Create a home screen scaffold Collection
Conveniently add standard UI elements and follow Material Design guidelines.
@Composable fun BottomAppBarExample() { Scaffold( bottomBar = { BottomAppBar( actions = { IconButton(onClick = { /* do something */ }) { Icon(Icons.Filled.Check, contentDescription = "Localized description") } IconButton(onClick = { /* do something */ }) { Icon( Icons.Filled.Edit, contentDescription = "Localized description", ) } IconButton(onClick = { /* do something */ }) { Icon( Icons.Filled.Mic, contentDescription = "Localized description", ) } IconButton(onClick = { /* do something */ }) { Icon( Icons.Filled.Image, contentDescription = "Localized description", ) } }, floatingActionButton = { FloatingActionButton( onClick = { /* do something */ }, containerColor = BottomAppBarDefaults.bottomAppBarFabColor, elevation = FloatingActionButtonDefaults.bottomAppBarFabElevation() ) { Icon(Icons.Filled.Add, "Localized description") } } ) }, ) { innerPadding -> Text( modifier = Modifier.padding(innerPadding), text = "Example of a scaffold with a bottom app bar." ) } }
Display a bottom app bar
Create a bottom app bar to help users navigate and access functions in your app.
@Composable fun MediumTopAppBarExample() { val scrollBehavior = TopAppBarDefaults.enterAlwaysScrollBehavior(rememberTopAppBarState()) Scaffold( modifier = Modifier.nestedScroll(scrollBehavior.nestedScrollConnection), topBar = { MediumTopAppBar( colors = TopAppBarDefaults.topAppBarColors( containerColor = MaterialTheme.colorScheme.primaryContainer, titleContentColor = MaterialTheme.colorScheme.primary, ), title = { Text( "Medium Top App Bar", maxLines = 1, overflow = TextOverflow.Ellipsis ) }, navigationIcon = { IconButton(onClick = { /* do something */ }) { Icon( imageVector = Icons.Filled.ArrowBack, contentDescription = "Localized description" ) } }, actions = { IconButton(onClick = { /* do something */ }) { Icon( imageVector = Icons.Filled.Menu, contentDescription = "Localized description" ) } }, scrollBehavior = scrollBehavior ) }, ) { innerPadding -> ScrollContent(innerPadding) } }
Display a top app bar
Create a top app bar to help users navigate and access functions in your app.
@Composable fun SmallTopAppBarExample() { Scaffold( topBar = { TopAppBar( colors = TopAppBarDefaults.topAppBarColors( containerColor = MaterialTheme.colorScheme.primaryContainer, titleContentColor = MaterialTheme.colorScheme.primary, ), title = { Text("Small Top App Bar") } } ) }
Create a scaffold component to hold the UI together
Containers provide the user access to key features and navigation items. There are two types of app bars, top app bars and bottom app bars.
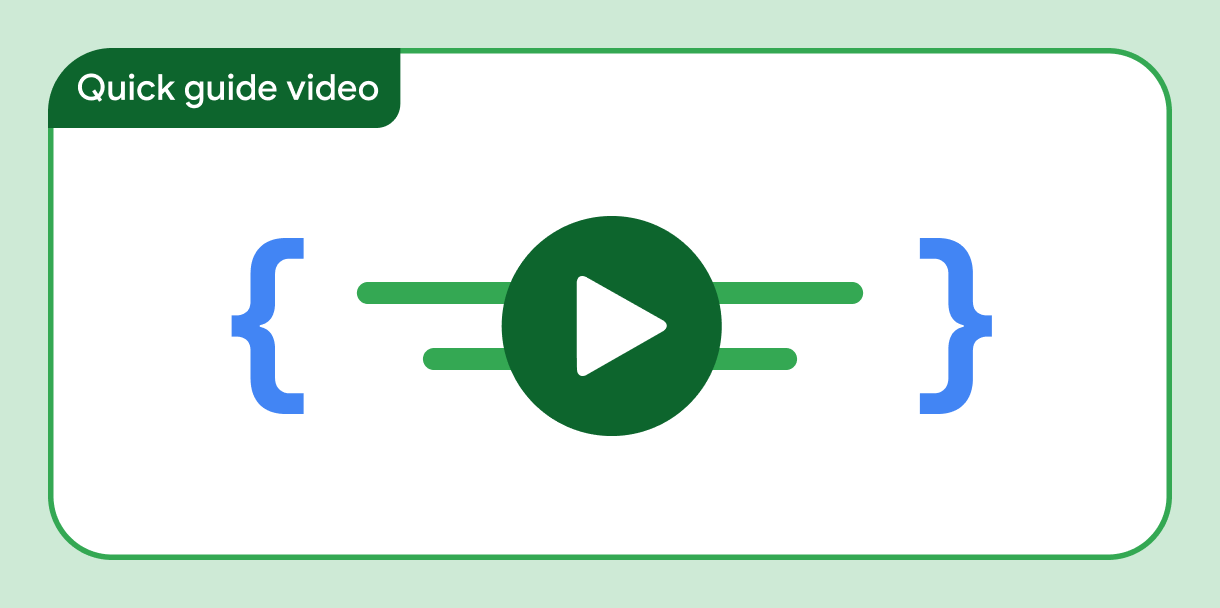
Insets in Compose
6 minutes
Learn how insets communicate to your app where system decorations are placed, and how Compose APIs help your content automatically move with the system bars, software keyboard, and the taskbar. Don't be afraid to go edge-to-edge!
Have questions or feedback
Go to our frequently asked questions page and learn about quick guides or reach out and let us know your thoughts.