アプリから Gemini API と Gemini ファミリーのモデルに直接アクセスするには、Android 向け Firebase SDK の Vertex AI を使用することをおすすめします。この SDK は、フルスタック アプリの構築と実行に役立つ、より大きな Firebase プラットフォームの一部です。
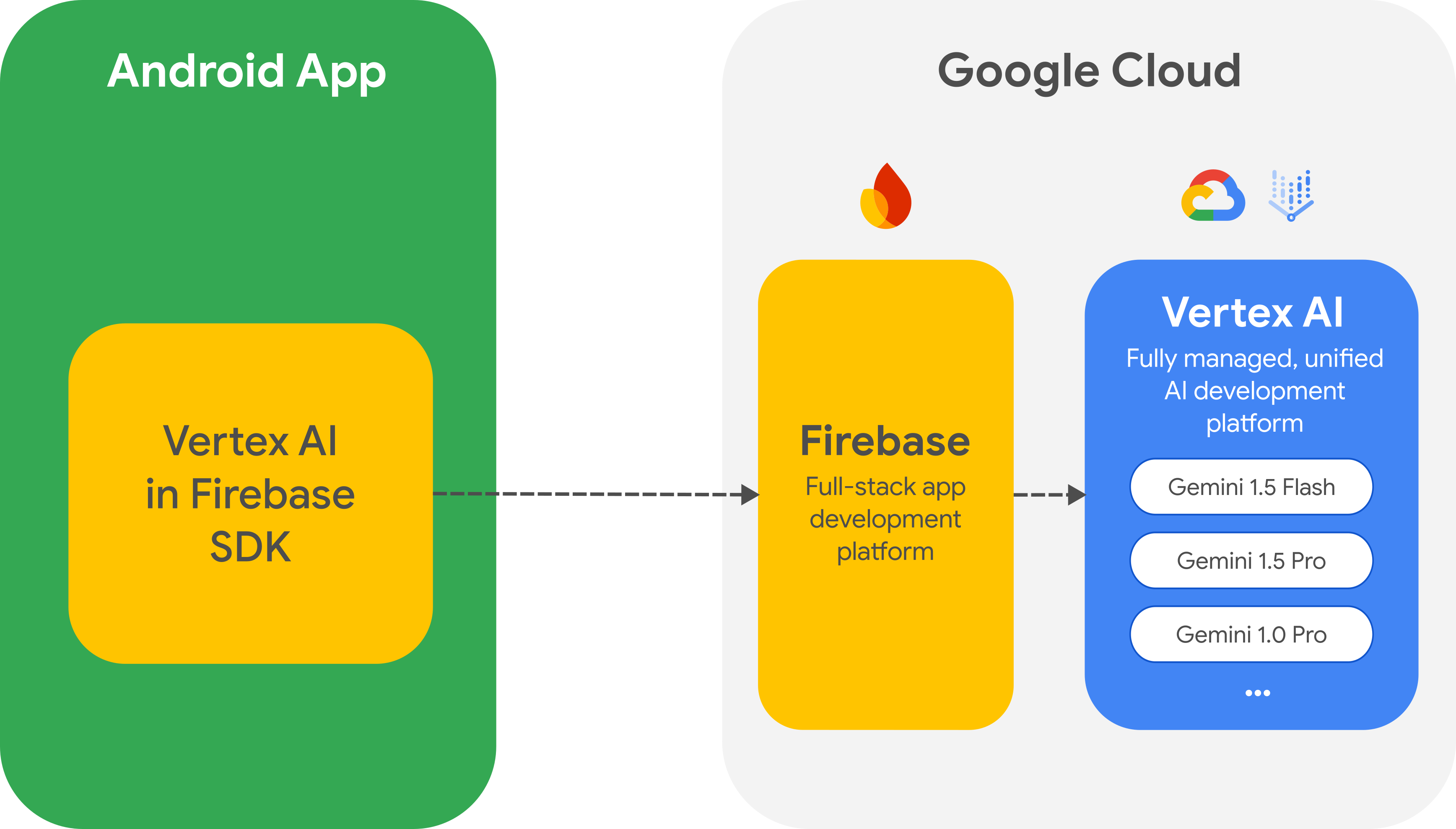
Google AI クライアント SDK から移行する
Vertex AI in Firebase SDK は Google AI クライアント SDK に似ていますが、Vertex AI in Firebase SDK には、本番環境のユースケース向けの重要なセキュリティ オプションやその他の機能が用意されています。たとえば、Firebase で Vertex AI を使用する場合は、次のものも使用できます。
Firebase App Check: 未承認のクライアントによる Gemini API の不正使用を防ぎます。
Firebase Remote Config: アプリの新しいバージョンをリリースしなくても、クラウドでアプリの値(モデル名など)を動的に設定、変更できます。
Cloud Storage for Firebase: Gemini API へのリクエストに大容量のメディア ファイルを含める。
Google AI クライアント SDK をアプリに統合済みの場合は、Firebase の Vertex AI に移行できます。
はじめに
アプリから Gemini API を直接操作する前に、プロンプトの使用方法を学び、Firebase とアプリを設定して SDK を使用できるようにする必要があります。
プロンプトで試す
Vertex AI Studio でプロンプトを試すことができます。Vertex AI Studio は、プロンプトの設計とプロトタイピング用の IDE です。ファイルをアップロードしてテキストと画像を含むプロンプトをテストし、プロンプトを保存して後で再利用できます。
ユースケースに適したプロンプトを作成することは、科学というよりは芸術に近く、そのためテストが重要になります。プロンプトについて詳しくは、Firebase のドキュメントをご覧ください。
Firebase プロジェクトを設定してアプリを Firebase に接続する
アプリから Gemini API を呼び出す準備ができたら、Vertex AI in Firebase のスタートガイドの手順に沿って、アプリに Firebase と SDK を設定します。このスタートガイドでは、このガイドの以降のすべてのタスクを行うことができます。
従量課金制の Blaze 料金プランの使用や必要な API の有効化など、新規または既存の Firebase プロジェクトを設定します。
アプリを Firebase に接続して、アプリの登録や Firebase 構成ファイル(
google-services.json
)のアプリへの追加などを行います。
Gradle 依存関係を追加する
アプリ モジュールに次の Gradle 依存関係を追加します。
Kotlin
dependencies { ... implementation("com.google.firebase:firebase-vertexai:16.0.2") }
Java
dependencies { [...] implementation("com.google.firebase:firebase-vertexai:16.0.2") // Required to use `ListenableFuture` from Guava Android for one-shot generation implementation("com.google.guava:guava:31.0.1-android") // Required to use `Publisher` from Reactive Streams for streaming operations implementation("org.reactivestreams:reactive-streams:1.0.4") }
Vertex AI サービスと生成モデルを初期化する
まず、GenerativeModel
をインスタンス化し、モデル名を指定します。
Kotlin
val generativeModel = Firebase.vertexAI.generativeModel("gemini-1.5-flash")
Java
GenerativeModel gm = FirebaseVertexAI.getInstance().generativeModel("gemini-1.5-flash");
Firebase のドキュメントでは、Vertex AI in Firebase で使用できるモデルの詳細を確認できます。モデル パラメータの構成についても確認してください。
アプリから Gemini API を操作する
Firebase とアプリを設定して SDK を使用できるようになったので、アプリから Gemini API を操作できるようになりました。
テキストを生成
テキスト レスポンスを生成するには、プロンプトを使用して generateContent()
を呼び出します。
Kotlin
// Note: `generateContent()` is a `suspend` function, which integrates well // with existing Kotlin code. scope.launch { val response = model.generateContent("Write a story about the green robot") }
Java
// In Java, create a `GenerativeModelFutures` from the `GenerativeModel`. // Note that `generateContent()` returns a `ListenableFuture`. Learn more: // https://developer.android.com/develop/background-work/background-tasks/asynchronous/listenablefuture GenerativeModelFutures model = GenerativeModelFutures.from(gm); Content prompt = new Content.Builder() .addText("Write a story about a green robot.") .build(); ListenableFuture<GenerateContentResponse> response = model.generateContent(prompt); Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() { @Override public void onSuccess(GenerateContentResponse result) { String resultText = result.getText(); } @Override public void onFailure(Throwable t) { t.printStackTrace(); } }, executor);
画像やその他のメディアからテキストを生成する
テキストと画像などのメディアを含むプロンプトからテキストを生成することもできます。generateContent()
を呼び出すときに、メディアをインライン データとして渡すことができます(次の例を参照)。または、Firebase 用の Cloud Storage URL を使用して、リクエストに大きなメディア ファイルを含めることもできます。
Kotlin
scope.launch { val response = model.generateContent( content { image(bitmap) text("what is the object in the picture?") } ) }
Java
GenerativeModelFutures model = GenerativeModelFutures.from(gm); Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.sparky); Content prompt = new Content.Builder() .addImage(bitmap) .addText("What developer tool is this mascot from?") .build(); ListenableFuture<GenerateContentResponse> response = model.generateContent(prompt); Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() { @Override public void onSuccess(GenerateContentResponse result) { String resultText = result.getText(); } @Override public void onFailure(Throwable t) { t.printStackTrace(); } }, executor);
マルチターン チャット
マルチターンの会話をサポートすることもできます。startChat()
関数でチャットを初期化します。必要に応じて、メッセージ履歴を指定できます。次に、sendMessage()
関数を呼び出してチャット メッセージを送信します。
Kotlin
val chat = generativeModel.startChat( history = listOf( content(role = "user") { text("Hello, I have 2 dogs in my house.") }, content(role = "model") { text("Great to meet you. What would you like to know?") } ) ) scope.launch { val response = chat.sendMessage("How many paws are in my house?") }
Java
// (Optional) create message history Content.Builder userContentBuilder = new Content.Builder(); userContentBuilder.setRole("user"); userContentBuilder.addText("Hello, I have 2 dogs in my house."); Content userContent = userContentBuilder.build(); Content.Builder modelContentBuilder = new Content.Builder(); modelContentBuilder.setRole("model"); modelContentBuilder.addText("Great to meet you. What would you like to know?"); Content modelContent = userContentBuilder.build(); List<Content> history = Arrays.asList(userContent, modelContent); // Initialize the chat ChatFutures chat = model.startChat(history); // Create a new user message Content.Builder messageBuilder = new Content.Builder(); messageBuilder.setRole("user"); messageBuilder.addText("How many paws are in my house?"); Content message = messageBuilder.build(); Publisher<GenerateContentResponse> streamingResponse = chat.sendMessageStream(message); StringBuilder outputContent = new StringBuilder(); streamingResponse.subscribe(new Subscriber<GenerateContentResponse>() { @Override public void onNext(GenerateContentResponse generateContentResponse) { String chunk = generateContentResponse.getText(); outputContent.append(chunk); } @Override public void onComplete() { // ... } @Override public void onError(Throwable t) { t.printStackTrace(); } @Override public void onSubscribe(Subscription s) { s.request(Long.MAX_VALUE); } });
レスポンスをストリーミングする
モデル生成の結果全体を待たずに、ストリーミングを使用して部分的な結果を処理することで、インタラクションを高速化できます。generateContentStream()
を使用してレスポンスをストリーミングします。
Kotlin
scope.launch { var outputContent = "" generativeModel.generateContentStream(inputContent) .collect { response -> outputContent += response.text } }
Java
// Note that in Java the method `generateContentStream()` returns a // Publisher from the Reactive Streams library. // https://www.reactive-streams.org/ GenerativeModelFutures model = GenerativeModelFutures.from(gm); // Provide a prompt that contains text Content prompt = new Content.Builder() .addText("Write a story about a green robot.") .build(); Publisher<GenerateContentResponse> streamingResponse = model.generateContentStream(prompt); StringBuilder outputContent = new StringBuilder(); streamingResponse.subscribe(new Subscriber<GenerateContentResponse>() { @Override public void onNext(GenerateContentResponse generateContentResponse) { String chunk = generateContentResponse.getText(); outputContent.append(chunk); } @Override public void onComplete() { // ... } @Override public void onError(Throwable t) { t.printStackTrace(); } @Override public void onSubscribe(Subscription s) { s.request(Long.MAX_VALUE); } });
次のステップ
- GitHub の Firebase の Vertex AI サンプルアプリを確認する。
- Firebase App Check の設定など、本番環境への準備を検討します。これにより、不正なクライアントによる Gemini API の不正使用を防ぐことができます。
- Vertex AI in Firebase の詳細については、Firebase のドキュメントをご覧ください。