Google AI 클라이언트 SDK를 사용하면 Android 앱에서 직접 Gemini API를 호출하고 Gemini 모델 제품군을 사용할 수 있습니다.
무료 등급을 사용하면 무료로 실험할 수 있습니다. 다른 가격 책정 세부정보는 가격 책정 가이드를 참고하세요.
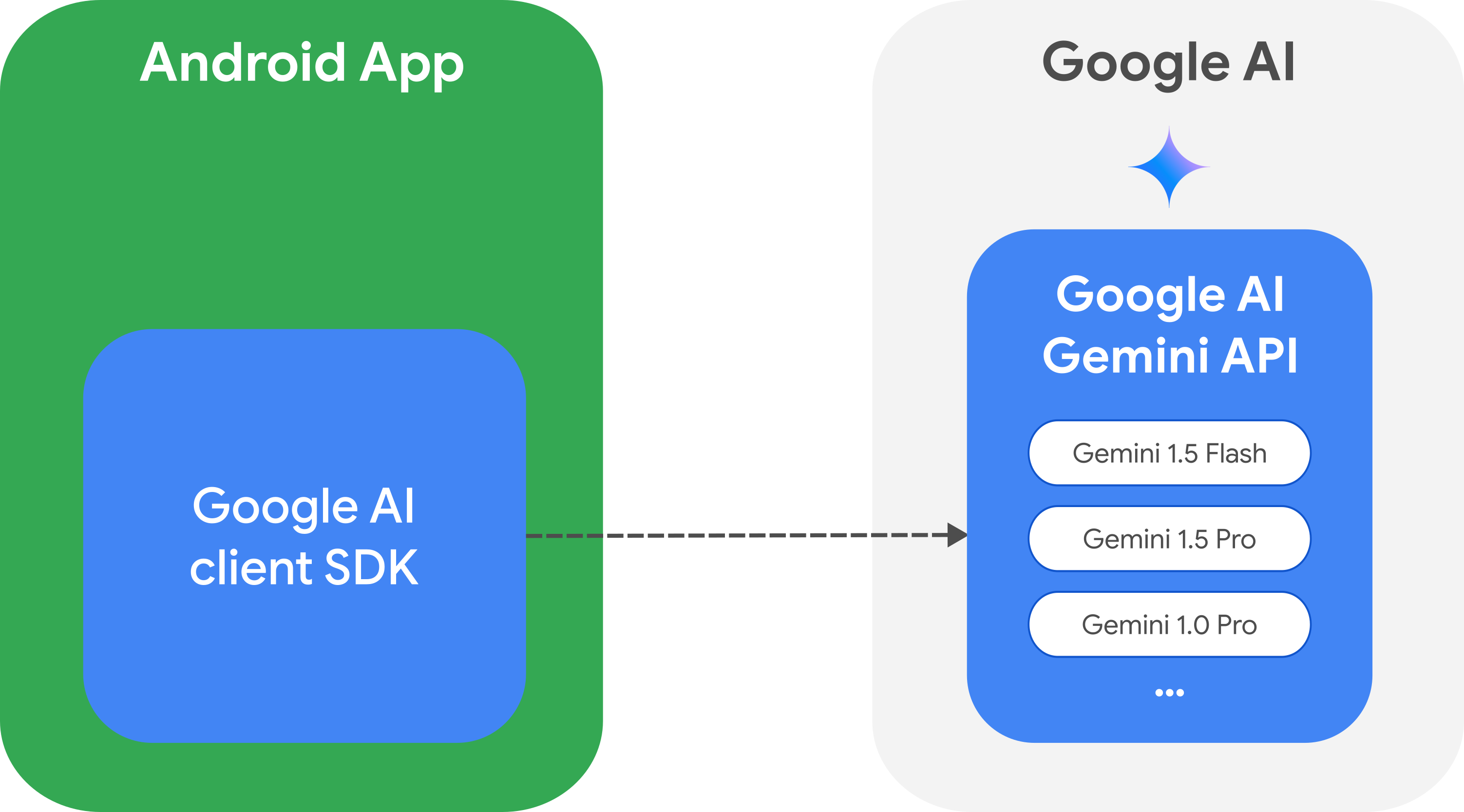
시작하기
앱에서 Gemini API와 직접 상호작용하기 전에 메시지 표시를 숙지하고 API 키를 생성하고 SDK를 사용하도록 앱을 설정하는 등 몇 가지 작업을 먼저 해야 합니다.
프롬프트 실험
먼저 Google AI Studio에서 프롬프트의 프로토타입을 만듭니다.
Google AI 스튜디오는 프롬프트 설계 및 프로토타입 제작을 위한 IDE입니다. 파일을 업로드하여 텍스트와 이미지로 프롬프트를 테스트하고 나중에 다시 방문할 프롬프트를 저장할 수 있습니다.
사용 사례에 적합한 프롬프트를 만드는 것은 과학보다는 예술에 가깝기 때문에 실험이 중요합니다. 프롬프트에 관한 자세한 내용은 공식 Google AI 문서를 참고하세요.
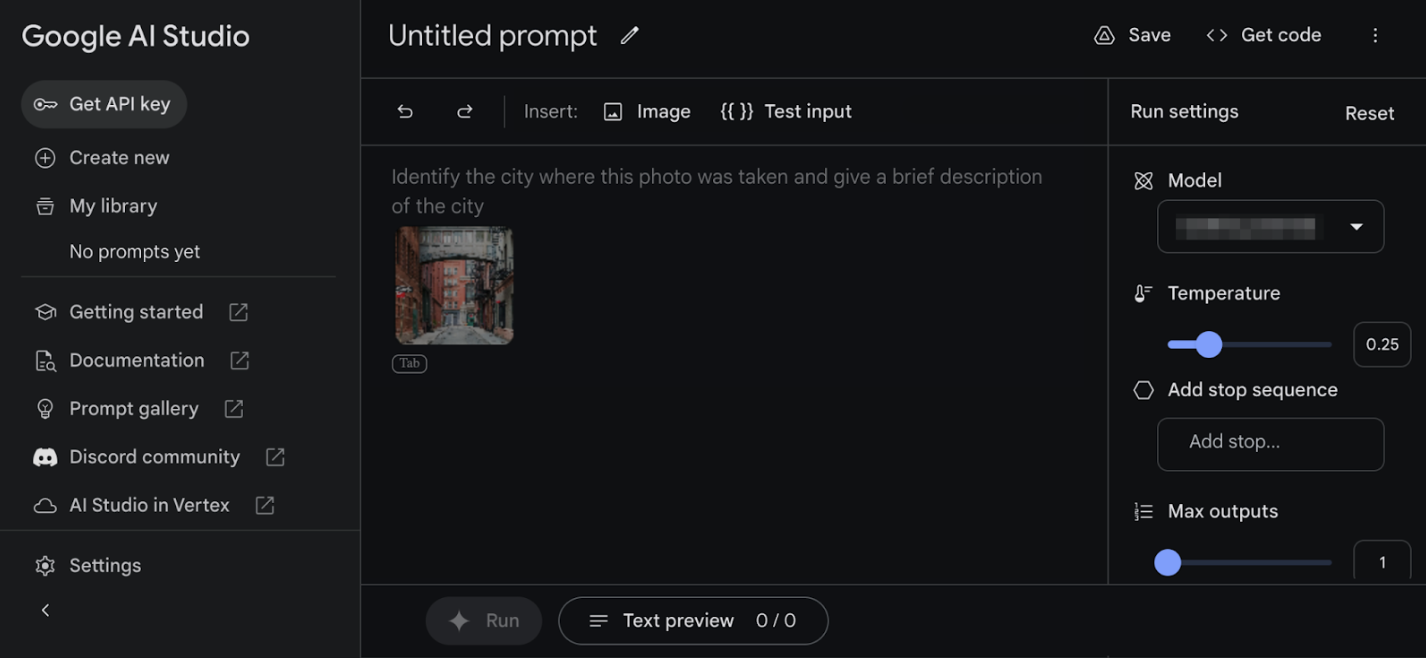
Google AI 스튜디오의 고급 기능에 관한 자세한 내용은 Google AI 스튜디오 빠른 시작을 참고하세요.
API 키 생성하기
메시지가 마음에 들면 API 키 가져오기를 클릭하여 Gemini API 키를 생성합니다. 키는 애플리케이션과 번들로 제공되며 이는 실험 및 프로토타입 제작에는 적합하지만 프로덕션 사용 사례에는 권장되지 않습니다.
또한 API 키가 소스 코드 저장소에 커밋되지 않도록 하려면 Secrets Gradle 플러그인을 사용하세요.
Gradle 종속 항목 추가
앱에 Google AI 클라이언트 SDK의 종속 항목을 추가합니다.
Kotlin
dependencies { [...] implementation("com.google.ai.client.generativeai:generativeai:0.7.0") }
자바
dependencies { [...] implementation("com.google.ai.client.generativeai:generativeai:0.7.0") // Required to use `ListenableFuture` from Guava Android for one-shot generation implementation("com.google.guava:guava:31.0.1-android") // Required to use `Publisher` from Reactive Streams for streaming operations implementation("org.reactivestreams:reactive-streams:1.0.4") }
GenerativeModel
를 만드는 방법
먼저 다음을 제공하여 GenerativeModel
를 인스턴스화합니다.
- 모델 이름:
gemini-1.5-flash
,gemini-1.5-pro
또는gemini-1.0-pro
- Google AI Studio로 생성된 API 키
원하는 경우 모델 매개변수를 정의하고 온도, topK, topP, 최대 출력 토큰 값을 제공할 수 있습니다.
다음 주제에 대한 안전 기능을 정의할 수도 있습니다.
HARASSMENT
HATE_SPEECH
SEXUALLY_EXPLICIT
DANGEROUS_CONTENT
Kotlin
val model = GenerativeModel( model = "gemini-1.5-flash-001", apiKey = BuildConfig.apikey, generationConfig = generationConfig { temperature = 0.15f topK = 32 topP = 1f maxOutputTokens = 4096 }, safetySettings = listOf( SafetySetting(HarmCategory.HARASSMENT, BlockThreshold.MEDIUM_AND_ABOVE), SafetySetting(HarmCategory.HATE_SPEECH, BlockThreshold.MEDIUM_AND_ABOVE), SafetySetting(HarmCategory.SEXUALLY_EXPLICIT, BlockThreshold.MEDIUM_AND_ABOVE), SafetySetting(HarmCategory.DANGEROUS_CONTENT, BlockThreshold.MEDIUM_AND_ABOVE), ) )
자바
GenerationConfig.Builder configBuilder = new GenerationConfig.Builder(); configBuilder.temperature = 0.15f; configBuilder.topK = 32; configBuilder.topP = 1f; configBuilder.maxOutputTokens = 4096; ArrayList<SafetySetting> safetySettings = new ArrayList(); safetySettings.add(new SafetySetting(HarmCategory.HARASSMENT, BlockThreshold.MEDIUM_AND_ABOVE)); safetySettings.add(new SafetySetting(HarmCategory.HATE_SPEECH, BlockThreshold.MEDIUM_AND_ABOVE)); safetySettings.add(new SafetySetting(HarmCategory.SEXUALLY_EXPLICIT, BlockThreshold.MEDIUM_AND_ABOVE)); safetySettings.add(new SafetySetting(HarmCategory.DANGEROUS_CONTENT, BlockThreshold.MEDIUM_AND_ABOVE)); GenerativeModel gm = new GenerativeModel( "gemini-1.5-flash-001", BuildConfig.apiKey, configBuilder.build(), safetySettings );
앱에서 Google AI 클라이언트 SDK 사용
이제 API 키를 가져오고 SDK를 사용하도록 앱을 설정했으므로 Gemini API와 상호작용할 수 있습니다.
텍스트 생성
텍스트 응답을 생성하려면 프롬프트와 함께 generateContent()
를 호출합니다.
Kotlin
scope.launch { val response = model.generateContent("Write a story about a green robot.") }
자바
// In Java, create a GenerativeModelFutures from the GenerativeModel. // generateContent() returns a ListenableFuture. // Learn more: // https://developer.android.com/develop/background-work/background-tasks/asynchronous/listenablefuture GenerativeModelFutures model = GenerativeModelFutures.from(gm); Content content = new Content.Builder() .addText("Write a story about a green robot.") .build(); Executor executor = // ... ListenableFuture<GenerateContentResponse> response = model.generateContent(content); Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() { @Override public void onSuccess(GenerateContentResponse result) { String resultText = result.getText(); } @Override public void onFailure(Throwable t) { t.printStackTrace(); } }, executor);
generateContent()
는 기존 Kotlin 코드와 잘 통합되는 suspend
함수입니다.
이미지 및 기타 미디어에서 텍스트 생성
텍스트와 이미지 또는 기타 미디어가 포함된 프롬프트에서 텍스트를 생성할 수도 있습니다. generateContent()
를 호출할 때 미디어를 인라인 데이터로 전달할 수 있습니다(아래 예 참고).
Kotlin
scope.launch { val response = model.generateContent( content { image(bitmap) text("What is the object in this picture?") } ) }
자바
Content content = new Content.Builder() .addImage(bitmap) .addText("What is the object in this picture?") .build(); Executor executor = // ... ListenableFuture<GenerateContentResponse> response = model.generateContent(content); Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() { @Override public void onSuccess(GenerateContentResponse result) { String resultText = result.getText(); } @Override public void onFailure(Throwable t) { t.printStackTrace(); } }, executor);
멀티턴 채팅
멀티턴 대화를 지원할 수도 있습니다. startChat()
함수로 채팅을 초기화합니다. 원하는 경우 메시지 기록을 제공할 수 있습니다. 그런 다음 sendMessage()
함수를 호출하여 채팅 메시지를 보냅니다.
Kotlin
val chat = model.startChat( history = listOf( content(role = "user") { text("Hello, I have 2 dogs in my house.") }, content(role = "model") { text("Great to meet you. What would you like to know?") } ) ) scope.launch { val response = chat.sendMessage("How many paws are in my house?") }
자바
Content.Builder userContentBuilder = new Content.Builder(); userContentBuilder.setRole("user"); userContentBuilder.addText("Hello, I have 2 dogs in my house."); Content userContent = userContentBuilder.build(); // (Optional) create message history Content.Builder modelContentBuilder = new Content.Builder(); modelContentBuilder.setRole("model"); modelContentBuilder.addText("Great to meet you. What would you like to know?"); Content modelContent = userContentBuilder.build(); List<Content> history = Arrays.asList(userContent, modelContent); // Initialize the chat ChatFutures chat = model.startChat(history); Content.Builder userMessageBuilder = new Content.Builder(); userMessageBuilder.setRole("user"); userMessageBuilder.addText("How many paws are in my house?"); Content userMessage = userMessageBuilder.build(); Executor executor = // ... ListenableFuture<GenerateContentResponse> response = chat.sendMessage(userMessage); Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() { @Override public void onSuccess(GenerateContentResponse result) { String resultText = result.getText(); } @Override public void onFailure(Throwable t) { t.printStackTrace(); } }, executor);
응답 스트리밍
모델 생성의 전체 결과를 기다리지 않고 대신 스트리밍을 사용하여 부분 결과를 처리하면 더 빠른 상호작용을 얻을 수 있습니다. generateContentStream()
를 사용하여 응답을 스트리밍합니다.
Kotlin
someScope.launch { var outputContent = "" generativeModel.generateContentStream(inputContent) .collect { response -> outputContent += response.text } }
자바
// In Java, the method generateContentStream() returns a Publisher // from the Reactive Streams library. // https://www.reactive-streams.org/ Publisher<GenerateContentResponse> streamingResponse = model.generateContentStream(content); StringBuilder outputContent = new StringBuilder(); streamingResponse.subscribe(new Subscriber<GenerateContentResponse>() { @Override public void onNext(GenerateContentResponse generateContentResponse) { String chunk = generateContentResponse.getText(); outputContent.append(chunk); } @Override public void onComplete() { // ... } @Override public void onError(Throwable t) { t.printStackTrace(); } @Override public void onSubscribe(Subscription s) { s.request(Long.MAX_VALUE); // Request all messages } });
Android 스튜디오
Android 스튜디오에서는 시작하는 데 도움이 되는 추가 도구를 제공합니다.
- Gemini API 시작 템플릿: 이 시작 템플릿을 사용하면 Android 스튜디오에서 직접 API 키를 만들고 Gemini API를 사용하는 데 필요한 Android 종속 항목이 포함된 프로젝트를 생성할 수 있습니다.
- 생성형 AI 샘플: 이 샘플을 사용하면 Android 스튜디오에서 Android용 Google AI 클라이언트 SDK 샘플 앱을 가져올 수 있습니다.
다음 단계
- GitHub에서 Android용 Google AI 클라이언트 SDK 샘플 앱을 검토합니다.
- 공식 Google AI 문서에서 Google AI 클라이언트 SDK에 대해 자세히 알아보세요.