توفّر CameraX واجهة برمجة تطبيقات الإضافات للوصول إلى الإضافات التي نفذتها الشركات المصنّعة للأجهزة على أجهزة Android المختلفة. للحصول على قائمة بأوضاع الإضافات المتوافقة، يُرجى الاطّلاع على إضافات الكاميرا.
للحصول على قائمة بالأجهزة المتوافقة، يُرجى الاطّلاع على الأجهزة المتوافقة.
بنية الإضافات
توضح الصورة التالية بنية إضافات الكاميرا.
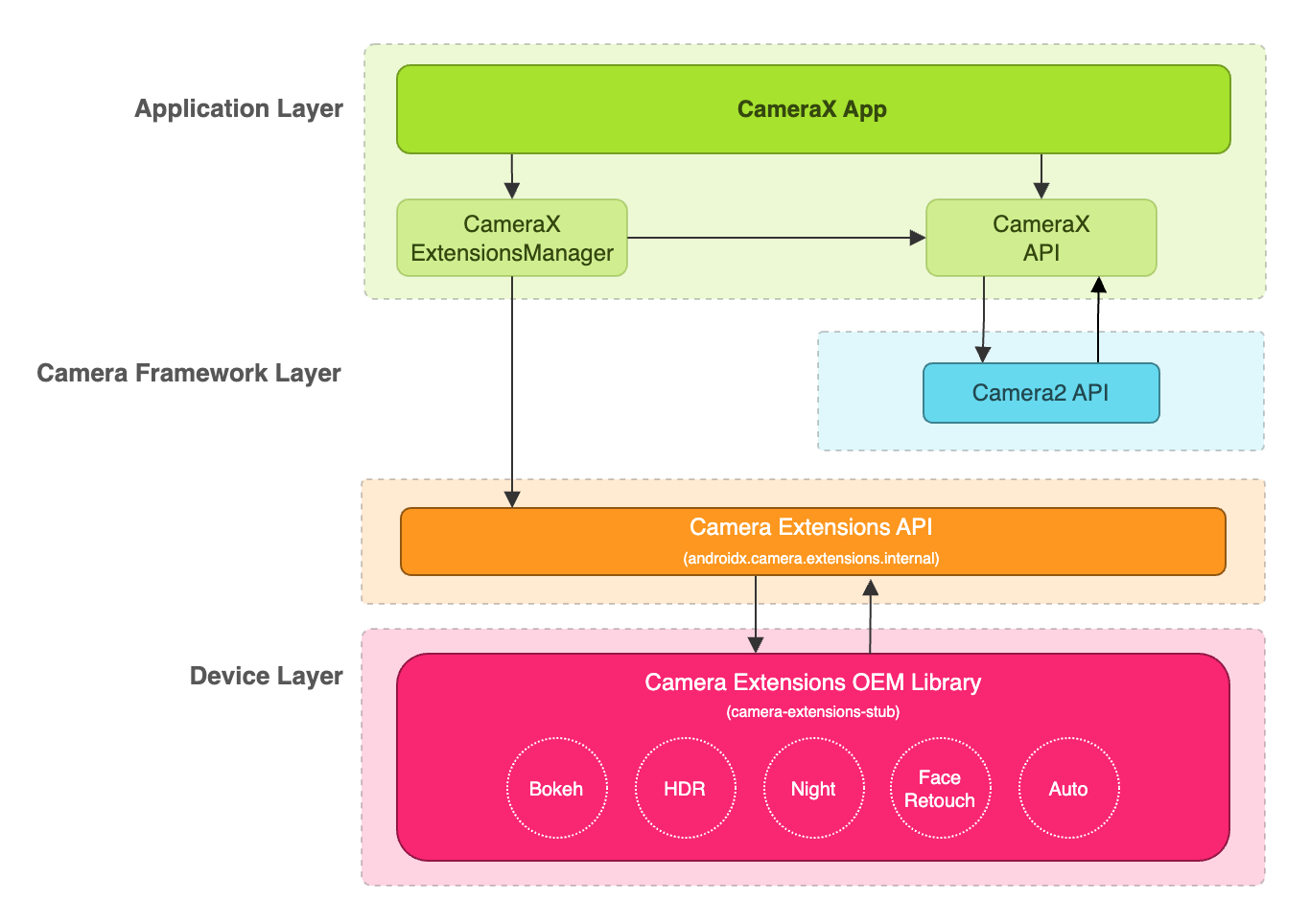
يمكن لتطبيق CameraX استخدام الإضافات من خلال واجهة برمجة تطبيقات إضافات CameraX. تشير رسالة الأشكال البيانية تدير واجهة برمجة تطبيقات إضافات للكاميرا طلبات البحث عن الإضافات المتاحة، وتهيئ جلسة كاميرا إضافية، والتواصل مع المصنّع الأصلي للجهاز لإضافات الكاميرا المكتبة. يتيح ذلك لتطبيقك استخدام إمكانيات مثل الوضع الليلي" و"النطاق العالي الديناميكية" و"تلقائي" خلفية ضبابية أو ميزة "تجميل الوجه"
تفعيل إضافة لالتقاط الصور ومعاينتها
قبل استخدام واجهة برمجة تطبيقات الإضافات، عليك استرداد مثيل ExtensionsManager
باستخدام ExtensionsManager#getInstanceAsync(Context, CameraProvider)
. سيتيح لك ذلك الاستعلام عن مدى توفّر الإضافة.
المعلومات. بعد ذلك، يمكنك استرداد إضافة مفعَّلة CameraSelector
. تشير رسالة الأشكال البيانية
على تطبيق "وضع الإضافة" على التقاط الصور ومعاينة حالات الاستخدام
عن طريق استدعاء bindToLifecycle()
مع تفعيل إضافة CameraSelector
.
لتنفيذ الإضافة في حالات الاستخدام المتعلّقة بالتقاط الصور ومعاينة الصورة، راجع نموذج التعليمة البرمجية التالي:
Kotlin
import androidx.camera.extensions.ExtensionMode import androidx.camera.extensions.ExtensionsManager override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) val lifecycleOwner = this val cameraProviderFuture = ProcessCameraProvider.getInstance(applicationContext) cameraProviderFuture.addListener({ // Obtain an instance of a process camera provider // The camera provider provides access to the set of cameras associated with the device. // The camera obtained from the provider will be bound to the activity lifecycle. val cameraProvider = cameraProviderFuture.get() val extensionsManagerFuture = ExtensionsManager.getInstanceAsync(applicationContext, cameraProvider) extensionsManagerFuture.addListener({ // Obtain an instance of the extensions manager // The extensions manager enables a camera to use extension capabilities available on // the device. val extensionsManager = extensionsManagerFuture.get() // Select the camera val cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA // Query if extension is available. // Not all devices will support extensions or might only support a subset of // extensions. if (extensionsManager.isExtensionAvailable(cameraSelector, ExtensionMode.NIGHT)) { // Unbind all use cases before enabling different extension modes. try { cameraProvider.unbindAll() // Retrieve a night extension enabled camera selector val nightCameraSelector = extensionsManager.getExtensionEnabledCameraSelector( cameraSelector, ExtensionMode.NIGHT ) // Bind image capture and preview use cases with the extension enabled camera // selector. val imageCapture = ImageCapture.Builder().build() val preview = Preview.Builder().build() // Connect the preview to receive the surface the camera outputs the frames // to. This will allow displaying the camera frames in either a TextureView // or SurfaceView. The SurfaceProvider can be obtained from the PreviewView. preview.setSurfaceProvider(surfaceProvider) // Returns an instance of the camera bound to the lifecycle // Use this camera object to control various operations with the camera // Example: flash, zoom, focus metering etc. val camera = cameraProvider.bindToLifecycle( lifecycleOwner, nightCameraSelector, imageCapture, preview ) } catch (e: Exception) { Log.e(TAG, "Use case binding failed", e) } } }, ContextCompat.getMainExecutor(this)) }, ContextCompat.getMainExecutor(this)) }
Java
import androidx.camera.extensions.ExtensionMode; import androidx.camera.extensions.ExtensionsManager; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); final LifecycleOwner lifecycleOwner = this; final ListenableFuturecameraProviderFuture = ProcessCameraProvider.getInstance(getApplicationContext()); cameraProviderFuture.addListener(() -> { try { // Obtain an instance of a process camera provider // The camera provider provides access to the set of cameras associated with the // device. The camera obtained from the provider will be bound to the activity // lifecycle. final ProcessCameraProvider cameraProvider = cameraProviderFuture.get(); final ListenableFuture extensionsManagerFuture = ExtensionsManager.getInstanceAsync(getApplicationContext(), cameraProvider); extensionsManagerFuture.addListener(() -> { // Obtain an instance of the extensions manager // The extensions manager enables a camera to use extension capabilities available // on the device. try { final ExtensionsManager extensionsManager = extensionsManagerFuture.get(); // Select the camera final CameraSelector cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA; // Query if extension is available. // Not all devices will support extensions or might only support a subset of // extensions. if (extensionsManager.isExtensionAvailable( cameraSelector, ExtensionMode.NIGHT )) { // Unbind all use cases before enabling different extension modes. cameraProvider.unbindAll(); // Retrieve extension enabled camera selector final CameraSelector nightCameraSelector = extensionsManager .getExtensionEnabledCameraSelector(cameraSelector, ExtensionMode.NIGHT); // Bind image capture and preview use cases with the extension enabled camera // selector. final ImageCapture imageCapture = new ImageCapture.Builder().build(); final Preview preview = new Preview.Builder().build(); // Connect the preview to receive the surface the camera outputs the frames // to. This will allow displaying the camera frames in either a TextureView // or SurfaceView. The SurfaceProvider can be obtained from the PreviewView. preview.setSurfaceProvider(surfaceProvider); cameraProvider.bindToLifecycle( lifecycleOwner, nightCameraSelector, imageCapture, preview ); } } catch (ExecutionException | InterruptedException e) { throw new RuntimeException(e); } }, ContextCompat.getMainExecutor(this)); } catch (ExecutionException | InterruptedException e) { throw new RuntimeException(e); } }, ContextCompat.getMainExecutor(this)); }
إيقاف الإضافة
لإيقاف إضافات المورِّدين، يجب إلغاء ربط جميع حالات الاستخدام وإعادة ربط التقاط الصورة.
ومعاينة حالات الاستخدام من خلال أداة اختيار عادية للكاميرا على سبيل المثال، أعد الربط
الكاميرا الخلفية باستخدام "CameraSelector.DEFAULT_BACK_CAMERA
".
التبعيات
تم تنفيذ واجهة برمجة تطبيقات إضافات CameraX في مكتبة camera-extensions
.
تعتمد الإضافات على وحدات CameraX الأساسية (core
وcamera2
lifecycle
).
Groovy
dependencies { def camerax_version = "1.2.0-rc01" implementation "androidx.camera:camera-core:${camerax_version}" implementation "androidx.camera:camera-camera2:${camerax_version}" implementation "androidx.camera:camera-lifecycle:${camerax_version}" //the CameraX Extensions library implementation "androidx.camera:camera-extensions:${camerax_version}" ... }
Kotlin
dependencies { val camerax_version = "1.2.0-rc01" implementation("androidx.camera:camera-core:${camerax_version}") implementation("androidx.camera:camera-camera2:${camerax_version}") implementation("androidx.camera:camera-lifecycle:${camerax_version}") // the CameraX Extensions library implementation("androidx.camera:camera-extensions:${camerax_version}") ... }
إزالة واجهة برمجة التطبيقات القديمة
مع واجهة برمجة التطبيقات الإضافات الجديدة التي تم إصدارها في 1.0.0-alpha26
، الإصدار القديم
واجهة برمجة التطبيقات الإضافات التي تم إصدارها في آب (أغسطس) 2019 متوقفة الآن. البدء بـ
الإصدار 1.0.0-alpha28
، تمت إزالة واجهة برمجة تطبيقات الإضافات القديمة من
المكتبة. يجب أن تحصل التطبيقات التي تستخدم واجهة برمجة التطبيقات الإضافات الجديدة الآن على
الإضافة التي تم تفعيل الإضافة فيها CameraSelector
واستخدامه لربط حالات الاستخدام.
يجب نقل بيانات التطبيقات التي تستخدم واجهة برمجة تطبيقات الإضافات القديمة إلى الواجهة الجديدة واجهة برمجة التطبيقات الإضافية لضمان التوافق مع الميزات القادمة في CameraX والإصدارات.
مصادر إضافية
للتعرف على مزيد من المعلومات حول CameraX، يمكنك الرجوع إلى الموارد الإضافية التالية.
درس تطبيقي حول الترميز
نموذج التعليمات البرمجية
مراجع أخرى
أداة التحقّق من إضافات مورّدي CameraX