几乎所有多设备体验都是从查找可用设备开始的。为了简化这项常见任务,我们提供了 Device Discovery API。
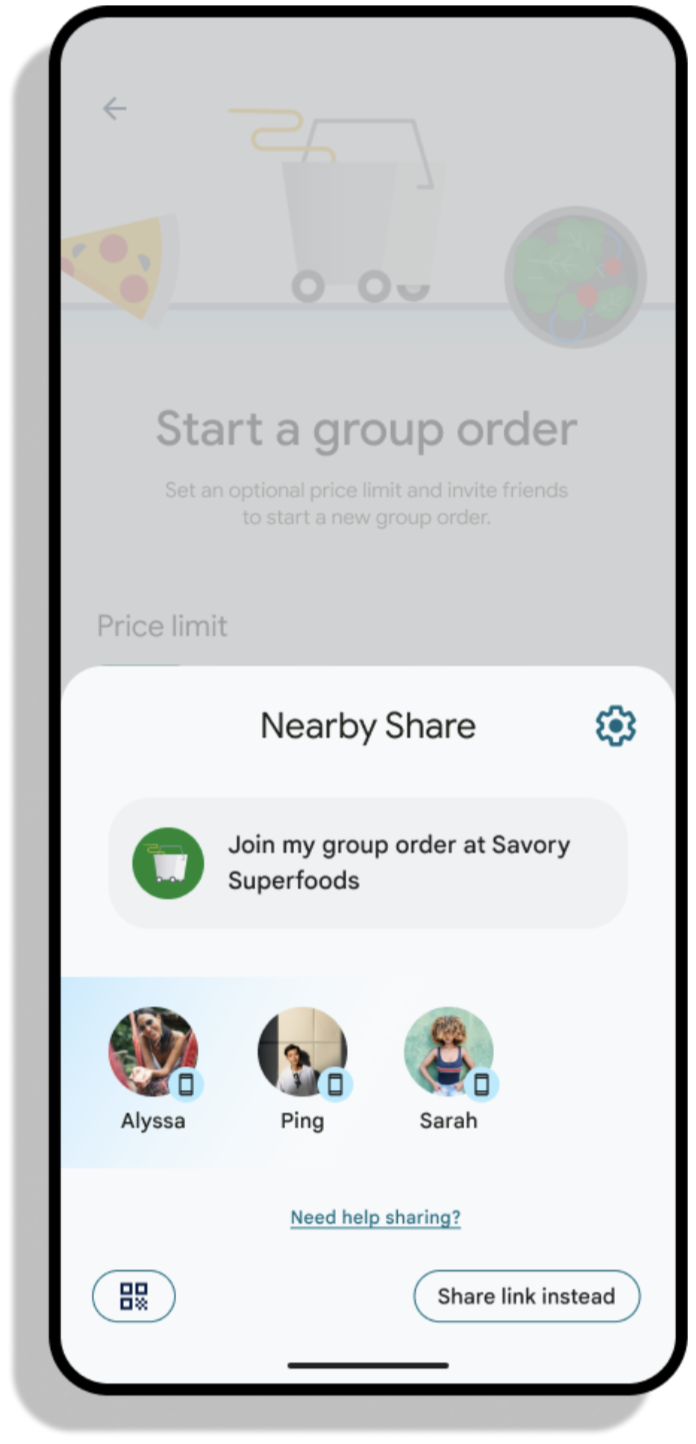
启动设备选择对话框
设备发现使用系统对话框来让用户选择目标设备。如需启动设备选择对话框,您首先需要获取设备发现客户端并注册结果接收器。请注意,与 registerForActivityResult
类似,此接收器必须无条件地注册为 activity 或 fragment 初始化路径的一部分。
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) devicePickerLauncher = Discovery.create(this).registerForResult(this, handleDevices) }
Java
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); devicePickerLauncher = Discovery.create(this).registerForResult(this, handleDevices); }
在上面的代码段中,我们有一个未定义的 handleDevices
对象。在用户选择要连接的设备后,以及 SDK 成功连接到其他设备后,此回调会收到所选 Participants
的列表。
Kotlin
handleDevices = OnDevicePickerResultListener { participants -> participants.forEach { // Use participant info } }
Java
handleDevices = participants -> { for (Participant participant : participants) { // Use participant info } }
注册设备选择器后,使用 devicePickerLauncher
实例启动它。DevicePickerLauncher.launchDevicePicker
接受两个参数:设备过滤器列表(见下文)和 startComponentRequest
。startComponentRequest
用于指示应在接收设备上启动哪个 activity,以及向用户显示请求的原因。
Kotlin
devicePickerLauncher.launchDevicePicker( listOf(), startComponentRequest { action = "com.example.crossdevice.MAIN" reason = "I want to say hello to you" }, )
Java
devicePickerLauncher.launchDevicePickerFuture( Collections.emptyList(), new StartComponentRequest.Builder() .setAction("com.example.crossdevice.MAIN") .setReason("I want to say hello to you") .build());
接受连接请求
当用户在设备选择器中选择设备时,接收设备上会显示一个对话框,要求用户接受连接。接受后,系统会启动目标 activity,该 activity 可在 onCreate
和 onNewIntent
中进行处理。
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) handleIntent(getIntent()) } override fun onNewIntent(intent: Intent) { super.onNewIntent(intent) handleIntent(intent) } private fun handleIntent(intent: Intent) { val participant = Discovery.create(this).getParticipantFromIntent(intent) // Accept connection from participant (see below) }
Java
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); handleIntent(getIntent()); } @Override public void onNewIntent(Intent intent) { super.onNewIntent(intent); handleIntent(intent); } private void handleIntent(Intent intent) { Participant participant = Discovery.create(this).getParticipantFromIntent(intent); // Accept connection from participant (see below) }
设备过滤条件
发现设备时,通常希望对这些设备进行过滤,以仅显示与当前用例相关的设备。例如:
- 仅显示带摄像头的设备,以便扫描二维码
- 仅显示电视,提供大屏幕观看体验
对于此开发者预览版,我们首先能够过滤属于同一用户的设备。
您可以使用 DeviceFilter
类指定设备过滤器:
Kotlin
val deviceFilters = listOf(DeviceFilter.trustRelationshipFilter(MY_DEVICES_ONLY))
Java
List<DeviceFilter> deviceFilters = Arrays.asList(DeviceFilter.trustRelationshipFilter(MY_DEVICES_ONLY));
定义设备过滤条件后,您可以启动设备发现。
Kotlin
devicePickerLauncher.launchDevicePicker(deviceFilters, startComponentRequest)
Java
Futures.addCallback( devicePickerLauncher.launchDevicePickerFuture(deviceFilters, startComponentRequest), new FutureCallback<Void>() { @Override public void onSuccess(Void result) { // do nothing, result will be returned to handleDevices callback } @Override public void onFailure(Throwable t) { // handle error } }, mainExecutor);
请注意,launchDevicePicker
是一个使用 suspend
关键字的异步函数。