应用的功能块应能在各种尺寸的 Wear OS 设备上正常运行,充分利用额外的空间(如果有),并且仍能在小屏幕上获得出色的显示效果。本指南提供了实现此用户体验的建议。
如需详细了解自适应布局的设计原则,请参阅设计指南。
使用 ProtoLayout 构建响应式布局
ProtoLayout Material 库提供了预定义的布局来帮助您构建功能块。这些布局已设计为适应屏幕尺寸。
请参阅 Figma 设计布局,其中介绍了可用的规范布局以及如何使用这些规范构建设计:
对于大多数用例,我们建议使用 PrimaryLayout
或 EdgeContentLayout
作为顶级布局。使用 setResponsiveContentInsetEnabled
设置响应式行为,例如:
PrimaryLayout.Builder(deviceParameters)
.setResponsiveContentInsetEnabled(true)
.setContent(
// ...
)
.build()
通过断点提供差异化的体验
ProtoLayout Material 库中的布局已经是自适应的,并会确保元素放置和可见性正确无误。不过,在某些情况下,您可能需要更改可见元素的数量以获得最佳结果。例如,您可能希望在较小的显示屏上放置 3 个按钮,在较大的显示屏上放置 5 个按钮。
如需实现这种差异化体验,请使用屏幕尺寸断点。如需在屏幕尺寸超过 225 dp 时显示其他布局,请执行以下操作:
PrimaryLayout.Builder(deviceParameters)
.setResponsiveContentInsetEnabled(true)
.setContent(
MultiButtonLayout.Builder()
.addButtonContent(button1)
.addButtonContent(button2)
.addButtonContent(button3)
.apply {
if (deviceParameters.screenHeightDp >= 225) {
addButtonContent(button4)
addButtonContent(button5)
}
}
.build()
)
.setPrimaryLabelTextContent(label)
.setPrimaryChipContent(compactChip)
.build()
设计指南介绍了其他机会。
使用预览功能在不同尺寸的屏幕上测试功能块
请务必在不同屏幕尺寸上测试布局。使用功能块预览注解以及 TilePreviewHelper
和 TilePreviewData
类:
@Preview(device = WearDevices.LARGE_ROUND)
fun smallPreview(context: Context) = TilePreviewData(
onTileRequest = { request ->
TilePreviewHelper.singleTimelineEntryTileBuilder(
buildLayout()
).build()
}
)
这样,您就可以直接在 Android Studio 中预览功能块布局。上文中的断点示例展示了在预览时,如何在屏幕空间允许的情况下显示其他按钮:
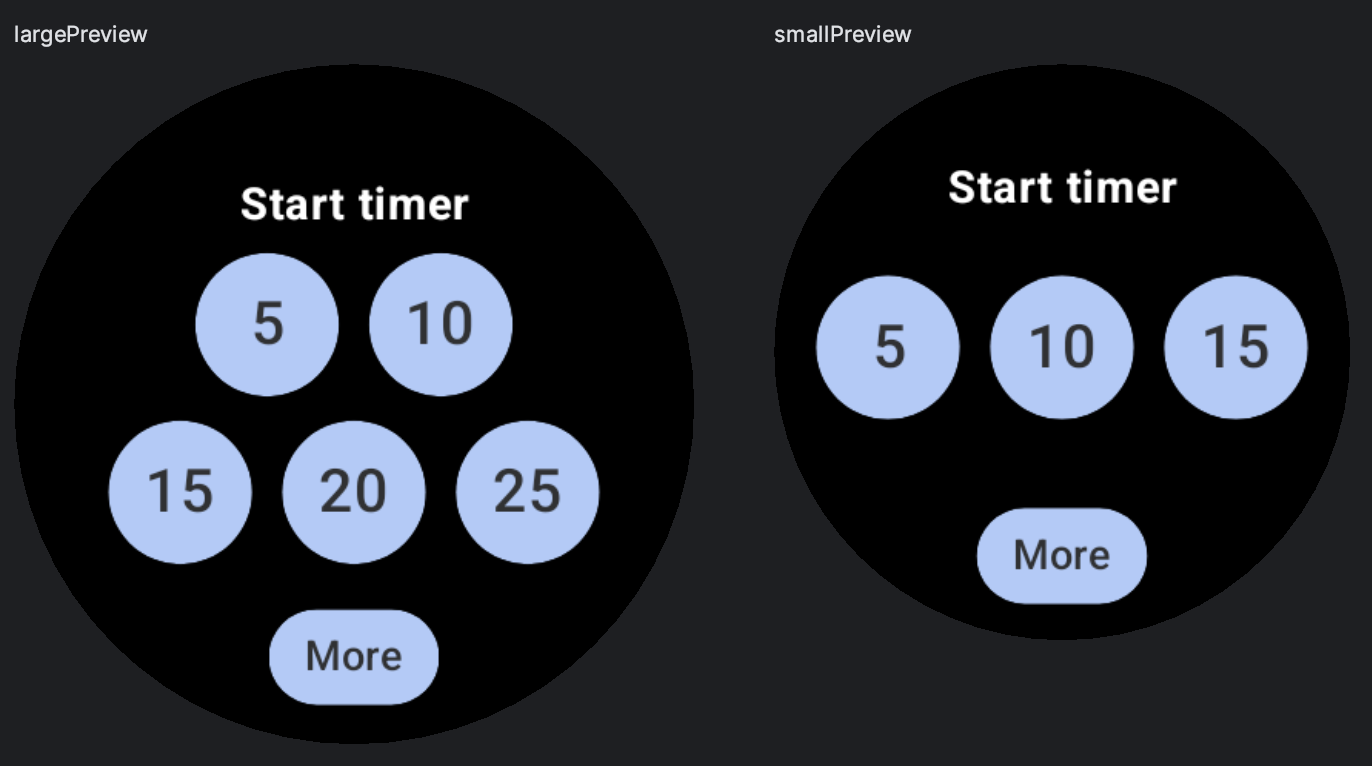
显示断点效果的小屏幕和大屏幕
如需查看完整示例,请参阅 GitHub 上的媒体图块示例。