本文档介绍了如何将 Credential Manager API 与使用 WebView 的 Android 应用相集成。
概览
在深入了解集成过程之前,请务必了解原生 Android 代码、在 WebView 中呈现的 Web 组件(用于管理应用身份验证)和后端之间的通信流。该流程涉及注册(创建凭据)和身份验证(获取现有凭据)。
注册(创建通行密钥)
- 后端生成初始注册 JSON 并将其发送到在 WebView 中呈现的网页。
- 网页使用
navigator.credentials.create()
注册新凭据。您将在后续步骤中使用注入的 JavaScript 替换此方法,以便将请求发送到 Android 应用。 - Android 应用使用 Credential Manager API 来构建凭据请求,并将其用于
createCredential
。 - Credential Manager API 与应用共享公钥凭据。
- 应用将公钥凭据发送回网页,以便注入的 JavaScript 可以解析响应。
- 网页将公钥发送到后端,后端会验证并保存公钥。
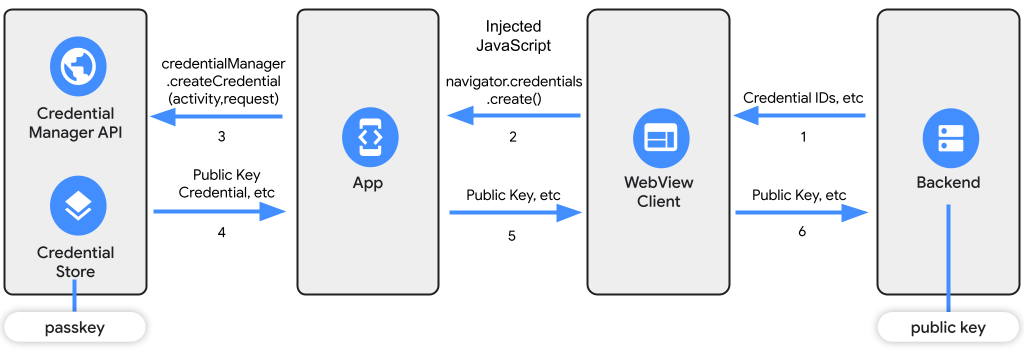
身份验证(获取通行密钥)
- 后端生成身份验证 JSON 以获取凭据,并将此凭据发送到在 WebView 客户端中呈现的网页。
- 网页使用
navigator.credentials.get
。使用注入的 JavaScript 替换此方法,将请求重定向到 Android 应用。 - 应用通过调用
getCredential
来使用 Credential Manager API 检索凭据。 - Credential Manager API 会将凭据返回给应用。
- 应用获得私钥的数字签名并将其发送到网页,以便注入的 JavaScript 可以解析响应。
- 然后,网页将其发送到使用公钥验证数字签名的服务器。
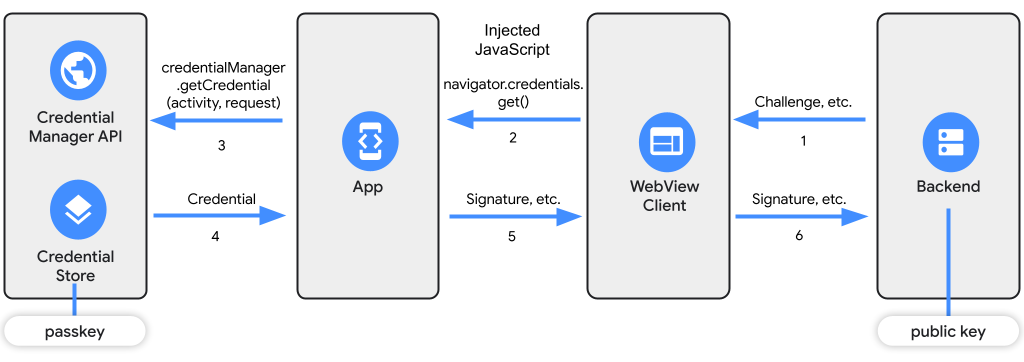
此流程也可用于密码或联合用户身份系统。
前提条件
如需使用 Credential Manager API,请完成 Credential Manager 指南的前提条件部分中列出的步骤,并务必执行以下操作:
JavaScript 通信
如需允许 WebView 中的 JavaScript 与原生 Android 代码相互通信,您需要发送消息和处理这两种环境之间的请求。为此,请将自定义 JavaScript 代码注入 WebView。这样,您就可以修改 Web 内容的行为并与原生 Android 代码交互。
JavaScript 注入
以下 JavaScript 代码可在 WebView 与 Android 应用之间建立通信。它会替换 WebAuthn API 使用的 navigator.credentials.create()
和 navigator.credentials.get()
方法,以便执行上文所述的注册和身份验证流程。
在您的应用中使用此 JavaScript 代码的精简版。
为通行密钥创建监听器
设置用于处理与 JavaScript 的通信的 PasskeyWebListener
类。此类应从 WebViewCompat.WebMessageListener
继承。此类接收来自 JavaScript 的消息,并在 Android 应用中执行必要的操作。
Kotlin
// The class talking to Javascript should inherit:
class PasskeyWebListener(
private val activity: Activity,
private val coroutineScope: CoroutineScope,
private val credentialManagerHandler: CredentialManagerHandler
) : WebViewCompat.WebMessageListener
// ... Implementation details
Java
// The class talking to Javascript should inherit:
class PasskeyWebListener implements WebViewCompat.WebMessageListener {
// Implementation details
private Activity activity;
// Handles get/create methods meant for Java:
private CredentialManagerHandler credentialManagerHandler;
public PasskeyWebListener(
Activity activity,
CredentialManagerHandler credentialManagerHandler
) {
this.activity = activity;
this.credentialManagerHandler = credentialManagerHandler;
}
// ... Implementation details
}
在 PasskeyWebListener
中,按照下面几个部分所述的内容实现请求和响应的逻辑。
处理身份验证请求
为了处理 WebAuthn navigator.credentials.create()
或 navigator.credentials.get()
操作的请求,当 JavaScript 代码向 Android 应用发送消息时,系统会调用 PasskeyWebListener
类的 onPostMessage
方法:
Kotlin
class PasskeyWebListener(...)... {
// ...
/** havePendingRequest is true if there is an outstanding WebAuthn request.
There is only ever one request outstanding at a time. */
private var havePendingRequest = false
/** pendingRequestIsDoomed is true if the WebView has navigated since
starting a request. The FIDO module cannot be canceled, but the response
will never be delivered in this case. */
private var pendingRequestIsDoomed = false
/** replyChannel is the port that the page is listening for a response on.
It is valid if havePendingRequest is true. */
private var replyChannel: ReplyChannel? = null
/**
* Called by the page during a WebAuthn request.
*
* @param view Creates the WebView.
* @param message The message sent from the client using injected JavaScript.
* @param sourceOrigin The origin of the HTTPS request. Should not be null.
* @param isMainFrame Should be set to true. Embedded frames are not
supported.
* @param replyProxy Passed in by JavaScript. Allows replying when wrapped in
the Channel.
* @return The message response.
*/
@UiThread
override fun onPostMessage(
view: WebView,
message: WebMessageCompat,
sourceOrigin: Uri,
isMainFrame: Boolean,
replyProxy: JavaScriptReplyProxy,
) {
val messageData = message.data ?: return
onRequest(
messageData,
sourceOrigin,
isMainFrame,
JavaScriptReplyChannel(replyProxy)
)
}
private fun onRequest(
msg: String,
sourceOrigin: Uri,
isMainFrame: Boolean,
reply: ReplyChannel,
) {
msg?.let {
val jsonObj = JSONObject(msg);
val type = jsonObj.getString(TYPE_KEY)
val message = jsonObj.getString(REQUEST_KEY)
if (havePendingRequest) {
postErrorMessage(reply, "The request already in progress", type)
return
}
replyChannel = reply
if (!isMainFrame) {
reportFailure("Requests from subframes are not supported", type)
return
}
val originScheme = sourceOrigin.scheme
if (originScheme == null || originScheme.lowercase() != "https") {
reportFailure("WebAuthn not permitted for current URL", type)
return
}
// Verify that origin belongs to your website,
// it's because the unknown origin may gain credential info.
if (isUnknownOrigin(originScheme)) {
return
}
havePendingRequest = true
pendingRequestIsDoomed = false
// Use a temporary "replyCurrent" variable to send the data back, while
// resetting the main "replyChannel" variable to null so it’s ready for
// the next request.
val replyCurrent = replyChannel
if (replyCurrent == null) {
Log.i(TAG, "The reply channel was null, cannot continue")
return;
}
when (type) {
CREATE_UNIQUE_KEY ->
this.coroutineScope.launch {
handleCreateFlow(credentialManagerHandler, message, replyCurrent)
}
GET_UNIQUE_KEY -> this.coroutineScope.launch {
handleGetFlow(credentialManagerHandler, message, replyCurrent)
}
else -> Log.i(TAG, "Incorrect request json")
}
}
}
private suspend fun handleCreateFlow(
credentialManagerHandler: CredentialManagerHandler,
message: String,
reply: ReplyChannel,
) {
try {
havePendingRequest = false
pendingRequestIsDoomed = false
val response = credentialManagerHandler.createPasskey(message)
val successArray = ArrayList<Any>();
successArray.add("success");
successArray.add(JSONObject(response.registrationResponseJson));
successArray.add(CREATE_UNIQUE_KEY);
reply.send(JSONArray(successArray).toString())
replyChannel = null // setting initial replyChannel for the next request
} catch (e: CreateCredentialException) {
reportFailure(
"Error: ${e.errorMessage} w type: ${e.type} w obj: $e",
CREATE_UNIQUE_KEY
)
} catch (t: Throwable) {
reportFailure("Error: ${t.message}", CREATE_UNIQUE_KEY)
}
}
companion object {
const val TYPE_KEY = "type"
const val REQUEST_KEY = "request"
const val CREATE_UNIQUE_KEY = "create"
const val GET_UNIQUE_KEY = "get"
}
}
Java
class PasskeyWebListener implements ... {
// ...
/**
* Called by the page during a WebAuthn request.
*
* @param view Creates the WebView.
* @param message The message sent from the client using injected JavaScript.
* @param sourceOrigin The origin of the HTTPS request. Should not be null.
* @param isMainFrame Should be set to true. Embedded frames are not
supported.
* @param replyProxy Passed in by JavaScript. Allows replying when wrapped in
the Channel.
* @return The message response.
*/
@UiThread
public void onPostMessage(
@NonNull WebView view,
@NonNull WebMessageCompat message,
@NonNull Uri sourceOrigin,
Boolean isMainFrame,
@NonNull JavaScriptReplyProxy replyProxy,
) {
if (messageData == null) {
return;
}
onRequest(
messageData,
sourceOrigin,
isMainFrame,
JavaScriptReplyChannel(replyProxy)
)
}
private void onRequest(
String msg,
Uri sourceOrigin,
boolean isMainFrame,
ReplyChannel reply
) {
if (msg != null) {
try {
JSONObject jsonObj = new JSONObject(msg);
String type = jsonObj.getString(TYPE_KEY);
String message = jsonObj.getString(REQUEST_KEY);
boolean isCreate = type.equals(CREATE_UNIQUE_KEY);
boolean isGet = type.equals(GET_UNIQUE_KEY);
if (havePendingRequest) {
postErrorMessage(reply, "The request already in progress", type);
return;
}
replyChannel = reply;
if (!isMainFrame) {
reportFailure("Requests from subframes are not supported", type);
return;
}
String originScheme = sourceOrigin.getScheme();
if (originScheme == null || !originScheme.toLowerCase().equals("https")) {
reportFailure("WebAuthn not permitted for current URL", type);
return;
}
// Verify that origin belongs to your website,
// Requests of unknown origin may gain access to credential info.
if (isUnknownOrigin(originScheme)) {
return;
}
havePendingRequest = true;
pendingRequestIsDoomed = false;
// Use a temporary "replyCurrent" variable to send the data back,
// while resetting the main "replyChannel" variable to null so it’s
// ready for the next request.
ReplyChannel replyCurrent = replyChannel;
if (replyCurrent == null) {
Log.i(TAG, "The reply channel was null, cannot continue");
return;
}
if (isCreate) {
handleCreateFlow(credentialManagerHandler, message, replyCurrent));
} else if (isGet) {
handleGetFlow(credentialManagerHandler, message, replyCurrent));
} else {
Log.i(TAG, "Incorrect request json");
}
} catch (JSONException e) {
e.printStackTrace();
}
}
}
}
如需了解 handleCreateFlow
和 handleGetFlow
,请参阅 GitHub 上的示例。
处理响应
如需处理从原生应用发送到网页的响应,请在 JavaScriptReplyChannel
中添加 JavaScriptReplyProxy
。
Kotlin
class PasskeyWebListener(...)... {
// ...
// The setup for the reply channel allows communication with JavaScript.
private class JavaScriptReplyChannel(private val reply: JavaScriptReplyProxy) :
ReplyChannel {
override fun send(message: String?) {
try {
reply.postMessage(message!!)
} catch (t: Throwable) {
Log.i(TAG, "Reply failure due to: " + t.message);
}
}
}
// ReplyChannel is the interface where replies to the embedded site are
// sent. This allows for testing since AndroidX bans mocking its objects.
interface ReplyChannel {
fun send(message: String?)
}
}
Java
class PasskeyWebListener implements ... {
// ...
// The setup for the reply channel allows communication with JavaScript.
private static class JavaScriptReplyChannel implements ReplyChannel {
private final JavaScriptReplyProxy reply;
JavaScriptReplyChannel(JavaScriptReplyProxy reply) {
this.reply = reply;
}
@Override
public void send(String message) {
reply.postMessage(message);
}
}
// ReplyChannel is the interface where replies to the embedded site are
// sent. This allows for testing since AndroidX bans mocking its objects.
interface ReplyChannel {
void send(String message);
}
}
请务必捕获原生应用中的所有错误,并将其发送回 JavaScript 端。
与 WebView 集成
本部分介绍了如何设置 WebView 集成。
初始化 WebView
在 Android 应用的 activity 中,初始化 WebView
并设置相应的 WebViewClient
。WebViewClient
会处理与注入 WebView
中的 JavaScript 代码的通信。
设置 WebView 并调用 Credential Manager:
Kotlin
val credentialManagerHandler = CredentialManagerHandler(this)
// ...
AndroidView(factory = {
WebView(it).apply {
settings.javaScriptEnabled = true
// Test URL:
val url = "https://credman-web-test.glitch.me/"
val listenerSupported = WebViewFeature.isFeatureSupported(
WebViewFeature.WEB_MESSAGE_LISTENER
)
if (listenerSupported) {
// Inject local JavaScript that calls Credential Manager.
hookWebAuthnWithListener(this, this@MainActivity,
coroutineScope, credentialManagerHandler)
} else {
// Fallback routine for unsupported API levels.
}
loadUrl(url)
}
}
)
Java
// Example shown in the onCreate method of an Activity
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
WebView webView = findViewById(R.id.web_view);
// Test URL:
String url = "https://credman-web-test.glitch.me/";
Boolean listenerSupported = WebViewFeature.isFeatureSupported(
WebViewFeature.WEB_MESSAGE_LISTENER
);
if (listenerSupported) {
// Inject local JavaScript that calls Credential Manager.
hookWebAuthnWithListener(webView, this,
coroutineScope, credentialManagerHandler)
} else {
// Fallback routine for unsupported API levels.
}
webView.loadUrl(url);
}
创建一个新的 WebView 客户端对象,并将 JavaScript 注入网页:
Kotlin
// This is an example call into hookWebAuthnWithListener
val passkeyWebListener = PasskeyWebListener(
activity, coroutineScope, credentialManagerHandler
)
val webViewClient = object : WebViewClient() {
override fun onPageStarted(view: WebView?, url: String?, favicon: Bitmap?) {
super.onPageStarted(view, url, favicon)
// Handle page load events
passkeyWebListener.onPageStarted();
webView.evaluateJavascript(PasskeyWebListener.INJECTED_VAL, null)
}
}
webView.webViewClient = webViewClient
Java
// This is an example call into hookWebAuthnWithListener
PasskeyWebListener passkeyWebListener = new PasskeyWebListener(
activity, credentialManagerHandler
)
WebViewClient webiewClient = new WebViewClient() {
@Override
public void onPageStarted(WebView view, String url, Bitmap favicon) {
super.onPageStarted(view, url, favicon);
// Handle page load events
passkeyWebListener.onPageStarted();
webView.evaulateJavascript(PasskeyWebListener.INJECTED_VAL, null);
}
};
webView.setWebViewClient(webViewClient);
设置 Web 消息监听器
如需允许在 JavaScript 和 Android 应用之间发布消息,请使用 WebViewCompat.addWebMessageListener
方法设置 Web 消息监听器。
Kotlin
val rules = setOf("*")
if (WebViewFeature.isFeatureSupported(WebViewFeature.WEB_MESSAGE_LISTENER)) {
WebViewCompat.addWebMessageListener(
webView, PasskeyWebListener.INTERFACE_NAME, rules, passkeyWebListener
)
}
Java
Set<String> rules = new HashSet<>(Arrays.asList("*"));
if (WebViewFeature.isFeatureSupported(WebViewFeature.WEB_MESSAGE_LISTENER)) {
WebViewCompat.addWebMessageListener(
webView, PasskeyWebListener.INTERFACE_NAME, rules, passkeyWebListener
)
}
Web 集成
如需了解如何构建 Web 集成,请参阅为无密码登录创建通行密钥和通过表单自动填充功能使用通行密钥登录。
测试和部署
在受控环境中全面测试整个流程,以确保 Android 应用、网页和后端之间能够正常通信。
将集成解决方案部署到生产环境中,确保后端可以处理传入的注册和身份验证请求。后端代码应生成初始 JSON 以用于注册 (create) 和身份验证 (get) 流程。它还应处理对从网页收到的响应的确认和验证。
确认实现与用户体验建议相符。
重要提示
- 使用提供的 JavaScript 代码处理
navigator.credentials.create()
和navigator.credentials.get()
操作。 PasskeyWebListener
类是 Android 应用与 WebView 中的 JavaScript 代码之间的桥梁。它负责处理消息传递、通信和执行所需操作。- 调整提供的代码段,以适应您的项目结构、命名惯例和您可能有的任何特定要求。
- 捕获原生应用端的错误,并将其发送回 JavaScript 端。
按照本指南中的说明操作,将 Credential Manager API 集成到使用 WebView 的 Android 应用中,即可让用户安全、顺畅地获享已启用通行密钥的登录体验,同时有效管理用户的凭据。