テキストの表示を文字単位でアニメーション化できるため、タイプライターのように入力が流れるように見える効果を実現できます。
バージョンの互換性
この実装では、プロジェクトの minSDK を API レベル 21 以上に設定する必要があります。
依存関係
テキストを文字ごとにアニメーション化する
このコードは、テキストを文字ごとにアニメーション化します。インデックスを追跡して、表示されるテキストの量を制御します。表示されるテキストは動的に更新され、現在のインデックスまでの文字のみが表示されます。最後に、変数が変更されるとアニメーションが実行されます。
@Composable private fun AnimatedText() { val text = "This text animates as though it is being typed \uD83E\uDDDE\u200D♀\uFE0F \uD83D\uDD10 \uD83D\uDC69\u200D❤\uFE0F\u200D\uD83D\uDC68 \uD83D\uDC74\uD83C\uDFFD" // Use BreakIterator as it correctly iterates over characters regardless of how they are // stored, for example, some emojis are made up of multiple characters. // You don't want to break up an emoji as it animates, so using BreakIterator will ensure // this is correctly handled! val breakIterator = remember(text) { BreakIterator.getCharacterInstance() } // Define how many milliseconds between each character should pause for. This will create the // illusion of an animation, as we delay the job after each character is iterated on. val typingDelayInMs = 50L var substringText by remember { mutableStateOf("") } LaunchedEffect(text) { // Initial start delay of the typing animation delay(1000) breakIterator.text = StringCharacterIterator(text) var nextIndex = breakIterator.next() // Iterate over the string, by index boundary while (nextIndex != BreakIterator.DONE) { substringText = text.subSequence(0, nextIndex).toString() // Go to the next logical character boundary nextIndex = breakIterator.next() delay(typingDelayInMs) } } Text(substringText)
コードに関する主なポイント
BreakIterator
は、文字の保存方法に関係なく、文字を正しく反復処理します。たとえば、アニメーション 絵文字は複数の文字で構成されています。BreakIterator
を使用すると、アニメーションが中断されないように、アニメーション 絵文字が 1 つの文字として処理されます。LaunchedEffect
はコルーチンを開始して、文字間に遅延を導入します。コードブロックをクリック リスナー(または他の任意のイベント)に置き換えて、アニメーションをトリガーできます。Text
コンポーザブルは、substringText
の値が更新されるたびに再レンダリングされます。
結果
このガイドを含むコレクション
このガイドは、Android 開発の幅広い目標を網羅する、厳選されたクイックガイド コレクションの一部です。
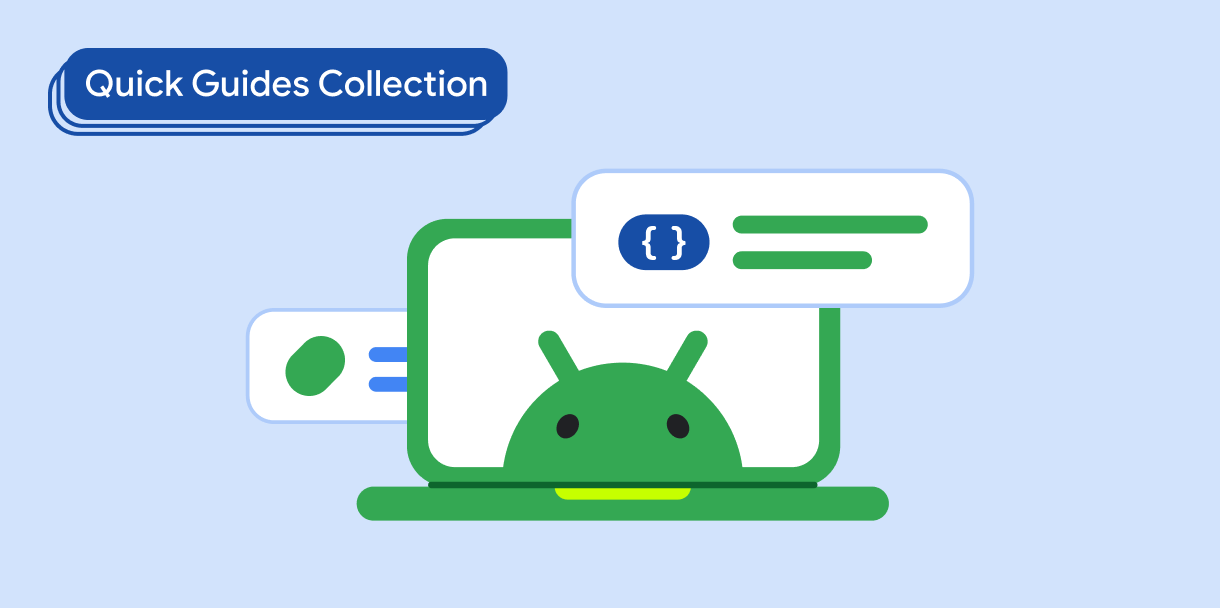
表示テキスト
テキストは UI の主要な構成要素です。アプリでテキストを表示して優れたユーザー エクスペリエンスを提供するさまざまな方法を学びます。
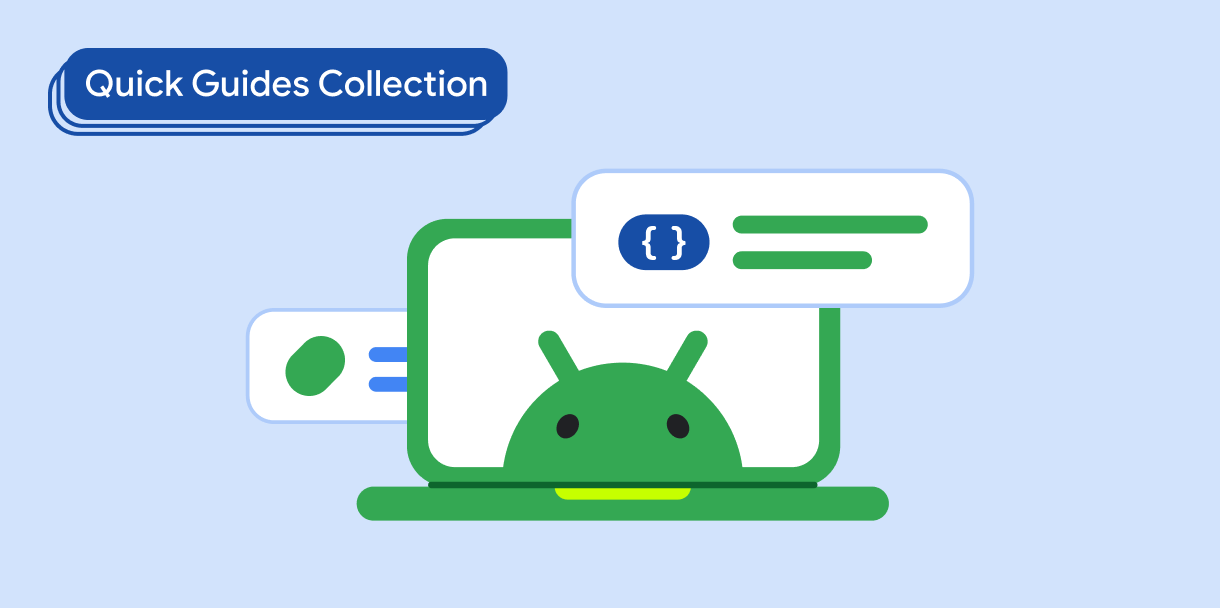
Compose の基本(動画コレクション)
この動画シリーズでは、さまざまな Compose API を紹介し、利用可能な API とその使用方法を簡単に説明します。
ご質問やフィードバックがある場合
よくある質問のページでクイックガイドをご覧になるか、お問い合わせフォームからご意見をお寄せください。