快速指南集合
显示交互式组件
为界面选择合适的组件,并了解如何在应用中实现该组件。
创建一个框架组件来将界面整合在一起
一种基本结构,可为复杂的界面提供标准化平台。基架可将界面的不同部分(例如应用栏和悬浮操作按钮)整合在一起,从而让应用具有一致的外观和风格。
显示应用栏
容器可让用户访问关键功能和导航项。应用栏分为两种类型:顶部应用栏和底部应用栏。
创建按钮
借助这些基本组件,用户可以触发定义的操作。按钮有 5 种类型。查看这五种按钮类型的外观,以及应在何处使用它们,并了解如何实现它们。
创建悬浮操作按钮 (FAB
一种高强调度按钮,可让用户在应用中执行主要操作。此按钮组件用于宣传单个重点操作,该操作是用户最常采用的路径,通常固定在屏幕的右下角。
创建一个用作容器的卡片
充当界面的 Material Design 容器。卡片通常用于呈现单个连贯的内容。卡片与其他容器的区别在于,它侧重于展示单一内容。
创建用于表示复杂实体的条状标签
一种紧凑的互动式界面元素,用于表示联系人或标签等复杂实体,通常带有图标和标签。条状标签可以是可选中、可关闭或可点击的。
显示弹出式消息或请求用户输入
在主应用内容之上的层上显示弹出式消息或请求用户输入。此组件会创建干扰性界面体验,以吸引用户注意力。
创建进度指示器
直观地显示操作状态。使用动画来提醒用户进度(例如数据加载或处理)即将完成,这些指示器可以表明正在进行处理,但不表明即将完成。
为一系列值创建滑块
让用户能够从连续范围内的一系列值中进行选择,例如设置音量或过滤价格范围内的图表数据。
添加用户可以切换的开关
允许用户在两种状态之间切换。用户可以拖动或点击开关的滑块来更改当前状态。
创建底部动作条
显示固定在屏幕底部的辅助内容。您可以使用标准底部动作条或模态底部动作条向用户显示次要内容。
使用抽屉式导航栏组件创建滑入式菜单
滑入式菜单,可让用户导航到应用的各个部分。用户可以通过从侧边滑动或点按菜单图标来激活它。
创建带有动作条的通知
在屏幕底部显示一条简短的通知。这样,您就可以在不中断用户体验的情况下提供有关操作或操作的反馈。动作条会在几秒钟后消失。用户也可以手动关闭这些通知。
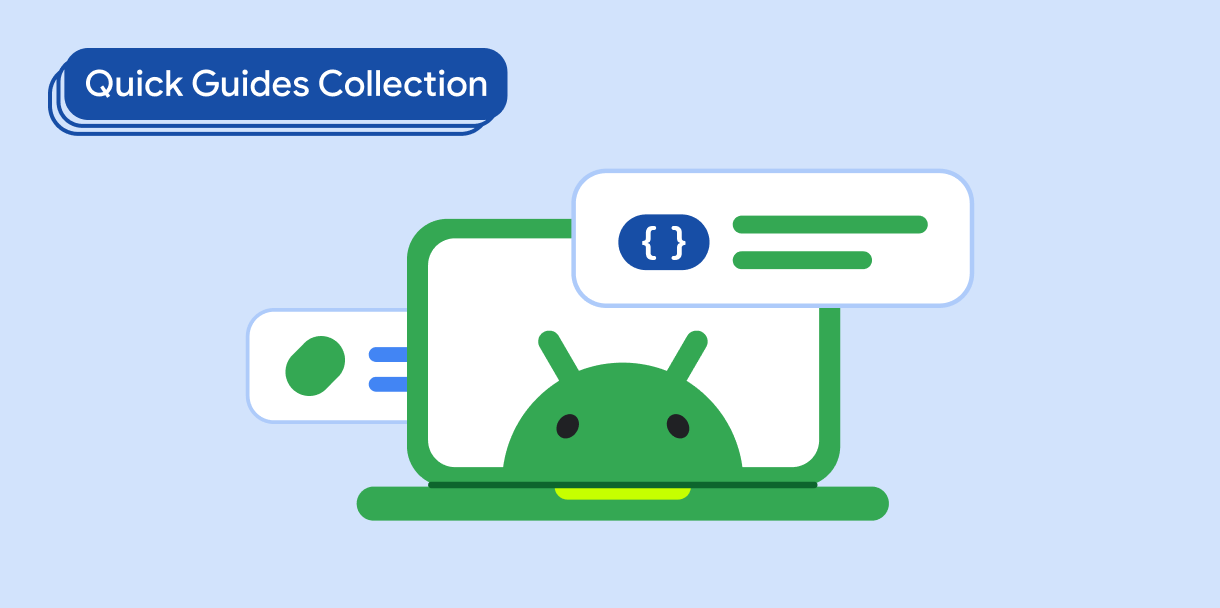
显示列表或网格
使用列表和网格高效地显示和排列一组项。
有问题或反馈
请访问我们的常见问题解答页面,了解简短指南,或与我们联系,告诉我们您的想法。