收看電視直播時,使用者會更換頻道,並在畫面上顯示 頻道和節目資訊。其他類型的資訊 例如訊息 (「請勿在家中離開」)、字幕或廣告需要保留。與任何電視一樣 應用程式,這類資訊不應幹擾畫面上播放的節目內容。
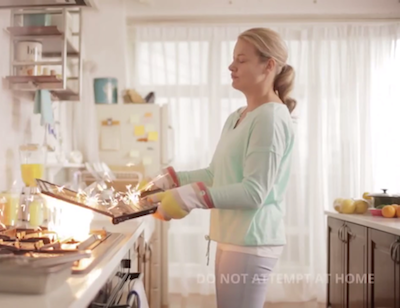
圖 1. 直播電視應用程式中的重疊訊息。
同時,考量到 YouTube 是否應顯示特定節目內容, 應用程式的內容、行為和通知使用者 內容遭到封鎖或無法播放。本課程將說明如何開發電視輸入裝置的使用者 使用者體驗
請嘗試 電視輸入服務範例應用程式。
將玩家與表面整合
您的電視輸入裝置必須將影片算繪到 Surface
物件,並由該物件傳遞
TvInputService.Session.onSetSurface()
方法。以下範例說明如何使用 MediaPlayer
例項玩遊戲
Surface
物件中的內容:
Kotlin
override fun onSetSurface(surface: Surface?): Boolean { player?.setSurface(surface) mSurface = surface return true } override fun onSetStreamVolume(volume: Float) { player?.setVolume(volume, volume) mVolume = volume }
Java
@Override public boolean onSetSurface(Surface surface) { if (player != null) { player.setSurface(surface); } mSurface = surface; return true; } @Override public void onSetStreamVolume(float volume) { if (player != null) { player.setVolume(volume, volume); } mVolume = volume; }
同樣地,下列步驟說明如何使用 ExoPlayer:
Kotlin
override fun onSetSurface(surface: Surface?): Boolean { player?.createMessage(videoRenderer)?.apply { type = MSG_SET_SURFACE payload = surface send() } mSurface = surface return true } override fun onSetStreamVolume(volume: Float) { player?.createMessage(audioRenderer)?.apply { type = MSG_SET_VOLUME payload = volume send() } mVolume = volume }
Java
@Override public boolean onSetSurface(@Nullable Surface surface) { if (player != null) { player.createMessage(videoRenderer) .setType(MSG_SET_SURFACE) .setPayload(surface) .send(); } mSurface = surface; return true; } @Override public void onSetStreamVolume(float volume) { if (player != null) { player.createMessage(videoRenderer) .setType(MSG_SET_VOLUME) .setPayload(volume) .send(); } mVolume = volume; }
使用疊加層
使用重疊元素顯示字幕、訊息、廣告或 MHEG-5 資料廣播。根據預設,
已停用重疊。您可以在建立工作階段時,呼叫
TvInputService.Session.setOverlayViewEnabled(true)
,
如以下範例所示:
Kotlin
override fun onCreateSession(inputId: String): Session = onCreateSessionInternal(inputId).apply { setOverlayViewEnabled(true) sessions.add(this) }
Java
@Override public final Session onCreateSession(String inputId) { BaseTvInputSessionImpl session = onCreateSessionInternal(inputId); session.setOverlayViewEnabled(true); sessions.add(session); return session; }
將 View
物件用於疊加層 (從 TvInputService.Session.onCreateOverlayView()
傳回),如下所示:
Kotlin
override fun onCreateOverlayView(): View = (context.getSystemService(LAYOUT_INFLATER_SERVICE) as LayoutInflater).run { inflate(R.layout.overlayview, null).apply { subtitleView = findViewById<SubtitleView>(R.id.subtitles).apply { // Configure the subtitle view. val captionStyle: CaptionStyleCompat = CaptionStyleCompat.createFromCaptionStyle(captioningManager.userStyle) setStyle(captionStyle) setFractionalTextSize(captioningManager.fontScale) } } }
Java
@Override public View onCreateOverlayView() { LayoutInflater inflater = (LayoutInflater) getSystemService(LAYOUT_INFLATER_SERVICE); View view = inflater.inflate(R.layout.overlayview, null); subtitleView = (SubtitleView) view.findViewById(R.id.subtitles); // Configure the subtitle view. CaptionStyleCompat captionStyle; captionStyle = CaptionStyleCompat.createFromCaptionStyle( captioningManager.getUserStyle()); subtitleView.setStyle(captionStyle); subtitleView.setFractionalTextSize(captioningManager.fontScale); return view; }
疊加層的版面配置定義可能如下所示:
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent"> <com.google.android.exoplayer.text.SubtitleView android:id="@+id/subtitles" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="bottom|center_horizontal" android:layout_marginLeft="16dp" android:layout_marginRight="16dp" android:layout_marginBottom="32dp" android:visibility="invisible"/> </FrameLayout>
控管內容
使用者選取頻道時,電視輸入裝置會處理 TvInputService.Session
物件中的 onTune()
回呼。系統電視
應用程式的家長監護功能會根據內容分級,決定要顯示哪些內容。
以下各節將說明如何使用
TvInputService.Session
notify
種方法,
以便與系統的 TV 應用程式通訊
設為無法觀看影片
使用者變更頻道時,請確保螢幕並未顯示任何灰色
影片假影。呼叫 TvInputService.Session.onTune()
時,
呼叫 TvInputService.Session.notifyVideoUnavailable()
即可避免系統顯示該部影片
並傳遞 VIDEO_UNAVAILABLE_REASON_TUNING
常數,如
如以下範例所示
Kotlin
override fun onTune(channelUri: Uri): Boolean { subtitleView?.visibility = View.INVISIBLE notifyVideoUnavailable(TvInputManager.VIDEO_UNAVAILABLE_REASON_TUNING) unblockedRatingSet.clear() dbHandler.apply { removeCallbacks(playCurrentProgramRunnable) playCurrentProgramRunnable = PlayCurrentProgramRunnable(channelUri) post(playCurrentProgramRunnable) } return true }
Java
@Override public boolean onTune(Uri channelUri) { if (subtitleView != null) { subtitleView.setVisibility(View.INVISIBLE); } notifyVideoUnavailable(TvInputManager.VIDEO_UNAVAILABLE_REASON_TUNING); unblockedRatingSet.clear(); dbHandler.removeCallbacks(playCurrentProgramRunnable); playCurrentProgramRunnable = new PlayCurrentProgramRunnable(channelUri); dbHandler.post(playCurrentProgramRunnable); return true; }
接著,當內容算繪到 Surface
時,您可以呼叫
TvInputService.Session.notifyVideoAvailable()
允許影片顯示,如下所示:
Kotlin
fun onRenderedFirstFrame(surface:Surface) { firstFrameDrawn = true notifyVideoAvailable() }
Java
@Override public void onRenderedFirstFrame(Surface surface) { firstFrameDrawn = true; notifyVideoAvailable(); }
這個轉場效果只會持續到一秒,但呈現空白畫面 比起允許圖片閃爍顯示奇怪和晃動畫面的效果更好。
另請參閱「將玩家與途徑整合」,進一步瞭解工作內容
搭配 Surface
來轉譯影片
提供家長監護功能
如要判斷特定內容是否遭到家長監護功能和內容分級封鎖,請查看
TvInputManager
類別方法、isParentalControlsEnabled()
和 isRatingBlocked(android.media.tv.TvContentRating)
。個人中心
請確認您的內容 TvContentRating
並設定目前允許的內容分級。以下範例說明這些考量因素。
Kotlin
private fun checkContentBlockNeeded() { currentContentRating?.also { rating -> if (!tvInputManager.isParentalControlsEnabled || !tvInputManager.isRatingBlocked(rating) || unblockedRatingSet.contains(rating)) { // Content rating is changed so we don't need to block anymore. // Unblock content here explicitly to resume playback. unblockContent(null) return } } lastBlockedRating = currentContentRating player?.run { // Children restricted content might be blocked by TV app as well, // but TIF should do its best not to show any single frame of blocked content. releasePlayer() } notifyContentBlocked(currentContentRating) }
Java
private void checkContentBlockNeeded() { if (currentContentRating == null || !tvInputManager.isParentalControlsEnabled() || !tvInputManager.isRatingBlocked(currentContentRating) || unblockedRatingSet.contains(currentContentRating)) { // Content rating is changed so we don't need to block anymore. // Unblock content here explicitly to resume playback. unblockContent(null); return; } lastBlockedRating = currentContentRating; if (player != null) { // Children restricted content might be blocked by TV app as well, // but TIF should do its best not to show any single frame of blocked content. releasePlayer(); } notifyContentBlocked(currentContentRating); }
確定內容是否應遭到封鎖後,請通知系統電視
方法是呼叫
TvInputService.Session
方法 notifyContentAllowed()
或
notifyContentBlocked()
,如上一個範例所示。
使用 TvContentRating
類別產生系統定義的字串,
COLUMN_CONTENT_RATING
和
TvContentRating.createRating()
方法,如下所示:
Kotlin
val rating = TvContentRating.createRating( "com.android.tv", "US_TV", "US_TV_PG", "US_TV_D", "US_TV_L" )
Java
TvContentRating rating = TvContentRating.createRating( "com.android.tv", "US_TV", "US_TV_PG", "US_TV_D", "US_TV_L");
處理音軌選取作業
TvTrackInfo
類別包含媒體軌相關資訊,例如
發行類型 (影片、音訊或字幕) 等。
如果電視輸入工作階段首次能夠取得追蹤資訊,應呼叫
TvInputService.Session.notifyTracksChanged()
,其中包含要更新系統 TV 應用程式的所有測試群組清單。有地點時
是異動追蹤資訊,呼叫
notifyTracksChanged()
來更新系統。
系統 TV 應用程式提供介面,可讓使用者選取特定音軌 (如有多個音軌)
曲目適用於指定的音軌類型;例如不同語言的字幕你的電視
輸入內容會回應
onSelectTrack()
從系統 TV 應用程式撥號至
notifyTrackSelected()
,如以下範例所示。請注意,如果 null
傳遞做為音軌 ID,這會取消選取音軌。
Kotlin
override fun onSelectTrack(type: Int, trackId: String?): Boolean = mPlayer?.let { player -> if (type == TvTrackInfo.TYPE_SUBTITLE) { if (!captionEnabled && trackId != null) return false selectedSubtitleTrackId = trackId subtitleView.visibility = if (trackId == null) View.INVISIBLE else View.VISIBLE } player.trackInfo.indexOfFirst { it.trackType == type }.let { trackIndex -> if( trackIndex >= 0) { player.selectTrack(trackIndex) notifyTrackSelected(type, trackId) true } else false } } ?: false
Java
@Override public boolean onSelectTrack(int type, String trackId) { if (player != null) { if (type == TvTrackInfo.TYPE_SUBTITLE) { if (!captionEnabled && trackId != null) { return false; } selectedSubtitleTrackId = trackId; if (trackId == null) { subtitleView.setVisibility(View.INVISIBLE); } } int trackIndex = -1; MediaPlayer.TrackInfo[] trackInfos = player.getTrackInfo(); for (int index = 0; index < trackInfos.length; index++) { MediaPlayer.TrackInfo trackInfo = trackInfos[index]; if (trackInfo.getTrackType() == type) { trackIndex = index; break; } } if (trackIndex >= 0) { player.selectTrack(trackIndex); notifyTrackSelected(type, trackId); return true; } } return false; }