為了讓首次使用者瞭解如何充分運用應用程式,請在應用程式啟動時提供新手上路資訊。以下是一些新手上路資訊的範例:
- 提供詳細資訊,說明使用者首次存取管道應用程式時可使用哪些管道。
- 吸引使用者註意應用程式中值得注意的功能。
- 說明使用者首次使用應用程式時必須採取的所有必要或建議步驟。
Leanback androidx 程式庫提供 OnboardingSupportFragment
類別,用於顯示初次使用者資訊。本指南說明如何使用 OnboardingSupportFragment
類別,提供應用程式首次啟動時所顯示的簡介資訊。
OnboardingSupportFragment
採用 TV UI 最佳做法,以與電視 UI 樣式相符的方式呈現資訊,且能在電視裝置上輕鬆瀏覽。
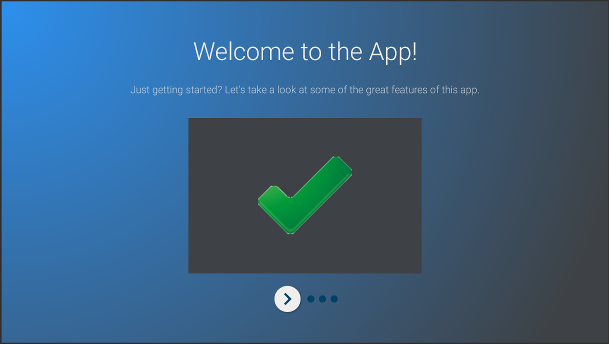
圖 1 OnboardingSupportFragment
範例。
OnboardingSupportFragment
不適合所有用途。當您需要加入需要使用者輸入的 UI 元素 (例如按鈕和欄位) 時,請勿使用 OnboardingSupportFragment
。此外,請勿將 OnboardingSupportFragment
用於使用者會定期執行的工作。最後,如果您需要顯示需要使用者輸入內容的多頁面 UI,請考慮使用 GuidedStepSupportFragment
。
新增 OnboardingSupportFragment
若要在應用程式中新增 OnboardingSupportFragment
,請實作擴充 OnboardingSupportFragment
類別的類別。使用活動的版面配置 XML,或以程式輔助方式,將這個片段新增至活動。請確認活動或片段使用從 Theme_Leanback_Onboarding
衍生的主題,如「自訂主題」一節所述。
在應用程式主要活動的 onCreate()
方法中,使用指向 OnboardingSupportFragment
父項活動的 Intent
呼叫 startActivity()
。這有助於確保 OnboardingSupportFragment
在應用程式啟動時立即顯示。
為確保 OnboardingSupportFragment
只會在使用者首次啟動應用程式時出現,請使用 SharedPreferences
物件追蹤使用者是否已查看 OnboardingSupportFragment
。定義當使用者完成查看 OnboardingSupportFragment
時,變更為 true 的布林值。在主要活動的 onCreate()
方法中檢查這個值,只有在值為 false 時,才啟動 OnboardingSupportFragment
父項活動。
以下範例顯示了 onCreate()
的覆寫值,用於檢查 SharedPreferences
值;如果沒有設為 true,則呼叫 startActivity()
以顯示 OnboardingSupportFragment
:
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) PreferenceManager.getDefaultSharedPreferences(this).apply { // Check if we need to display our OnboardingSupportFragment if (!getBoolean(MyOnboardingSupportFragment.COMPLETED_ONBOARDING_PREF_NAME, false)) { // The user hasn't seen the OnboardingSupportFragment yet, so show it startActivity(Intent(this@OnboardingActivity, OnboardingActivity::class.java)) } } }
Java
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); SharedPreferences sharedPreferences = PreferenceManager.getDefaultSharedPreferences(this); // Check if we need to display our OnboardingSupportFragment if (!sharedPreferences.getBoolean( MyOnboardingSupportFragment.COMPLETED_ONBOARDING_PREF_NAME, false)) { // The user hasn't seen the OnboardingSupportFragment yet, so show it startActivity(new Intent(this, OnboardingActivity.class)); } }
使用者查看 OnboardingSupportFragment
後,請使用 SharedPreferences
物件將其標示為已查看。方法是覆寫 OnboardingSupportFragment
中的 onFinishFragment()
,並將 SharedPreferences
值設為 true,如以下範例所示:
Kotlin
override fun onFinishFragment() { super.onFinishFragment() // User has seen OnboardingSupportFragment, so mark our SharedPreferences // flag as completed so that we don't show our OnboardingSupportFragment // the next time the user launches the app PreferenceManager.getDefaultSharedPreferences(context).edit().apply { putBoolean(COMPLETED_ONBOARDING_PREF_NAME, true) apply() } }
Java
@Override protected void onFinishFragment() { super.onFinishFragment(); // User has seen OnboardingSupportFragment, so mark our SharedPreferences // flag as completed so that we don't show our OnboardingSupportFragment // the next time the user launches the app SharedPreferences.Editor sharedPreferencesEditor = PreferenceManager.getDefaultSharedPreferences(getContext()).edit(); sharedPreferencesEditor.putBoolean( COMPLETED_ONBOARDING_PREF_NAME, true); sharedPreferencesEditor.apply(); }
新增 OnboardingSupportFragment 頁面
OnboardingSupportFragment
會以一系列已排序的頁面顯示內容。新增 OnboardingSupportFragment
後,您需要定義新手上路頁面。每個頁面都可以提供標題、說明,以及數個可包含圖片或動畫的子檢視畫面。
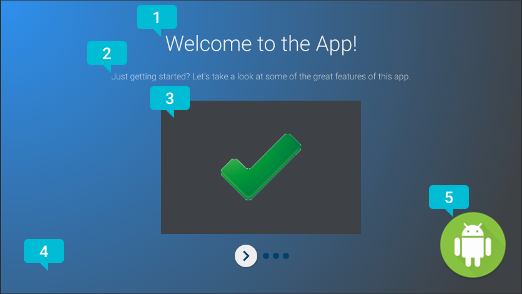
圖 2. OnboardingSupportFragment
網頁元素。
圖 2 顯示的是摘要頁面,其中摘要標示了 OnboardingSupportFragment
可提供的自訂網頁元素。頁面元素如下:
- 網頁標題。
- 頁面說明。
- 網頁內容檢視 (在本例中為灰色方塊),有一個簡單的綠色勾號。 這是選用檢視畫面,這個檢視畫面會顯示網頁的詳細資料。例如,您可以提供螢幕截圖,凸顯頁面說明的應用程式功能。
- 頁面背景檢視畫面,本例中有一個簡單的藍色漸層。這個檢視畫面一律會在頁面上其他檢視畫面背後轉譯。這是選用檢視畫面,
- 頁面前景檢視畫面 (本例中為標誌)。這個檢視畫面一律會顯示在頁面上所有其他檢視畫面的前方。這是選用檢視畫面,
初次建立 OnboardingSupportFragment
或附加至父項活動時,初始化頁面資訊,因為系統會在建立片段檢視畫面時要求頁面資訊。您可以在類別建構函式中初始化網頁資訊,或在 onAttach()
覆寫值中初始化。
覆寫下列每個將頁面資訊提供給系統的方法:
getPageCount()
會傳回OnboardingSupportFragment
中的頁數。getPageTitle()
會傳回要求的頁碼標題。getPageDescription()
會傳回要求的頁面編號說明。
覆寫以下每個方法,提供選用子檢視畫面來顯示圖片或動畫:
onCreateBackgroundView()
會傳回您建立的View
做為背景檢視畫面;如果不需要背景檢視畫面,則會傳回空值。onCreateContentView()
會傳回您建立的View
做為內容檢視畫面;如果不需要內容檢視畫面,則會傳回空值。onCreateForegroundView()
會傳回您建立的View
做為前景檢視畫面,如果不需要前景檢視畫面,則會傳回空值。
系統會將您建立的 View
新增至網頁版面配置。以下範例會覆寫 onCreateContentView()
並傳回 ImageView
:
Kotlin
private lateinit var contentView: ImageView ... override fun onCreateContentView(inflater: LayoutInflater?, container: ViewGroup?): View? { return ImageView(context).apply { scaleType = ImageView.ScaleType.CENTER_INSIDE setImageResource(R.drawable.onboarding_content_view) setPadding(0, 32, 0, 32) contentView = this } }
Java
private ImageView contentView; ... @Override protected View onCreateContentView(LayoutInflater inflater, ViewGroup container) { contentView = new ImageView(getContext()); contentView.setScaleType(ImageView.ScaleType.CENTER_INSIDE); contentView.setImageResource(R.drawable.onboarding_content_view); contentView.setPadding(0, 32, 0, 32); return contentView; }
新增初始標誌畫面
您的 OnboardingSupportFragment
可以從選用標誌畫面開始,開始顯示應用程式說明。如要將 Drawable
做為標誌畫面顯示,請在 OnboardingSupportFragment
的 onCreate()
方法中,使用 Drawable
的 ID 呼叫 setLogoResourceId()
。系統會淡入並短暫顯示 Drawable
,然後在顯示 OnboardingSupportFragment
的第一頁之前淡出 Drawable
。
如果想為標誌畫面提供自訂動畫,而非呼叫 setLogoResourceId()
,請覆寫 onCreateLogoAnimation()
並傳回可轉譯自訂動畫的 Animator
物件,如以下範例所示:
Kotlin
public override fun onCreateLogoAnimation(): Animator = AnimatorInflater.loadAnimator(context, R.animator.onboarding_logo_screen_animation)
Java
@Override public Animator onCreateLogoAnimation() { return AnimatorInflater.loadAnimator(getContext(), R.animator.onboarding_logo_screen_animation); }
自訂頁面動畫
系統會在顯示 OnboardingSupportFragment
的第一頁,以及使用者前往其他頁面時,使用預設動畫。您可以覆寫 OnboardingSupportFragment
中的方法,自訂這些動畫。
如要自訂顯示在第一頁的動畫,請覆寫 onCreateEnterAnimation()
並傳回 Animator
。以下範例會建立 Animator
,可水平縮放內容檢視區塊:
Kotlin
override fun onCreateEnterAnimation(): Animator = ObjectAnimator.ofFloat(contentView, View.SCALE_X, 0.2f, 1.0f) .setDuration(ANIMATION_DURATION)
Java
@Override protected Animator onCreateEnterAnimation() { Animator startAnimator = ObjectAnimator.ofFloat(contentView, View.SCALE_X, 0.2f, 1.0f).setDuration(ANIMATION_DURATION); return startAnimator; }
如要自訂使用者前往不同頁面時顯示的動畫,請覆寫 onPageChanged()
。在 onPageChanged()
方法中,建立 Animator
物件來移除上一頁並顯示下一頁,並將這些物件新增至 AnimatorSet
,然後播放這組物件。下例使用淡出動畫來移除上一頁、更新內容檢視圖片,並使用淡入效果動畫顯示下一頁:
Kotlin
override fun onPageChanged(newPage: Int, previousPage: Int) { // Create a fade-out animation for previousPage and, once // done, swap the contentView image with the next page's image val fadeOut = ObjectAnimator.ofFloat(mContentView, View.ALPHA, 1.0f, 0.0f) .setDuration(ANIMATION_DURATION) .apply { addListener(object : AnimatorListenerAdapter() { override fun onAnimationEnd(animation: Animator) { mContentView.setImageResource(pageImages[newPage]) } }) } // Create a fade-in animation for nextPage val fadeIn = ObjectAnimator.ofFloat(mContentView, View.ALPHA, 0.0f, 1.0f) .setDuration(ANIMATION_DURATION) // Create AnimatorSet with fade-out and fade-in animators and start it AnimatorSet().apply { playSequentially(fadeOut, fadeIn) start() } }
Java
@Override protected void onPageChanged(final int newPage, int previousPage) { // Create a fade-out animation for previousPage and, once // done, swap the contentView image with the next page's image Animator fadeOut = ObjectAnimator.ofFloat(mContentView, View.ALPHA, 1.0f, 0.0f).setDuration(ANIMATION_DURATION); fadeOut.addListener(new AnimatorListenerAdapter() { @Override public void onAnimationEnd(Animator animation) { mContentView.setImageResource(pageImages[newPage]); } }); // Create a fade-in animation for nextPage Animator fadeIn = ObjectAnimator.ofFloat(mContentView, View.ALPHA, 0.0f, 1.0f).setDuration(ANIMATION_DURATION); // Create AnimatorSet with fade-out and fade-in animators and start it AnimatorSet set = new AnimatorSet(); set.playSequentially(fadeOut, fadeIn); set.start(); }
如要進一步瞭解如何建立 Animator
物件和 AnimatorSet
物件,請參閱
屬性動畫總覽。
自訂主題
所有 OnboardingSupportFragment
實作都必須使用 Theme_Leanback_Onboarding
主題,或沿用自 Theme_Leanback_Onboarding
的主題。透過下列任一做法設定 OnboardingSupportFragment
的主題:
- 設定
OnboardingSupportFragment
的父項活動,以使用所需主題。以下範例說明如何在應用程式資訊清單中設定活動以使用Theme_Leanback_Onboarding
:<activity android:name=".OnboardingActivity" android:enabled="true" android:exported="true" android:theme="@style/Theme.Leanback.Onboarding"> </activity>
-
在自訂活動主題中使用
LeanbackOnboardingTheme_onboardingTheme
屬性,在父項活動中設定主題。請將這個屬性指向另一個只有活動中使用OnboardingSupportFragment
物件的自訂主題。如果您的活動已使用自訂主題,且您不想將OnboardingSupportFragment
樣式套用至活動中的其他檢視畫面,請使用這個方法。 - 覆寫
onProvideTheme()
並傳回所需主題。如果多項活動使用OnboardingSupportFragment
,或是父項活動無法使用所需主題,請採用這種做法。以下範例會覆寫onProvideTheme()
並傳回Theme_Leanback_Onboarding
:Kotlin
override fun onProvideTheme(): Int = R.style.Theme_Leanback_Onboarding
Java
@Override public int onProvideTheme() { return R.style.Theme_Leanback_Onboarding; }