微调框提供了一种方法,可让用户从值集内快速选择一个值。默认情况下 状态,旋转图标会显示其当前所选的值。点按旋转图标 会显示一个菜单,其中显示用户可以选择的所有其他值。
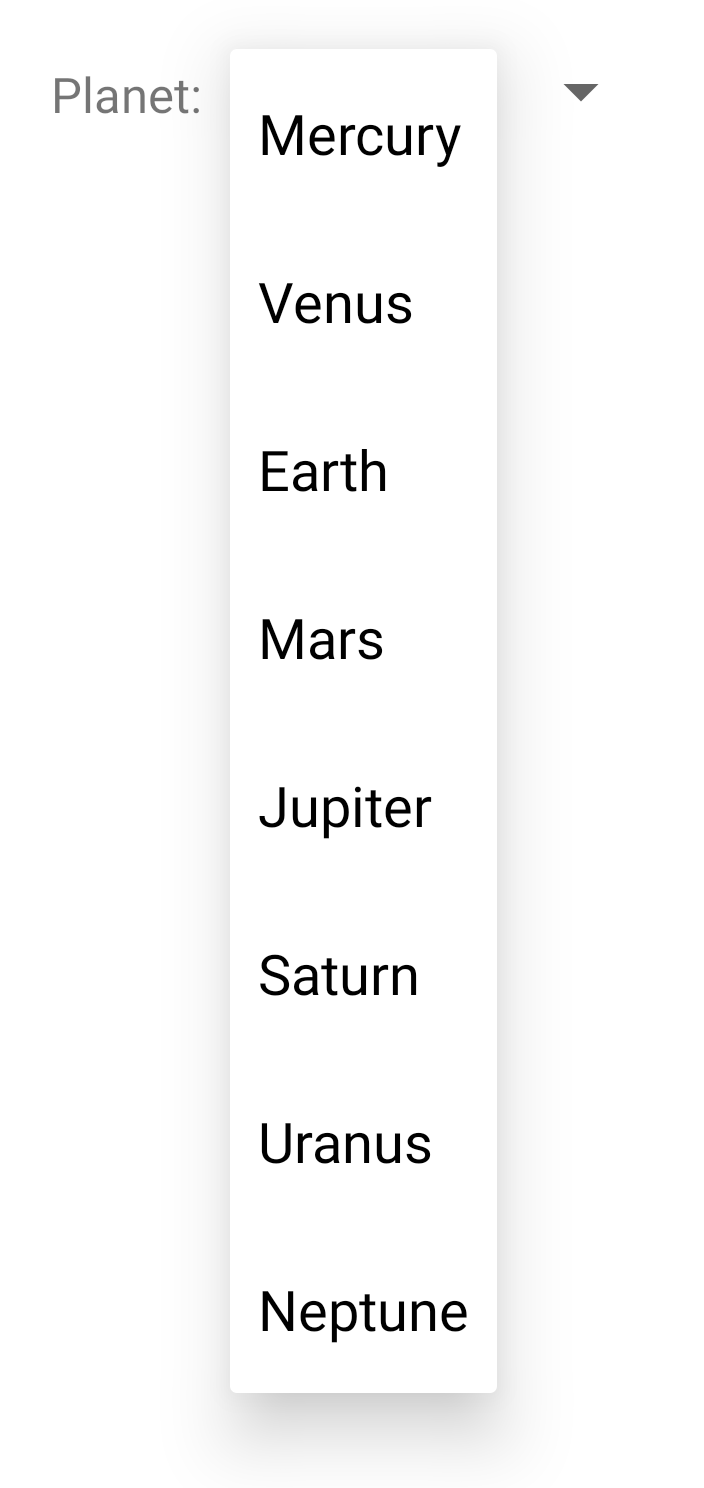
您可以使用
Spinner
对象,您通常在 XML 布局中
<Spinner>
元素。具体可见于
示例:
<Spinner
android:id="@+id/planets_spinner"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
要使用选项列表填充旋转图标,请指定
SpinnerAdapter
在您的
Activity
或
Fragment
源代码。
如果您使用 Material Design 组件
实验组
下拉菜单相当于 Spinner
。
使用用户选项填充旋转图标
您为旋转图标提供的选项可能来自任何来源,但您可以
必须通过 SpinnerAdapter
(例如
ArrayAdapter
如果选项以数组或
CursorAdapter
如果可从数据库查询中获取选项,则会发生此错误。
例如,如果预先确定了微调框的可用选项, 您可以为其提供在 字符串资源 文件:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string-array name="planets_array">
<item>Mercury</item>
<item>Venus</item>
<item>Earth</item>
<item>Mars</item>
<item>Jupiter</item>
<item>Saturn</item>
<item>Uranus</item>
<item>Neptune</item>
</string-array>
</resources>
对于这样的数组,您可以在
Activity
或 Fragment
,用于为旋转图标提供
数组(使用 ArrayAdapter
实例):
val spinner: Spinner = findViewById(R.id.planets_spinner)
// Create an ArrayAdapter using the string array and a default spinner layout.
ArrayAdapter.createFromResource(
this,
R.array.planets_array,
android.R.layout.simple_spinner_item
).also { adapter ->
// Specify the layout to use when the list of choices appears.
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item)
// Apply the adapter to the spinner.
spinner.adapter = adapter
}
Spinner spinner = (Spinner) findViewById(R.id.planets_spinner);
// Create an ArrayAdapter using the string array and a default spinner layout.
ArrayAdapter<CharSequence> adapter = ArrayAdapter.createFromResource(
this,
R.array.planets_array,
android.R.layout.simple_spinner_item
);
// Specify the layout to use when the list of choices appears.
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
// Apply the adapter to the spinner.
spinner.setAdapter(adapter);
通过
createFromResource()
方法允许您基于字符串数组创建 ArrayAdapter
。通过
此方法的第三个参数是布局资源,用于定义
选中的选项会显示在旋转图标控件中。该平台为
simple_spinner_item
布局。这是默认布局,除非您想为
旋转图标外观。
致电
setDropDownViewResource(int)
可指定适配器用于显示旋转图标选项列表的布局。
simple_spinner_dropdown_item
是平台定义的另一种标准布局
致电
setAdapter()
将适配器应用到 Spinner
。
响应用户的选择
当用户从旋转图标的菜单中选择某个项目时,
Spinner
个对象
会收到一个 on-item-selected 事件。
要为旋转图标定义选择事件处理脚本,请实现
AdapterView.OnItemSelectedListener
和相应的
onItemSelected()
回调方法。例如,下面是 接口的
Activity
:
class SpinnerActivity : Activity(), AdapterView.OnItemSelectedListener {
...
override fun onItemSelected(parent: AdapterView<*>, view: View?, pos: Int, id: Long) {
// An item is selected. You can retrieve the selected item using
// parent.getItemAtPosition(pos).
}
override fun onNothingSelected(parent: AdapterView<*>) {
// Another interface callback.
}
}
public class SpinnerActivity extends Activity implements OnItemSelectedListener {
...
public void onItemSelected(AdapterView<?> parent, View view,
int pos, long id) {
// An item is selected. You can retrieve the selected item using
// parent.getItemAtPosition(pos).
}
public void onNothingSelected(AdapterView<?> parent) {
// Another interface callback.
}
}
通过
AdapterView.OnItemSelectedListener
接口需要
onItemSelected()
和
onNothingSelected()
回调方法。
通过调用
setOnItemSelectedListener()
:
val spinner: Spinner = findViewById(R.id.planets_spinner)
spinner.onItemSelectedListener = this
Spinner spinner = (Spinner) findViewById(R.id.planets_spinner);
spinner.setOnItemSelectedListener(this);
如果您实现 AdapterView.OnItemSelectedListener
与您的 Activity
或 Fragment
交互,如
对于前面的示例,您可以将 this
作为接口实例传递。