability and enhance user experience.
@Composable fun AnnotatedClickableText() { val annotatedText = buildAnnotatedString { append("Go to ") // We attach this *URL* annotation to the following content // until `pop()` is called pushStringAnnotation( tag = "URL", annotation = "https://developer.android.com" ) withStyle( style = SpanStyle( color = Color.Green, fontWeight = FontWeight.Bold ) ) { append("Android Developers") } pop() append(" and check the ") pushStringAnnotation( tag = "URL", annotation = "https://developer.android.com/jetpack/compose" ) withStyle( style = SpanStyle( color = Color.Blue, fontWeight = FontWeight.Bold ) ) { append("Compose guidelines.") } pop() } ClickableText(text = annotatedText, onClick = { offset -> annotatedText.getStringAnnotations( tag = "URL", start = offset, end = offset ).firstOrNull()?.let { annotation -> // If yes, we log its value Log.d("Clicked URL", annotation.item) } }) }
Support multiple links in a single string of text
Support multiple links in a single string of text to give users options where to go and increase engagement.
@Composable fun LetterByLetterAnimatedText() { val text = "This text animates as though it is being typed \uD83E\uDDDE\u200D♀\uFE0F \uD83D\uDD10 \uD83D\uDC69\u200D❤\uFE0F\u200D\uD83D\uDC68 \uD83D\uDC74\uD83C\uDFFD" // Iterate over the characters. val breakIterator = remember(text) { BreakIterator.getCharacterInstance() } // Define the duration (milliseconds) of the pause before each successive // character is displayed. These pauses between characters create the // illusion of an animation. val typingDelayInMs = 50L var substringText by remember { mutableStateOf("") } LaunchedEffect(text) { // Initial start delay of the typing animation delay(1000) breakIterator.text = StringCharacterIterator(text) var nextIndex = breakIterator.next() // Iterate over the string, by index boundary while (nextIndex != BreakIterator.DONE) { substringText = text.subSequence(0, nextIndex).toString() // Go to the next logical character boundary nextIndex = breakIterator.next() delay(typingDelayInMs) } } Text(substringText) }
Animate text character-by-character as the user type
Animate text character-by-character as the user types to improve readability and user engagement.
@Composable fun DialogExamples() { // ... val openAlertDialog = remember { mutableStateOf(false) } // ... when { // ... openAlertDialog.value -> { AlertDialogExample( onDismissRequest = { openAlertDialog.value = false }, onConfirmation = { openAlertDialog.value = false println("Confirmation registered") // Add logic here to handle confirmation. }, dialogTitle = "Alert dialog example", dialogText = "This is an example of an alert dialog with buttons.", icon = Icons.Default.Info ) } } } }
Display pop-up messages or request user input
Dialogs display pop-up messages or request user input on a layer above the main app content.
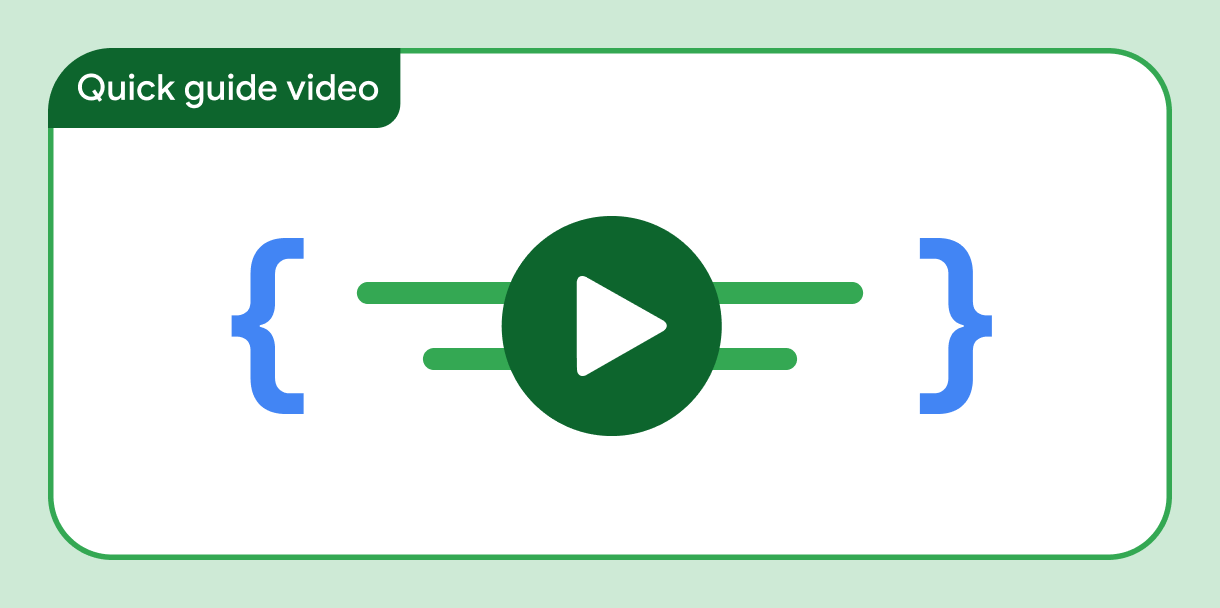
Draw text in Compose
1 minute
See how to use Compose APIs specifically designed to draw text on a canvas. This segment shows the code to draw an emoji font in a rounded rectangle.
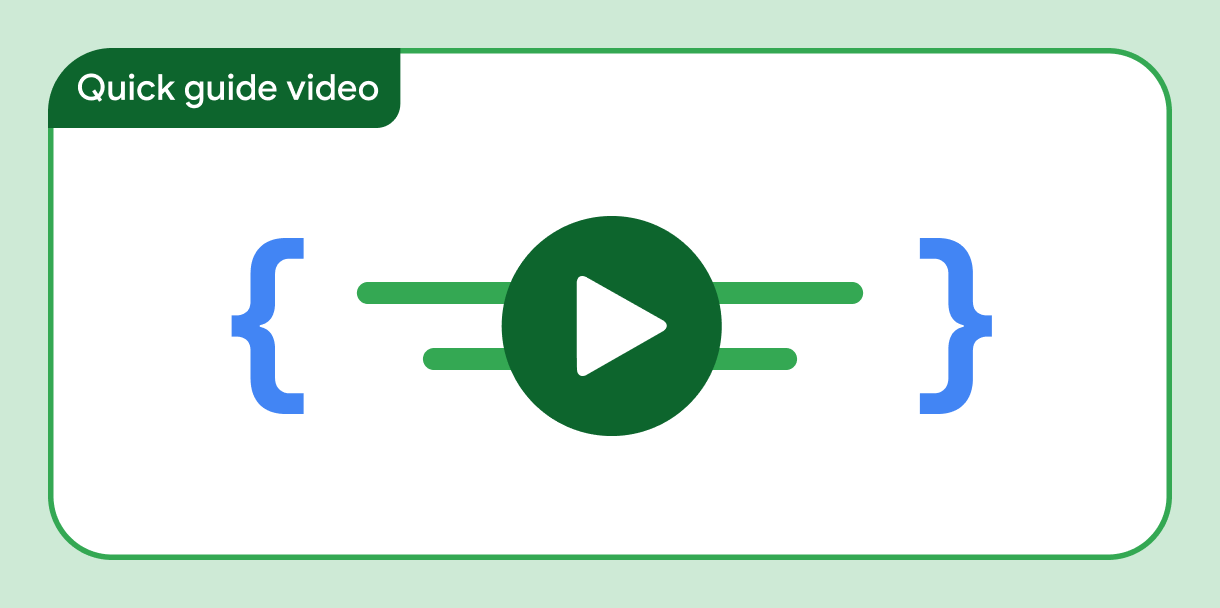
Accessibility in Compose
5 minutes
Add accessibility features to your app, transforming what's shown on screen to a more fitting format for users with specific needs. See how to increase your app's reach and versatility with a small amount of work.
Have questions or feedback
Go to our frequently asked questions page and learn about quick guides or reach out and let us know your thoughts.