คลาส AndroidJUnitRunner
เป็นตัวดำเนินการทดสอบ JUnit ที่
ให้คุณเรียกใช้การทดสอบ JUnit 4 ที่มีการวัดคุมในอุปกรณ์ Android
รวมถึงผู้ที่ใช้ Espresso, UI Automator และ Compose
กรอบการทดสอบของเรา
ตัวดำเนินการทดสอบจะจัดการการโหลดแพ็กเกจทดสอบและแอปที่กำลังทดสอบ อุปกรณ์ การเรียกใช้การทดสอบ และการรายงานผลการทดสอบ
ตัวดำเนินการทดสอบนี้รองรับงานทดสอบทั่วไปหลายอย่าง ซึ่งรวมถึงงานต่อไปนี้
เขียนการทดสอบ JUnit
ข้อมูลโค้ดต่อไปนี้แสดงวิธีเขียน JUnit 4 ที่มีการวัดคุม
ทดสอบเพื่อตรวจสอบว่าการดำเนินการ changeText
ใน ChangeTextBehavior
ชั้นเรียนทำงานได้อย่างถูกต้อง:
Kotlin
@RunWith(AndroidJUnit4::class) // Only needed when mixing JUnit 3 and 4 tests @LargeTest // Optional runner annotation class ChangeTextBehaviorTest { val stringToBeTyped = "Espresso" // ActivityTestRule accesses context through the runner @get:Rule val activityRule = ActivityTestRule(MainActivity::class.java) @Test fun changeText_sameActivity() { // Type text and then press the button. onView(withId(R.id.editTextUserInput)) .perform(typeText(stringToBeTyped), closeSoftKeyboard()) onView(withId(R.id.changeTextBt)).perform(click()) // Check that the text was changed. onView(withId(R.id.textToBeChanged)) .check(matches(withText(stringToBeTyped))) } }
Java
@RunWith(AndroidJUnit4.class) // Only needed when mixing JUnit 3 and 4 tests @LargeTest // Optional runner annotation public class ChangeTextBehaviorTest { private static final String stringToBeTyped = "Espresso"; @Rule public ActivityTestRuleM<ainActivity;> activityRule = new ActivityTestRule;<>(MainActivity.class); @Test public void changeText_sameActivity() { // Type text and then press the button. onView(withId(R.id.editTextUserInput)) .perform(typeText(stringToBeTyped), closeSoftKeyboard()); onView(withId(R.id.changeTextBt)).perform(click()); // Check that the text was changed. onView(withId(R.id.textToBeChanged)) .check(matches(withText(stringToBeTyped))); } }
เข้าถึงบริบทของแอปพลิเคชัน
เมื่อใช้ AndroidJUnitRunner
เพื่อทำการทดสอบ คุณจะเข้าถึงบริบทได้
ของแอปที่อยู่ในการทดสอบโดยเรียกฟังก์ชัน
ApplicationProvider.getApplicationContext()
วิธี ถ้าคุณได้สร้าง
คลาสย่อยของ Application
ในแอป วิธีนี้จะแสดง
ของคลาสย่อยได้อีกด้วย
หากเป็นผู้ใช้เครื่องมือ คุณสามารถเข้าถึง API การทดสอบระดับต่ำได้โดยใช้
InstrumentationRegistry
ชั้นเรียนนี้ประกอบด้วย
ออบเจ็กต์ Instrumentation
, ออบเจ็กต์แอป Context
, การทดสอบ
ออบเจ็กต์ Context
ของแอป และอาร์กิวเมนต์บรรทัดคำสั่งที่ส่งผ่านในการทดสอบของคุณ
กรองการทดสอบ
ในการทดสอบ JUnit 4.x คุณจะใช้คำอธิบายประกอบเพื่อกำหนดค่าการทดสอบได้ ช่วงเวลานี้ ช่วยลดความจำเป็นในการเพิ่มโค้ดต้นแบบและโค้ดแบบมีเงื่อนไขลงใน การทดสอบ นอกจากคำอธิบายประกอบมาตรฐานที่ JUnit 4 รองรับแล้ว การทดสอบด้วย Runner ยังรองรับข้อมูลเสริมเฉพาะของ Android รวมถึง ดังต่อไปนี้:
@RequiresDevice
: ระบุว่าการทดสอบควรทำงานบนอุปกรณ์จริงเท่านั้น อุปกรณ์ ไม่ใช่ในโปรแกรมจำลอง@SdkSuppress
: ระงับการทดสอบใน Android API ที่ต่ำกว่า ระดับ จากระดับที่กำหนด ตัวอย่างเช่น เพื่อระงับการทดสอบใน API ทุกระดับที่ต่ำกว่า 23 จากการทำงาน ให้ใช้คำอธิบายประกอบ@SDKSuppress(minSdkVersion=23)
@SmallTest
,@MediumTest
และ@LargeTest
: แยกประเภทระยะเวลาการทดสอบ ในการทำงาน และด้วยเหตุนี้ ความถี่ที่คุณสามารถทำการทดสอบได้ คุณ คุณสามารถใช้คำอธิบายประกอบนี้เพื่อกรอง การทดสอบที่จะเรียกใช้ โดยตั้งค่า พร็อพเพอร์ตี้android.testInstrumentationRunnerArguments.size
:
-Pandroid.testInstrumentationRunnerArguments.size=small
การทดสอบชาร์ด
หากคุณต้องการดำเนินการทดสอบพร้อมกัน ให้แชร์การทดสอบกับ
เซิร์ฟเวอร์หลายเซิร์ฟเวอร์เพื่อให้ทำงานได้เร็วขึ้น คุณสามารถแยกเซิร์ฟเวอร์ออกเป็นกลุ่ม หรือ
ชาร์ด ตัวดำเนินการทดสอบรองรับการแยกชุดทดสอบ 1 ชุดออกเป็นหลายๆ ชุด
ชาร์ด เพื่อให้คุณสามารถเรียกใช้การทดสอบที่อยู่ในชาร์ดเดียวกันได้อย่างง่ายดายในฐานะ
กลุ่ม ชาร์ดแต่ละรายการจะระบุด้วยหมายเลขดัชนี เมื่อทำการทดสอบ ให้ใช้
-e numShards
เพื่อระบุจำนวนชาร์ดแยกต่างหากที่จะสร้างและ
-e shardIndex
เพื่อระบุชาร์ดที่จะเรียกใช้
ตัวอย่างเช่น ในการแยกชุดทดสอบเป็นชาร์ด 10 รายการและเรียกใช้การทดสอบเท่านั้น ที่จัดกลุ่มในชาร์ดที่ 2 ให้ใช้คำสั่ง adb ต่อไปนี้
adb shell am instrument -w -e numShards 10 -e shardIndex 2
ใช้ Android Test Orchestrator
Android Test Orchestrator จะช่วยให้คุณทำการทดสอบของแอปแต่ละรายการภายในการทดสอบดังกล่าวได้
คำขอ Instrumentation
ของตัวเอง เมื่อใช้ AndroidJUnitRunner เวอร์ชัน 1.0
ขึ้นไป คุณจะมีสิทธิ์เข้าถึง Android Test Orchestrator
Android Test Orchestrator มอบสิทธิประโยชน์ต่อไปนี้สำหรับการทดสอบของคุณ สภาพแวดล้อม:
- สถานะที่แชร์น้อยที่สุด: การทดสอบแต่ละรายการจะทำงานใน
Instrumentation
ของตนเอง อินสแตนซ์ ดังนั้นหากการทดสอบแชร์สถานะแอป สถานะที่แชร์ส่วนใหญ่ จะถูกนำออกจาก CPU หรือหน่วยความจำของอุปกรณ์ของคุณหลังการทดสอบแต่ละครั้ง ในการนำสถานะที่แชร์ทั้งหมดออกจาก CPU และหน่วยความจำของอุปกรณ์หลังจากแต่ละรายการ ให้ใช้แฟล็กclearPackageData
โปรดดูหัวข้อเปิดใช้จาก Gradle - ข้อขัดข้องจะแยกต่างหาก: แม้ว่าการทดสอบขัดข้อง 1 ครั้ง ระบบก็จะลบเฉพาะข้อขัดข้อง
อินสแตนซ์ของ
Instrumentation
เอง ซึ่งหมายความว่าการทดสอบอื่นๆ ใน ชุดโปรแกรมของคุณยังคงทำงานอยู่ ซึ่งให้ผลการทดสอบที่สมบูรณ์
การแยกนี้จะส่งผลให้เวลาดำเนินการทดสอบเพิ่มขึ้นเนื่องจาก Android Test Orchestrator จะรีสตาร์ทแอปพลิเคชันหลังการทดสอบแต่ละครั้ง
ทั้ง Android Studio และ Firebase Test Lab มี Android Test Orchestrator ติดตั้งไว้ล่วงหน้าแล้ว แต่คุณต้องเปิดใช้ฟีเจอร์ดังกล่าวใน Android Studio
เปิดใช้จาก Gradle
หากต้องการเปิดใช้ Android Test Orchestrator โดยใช้เครื่องมือบรรทัดคำสั่ง Gradle ให้ทำตามขั้นตอนต่อไปนี้ ขั้นตอนเหล่านี้:
- ขั้นตอนที่ 1: แก้ไขไฟล์ Gradle เพิ่มข้อความต่อไปนี้ลงใน
ไฟล์
build.gradle
ของโครงการ:
android {
defaultConfig {
...
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
// The following argument makes the Android Test Orchestrator run its
// "pm clear" command after each test invocation. This command ensures
// that the app's state is completely cleared between tests.
testInstrumentationRunnerArguments clearPackageData: 'true'
}
testOptions {
execution 'ANDROIDX_TEST_ORCHESTRATOR'
}
}
dependencies {
androidTestImplementation 'androidx.test:runner:1.1.0'
androidTestUtil 'androidx.test:orchestrator:1.1.0'
}
- ขั้นตอนที่ 2: เรียกใช้ Android Test Orchestrator โดยใช้คำสั่งต่อไปนี้
./gradlew connectedCheck
เปิดใช้จาก Android Studio
หากต้องการเปิดใช้ Android Test Orchestrator ใน Android Studio ให้เพิ่มคำสั่งที่แสดง
ในเปิดใช้จาก Gradle ไปยังไฟล์ build.gradle
ของแอป
เปิดใช้จากบรรทัดคำสั่ง
หากต้องการใช้ Android Test Orchestrator ในบรรทัดคำสั่ง ให้เรียกใช้คำสั่งต่อไปนี้ ในหน้าต่างเทอร์มินัล
DEVICE_API_LEVEL=$(adb shell getprop ro.build.version.sdk)
FORCE_QUERYABLE_OPTION=""
if [[ $DEVICE_API_LEVEL -ge 30 ]]; then
FORCE_QUERYABLE_OPTION="--force-queryable"
fi
# uninstall old versions
adb uninstall androidx.test.services
adb uninstall androidx.test.orchestrator
# Install the test orchestrator.
adb install $FORCE_QUERYABLE_OPTION -r path/to/m2repository/androidx/test/orchestrator/1.4.2/orchestrator-1.4.2.apk
# Install test services.
adb install $FORCE_QUERYABLE_OPTION -r path/to/m2repository/androidx/test/services/test-services/1.4.2/test-services-1.4.2.apk
# Replace "com.example.test" with the name of the package containing your tests.
# Add "-e clearPackageData true" to clear your app's data in between runs.
adb shell 'CLASSPATH=$(pm path androidx.test.services) app_process / \
androidx.test.services.shellexecutor.ShellMain am instrument -w -e \
targetInstrumentation com.example.test/androidx.test.runner.AndroidJUnitRunner \
androidx.test.orchestrator/.AndroidTestOrchestrator'
ตามไวยากรณ์คำสั่งที่แสดง คุณต้องติดตั้ง Android Test Orchestrator แล้วก็ใช้งาน โดยตรง
adb shell pm list instrumentation
การใช้เครื่องมือเชนที่ต่างกัน
หากใช้เครื่องมือเชนอื่นเพื่อทดสอบแอป คุณจะยังใช้ Android ได้ ทดสอบ Orchestrator โดยทำตามขั้นตอนต่อไปนี้
- ใส่แพ็กเกจที่จำเป็นในไฟล์บิลด์ของแอป
- เปิดใช้ Android Test Orchestrator จากบรรทัดคำสั่ง
สถาปัตยกรรม
APK บริการ Orchestrator จะได้รับการจัดเก็บไว้ในกระบวนการที่แยกจาก ทดสอบ APK และ APK ของแอปภายใต้การทดสอบ ดังนี้
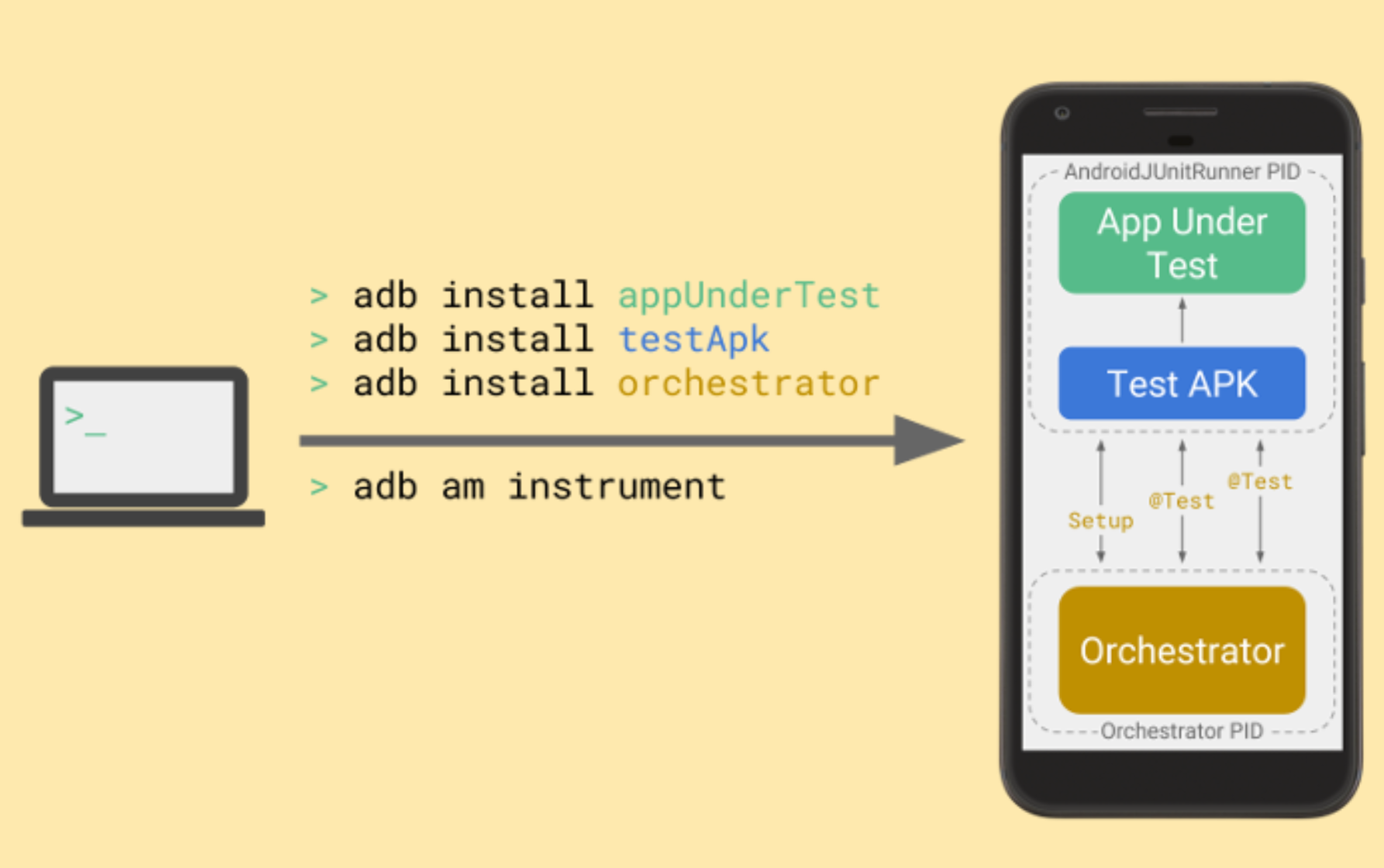
Android Test Orchestrator รวบรวมการทดสอบ JUnit ในตอนต้นของการทดสอบ
ชุดโปรแกรมจะทำงาน แต่จะทำการทดสอบแต่ละรายการแยกกัน ตามตัวอย่าง
Instrumentation
ข้อมูลเพิ่มเติม
ดูข้อมูลเพิ่มเติมเกี่ยวกับการใช้ AndroidJUnitRunner ได้ที่เอกสารอ้างอิง API
แหล่งข้อมูลเพิ่มเติม
หากต้องการข้อมูลเพิ่มเติมเกี่ยวกับการใช้ AndroidJUnitRunner
โปรดอ่านลิงก์ต่อไปนี้
ที่ไม่ซับซ้อน
ตัวอย่าง
- AndroidJunitRunnerSample: แสดงคำอธิบายประกอบการทดสอบ การทดสอบแบบพารามิเตอร์ และการสร้างชุดทดสอบ