Set up unit tests
This section provides examples of how to set up unit tests to check that your app interacts with keyed app states as expected.
Step 1: Set up your classes to accept KeyedAppStatesReporter
as a parameter
Instead of calling create()
directly, modify your classes to accept
KeyedAppStatesReporter
as a parameter like in the example BatteryManager
class below:
Kotlin
class BatteryManager(val reporter:KeyedAppStatesReporter) { fun lowBattery(battery:Int) { reporter.setStatesImmediate( hashSetOf(KeyedAppState.builder() .setKey("battery") .setSeverity(KeyedAppState.SEVERITY_INFO) .setMessage("Battery is low") .setData(battery.toString()) .build())) } }
Java
public class BatteryManager { private final KeyedAppStatesReporter reporter; public BatteryManager(KeyedAppStatesReporter reporter) { this.reporter = reporter; } public void lowBattery(int battery) { final Collectionstates = new HashSet<>(); states.add(KeyedAppState.builder() .setKey("battery") .setSeverity(KeyedAppState.SEVERITY_INFO) .setMessage("Battery is low") .setData(Integer.toString(battery)) .build(); reporter.setStatesImmediate(states); } }
Next, use KeyedAppStatesReporter.create
to get an instance to pass
wherever BatteryManager
is created.
Step 2: Add the enterprise feedback testing library to your build.gradle
file
Add the following dependency to your app's
build.gradle
file:
dependencies { testImplementation 'androidx.enterprise:enterprise-feedback-testing:1.0.0' }
Step 3: Create a FakeKeyedAppStatesReporter
and pass it into your class
Kotlin
val reporter = FakeKeyedAppStatesReporter(); val batteryManager = BatteryManager(reporter);
Java
FakeKeyedAppStatesReporter reporter = new FakeKeyedAppStatesReporter(); BatteryManager batteryManager = new BatteryManager(reporter);
Step 4: Assert interactions with FakeKeyedAppStatesReporter
For example, to check that no states have been set:
Kotlin
assertThat(reporter.keyedAppStates).isEmpty();
Java
assertThat(reporter.getKeyedAppStates()).isEmpty();
Or that a particular state has been requested to be uploaded:
Kotlin
assertThat(reporter.uploadedKeyedAppStatesByKey["battery"]).isNotNull()
Java
assertThat(reporter.getUploadedKeyedAppStatesByKey().get("battery")).isNotNull();
Send test feedback to Test DPC
A sample device policy controller, called Test DPC, is capable of receiving app feedback and is available for download.
Step 1: Install Test DPC
Install the latest version of Test DPC from the Play Store. Next, set Test DPC as the admin of the device:
adb shell dpm set-device-owner com.afwsamples.testdpc/.DeviceAdminReceiver
Step 2: Enable App feedback notifications
In Test DPC's menu, enable App feedback notifications.
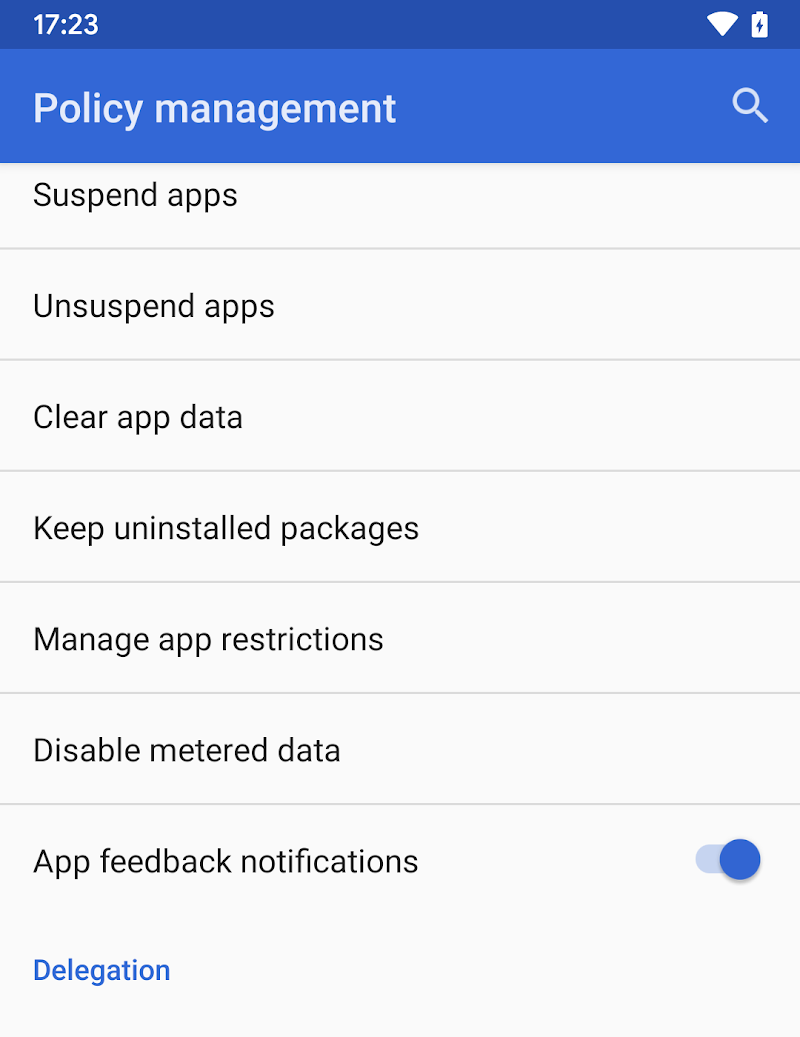
Trigger an event that set a keyed app state. If successful, Test DPC will show the feedback in notifications:
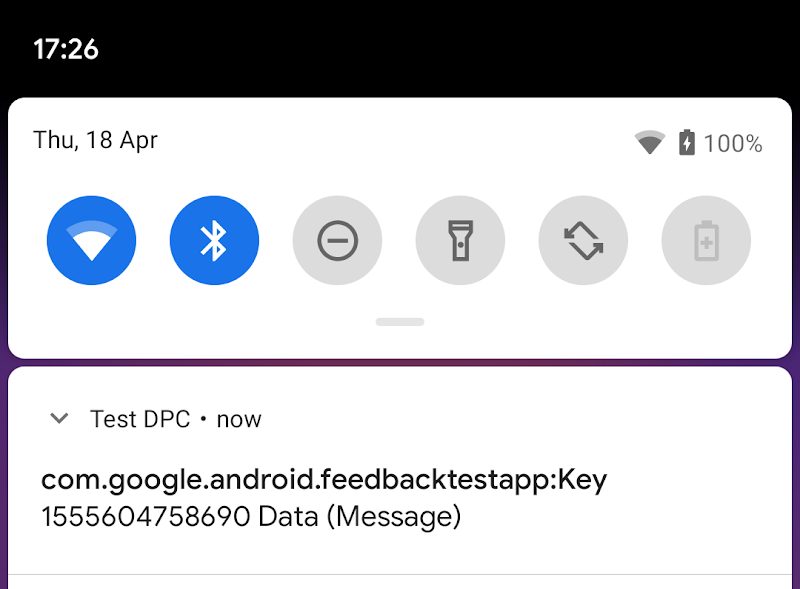