The Android system triggers a configuration change every time a keyboard is attached to or detached from a device. To ensure a seamless user experience and maximize user productivity on large screen devices with detachable keyboards, your app needs to effectively manage keyboard configuration changes.
Prevent activity recreation on keyboard change
To prevent your activity from being recreated when a detachable keyboard is
attached or detached, add keyboard-related values to the configChanges
attribute of your app manifest and add a view to the activity's view hierarchy
so your app can listen for configuration changes.
1. Declare the configChanges
attribute
Update the <activity>
element in the app manifest by adding the
keyboard|keyboardHidden
values to the list of already managed configuration
changes:
<activity
...
android:configChanges="...|keyboard|keyboardHidden">
2. Add an empty view to the view hierarchy
Declare a new view and add your handler code inside the view's
onConfigurationChanged()
method:
Kotlin
val v = object : View(this) { override fun onConfigurationChanged(newConfig: Configuration?) { super.onConfigurationChanged(newConfig) // Handler code here. } }
Java
View v = new View(this) { @Override protected void onConfigurationChanged(Configuration newConfig) { super.onConfigurationChanged(newConfig); // Handler code here. } };
Key points
android:configChanges
: Attribute of the app manifest's<activity>
element. Informs the system about configuration changes the app manages.View#onConfigurationChanged()
: Method that reacts to propagation of a new app configuration.
Results
Your app now responds to an external keyboard being attached or detached without recreating the running activity.
Collections that contain this guide
This guide is part of these curated Quick Guide collections that cover broader Android development goals:
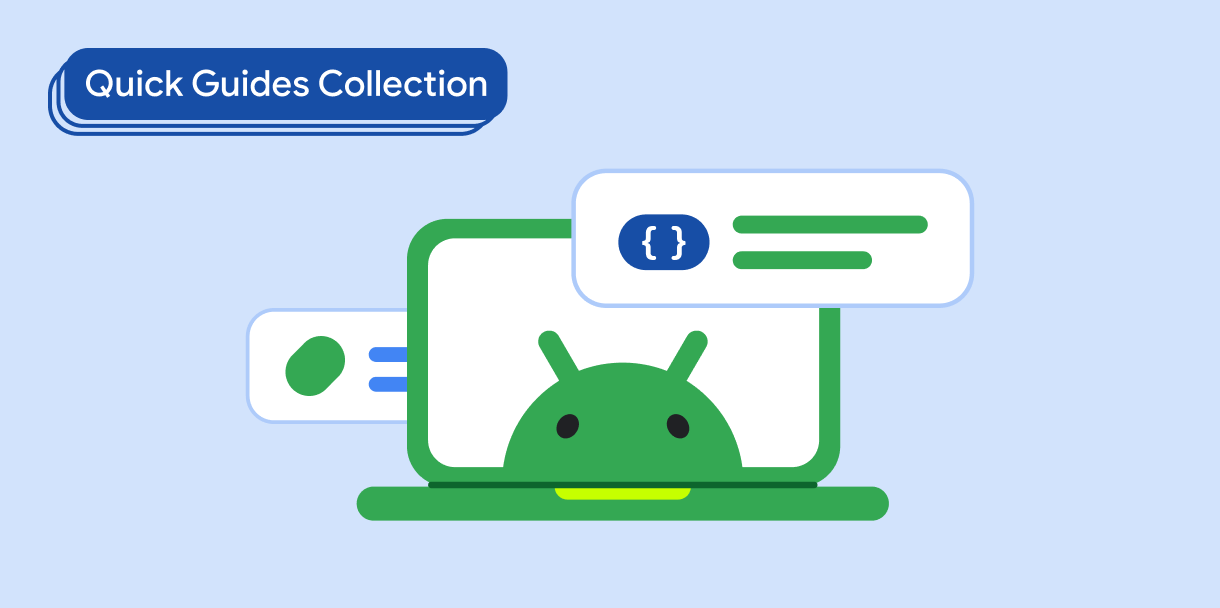