כדי לגשת ל-Gemini API ולמשפחת המודלים של Gemini ישירות מהאפליקציה, מומלץ להשתמש ב-Vertex AI ב-Firebase SDK ל-Android. ערכת ה-SDK הזו היא חלק מפלטפורמת Firebase הרחבה שעוזרת לכם ליצור ולהריץ אפליקציות בסביבה מלאה.
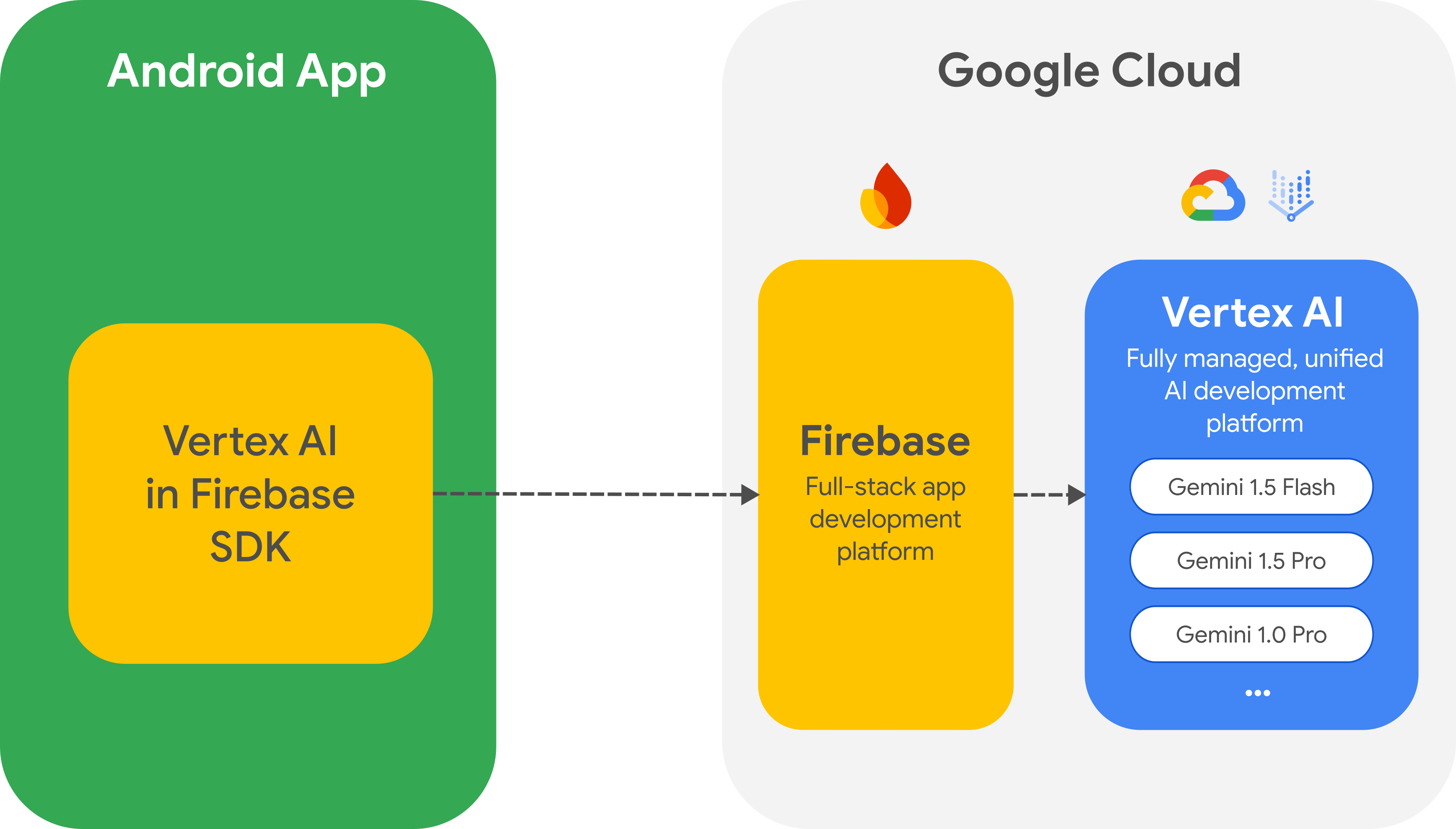
מעבר מ-Google AI Client SDK
Vertex AI ב-Firebase SDK דומה ל-Google AI client SDK, אבל ה-Vertex AI ב-Firebase SDK מציע אפשרויות אבטחה קריטיות ותכונות נוספות לתרחישים לדוגמה בסביבת הייצור. לדוגמה, כשמשתמשים ב-Vertex AI ב-Firebase, אפשר גם להשתמש באפשרויות הבאות:
Firebase App Check כדי להגן על Gemini API מפני ניצול לרעה על ידי לקוחות לא מורשים.
הגדרת תצורה מרחוק ב-Firebase כדי להגדיר ולשנות באופן דינמי ערכים לאפליקציה בענן (לדוגמה, שמות מודלים) בלי צורך לפרסם גרסת אפליקציה חדשה.
Cloud Storage for Firebase כדי לכלול בקשה ל-Gemini API עם קובצי מדיה גדולים.
אם כבר שילבתם את ה-SDK של לקוח Google AI באפליקציה, תוכלו להעביר אותה ל-Vertex AI ב-Firebase.
תחילת העבודה
לפני שתבצעו אינטראקציה עם Gemini API ישירות מהאפליקציה, תצטרכו לבצע כמה פעולות, כולל היכרות עם ההנחיות והגדרת Firebase והאפליקציה לשימוש ב-SDK.
התנסות בהנחיות
אפשר להתנסות בהנחיות ב-Vertex AI Studio. Vertex AI Studio היא סביבת פיתוח משולבת (IDE) ליצירת פרומפטים וליצירת אב טיפוס. אפשר להעלות קבצים כדי לבדוק הנחיות עם טקסט ותמונות, ולשמור הנחיה כדי לחזור אליה מאוחר יותר.
יצירת ההנחיה המתאימה לתרחיש לדוגמה היא יותר אמנות מאשר מדע, ולכן חשוב מאוד לבצע ניסויים. מידע נוסף על הנחיות זמין במסמכי העזרה של Firebase.
הגדרת פרויקט Firebase וקישור האפליקציה ל-Firebase
כשתהיו מוכנים לבצע קריאה ל-Gemini API מהאפליקציה, תוכלו לפעול לפי ההוראות במדריך לתחילת העבודה עם Vertex AI ב-Firebase כדי להגדיר את Firebase ואת ה-SDK באפליקציה. המדריך הזה יעזור לכם לבצע את כל המשימות הבאות במדריך הזה.
מגדירים פרויקט Firebase חדש או קיים, כולל שימוש בתוכנית התמחור Blaze שמבוססת על תשלום לפי שימוש והפעלה של ממשקי ה-API הנדרשים.
מחברים את האפליקציה ל-Firebase, כולל רישום האפליקציה והוספת קובץ התצורה של Firebase (
google-services.json
) לאפליקציה.
מוסיפים את יחסי התלות ב-Gradle
מוסיפים את יחסי התלות הבאים של Gradle למודול האפליקציה:
Kotlin
dependencies { ... implementation("com.google.firebase:firebase-vertexai:16.0.2") }
Java
dependencies { [...] implementation("com.google.firebase:firebase-vertexai:16.0.2") // Required to use `ListenableFuture` from Guava Android for one-shot generation implementation("com.google.guava:guava:31.0.1-android") // Required to use `Publisher` from Reactive Streams for streaming operations implementation("org.reactivestreams:reactive-streams:1.0.4") }
איך מפעילים את שירות Vertex AI ואת המודל הגנרטיבי
מתחילים ביצירת מופע של GenerativeModel
וציון שם הדגם:
Kotlin
val generativeModel = Firebase.vertexAI.generativeModel("gemini-1.5-flash")
Java
GenerativeModel gm = FirebaseVertexAI.getInstance().generativeModel("gemini-1.5-flash");
במסמכי התיעוד של Firebase תוכלו לקרוא מידע נוסף על המודלים הזמינים לשימוש עם Vertex AI ב-Firebase. תוכלו גם לקרוא על הגדרת פרמטרים של מודל.
אינטראקציה עם Gemini API מהאפליקציה
אחרי שמגדירים את Firebase ואת האפליקציה לשימוש ב-SDK, אפשר לבצע אינטראקציה עם Gemini API מהאפליקציה.
יצירת טקסט
כדי ליצור תשובת טקסט, קוראים ל-generateContent()
עם ההנחיה.
Kotlin
// Note: `generateContent()` is a `suspend` function, which integrates well // with existing Kotlin code. scope.launch { val response = model.generateContent("Write a story about the green robot") }
Java
// In Java, create a `GenerativeModelFutures` from the `GenerativeModel`. // Note that `generateContent()` returns a `ListenableFuture`. Learn more: // https://developer.android.com/develop/background-work/background-tasks/asynchronous/listenablefuture GenerativeModelFutures model = GenerativeModelFutures.from(gm); Content prompt = new Content.Builder() .addText("Write a story about a green robot.") .build(); ListenableFuture<GenerateContentResponse> response = model.generateContent(prompt); Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() { @Override public void onSuccess(GenerateContentResponse result) { String resultText = result.getText(); } @Override public void onFailure(Throwable t) { t.printStackTrace(); } }, executor);
יצירת טקסט מתמונות וממדיה אחרת
אפשר גם ליצור טקסט מהנחיה שכוללת טקסט ותמונות או מדיה אחרת. כשקוראים ל-generateContent()
, אפשר להעביר את המדיה כנתונים מוטמעים (כמו בדוגמה שבהמשך). לחלופין, אפשר לכלול בקשה בקובצי מדיה גדולים באמצעות כתובות URL של Cloud Storage ל-Firebase.
Kotlin
scope.launch { val response = model.generateContent( content { image(bitmap) text("what is the object in the picture?") } ) }
Java
GenerativeModelFutures model = GenerativeModelFutures.from(gm); Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.sparky); Content prompt = new Content.Builder() .addImage(bitmap) .addText("What developer tool is this mascot from?") .build(); ListenableFuture<GenerateContentResponse> response = model.generateContent(prompt); Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() { @Override public void onSuccess(GenerateContentResponse result) { String resultText = result.getText(); } @Override public void onFailure(Throwable t) { t.printStackTrace(); } }, executor);
שיחה עם זיכרון
אפשר גם לתמוך בשיחות עם כמה תורנים. מפעילים את השיחה באמצעות הפונקציה startChat()
. אפשר גם לספק היסטוריית הודעות. לאחר מכן צריך להפעיל את הפונקציה sendMessage()
כדי לשלוח הודעות בצ'אט.
Kotlin
val chat = generativeModel.startChat( history = listOf( content(role = "user") { text("Hello, I have 2 dogs in my house.") }, content(role = "model") { text("Great to meet you. What would you like to know?") } ) ) scope.launch { val response = chat.sendMessage("How many paws are in my house?") }
Java
// (Optional) create message history Content.Builder userContentBuilder = new Content.Builder(); userContentBuilder.setRole("user"); userContentBuilder.addText("Hello, I have 2 dogs in my house."); Content userContent = userContentBuilder.build(); Content.Builder modelContentBuilder = new Content.Builder(); modelContentBuilder.setRole("model"); modelContentBuilder.addText("Great to meet you. What would you like to know?"); Content modelContent = userContentBuilder.build(); List<Content> history = Arrays.asList(userContent, modelContent); // Initialize the chat ChatFutures chat = model.startChat(history); // Create a new user message Content.Builder messageBuilder = new Content.Builder(); messageBuilder.setRole("user"); messageBuilder.addText("How many paws are in my house?"); Content message = messageBuilder.build(); Publisher<GenerateContentResponse> streamingResponse = chat.sendMessageStream(message); StringBuilder outputContent = new StringBuilder(); streamingResponse.subscribe(new Subscriber<GenerateContentResponse>() { @Override public void onNext(GenerateContentResponse generateContentResponse) { String chunk = generateContentResponse.getText(); outputContent.append(chunk); } @Override public void onComplete() { // ... } @Override public void onError(Throwable t) { t.printStackTrace(); } @Override public void onSubscribe(Subscription s) { s.request(Long.MAX_VALUE); } });
שידור התשובה
כדי לקבל אינטראקציות מהירות יותר, אפשר לא להמתין לתוצאה המלאה של יצירת המודל, אלא להשתמש בסטרימינג כדי לטפל בתוצאות חלקיות. משתמשים ב-generateContentStream()
כדי לשדר תשובה.
Kotlin
scope.launch { var outputContent = "" generativeModel.generateContentStream(inputContent) .collect { response -> outputContent += response.text } }
Java
// Note that in Java the method `generateContentStream()` returns a // Publisher from the Reactive Streams library. // https://www.reactive-streams.org/ GenerativeModelFutures model = GenerativeModelFutures.from(gm); // Provide a prompt that contains text Content prompt = new Content.Builder() .addText("Write a story about a green robot.") .build(); Publisher<GenerateContentResponse> streamingResponse = model.generateContentStream(prompt); StringBuilder outputContent = new StringBuilder(); streamingResponse.subscribe(new Subscriber<GenerateContentResponse>() { @Override public void onNext(GenerateContentResponse generateContentResponse) { String chunk = generateContentResponse.getText(); outputContent.append(chunk); } @Override public void onComplete() { // ... } @Override public void onError(Throwable t) { t.printStackTrace(); } @Override public void onSubscribe(Subscription s) { s.request(Long.MAX_VALUE); } });
השלבים הבאים
- אפליקציה לדוגמה של Vertex AI ב-Firebase ב-GitHub.
- כדאי להתחיל לחשוב על הכנה לקראת ייצור, כולל הגדרת Firebase App Check כדי להגן על Gemini API מפני ניצול לרעה על ידי לקוחות לא מורשים.
- מידע נוסף על Vertex AI ב-Firebase זמין במסמכי העזרה של Firebase.