Na urządzeniach z Androidem 8.0 (poziom interfejsu API 26) lub nowszym parowanie urządzenia towarzyszącego skanuje w imieniu aplikacji pobliskie urządzenia Bluetooth lub Wi-Fi bez konieczności uzyskiwania uprawnienia ACCESS_FINE_LOCATION
. Pomaga to w maksymalizacji ochrony prywatności użytkowników. Używaj tej metody do wstępnej konfiguracji urządzenia towarzyszącego, takiego jak inteligentny zegarek obsługujący technologię BLE. Ponadto parowanie urządzenia towarzyszącego wymaga włączenia usług lokalizacyjnych.
Parowanie urządzenia towarzyszącego nie tworzy połączeń samodzielnie ani nie umożliwia ciągłego skanowania. Aplikacje mogą używać interfejsów API łączności Bluetooth lub Wi-Fi do nawiązywania połączeń.
Po sparowaniu urządzenie może używać uprawnień REQUEST_COMPANION_RUN_IN_BACKGROUND
i REQUEST_COMPANION_USE_DATA_IN_BACKGROUND
, aby uruchamiać aplikację w tle. Aplikacje mogą też używać uprawnienia REQUEST_COMPANION_START_FOREGROUND_SERVICES_FROM_BACKGROUND
do uruchamiania usługi na pierwszym planie z tła.
Użytkownik może wybrać urządzenie z listy i przyznać aplikacji uprawnienia dostępu do tego urządzenia. Te uprawnienia zostaną cofnięte, jeśli odinstalujesz aplikację lub zadzwonisz do disassociate()
.
Aplikacja towarzysząca jest odpowiedzialna za usuwanie własnych powiązań, jeśli użytkownik ich już nie potrzebuje, np. gdy się wyloguje lub usunie powiązane urządzenia.
Wdrożenie parowania urządzeń towarzyszących
W tej sekcji wyjaśniamy, jak za pomocą CompanionDeviceManager
sparować aplikację z urządzeniami towarzyszącymi przez Bluetooth, BLE i Wi-Fi.
Określanie urządzeń towarzyszących
Poniższy przykładowy kod pokazuje, jak dodać flagę <uses-feature>
do pliku manifestu. To informuje system, że Twoja aplikacja ma zamiar skonfigurować urządzenia towarzyszące.
<uses-feature android:name="android.software.companion_device_setup"/>
Wyświetl listę urządzeń według DeviceFilter
Możesz wyświetlać wszystkie urządzenia towarzyszące w zasięgu, które pasują do podanych przez Ciebie DeviceFilter
(patrz rysunek 1). Jeśli chcesz ograniczyć skanowanie tylko do jednego urządzenia, możesz ustawić wartość parametru setSingleDevice()
na true
(jak pokazano na rysunku 2).
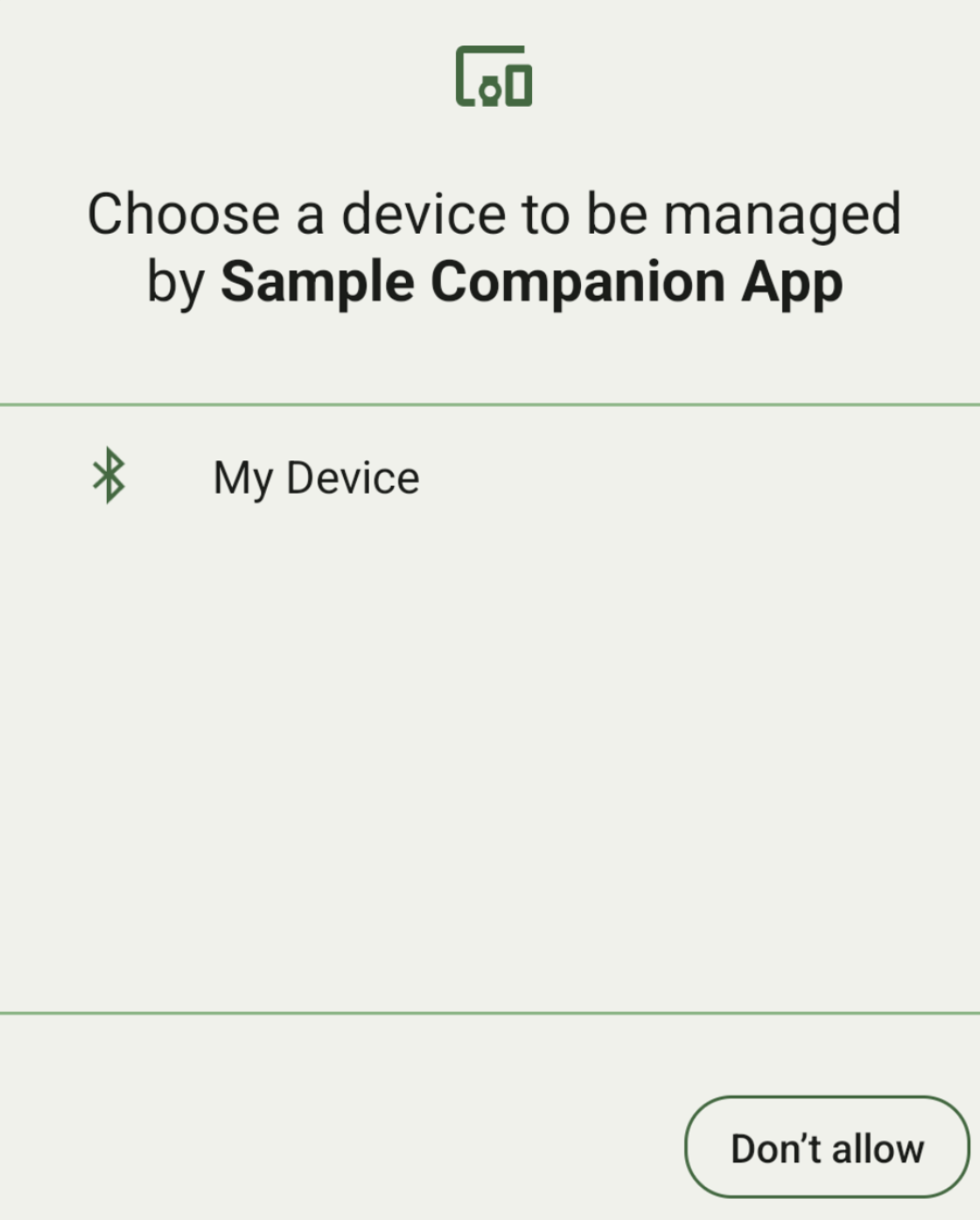
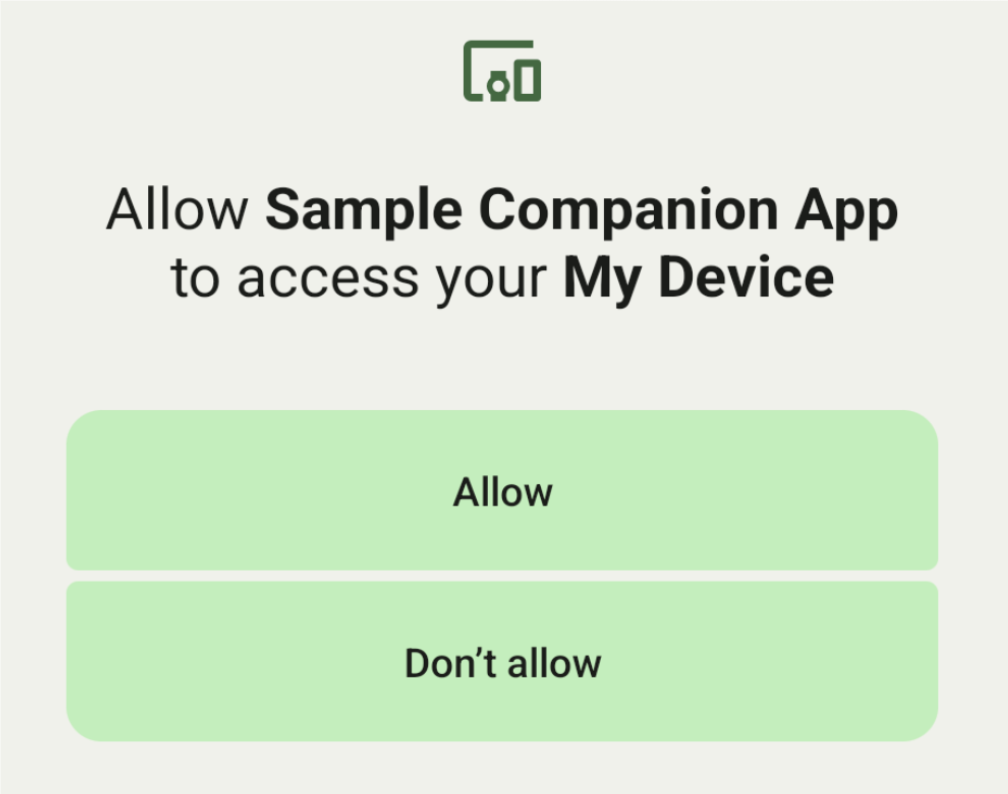
Oto podklasy DeviceFilter
, które można określić w AssociationRequest
:
Wszystkie 3 podklasy mają kreatory, które upraszczają konfigurowanie filtrów.
W tym przykładzie urządzenie skanuje w poszukiwaniu urządzenia Bluetooth z BluetoothDeviceFilter
.
Kotlin
val deviceFilter: BluetoothDeviceFilter = BluetoothDeviceFilter.Builder() // Match only Bluetooth devices whose name matches the pattern. .setNamePattern(Pattern.compile("My device")) // Match only Bluetooth devices whose service UUID matches this pattern. .addServiceUuid(ParcelUuid(UUID(0x123abcL, -1L)), null) .build()
Java
BluetoothDeviceFilter deviceFilter = new BluetoothDeviceFilter.Builder() // Match only Bluetooth devices whose name matches the pattern. .setNamePattern(Pattern.compile("My device")) // Match only Bluetooth devices whose service UUID matches this pattern. .addServiceUuid(new ParcelUuid(new UUID(0x123abcL, -1L)), null) .build();
Ustaw wartość parametru DeviceFilter
na AssociationRequest
, aby określić, jakiego typu urządzenia mają być wyszukiwane.CompanionDeviceManager
Kotlin
val pairingRequest: AssociationRequest = AssociationRequest.Builder() // Find only devices that match this request filter. .addDeviceFilter(deviceFilter) // Stop scanning as soon as one device matching the filter is found. .setSingleDevice(true) .build()
Java
AssociationRequest pairingRequest = new AssociationRequest.Builder() // Find only devices that match this request filter. .addDeviceFilter(deviceFilter) // Stop scanning as soon as one device matching the filter is found. .setSingleDevice(true) .build();
Po zainicjowaniu przez aplikację obiektu AssociationRequest
uruchom funkcję associate()
CompanionDeviceManager
. Funkcja associate()
przyjmuje argumenty AssociationRequest
i Callback
.
Funkcja Callback
zwraca wartośćIntentSender
w onAssociationPending
, gdy CompanionDeviceManager
znajdzie urządzenie i będzie gotowe do wyświetlenia okna z prośbą o zgodę użytkownika.
Gdy użytkownik potwierdzi urządzenie, AssociationInfo
urządzenia zostanie zwrócone w onAssociationCreated
.
Jeśli aplikacja nie znajdzie żadnych urządzeń, wywołanie zwrotne zwróci wartość onFailure
z komunikatem o błędzie.
Na urządzeniach z Androidem 13 (poziom API 33) lub nowszym:
Kotlin
val deviceManager = requireContext().getSystemService(Context.COMPANION_DEVICE_SERVICE) val executor: Executor = Executor { it.run() } deviceManager.associate(pairingRequest, executor, object : CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user // can select the device they want to pair with. override fun onAssociationPending(intentSender: IntentSender) { intentSender?.let { startIntentSenderForResult(it, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } } override fun onAssociationCreated(associationInfo: AssociationInfo) { // An association is created. } override fun onFailure(errorMessage: CharSequence?) { // To handle the failure. } })
Java
CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService(Context.COMPANION_DEVICE_SERVICE); Executor executor = new Executor() { @Override public void execute(Runnable runnable) { runnable.run(); } }; deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { executor, // Called when a device is found. Launch the IntentSender so the user can // select the device they want to pair with. @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult( chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0 ); } catch (IntentSender.SendIntentException e) { Log.e("MainActivity", "Failed to send intent"); } } @Override public void onAssociationCreated(AssociationInfo associationInfo) { // An association is created. } @Override public void onFailure(CharSequence errorMessage) { // To handle the failure. });
Na urządzeniach z Androidem 12L (poziom interfejsu API 32) lub starszym (wycofany):
Kotlin
val deviceManager = requireContext().getSystemService(Context.COMPANION_DEVICE_SERVICE) deviceManager.associate(pairingRequest, object : CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user // can select the device they want to pair with. override fun onDeviceFound(chooserLauncher: IntentSender) { startIntentSenderForResult(chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } override fun onFailure(error: CharSequence?) { // To handle the failure. } }, null)
Java
CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService(Context.COMPANION_DEVICE_SERVICE); deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user can // select the device they want to pair with. @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult( chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0 ); } catch (IntentSender.SendIntentException e) { Log.e("MainActivity", "Failed to send intent"); } } @Override public void onFailure(CharSequence error) { // To handle the failure. } }, null);
Wynik wyboru użytkownika jest wysyłany z powrotem do fragmentu w onActivityResult()
Twojej aktywności. Następnie możesz uzyskać dostęp do wybranego urządzenia.
Gdy użytkownik wybierze urządzenie Bluetooth, pojawi się BluetoothDevice
.
Gdy użytkownik wybierze urządzenie Bluetooth LE, pojawi się android.bluetooth.le.ScanResult
.
Gdy użytkownik wybierze urządzenie Wi-Fi, pojawi się android.net.wifi.ScanResult
.
Kotlin
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) { when (requestCode) { SELECT_DEVICE_REQUEST_CODE -> when(resultCode) { Activity.RESULT_OK -> { // The user chose to pair the app with a Bluetooth device. val deviceToPair: BluetoothDevice? = data?.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE) deviceToPair?.let { device -> device.createBond() // Continue to interact with the paired device. } } } else -> super.onActivityResult(requestCode, resultCode, data) } }
Java
@Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { if (resultCode != Activity.RESULT_OK) { return; } if (requestCode == SELECT_DEVICE_REQUEST_CODE && data != null) { BluetoothDevice deviceToPair = data.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE); if (deviceToPair != null) { deviceToPair.createBond(); // Continue to interact with the paired device. } } else { super.onActivityResult(requestCode, resultCode, data); } }
Pełny przykład:
Na urządzeniach z Androidem 13 (poziom API 33) lub nowszym:
Kotlin
private const val SELECT_DEVICE_REQUEST_CODE = 0 class MainActivity : AppCompatActivity() { private val deviceManager: CompanionDeviceManager by lazy { getSystemService(Context.COMPANION_DEVICE_SERVICE) as CompanionDeviceManager } val mBluetoothAdapter: BluetoothAdapter by lazy { val java = BluetoothManager::class.java getSystemService(java)!!.adapter } val executor: Executor = Executor { it.run() } override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // To skip filters based on names and supported feature flags (UUIDs), // omit calls to setNamePattern() and addServiceUuid() // respectively, as shown in the following Bluetooth example. val deviceFilter: BluetoothDeviceFilter = BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid(ParcelUuid(UUID(0x123abcL, -1L)), null) .build() // The argument provided in setSingleDevice() determines whether a single // device name or a list of them appears. val pairingRequest: AssociationRequest = AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build() // When the app tries to pair with a Bluetooth device, show the // corresponding dialog box to the user. deviceManager.associate(pairingRequest, executor, object : CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user // can select the device they want to pair with. override fun onAssociationPending(intentSender: IntentSender) { intentSender?.let { startIntentSenderForResult(it, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } } override fun onAssociationCreated(associationInfo: AssociationInfo) { // AssociationInfo object is created and get association id and the // macAddress. var associationId: int = associationInfo.id var macAddress: MacAddress = associationInfo.deviceMacAddress } override fun onFailure(errorMessage: CharSequence?) { // Handle the failure. } ) override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) { when (requestCode) { SELECT_DEVICE_REQUEST_CODE -> when(resultCode) { Activity.RESULT_OK -> { // The user chose to pair the app with a Bluetooth device. val deviceToPair: BluetoothDevice? = data?.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE) deviceToPair?.let { device -> device.createBond() // Maintain continuous interaction with a paired device. } } } else -> super.onActivityResult(requestCode, resultCode, data) } } }
Java
class MainActivityJava extends AppCompatActivity { private static final int SELECT_DEVICE_REQUEST_CODE = 0; Executor executor = new Executor() { @Override public void execute(Runnable runnable) { runnable.run(); } }; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService( Context.COMPANION_DEVICE_SERVICE ); // To skip filtering based on name and supported feature flags, // do not include calls to setNamePattern() and addServiceUuid(), // respectively. This example uses Bluetooth. BluetoothDeviceFilter deviceFilter = new BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid( new ParcelUuid(new UUID(0x123abcL, -1L)), null ) .build(); // The argument provided in setSingleDevice() determines whether a single // device name or a list of device names is presented to the user as // pairing options. AssociationRequest pairingRequest = new AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build(); // When the app tries to pair with the Bluetooth device, show the // appropriate pairing request dialog to the user. deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { executor, // Called when a device is found. Launch the IntentSender so the user can // select the device they want to pair with. @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult( chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0 ); } catch (IntentSender.SendIntentException e) { Log.e("MainActivity", "Failed to send intent"); } } @Override public void onAssociationCreated(AssociationInfo associationInfo) { // AssociationInfo object is created and get association id and the // macAddress. int associationId = associationInfo.getId(); MacAddress macAddress = associationInfo.getDeviceMacAddress(); } @Override public void onFailure(CharSequence errorMessage) { // Handle the failure. }); } @Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { if (resultCode != Activity.RESULT_OK) { return; } if (requestCode == SELECT_DEVICE_REQUEST_CODE) { if (resultCode == Activity.RESULT_OK && data != null) { BluetoothDevice deviceToPair = data.getParcelableExtra( CompanionDeviceManager.EXTRA_DEVICE ); if (deviceToPair != null) { deviceToPair.createBond(); // ... Continue interacting with the paired device. } } } else { super.onActivityResult(requestCode, resultCode, data); } } }
Na urządzeniach z Androidem 12L (poziom interfejsu API 32) lub starszym (wycofany):
Kotlin
private const val SELECT_DEVICE_REQUEST_CODE = 0 class MainActivity : AppCompatActivity() { private val deviceManager: CompanionDeviceManager by lazy { getSystemService(Context.COMPANION_DEVICE_SERVICE) as CompanionDeviceManager } override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // To skip filters based on names and supported feature flags (UUIDs), // omit calls to setNamePattern() and addServiceUuid() // respectively, as shown in the following Bluetooth example. val deviceFilter: BluetoothDeviceFilter = BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid(ParcelUuid(UUID(0x123abcL, -1L)), null) .build() // The argument provided in setSingleDevice() determines whether a single // device name or a list of them appears. val pairingRequest: AssociationRequest = AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build() // When the app tries to pair with a Bluetooth device, show the // corresponding dialog box to the user. deviceManager.associate(pairingRequest, object : CompanionDeviceManager.Callback() { override fun onDeviceFound(chooserLauncher: IntentSender) { startIntentSenderForResult(chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } override fun onFailure(error: CharSequence?) { // Handle the failure. } }, null) } override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) { when (requestCode) { SELECT_DEVICE_REQUEST_CODE -> when(resultCode) { Activity.RESULT_OK -> { // The user chose to pair the app with a Bluetooth device. val deviceToPair: BluetoothDevice? = data?.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE) deviceToPair?.let { device -> device.createBond() // Maintain continuous interaction with a paired device. } } } else -> super.onActivityResult(requestCode, resultCode, data) } } }
Java
class MainActivityJava extends AppCompatActivity { private static final int SELECT_DEVICE_REQUEST_CODE = 0; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService( Context.COMPANION_DEVICE_SERVICE ); // To skip filtering based on name and supported feature flags, // don't include calls to setNamePattern() and addServiceUuid(), // respectively. This example uses Bluetooth. BluetoothDeviceFilter deviceFilter = new BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid( new ParcelUuid(new UUID(0x123abcL, -1L)), null ) .build(); // The argument provided in setSingleDevice() determines whether a single // device name or a list of device names is presented to the user as // pairing options. AssociationRequest pairingRequest = new AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build(); // When the app tries to pair with the Bluetooth device, show the // appropriate pairing request dialog to the user. deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult(chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0); } catch (IntentSender.SendIntentException e) { // failed to send the intent } } @Override public void onFailure(CharSequence error) { // handle failure to find the companion device } }, null); } @Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { if (requestCode == SELECT_DEVICE_REQUEST_CODE) { if (resultCode == Activity.RESULT_OK && data != null) { BluetoothDevice deviceToPair = data.getParcelableExtra( CompanionDeviceManager.EXTRA_DEVICE ); if (deviceToPair != null) { deviceToPair.createBond(); // ... Continue interacting with the paired device. } } } else { super.onActivityResult(requestCode, resultCode, data); } } }
Profile urządzeń towarzyszących
W Androidzie 12 (poziom interfejsu API 31) i nowszych aplikacje towarzyszące, które zarządzają urządzeniami, takimi jak zegarki, mogą używać profili urządzeń towarzyszących, aby usprawnić proces konfiguracji poprzez przyznanie niezbędnych uprawnień podczas parowania. Więcej informacji znajdziesz w artykule Profile urządzeń towarzyszących.
Nie wyłączaj aplikacji towarzyszących
Od Androida 16 (poziom API 36)
Właściwości CompanionDeviceManager.startObservingDevicePresence(String)
i CompanionDeviceService.onDeviceAppeared()
zostały wycofane.
Do automatycznego zarządzania wiązaniem zaimplementowanego
CompanionDeviceService
należy użyćCompanionDeviceManager.startObservingDevicePresence (ObservingDevicePresenceRequest)
.- Stan powiązania
CompanionDeviceService
jest automatycznie zarządzany na podstawie stanu obecności powiązanego urządzenia towarzyszącego:- Usługa jest powiązana, gdy urządzenie towarzyszące znajduje się w zasięgu BLE lub jest połączone przez Bluetooth.
- Usługa staje się niewiążąca, gdy urządzenie towarzyszące znajdzie się poza zasięgiem BLE lub gdy jego połączenie Bluetooth zostanie zakończone.
- Stan powiązania
Aplikacja otrzyma wywołanie zwrotne na podstawie różnych wartości
DevicePresenceEvent
.Więcej informacji znajdziesz w sekcji
CompanionDeviceService.onDeviceEvent()
.