视差滚动是一种让背景内容和前景内容以不同速度滚动的技术。您可以通过实现此技术来增强应用的界面,在用户滚动时打造更具动态感的体验。
版本兼容性
此实现要求将项目 minSDK 设置为 API 级别 21 或更高级别。
依赖项
创建视差效果
如需实现视差效果,您需要将滚动可组合项的滚动值的一小部分应用于需要视差效果的可组合项。以下代码段会获取两个嵌套的视觉元素(一张图片和一段文本),并以不同的速度在同一方向滚动它们:
@Composable fun ParallaxEffect() { fun Modifier.parallaxLayoutModifier(scrollState: ScrollState, rate: Int) = layout { measurable, constraints -> val placeable = measurable.measure(constraints) val height = if (rate > 0) scrollState.value / rate else scrollState.value layout(placeable.width, placeable.height) { placeable.place(0, height) } } val scrollState = rememberScrollState() Column( modifier = Modifier .fillMaxWidth() .verticalScroll(scrollState), ) { Image( painterResource(id = R.drawable.cupcake), contentDescription = "Android logo", contentScale = ContentScale.Fit, // Reduce scrolling rate by half. modifier = Modifier.parallaxLayoutModifier(scrollState, 2) ) Text( text = stringResource(R.string.detail_placeholder), modifier = Modifier .background(Color.White) .padding(horizontal = 8.dp), ) } }
代码要点
- 创建自定义
layout
修饰符,以调整可组合项滚动的速率。 Image
的滚动速度比Text
慢,这两个可组合项以不同的速率垂直平移,从而产生了视差效果。
结果
包含本指南的集合
本指南属于以下精选快速入门集合,这些集合涵盖了更广泛的 Android 开发目标:
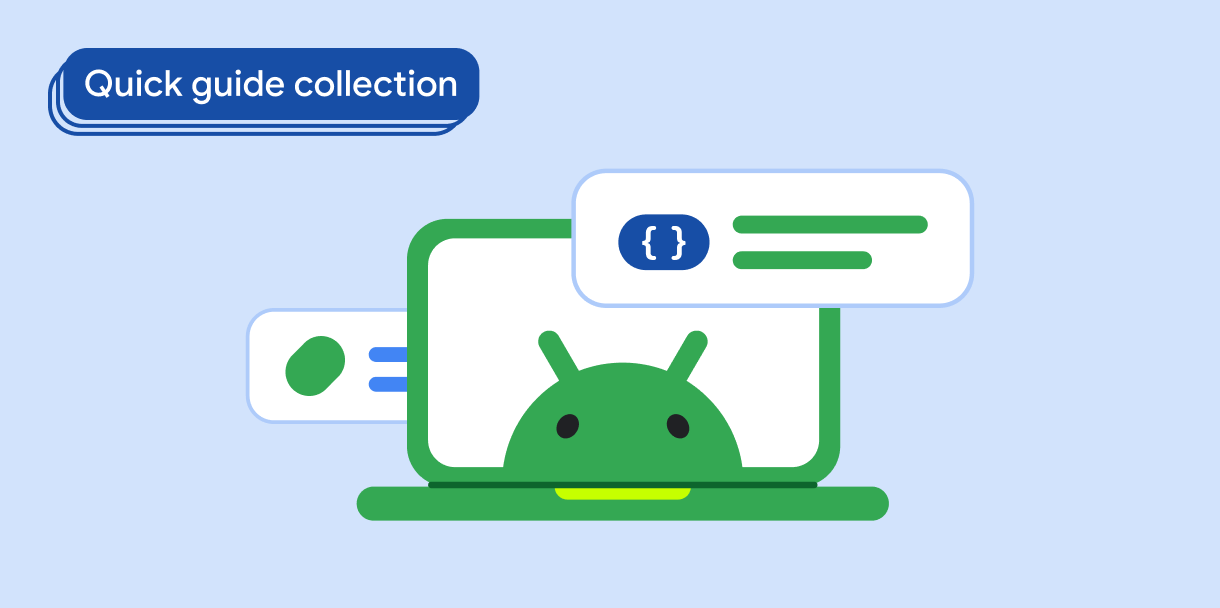
显示列表或网格
借助列表和网格,您的应用可以以视觉上令人愉悦且易于用户使用的形式显示集合。
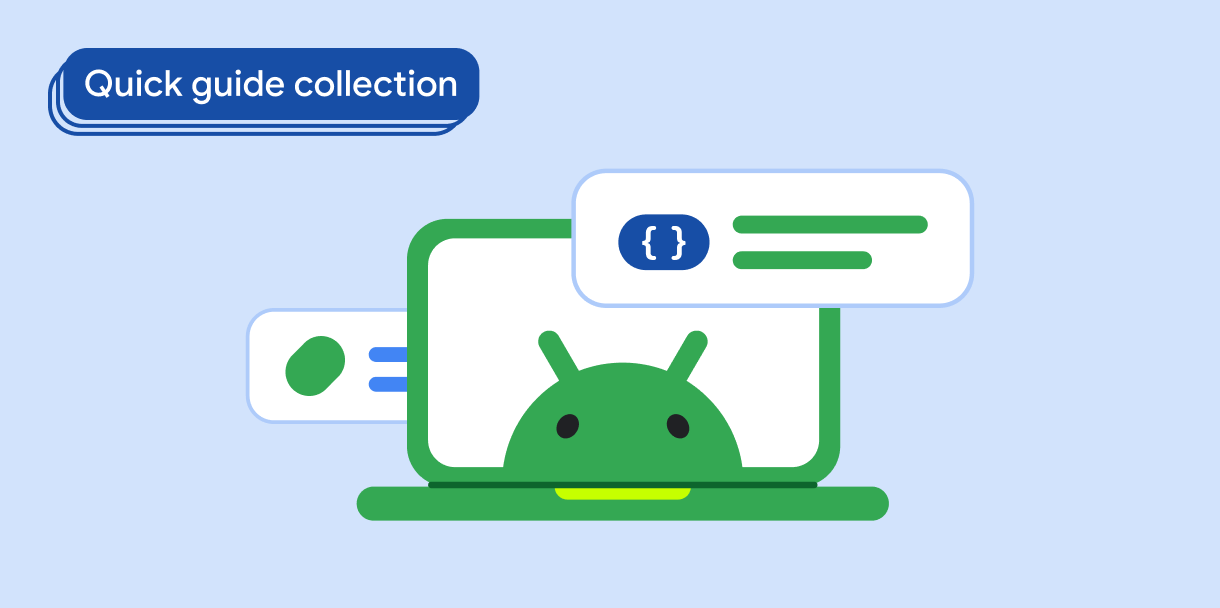
显示图片
了解如何使用明亮动人的视觉元素为 Android 应用打造美观的外观和风格。
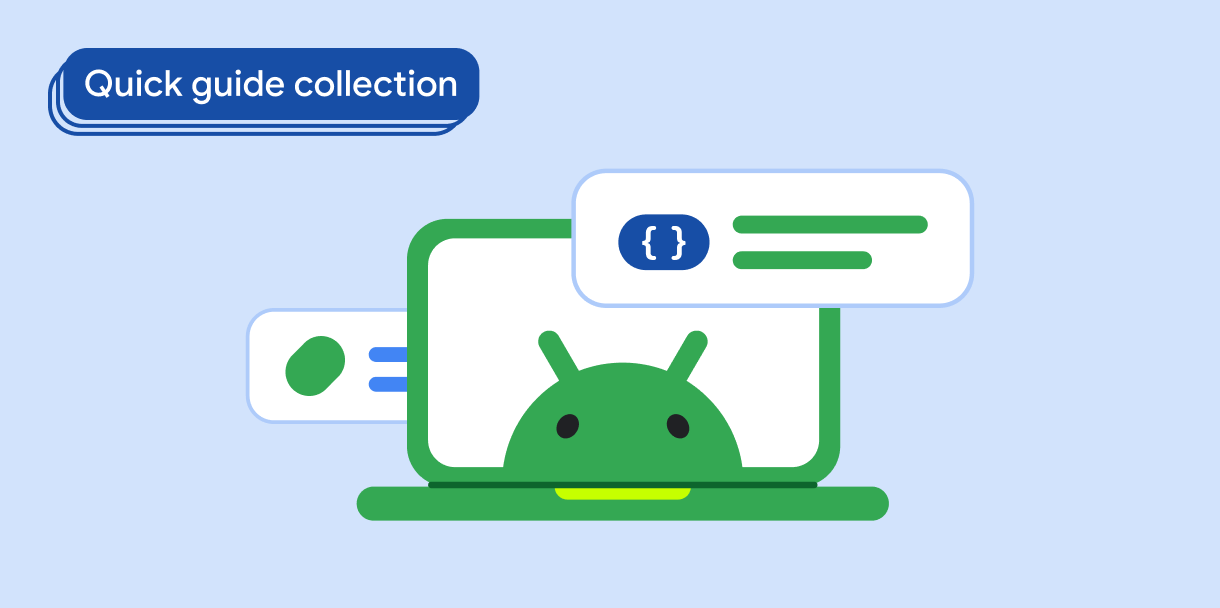
显示文本
文字对任何界面都属于核心内容。了解在应用中呈现文本的不同方式,以提供愉悦的用户体验。
有问题或反馈
请访问我们的常见问题解答页面,了解简短指南,或与我们联系,告诉我们您的想法。