Using page indicators, you can help your users understand their current position within your app's content, providing a visual indication of progress. These navigational cues are useful when you present content sequentially, such as carousel implementations, image galleries and slideshows, or pagination in lists.
Version compatibility
This implementation requires that your project minSDK be set to API level 21 or higher.
Dependencies
Create a horizontal pager with a custom page indicator
The following code creates a horizontal pager that has a page indicator, giving the user visual cues for how many pages there are and which page is visible:
val pagerState = rememberPagerState(pageCount = { 4 }) HorizontalPager( state = pagerState, modifier = Modifier.fillMaxSize() ) { page -> // Our page content Text( text = "Page: $page", ) } Row( Modifier .wrapContentHeight() .fillMaxWidth() .align(Alignment.BottomCenter) .padding(bottom = 8.dp), horizontalArrangement = Arrangement.Center ) { repeat(pagerState.pageCount) { iteration -> val color = if (pagerState.currentPage == iteration) Color.DarkGray else Color.LightGray Box( modifier = Modifier .padding(2.dp) .clip(CircleShape) .background(color) .size(16.dp) ) } }
Key points about the code
- The code implements a
HorizontalPager
, which lets you swipe horizontally between different pages of content. In this case, each page displays the page number. - The
rememberPagerState()
function initializes the pager and sets the number of pages to 4. This function creates a state object that tracks the current page, and lets you control the pager. - The
pagerState.currentPage
property is used to determine which page indicator should be highlighted. - The page indictator appears in a pager overlaid by a
Row
implementation. - A 16 dp circle is drawn for each page in the pager. The indicator for the
current page is displayed as
DarkGray
, while the rest of the indicator circles areLightGray
. - The
Text
composable within theHorizontalPager
displays the text "Page: [page number]" on each page.
Results
Collections that contain this guide
This guide is part of these curated Quick Guide collections that cover broader Android development goals:
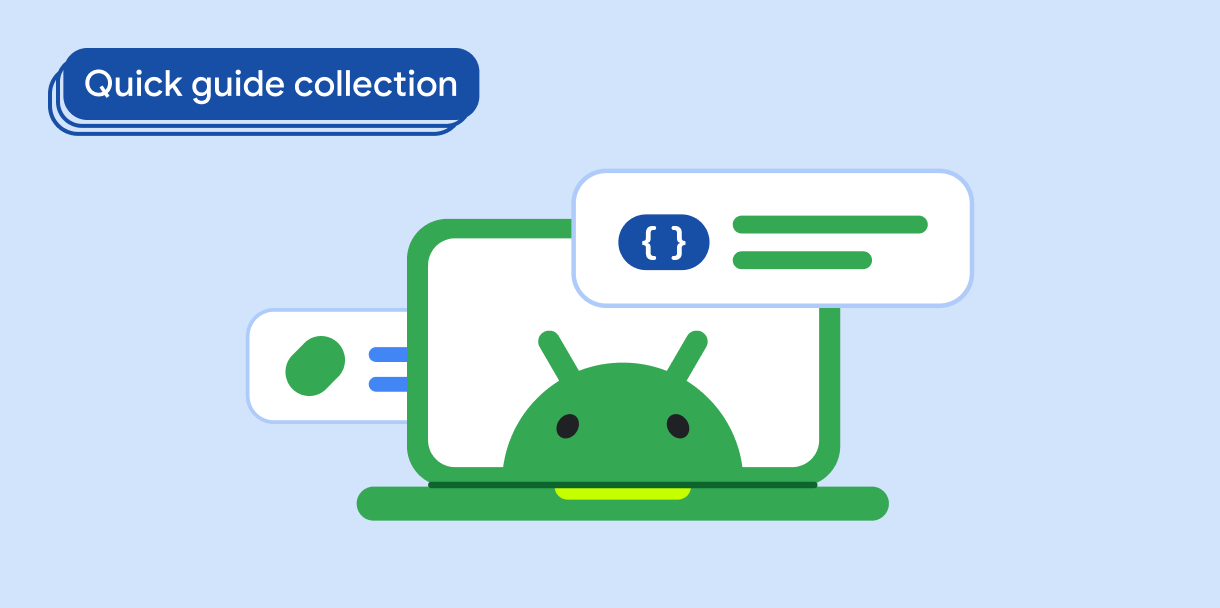
Display a list or grid
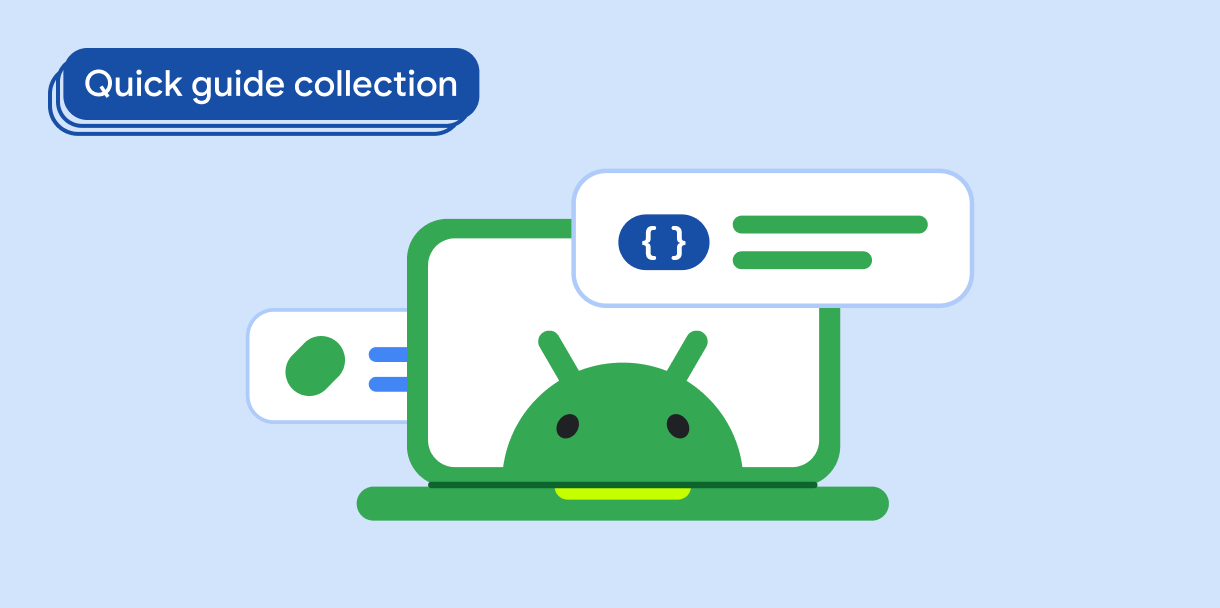