במכשירים עם Android מגרסה 8.0 (API ברמה 26) ואילך, ההתאמה של מכשיר נלווה מבצעת סריקה של מכשירי Bluetooth או Wi-Fi בקרבת מקום בשם האפליקציה, בלי שתצטרכו להעניק לה את ההרשאה ACCESS_FINE_LOCATION
. כך אפשר להגן בצורה מקסימלית על פרטיות המשתמשים. משתמשים בשיטה הזו כדי לבצע את ההגדרה הראשונית של מכשיר השותף, כמו שעון חכם עם תמיכה ב-BLE. בנוסף, כדי להתאים מכשיר נלווה צריך להפעיל את שירותי המיקום.
התאמת מכשיר נלווה לא יוצרת חיבורים בעצמה ולא מפעילה סריקת נתונים רציפה. אפליקציות יכולות להשתמש בממשקי API של קישוריות Bluetooth או Wi-Fi כדי ליצור חיבורים.
אחרי שמתאימים את המכשיר, הוא יכול להשתמש בהרשאות REQUEST_COMPANION_RUN_IN_BACKGROUND
ו-REQUEST_COMPANION_USE_DATA_IN_BACKGROUND
כדי להפעיל את האפליקציה מהרקע. אפליקציות יכולות גם להשתמש בהרשאה REQUEST_COMPANION_START_FOREGROUND_SERVICES_FROM_BACKGROUND
כדי להפעיל שירות שפועל בחזית מהרקע.
משתמש יכול לבחור מכשיר מהרשימה ולהעניק לאפליקציה הרשאות גישה למכשיר. ההרשאות האלה יבוטלו אם תסירו את האפליקציה או שתתקשרו למספר disassociate()
.
האפליקציה הנלווית אחראית לנקות את השיוך שלה אם המשתמש כבר לא זקוק לו, למשל כשהוא יוצא מהחשבון או מסיר מכשירים מקושרים.
הטמעת התאמה של מכשיר נלווה
בקטע הזה מוסבר איך משתמשים ב-CompanionDeviceManager
כדי להתאים את האפליקציה למכשירים נלווים באמצעות Bluetooth, BLE ו-Wi-Fi.
ציון מכשירים נלווים
בדוגמת הקוד הבאה מוסבר איך מוסיפים את הדגל <uses-feature>
לקובץ מניפסט. כך המערכת תדע שהאפליקציה שלכם מתכוונת להגדיר מכשירי שותף.
<uses-feature android:name="android.software.companion_device_setup"/>
הצגת רשימת מכשירים לפי DeviceFilter
אפשר להציג את כל מכשירי השותף שנמצאים בטווח ועומדים בDeviceFilter
שציינתם (כפי שמוצג באיור 1). אם רוצים להגביל את הסריקה למכשיר אחד בלבד, אפשר להגדיר את setSingleDevice()
ל-true
(כפי שמוצג באיור 2).
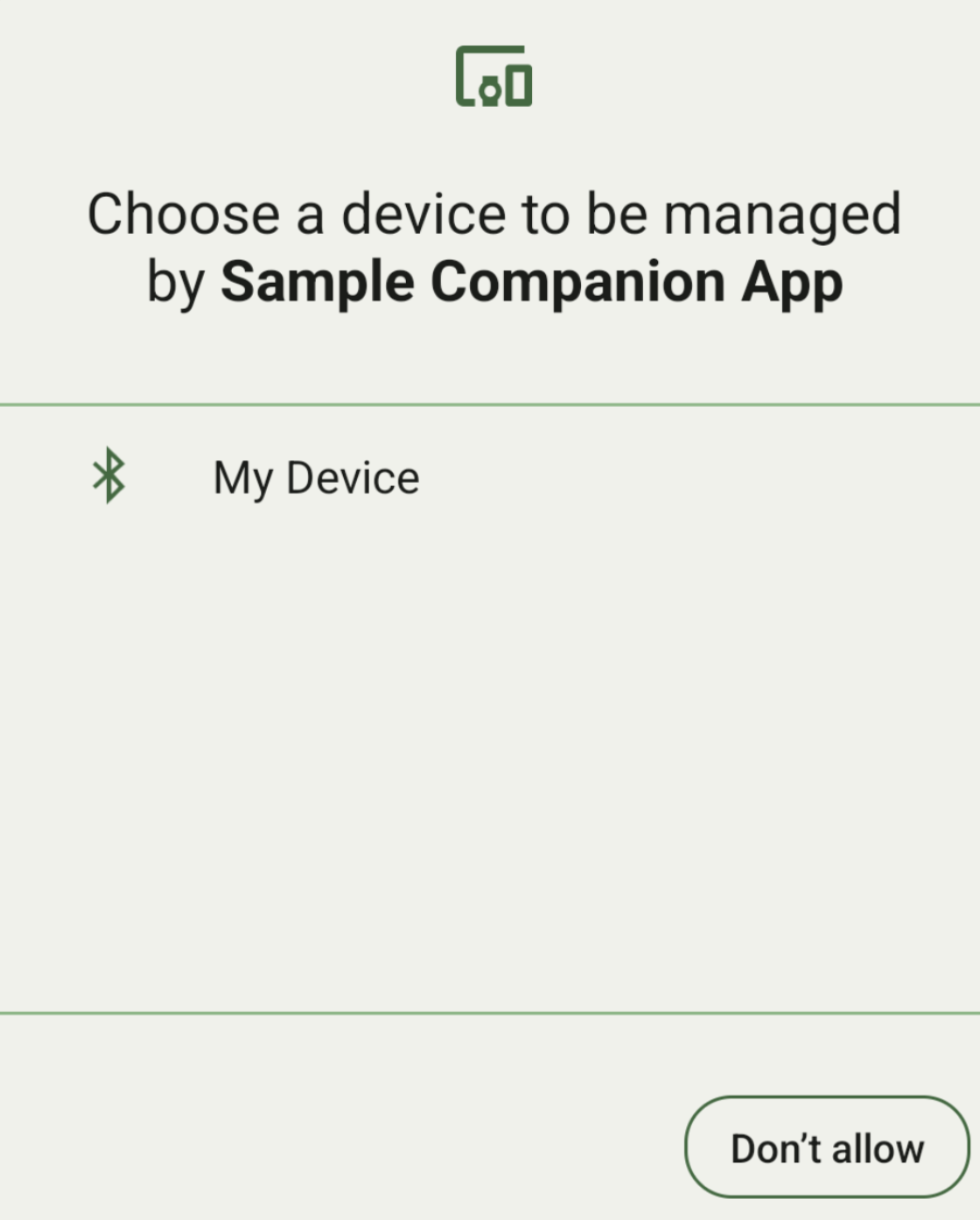
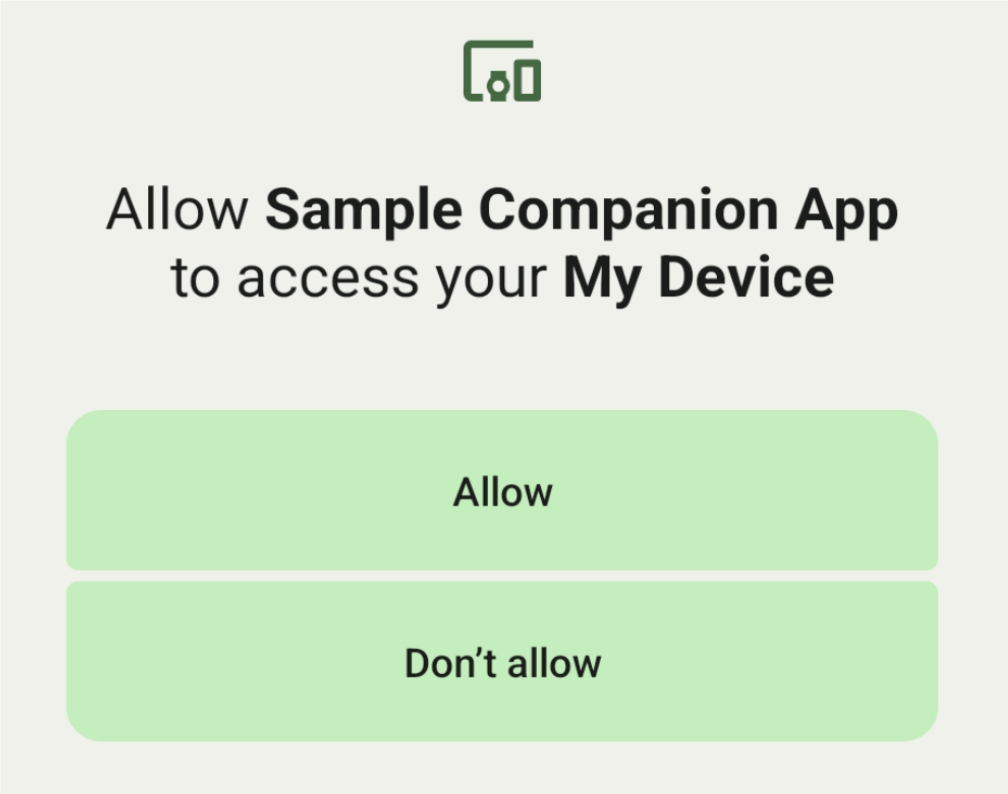
אלה תתי-הקטגוריות של DeviceFilter
שאפשר לציין ב-AssociationRequest
:
לכל שלוש המחלקות המשניות יש בוני גרסת build שמפשטים את הגדרת המסננים.
בדוגמה הבאה, מכשיר סורק מכשיר Bluetooth עם BluetoothDeviceFilter
.
Kotlin
val deviceFilter: BluetoothDeviceFilter = BluetoothDeviceFilter.Builder() // Match only Bluetooth devices whose name matches the pattern. .setNamePattern(Pattern.compile("My device")) // Match only Bluetooth devices whose service UUID matches this pattern. .addServiceUuid(ParcelUuid(UUID(0x123abcL, -1L)), null) .build()
Java
BluetoothDeviceFilter deviceFilter = new BluetoothDeviceFilter.Builder() // Match only Bluetooth devices whose name matches the pattern. .setNamePattern(Pattern.compile("My device")) // Match only Bluetooth devices whose service UUID matches this pattern. .addServiceUuid(new ParcelUuid(new UUID(0x123abcL, -1L)), null) .build();
מגדירים DeviceFilter
ל-AssociationRequest
כדי ש-CompanionDeviceManager
יוכל לקבוע את סוג המכשירים לחיפוש.
Kotlin
val pairingRequest: AssociationRequest = AssociationRequest.Builder() // Find only devices that match this request filter. .addDeviceFilter(deviceFilter) // Stop scanning as soon as one device matching the filter is found. .setSingleDevice(true) .build()
Java
AssociationRequest pairingRequest = new AssociationRequest.Builder() // Find only devices that match this request filter. .addDeviceFilter(deviceFilter) // Stop scanning as soon as one device matching the filter is found. .setSingleDevice(true) .build();
אחרי שהאפליקציה מאתחלת AssociationRequest
, מריצים את הפונקציה associate()
ב-CompanionDeviceManager
. הפונקציה associate()
מקבלת AssociationRequest
ו-Callback
.
הפונקציה Callback
מחזירה את הערך IntentSender
ב-onAssociationPending
כש-CompanionDeviceManager
מאתרת מכשיר והוא מוכן להציג תיבת דו-שיח להבעת הסכמה של המשתמש.
אחרי שהמשתמש מאשר את המכשיר, AssociationInfo
של המכשיר מוחזר ב-onAssociationCreated
.
אם האפליקציה לא תמצא מכשירים, הקריאה החוזרת תחזיר את הערך onFailure
עם הודעת שגיאה.
במכשירים שמותקנת בהם גרסת Android 13 (API ברמה 33) ואילך:
Kotlin
val deviceManager = requireContext().getSystemService(Context.COMPANION_DEVICE_SERVICE) val executor: Executor = Executor { it.run() } deviceManager.associate(pairingRequest, executor, object : CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user // can select the device they want to pair with. override fun onAssociationPending(intentSender: IntentSender) { intentSender?.let { startIntentSenderForResult(it, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } } override fun onAssociationCreated(associationInfo: AssociationInfo) { // An association is created. } override fun onFailure(errorMessage: CharSequence?) { // To handle the failure. } })
Java
CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService(Context.COMPANION_DEVICE_SERVICE); Executor executor = new Executor() { @Override public void execute(Runnable runnable) { runnable.run(); } }; deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { executor, // Called when a device is found. Launch the IntentSender so the user can // select the device they want to pair with. @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult( chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0 ); } catch (IntentSender.SendIntentException e) { Log.e("MainActivity", "Failed to send intent"); } } @Override public void onAssociationCreated(AssociationInfo associationInfo) { // An association is created. } @Override public void onFailure(CharSequence errorMessage) { // To handle the failure. });
במכשירים עם Android 12L (API ברמה 32) וגרסאות קודמות (הווצא משימוש):
Kotlin
val deviceManager = requireContext().getSystemService(Context.COMPANION_DEVICE_SERVICE) deviceManager.associate(pairingRequest, object : CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user // can select the device they want to pair with. override fun onDeviceFound(chooserLauncher: IntentSender) { startIntentSenderForResult(chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } override fun onFailure(error: CharSequence?) { // To handle the failure. } }, null)
Java
CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService(Context.COMPANION_DEVICE_SERVICE); deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user can // select the device they want to pair with. @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult( chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0 ); } catch (IntentSender.SendIntentException e) { Log.e("MainActivity", "Failed to send intent"); } } @Override public void onFailure(CharSequence error) { // To handle the failure. } }, null);
התוצאה של הבחירה של המשתמש נשלחת חזרה לקטע ב-onActivityResult()
של הפעילות. לאחר מכן תוכלו לגשת למכשיר שנבחר.
כשהמשתמש בוחר מכשיר Bluetooth, צפוי להופיע האירוע BluetoothDevice
.
כשהמשתמש בוחר מכשיר Bluetooth LE, צפוי להופיע הערך android.bluetooth.le.ScanResult
.
כשהמשתמש בוחר מכשיר Wi-Fi, צפוי להופיע android.net.wifi.ScanResult
.
Kotlin
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) { when (requestCode) { SELECT_DEVICE_REQUEST_CODE -> when(resultCode) { Activity.RESULT_OK -> { // The user chose to pair the app with a Bluetooth device. val deviceToPair: BluetoothDevice? = data?.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE) deviceToPair?.let { device -> device.createBond() // Continue to interact with the paired device. } } } else -> super.onActivityResult(requestCode, resultCode, data) } }
Java
@Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { if (resultCode != Activity.RESULT_OK) { return; } if (requestCode == SELECT_DEVICE_REQUEST_CODE && data != null) { BluetoothDevice deviceToPair = data.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE); if (deviceToPair != null) { deviceToPair.createBond(); // Continue to interact with the paired device. } } else { super.onActivityResult(requestCode, resultCode, data); } }
הדוגמה המלאה:
במכשירים שמותקנת בהם גרסת Android 13 (API ברמה 33) ואילך:
Kotlin
private const val SELECT_DEVICE_REQUEST_CODE = 0 class MainActivity : AppCompatActivity() { private val deviceManager: CompanionDeviceManager by lazy { getSystemService(Context.COMPANION_DEVICE_SERVICE) as CompanionDeviceManager } val mBluetoothAdapter: BluetoothAdapter by lazy { val java = BluetoothManager::class.java getSystemService(java)!!.adapter } val executor: Executor = Executor { it.run() } override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // To skip filters based on names and supported feature flags (UUIDs), // omit calls to setNamePattern() and addServiceUuid() // respectively, as shown in the following Bluetooth example. val deviceFilter: BluetoothDeviceFilter = BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid(ParcelUuid(UUID(0x123abcL, -1L)), null) .build() // The argument provided in setSingleDevice() determines whether a single // device name or a list of them appears. val pairingRequest: AssociationRequest = AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build() // When the app tries to pair with a Bluetooth device, show the // corresponding dialog box to the user. deviceManager.associate(pairingRequest, executor, object : CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user // can select the device they want to pair with. override fun onAssociationPending(intentSender: IntentSender) { intentSender?.let { startIntentSenderForResult(it, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } } override fun onAssociationCreated(associationInfo: AssociationInfo) { // AssociationInfo object is created and get association id and the // macAddress. var associationId: int = associationInfo.id var macAddress: MacAddress = associationInfo.deviceMacAddress } override fun onFailure(errorMessage: CharSequence?) { // Handle the failure. } ) override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) { when (requestCode) { SELECT_DEVICE_REQUEST_CODE -> when(resultCode) { Activity.RESULT_OK -> { // The user chose to pair the app with a Bluetooth device. val deviceToPair: BluetoothDevice? = data?.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE) deviceToPair?.let { device -> device.createBond() // Maintain continuous interaction with a paired device. } } } else -> super.onActivityResult(requestCode, resultCode, data) } } }
Java
class MainActivityJava extends AppCompatActivity { private static final int SELECT_DEVICE_REQUEST_CODE = 0; Executor executor = new Executor() { @Override public void execute(Runnable runnable) { runnable.run(); } }; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService( Context.COMPANION_DEVICE_SERVICE ); // To skip filtering based on name and supported feature flags, // do not include calls to setNamePattern() and addServiceUuid(), // respectively. This example uses Bluetooth. BluetoothDeviceFilter deviceFilter = new BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid( new ParcelUuid(new UUID(0x123abcL, -1L)), null ) .build(); // The argument provided in setSingleDevice() determines whether a single // device name or a list of device names is presented to the user as // pairing options. AssociationRequest pairingRequest = new AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build(); // When the app tries to pair with the Bluetooth device, show the // appropriate pairing request dialog to the user. deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { executor, // Called when a device is found. Launch the IntentSender so the user can // select the device they want to pair with. @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult( chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0 ); } catch (IntentSender.SendIntentException e) { Log.e("MainActivity", "Failed to send intent"); } } @Override public void onAssociationCreated(AssociationInfo associationInfo) { // AssociationInfo object is created and get association id and the // macAddress. int associationId = associationInfo.getId(); MacAddress macAddress = associationInfo.getDeviceMacAddress(); } @Override public void onFailure(CharSequence errorMessage) { // Handle the failure. }); } @Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { if (resultCode != Activity.RESULT_OK) { return; } if (requestCode == SELECT_DEVICE_REQUEST_CODE) { if (resultCode == Activity.RESULT_OK && data != null) { BluetoothDevice deviceToPair = data.getParcelableExtra( CompanionDeviceManager.EXTRA_DEVICE ); if (deviceToPair != null) { deviceToPair.createBond(); // ... Continue interacting with the paired device. } } } else { super.onActivityResult(requestCode, resultCode, data); } } }
במכשירים עם Android 12L (API ברמה 32) וגרסאות קודמות (הווצא משימוש):
Kotlin
private const val SELECT_DEVICE_REQUEST_CODE = 0 class MainActivity : AppCompatActivity() { private val deviceManager: CompanionDeviceManager by lazy { getSystemService(Context.COMPANION_DEVICE_SERVICE) as CompanionDeviceManager } override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // To skip filters based on names and supported feature flags (UUIDs), // omit calls to setNamePattern() and addServiceUuid() // respectively, as shown in the following Bluetooth example. val deviceFilter: BluetoothDeviceFilter = BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid(ParcelUuid(UUID(0x123abcL, -1L)), null) .build() // The argument provided in setSingleDevice() determines whether a single // device name or a list of them appears. val pairingRequest: AssociationRequest = AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build() // When the app tries to pair with a Bluetooth device, show the // corresponding dialog box to the user. deviceManager.associate(pairingRequest, object : CompanionDeviceManager.Callback() { override fun onDeviceFound(chooserLauncher: IntentSender) { startIntentSenderForResult(chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } override fun onFailure(error: CharSequence?) { // Handle the failure. } }, null) } override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) { when (requestCode) { SELECT_DEVICE_REQUEST_CODE -> when(resultCode) { Activity.RESULT_OK -> { // The user chose to pair the app with a Bluetooth device. val deviceToPair: BluetoothDevice? = data?.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE) deviceToPair?.let { device -> device.createBond() // Maintain continuous interaction with a paired device. } } } else -> super.onActivityResult(requestCode, resultCode, data) } } }
Java
class MainActivityJava extends AppCompatActivity { private static final int SELECT_DEVICE_REQUEST_CODE = 0; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService( Context.COMPANION_DEVICE_SERVICE ); // To skip filtering based on name and supported feature flags, // don't include calls to setNamePattern() and addServiceUuid(), // respectively. This example uses Bluetooth. BluetoothDeviceFilter deviceFilter = new BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid( new ParcelUuid(new UUID(0x123abcL, -1L)), null ) .build(); // The argument provided in setSingleDevice() determines whether a single // device name or a list of device names is presented to the user as // pairing options. AssociationRequest pairingRequest = new AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build(); // When the app tries to pair with the Bluetooth device, show the // appropriate pairing request dialog to the user. deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult(chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0); } catch (IntentSender.SendIntentException e) { // failed to send the intent } } @Override public void onFailure(CharSequence error) { // handle failure to find the companion device } }, null); } @Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { if (requestCode == SELECT_DEVICE_REQUEST_CODE) { if (resultCode == Activity.RESULT_OK && data != null) { BluetoothDevice deviceToPair = data.getParcelableExtra( CompanionDeviceManager.EXTRA_DEVICE ); if (deviceToPair != null) { deviceToPair.createBond(); // ... Continue interacting with the paired device. } } } else { super.onActivityResult(requestCode, resultCode, data); } } }
פרופילים של מכשירים נלווים
ב-Android 12 (API ברמה 31) ואילך, אפליקציות נלוות שמנהלות מכשירים כמו שעונים יכולות להשתמש בפרופילים של מכשירים נלווים כדי לייעל את תהליך ההגדרה על ידי מתן ההרשאות הנדרשות במהלך ההתאמה. למידע נוסף, ראו פרופילים של מכשירי נלווה.
איך לא לאפשר לאפליקציות נלוות להיכנס למצב שינה
החל מ-Android 16 (רמת API 36),
האפשרויות CompanionDeviceManager.startObservingDevicePresence(String)
ו-CompanionDeviceService.onDeviceAppeared()
הוצאו משימוש.
כדאי להשתמש ב-
CompanionDeviceManager.startObservingDevicePresence (ObservingDevicePresenceRequest)
כדי לנהל באופן אוטומטי את קישור ה-CompanionDeviceService
שהטמעתם.- מצב הקישור של
CompanionDeviceService
מנוהל באופן אוטומטי על סמך סטטוס הנוכחות של המכשיר הנלווה המשויך אליו:- השירות מחויב כשמכשיר השותף נמצא בטווח ה-BLE או מחובר באמצעות Bluetooth.
- השירות משוחרר כשמכשיר השותף יוצא מטווח ה-BLE או כשחיבור ה-Bluetooth שלו מסתיים.
- מצב הקישור של
האפליקציה תקבל שיחה חוזרת על סמך אירועים שונים של
DevicePresenceEvent
.פרטים נוספים זמינים במאמר
CompanionDeviceService.onDeviceEvent()
.