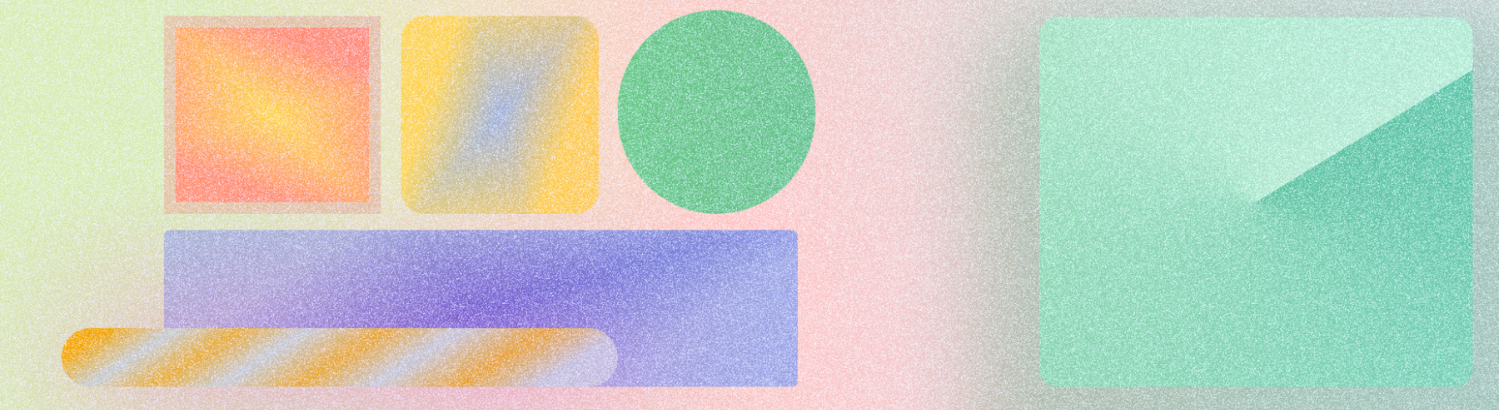
虽然您的应用可以只包含文本和颜色,但您可能需要添加更多视觉元素,例如徽标或插图。在向应用添加图形以及各种库来创建图形效果或添加动画方面,Android 有特殊的最佳实践。
Android 资源称为可绘制对象,即一种在屏幕上绘制的资源。包括但不限于位图、形状和矢量。
制作图片和图形时,请注意以下几点:
- 避免在素材资源中添加不可更改的文字。
- 尽可能先使用矢量格式。
- 为分辨率范围提供资源。
- 在背景图片和文字之间提供足够的纱罩。
- 虽然 Android 能够实现不同的图片效果(如渐变、着色和模糊处理),但有些效果开销更大。
- 动画矢量可绘制对象为小型界面动画提供可缩放的格式。
如何导出适用于 Android 的资源
- 请将素材资源名称的格式设置为小写。
- 设置要导出为 SVG 的简单素材资源。
- 将照片等复杂图片设置为以 WebP、PNG、JPG 格式导出。
- 如下表所示,设置正确的分辨率缩放比例。
屏幕尺寸 | 规模 |
---|---|
mdpi |
1 倍 |
hdpi |
1.5 倍 |
xhdpi |
2 倍 |
xxhdpi |
3 倍 |
xxxhdpi |
4 倍 |
您可以选择使用 Android Studio 将 SVG 转换为矢量可绘制对象 (VD)。将资源整理到与分辨率相对应的目录中,以便移交,如下图所示。例如,在文件夹名称中包含屏幕尺寸。
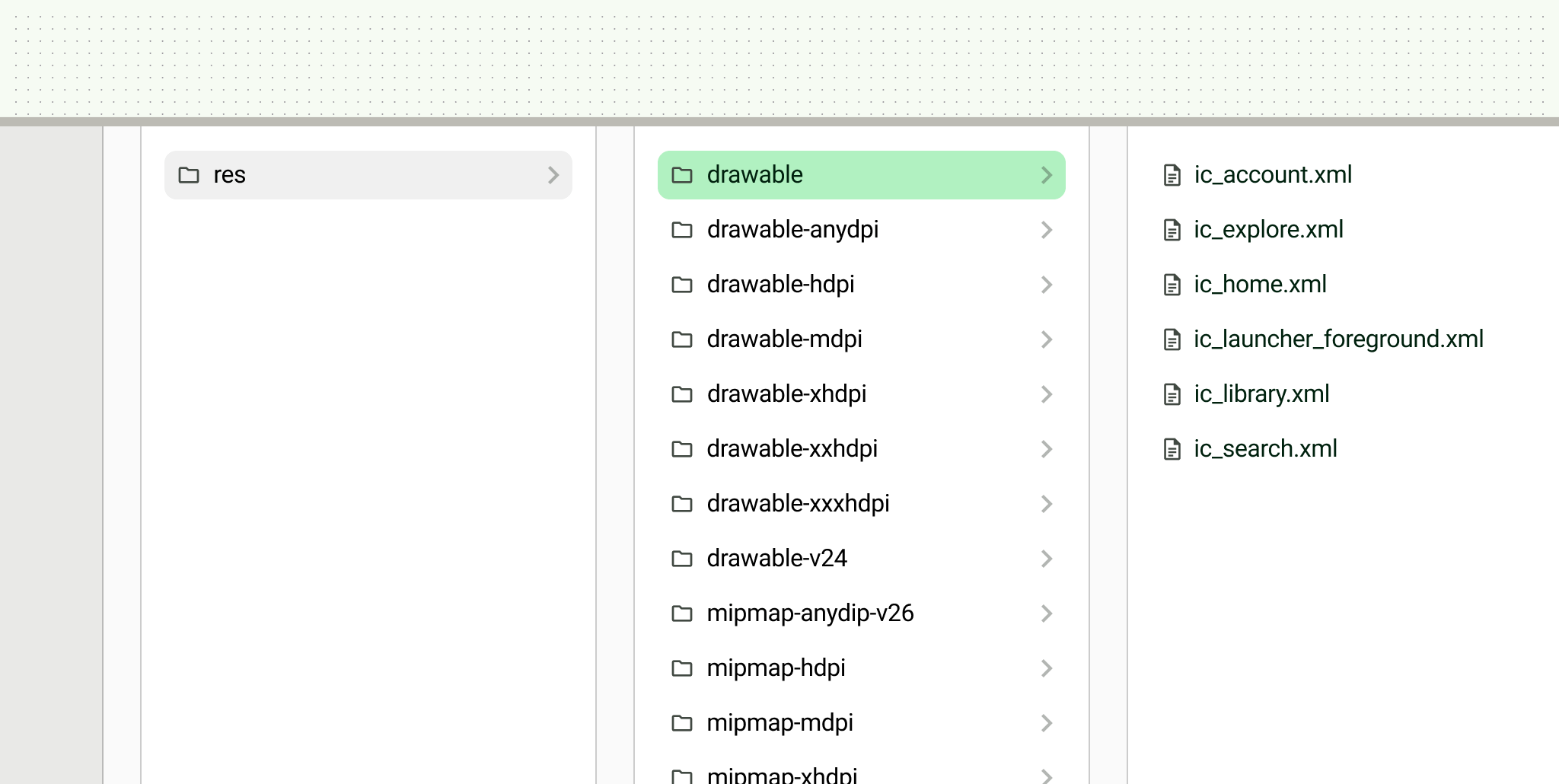
资产类型
Android 支持以下资源类型。
矢量
矢量图形是一种无损格式,这意味着它在缩放时不会丢失视觉信息。矢量由数学点组成,为了创建图像而填充这些数学点。
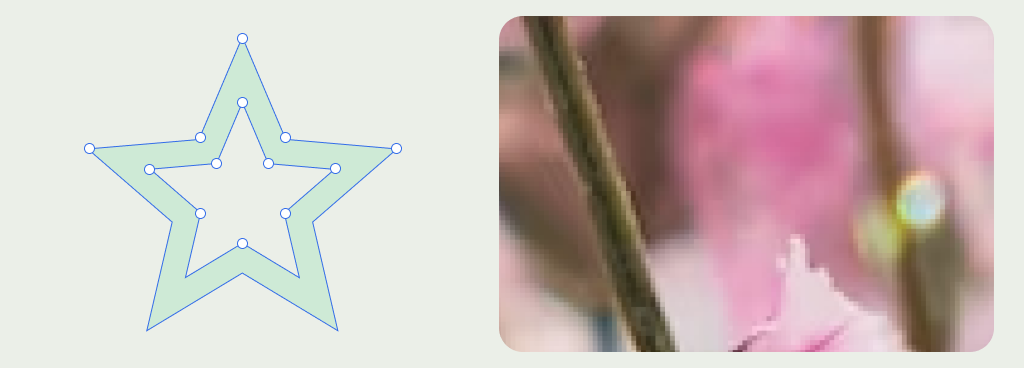
矢量格式
Android 支持以下矢量图像格式:SVG 和矢量可绘制对象。
矢量可绘制对象看起来类似于 SVG,但基于 XML。它们还支持各种属性,例如渐变。如需详细了解受支持的功能,请参阅 VectorDrawable
。您可以通过 Vector Asset Studio 将 SVG 转换为矢量可绘制对象。
用例
由于它们的尺寸较小,因此最好使用矢量格式创建图标。动画矢量可绘制对象可用于为图标添加动画效果。
- 插图是一种图形,有助于引导用户、解释概念或表达想法。它们通常展现品牌风格。
- 主打插图是突出显示其他内容的重点,用于设定整体外观和风格以及解释主要信息。
- 小程序图示较小,通常是内嵌的,并且支持周围的内容。
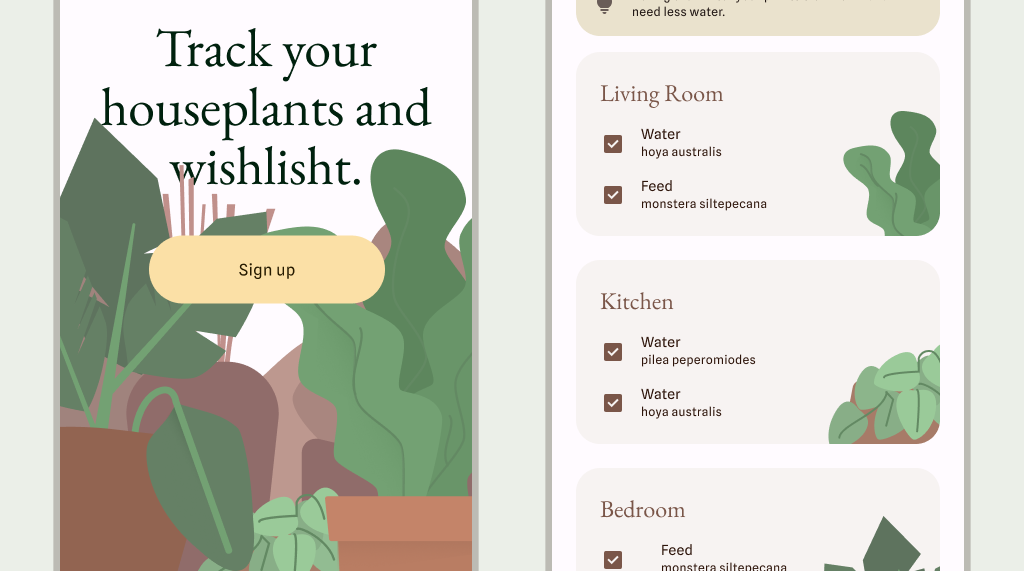
光栅
有损图形是指在通过压缩或缩放操作时丢失细节的图形,它由组成图像的像素组成。光栅图形通常用于照片等细节图像或复杂渐变。由于它们在缩放时会丢失细节,因此请导出这些图片的多种分辨率。
光栅格式
Android 支持以下光栅图片格式:PNG、GIF、JPG、WebP。
用例
用例包括具有一系列纹理且会产生宽色调色板和渐变效果的图片,或具有一组过于复杂的贝塞尔点的图片。使用场景可能还包括非常详细的照片素材资源,例如商品照片、营业地点详细信息等。
大小
制作素材资源时,请注意以下事项。
分辨率范围
您的应用应根据屏幕密度范围或分桶提供位图图形。操作系统会使用这些存储分区自动向相关设备显示正确的图形。通过为每个存储分区提供资源,确保每种设备上都显示高保真度图形。
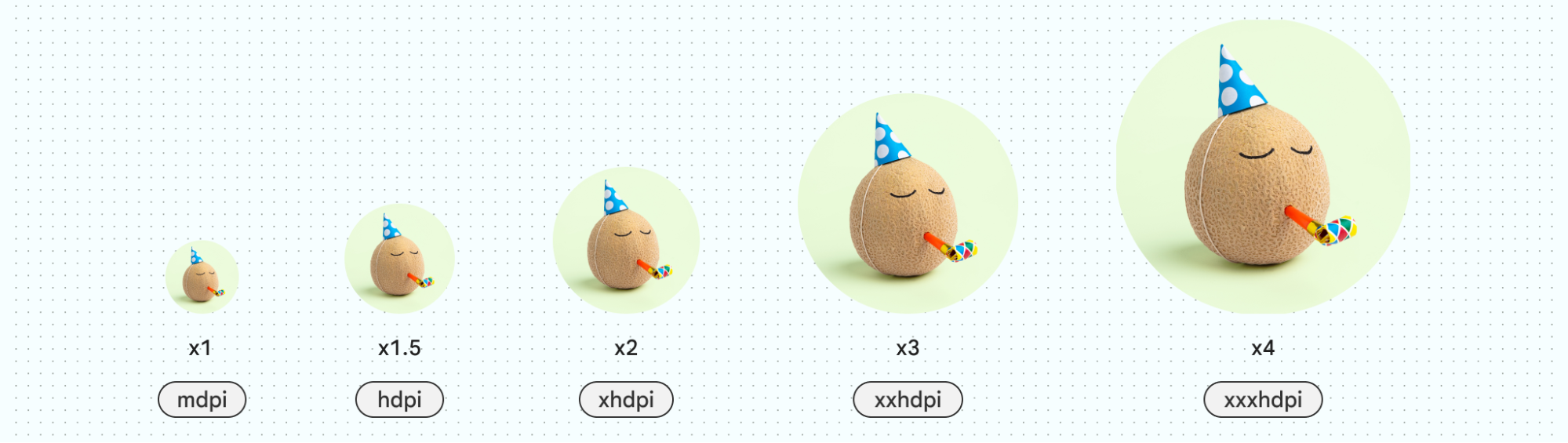
内边距
图标和类似的小资源应包括固有(内置)内边距,以便为资源提供足够的触摸目标空间并确保大小一致。
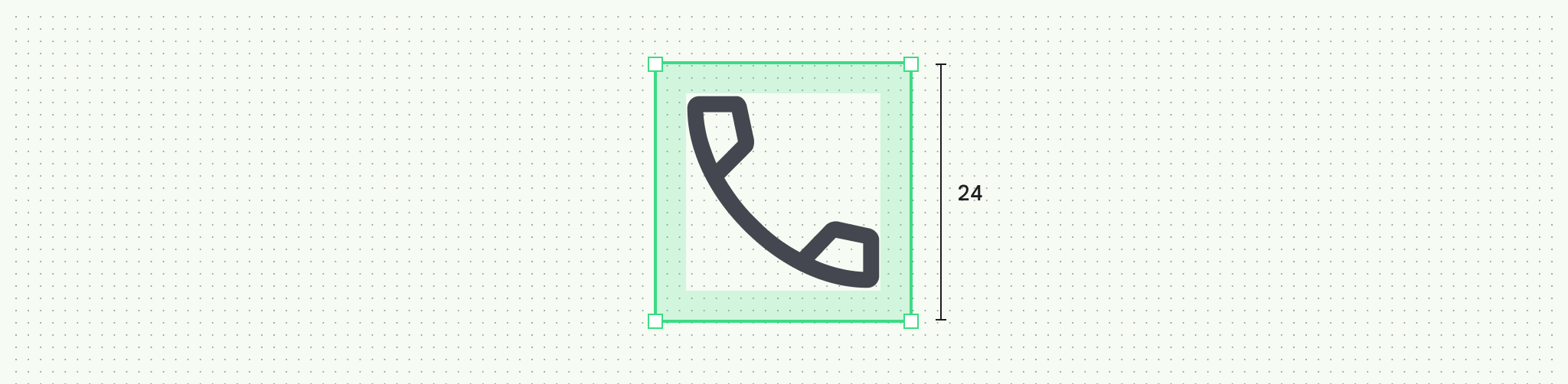
文件名
Android 资源均采用小写形式,且不包含分辨率后缀。
保持一致的命名惯例和结构,让您的文件和项目井然有序。例如,使用前缀“ic_...”为图标命名有助于整理项目中的所有图标,并有助于在开发过程中快速识别图标。
其他应用素材资源
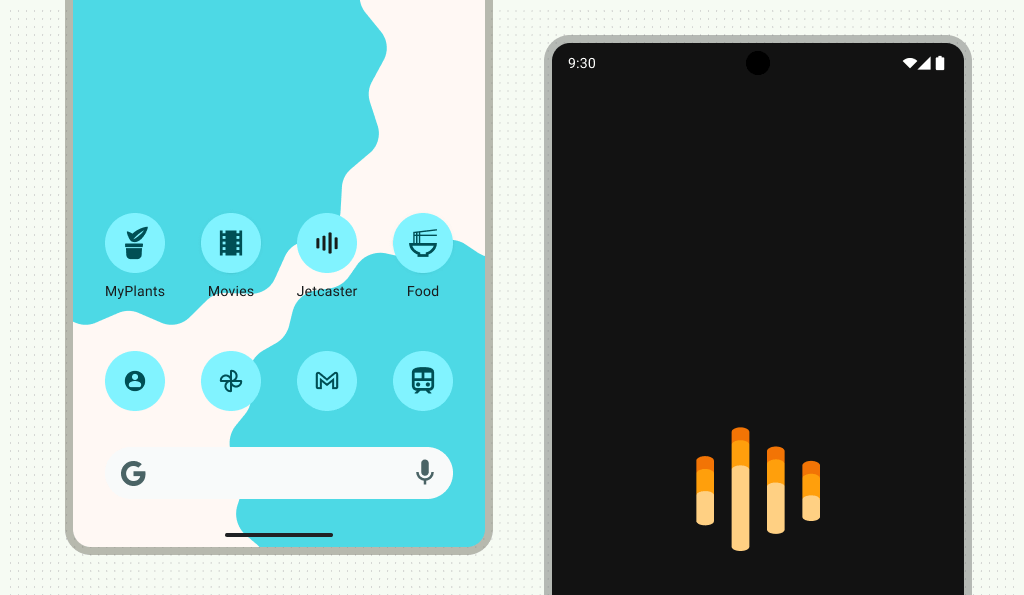
应用图标
可在主屏幕上找到启动器图标。在系统界面中查找单色图标,包括单色通知、状态栏和微件。
对于自适应图标,将应用图标格式设置为矢量可绘制对象;对于旧版图标,将格式设置为 PNG。如需详细了解如何创建和预览应用图标,请参阅设计和预览应用图标。
启动画面
从 Android 12 开始,您的应用可以在打开时显示一个动画启动画面,以便显示应用图标。
放置位置
请注意图片应如何缩放和在屏幕上的位置。Fit、Crop、FillHeight、 FillWidth、 FillBounds、Inside 和 None 可用于设置图片的缩放比例。
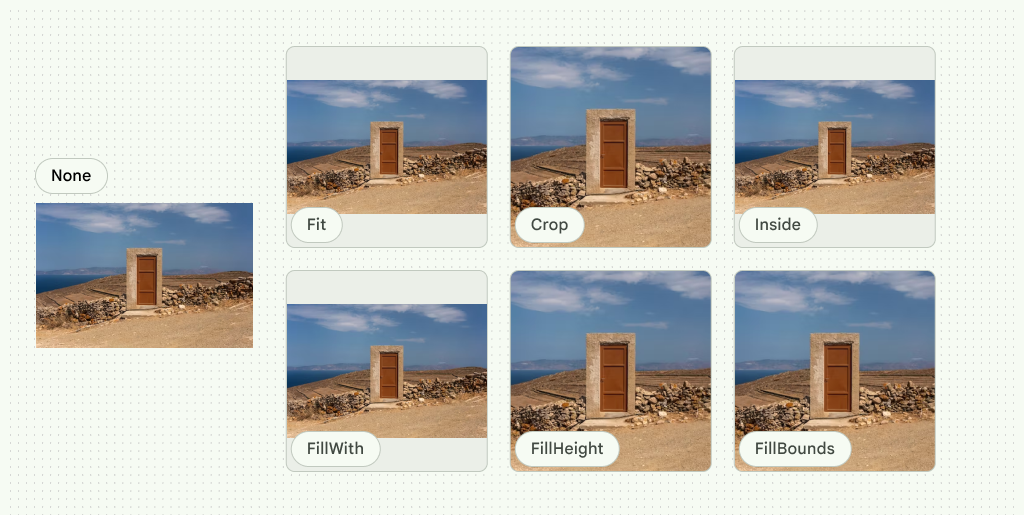
你还可以将图片裁剪为某种形状,以产生额外的效果。
自适应剪裁
如需以自适应方式显示图片,请定义图片在不同断点范围处的剪裁方式。在不同断点范围,剪裁功能可以:
- 保持一个固定的宽高比。
- 适应不同的宽高比。
- 保持固定的图片高度。
保持一个比率
调整图片大小可以跨断点范围保持一个固定比例。
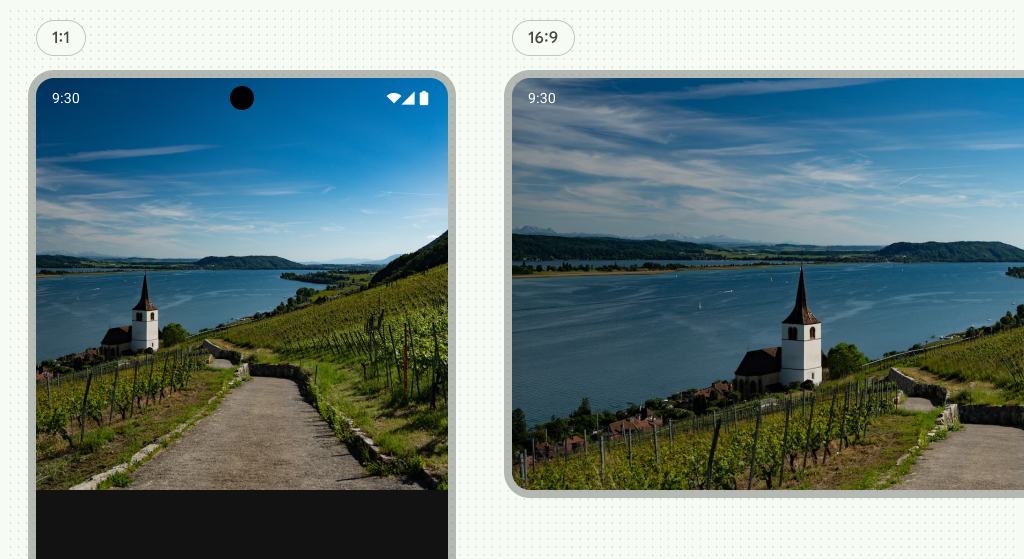
适应不同的宽高比
图像宽高比可以通过适应不同的断点范围而发生变化。例如,在图 9 中,在不同断点之间,图片宽高比从 1:1 更改为 16:9。
固定图片高度
调整图片大小时,可以在断点范围内和断点范围内保持固定的高度和固定宽度。图片保持固定的高度,而断点之间的宽度不固定。
特效
Android 包含各种内置的图片效果。以下是一些常见效果:
梯度
在 Compose 中,使用 Brush 在屏幕上绘制内容。您可以使用不同的画笔绘制不同颜色或渐变的形状。使用内置的渐变画笔来实现不同的渐变效果。您可以使用这些画笔指定要用于创建渐变的颜色的列表。
只要您提供停止点的颜色和百分比列表,渐变笔刷就可以通过添加颜色停止点和平铺效果来实现更高级的渐变效果。使用容器或形状来剪裁渐变、纯色填充或图片。

模糊处理
使用 Modifier.blur()
方法并提供模糊处理比率,将模糊处理效果应用于图片。请谨慎使用模糊处理功能,因为它们会影响性能,并且仅适用于搭载 Android 12 及更高版本的设备。如需了解详情,请参阅对图片可组合项进行模糊处理。
混合模式
Android 能够通过设计软件中类似的布尔运算和混合模式(例如并集或乘法)修改图片。如需了解详情,请参阅 BlendMode。
色调
使用混合模式和填充来为素材资源着色。这样,您就可以拥有单个资源并对其应用不同的颜色,从而减少生成的资源数量。
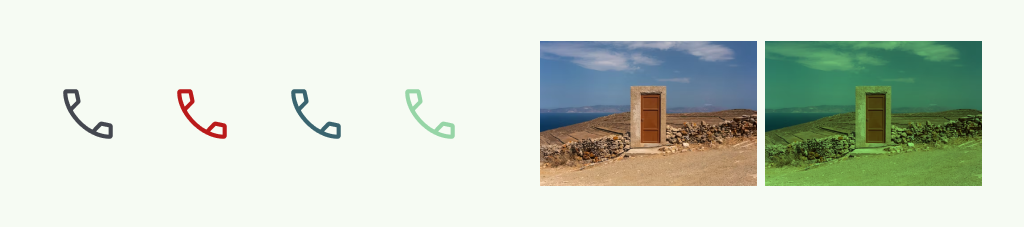
动画
您可以通过编程方式为图形添加动画效果,以便创建动态图形,而无需上传动态文件。这样可以提供更高的灵活性和更小的资产资源。
借助动画矢量可绘制对象,您可以创建小型界面动画。否则,请在 Compose 中详细了解如何使用关键帧添加动画效果。如需详细了解图片效果,请参阅自定义图片。