在与电视互动时,用户通常希望操作越简单越好 内容。对于许多电视用户而言,最理想的场景就是:坐下来,打开电视,然后观看。最少的步骤 让用户观看自己喜欢的内容通常是他们更喜欢的途径。
注意:请使用本文介绍的 API 提出建议 仅限在 Android 7.1(API 级别 25)及更低版本的 Android 版本中运行的应用中。为了提供 推荐在 Android 8.0(API 级别 26)及更高版本中运行的应用,则您的应用必须使用 推荐频道。
Android 框架通过提供推荐行来协助尽可能减少输入互动 。内容推荐会在电视主屏幕的第一行显示, 用户首次使用设备时从应用的内容目录中贡献推荐内容有助于 吸引用户再次使用您的应用
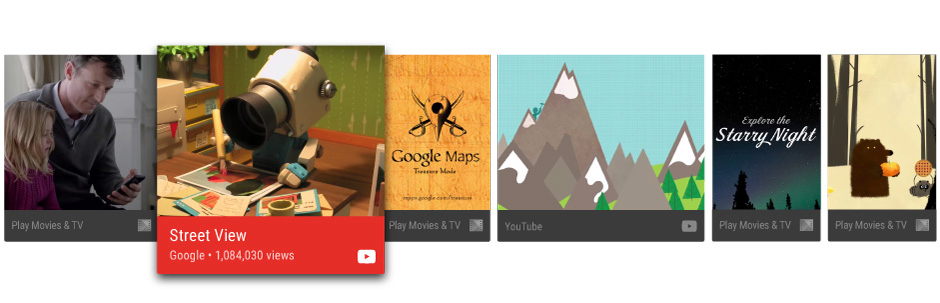
图 1. “推荐”行的示例。
本指南介绍了如何创建推荐并将其提供给 Android 框架 以便用户轻松发现和欣赏您的应用内容。另请参阅 该 <ph type="x-smartling-placeholder"></ph> Leanback 示例应用 ,了解所有最新动态。
创建推荐的最佳做法
推荐可以帮助用户快速找到他们喜爱的内容和应用。正在创建 贴合用户需求的优质推荐是创建 TV 应用提供出色的用户体验。因此,您应仔细考虑 您向用户提供的建议并对其进行密切管理。
推荐类型
创建推荐时,您应该将用户链接回未完成的观看活动或 推荐延伸至相关内容的活动。下面是一些特定类型的 建议:
- 供用户继续观看下一集的接续内容建议 观看系列视频。或者,针对已暂停的电影、电视节目或播客使用接续建议 因此用户只需点击几下即可返回观看暂停的内容。
- 新内容推荐,例如对于新的首播剧集,如果用户 已经看完了另一个系列此外,如果您的应用允许用户订阅、关注或跟踪 对所跟踪内容列表中未观看的内容使用新内容推荐。
- 根据用户的相关内容推荐历史观看行为。
如需详细了解如何设计推荐卡片以提供最佳用户体验,请参阅 Android TV 设计规范中的推荐行。
刷新推荐
刷新推荐时,不要只是将其移除并重新发布,因为这样做会导致 在“建议”行末尾显示的建议。一旦某个内容项(例如 电影,已播放, 从建议中移除。
自定义推荐
您可以通过设置界面来自定义推荐卡片以传达品牌信息 卡片的前景和背景图片、颜色、应用图标、标题和副标题等元素。 如需了解详情,请参阅 Android TV 设计规范中的推荐行。
对推荐进行分组
您可以选择根据推荐来源对推荐进行分组。例如,您的应用 可能会提供两组推荐:针对用户订阅内容的推荐、 以及推荐用户可能还未意识到的新热点内容。
在创建或更新组时,系统会分别对每个组的建议进行排名和排序 建议行。通过为推荐内容提供分组信息,您可以确保 以确保推荐内容不会列在不相关的推荐内容下方。
使用
NotificationCompat.Builder.setGroup()
,用于设置建议的分组键字符串。对于
例如,要将某条推荐标记为属于某个包含新热点内容的群组,
则可以调用 setGroup("trending")
。
创建推荐服务
内容推荐是通过后台处理创建的。为了让您的应用 来为推荐内容做贡献,不妨创建一项服务,定期从您的网站 添加到系统的推荐列表。
以下代码示例说明了如何将 IntentService
扩展到
为应用创建推荐服务:
Kotlin
class UpdateRecommendationsService : IntentService("RecommendationService") { override protected fun onHandleIntent(intent: Intent) { Log.d(TAG, "Updating recommendation cards") val recommendations = VideoProvider.getMovieList() if (recommendations == null) return var count = 0 try { val builder = RecommendationBuilder() .setContext(applicationContext) .setSmallIcon(R.drawable.videos_by_google_icon) for (entry in recommendations.entrySet()) { for (movie in entry.getValue()) { Log.d(TAG, "Recommendation - " + movie.getTitle()) builder.setBackground(movie.getCardImageUrl()) .setId(count + 1) .setPriority(MAX_RECOMMENDATIONS - count) .setTitle(movie.getTitle()) .setDescription(getString(R.string.popular_header)) .setImage(movie.getCardImageUrl()) .setIntent(buildPendingIntent(movie)) .build() if (++count >= MAX_RECOMMENDATIONS) { break } } if (++count >= MAX_RECOMMENDATIONS) { break } } } catch (e: IOException) { Log.e(TAG, "Unable to update recommendation", e) } } private fun buildPendingIntent(movie: Movie): PendingIntent { val detailsIntent = Intent(this, DetailsActivity::class.java) detailsIntent.putExtra("Movie", movie) val stackBuilder = TaskStackBuilder.create(this) stackBuilder.addParentStack(DetailsActivity::class.java) stackBuilder.addNextIntent(detailsIntent) // Ensure a unique PendingIntents, otherwise all // recommendations end up with the same PendingIntent detailsIntent.setAction(movie.getId().toString()) val intent = stackBuilder.getPendingIntent(0, PendingIntent.FLAG_UPDATE_CURRENT) return intent } companion object { private val TAG = "UpdateRecommendationsService" private val MAX_RECOMMENDATIONS = 3 } }
Java
public class UpdateRecommendationsService extends IntentService { private static final String TAG = "UpdateRecommendationsService"; private static final int MAX_RECOMMENDATIONS = 3; public UpdateRecommendationsService() { super("RecommendationService"); } @Override protected void onHandleIntent(Intent intent) { Log.d(TAG, "Updating recommendation cards"); HashMap<String, List<Movie>> recommendations = VideoProvider.getMovieList(); if (recommendations == null) return; int count = 0; try { RecommendationBuilder builder = new RecommendationBuilder() .setContext(getApplicationContext()) .setSmallIcon(R.drawable.videos_by_google_icon); for (Map.Entry<String, List<Movie>> entry : recommendations.entrySet()) { for (Movie movie : entry.getValue()) { Log.d(TAG, "Recommendation - " + movie.getTitle()); builder.setBackground(movie.getCardImageUrl()) .setId(count + 1) .setPriority(MAX_RECOMMENDATIONS - count) .setTitle(movie.getTitle()) .setDescription(getString(R.string.popular_header)) .setImage(movie.getCardImageUrl()) .setIntent(buildPendingIntent(movie)) .build(); if (++count >= MAX_RECOMMENDATIONS) { break; } } if (++count >= MAX_RECOMMENDATIONS) { break; } } } catch (IOException e) { Log.e(TAG, "Unable to update recommendation", e); } } private PendingIntent buildPendingIntent(Movie movie) { Intent detailsIntent = new Intent(this, DetailsActivity.class); detailsIntent.putExtra("Movie", movie); TaskStackBuilder stackBuilder = TaskStackBuilder.create(this); stackBuilder.addParentStack(DetailsActivity.class); stackBuilder.addNextIntent(detailsIntent); // Ensure a unique PendingIntents, otherwise all // recommendations end up with the same PendingIntent detailsIntent.setAction(Long.toString(movie.getId())); PendingIntent intent = stackBuilder.getPendingIntent(0, PendingIntent.FLAG_UPDATE_CURRENT); return intent; } }
为了让系统能够识别并运行此服务,请使用您的 应用清单中。以下代码段说明了如何将此类声明为服务:
<manifest ... > <application ... > ... <service android:name="com.example.android.tvleanback.UpdateRecommendationsService" android:enabled="true" /> </application> </manifest>
构建推荐
推荐服务开始运行后,必须创建推荐内容并将其传递给
Android 框架。框架以 Notification
对象的形式接收推荐,这些对象使用特定的模板并使用特定的标记
类别。
设置值
如需为推荐卡片设置界面元素值,请创建一个构建器类,该类遵循 如下所述的构建器模式首先,设置建议卡片的值 元素。
Kotlin
class RecommendationBuilder { ... fun setTitle(title: String): RecommendationBuilder { this.title = title return this } fun setDescription(description: String): RecommendationBuilder { this.description = description return this } fun setImage(uri: String): RecommendationBuilder { imageUri = uri return this } fun setBackground(uri: String): RecommendationBuilder { backgroundUri = uri return this } ...
Java
public class RecommendationBuilder { ... public RecommendationBuilder setTitle(String title) { this.title = title; return this; } public RecommendationBuilder setDescription(String description) { this.description = description; return this; } public RecommendationBuilder setImage(String uri) { imageUri = uri; return this; } public RecommendationBuilder setBackground(String uri) { backgroundUri = uri; return this; } ...
创建通知
设置值后,您就可以通过构建器分配值来构建通知了
类添加到通知中,然后调用 NotificationCompat.Builder.build()
。
此外,请务必调用
setLocalOnly()
因此NotificationCompat.BigPictureStyle
通知不会显示
。
以下代码示例演示了如何构建建议。
Kotlin
class RecommendationBuilder { ... @Throws(IOException::class) fun build(): Notification { ... val notification = NotificationCompat.BigPictureStyle( NotificationCompat.Builder(context) .setContentTitle(title) .setContentText(description) .setPriority(priority) .setLocalOnly(true) .setOngoing(true) .setColor(context.resources.getColor(R.color.fastlane_background)) .setCategory(Notification.CATEGORY_RECOMMENDATION) .setLargeIcon(image) .setSmallIcon(smallIcon) .setContentIntent(intent) .setExtras(extras)) .build() return notification } }
Java
public class RecommendationBuilder { ... public Notification build() throws IOException { ... Notification notification = new NotificationCompat.BigPictureStyle( new NotificationCompat.Builder(context) .setContentTitle(title) .setContentText(description) .setPriority(priority) .setLocalOnly(true) .setOngoing(true) .setColor(context.getResources().getColor(R.color.fastlane_background)) .setCategory(Notification.CATEGORY_RECOMMENDATION) .setLargeIcon(image) .setSmallIcon(smallIcon) .setContentIntent(intent) .setExtras(extras)) .build(); return notification; } }
运行推荐服务
应用的推荐服务必须定期运行,以便创建最新的
建议。要运行您的服务,请创建一个运行计时器并调用
定期更新。以下代码示例扩展了 BroadcastReceiver
类,以开始定期执行推荐服务
每半小时:
Kotlin
class BootupActivity : BroadcastReceiver() { override fun onReceive(context: Context, intent: Intent) { Log.d(TAG, "BootupActivity initiated") if (intent.action.endsWith(Intent.ACTION_BOOT_COMPLETED)) { scheduleRecommendationUpdate(context) } } private fun scheduleRecommendationUpdate(context: Context) { Log.d(TAG, "Scheduling recommendations update") val alarmManager = context.getSystemService(Context.ALARM_SERVICE) as AlarmManager val recommendationIntent = Intent(context, UpdateRecommendationsService::class.java) val alarmIntent = PendingIntent.getService(context, 0, recommendationIntent, 0) alarmManager.setInexactRepeating(AlarmManager.ELAPSED_REALTIME_WAKEUP, INITIAL_DELAY, AlarmManager.INTERVAL_HALF_HOUR, alarmIntent ) } companion object { private val TAG = "BootupActivity" private val INITIAL_DELAY:Long = 5000 } }
Java
public class BootupActivity extends BroadcastReceiver { private static final String TAG = "BootupActivity"; private static final long INITIAL_DELAY = 5000; @Override public void onReceive(Context context, Intent intent) { Log.d(TAG, "BootupActivity initiated"); if (intent.getAction().endsWith(Intent.ACTION_BOOT_COMPLETED)) { scheduleRecommendationUpdate(context); } } private void scheduleRecommendationUpdate(Context context) { Log.d(TAG, "Scheduling recommendations update"); AlarmManager alarmManager = (AlarmManager) context.getSystemService(Context.ALARM_SERVICE); Intent recommendationIntent = new Intent(context, UpdateRecommendationsService.class); PendingIntent alarmIntent = PendingIntent.getService(context, 0, recommendationIntent, 0); alarmManager.setInexactRepeating(AlarmManager.ELAPSED_REALTIME_WAKEUP, INITIAL_DELAY, AlarmManager.INTERVAL_HALF_HOUR, alarmIntent); } }
BroadcastReceiver
类的这一实现必须在启动之后运行
电视设备上的内容为此,请在您的应用中注册此类
清单中包含 intent 过滤器,该 intent 过滤器会监听设备启动过程的完成情况。通过
以下示例代码演示了如何将此配置添加到清单中:
<manifest ... > <application ... > <receiver android:name="com.example.android.tvleanback.BootupActivity" android:enabled="true" android:exported="false"> <intent-filter> <action android:name="android.intent.action.BOOT_COMPLETED"/> </intent-filter> </receiver> </application> </manifest>
重要提示:为了接收启动完成通知,您的应用
请求 RECEIVE_BOOT_COMPLETED
权限。
如需了解详情,请参阅 ACTION_BOOT_COMPLETED
。
在您的推荐服务类中onHandleIntent()
方法,按以下方式将建议发布给经理:
Kotlin
val notification = notificationBuilder.build() notificationManager.notify(id, notification)
Java
Notification notification = notificationBuilder.build(); notificationManager.notify(id, notification);