Android App Links 是指可将用户直接带往 Android 应用内特定内容的 HTTP 网址。Android App Links 可为您的应用带来更多流量,帮助您发现最常用的应用内容,并让用户更轻松地在已安装的应用中查找和分享内容。
若要添加对 Android App Links 的支持,请执行以下操作:
- 在清单中创建 intent 过滤器。
- 将代码添加到应用的 activity 中以处理传入链接。
- 使用 Digital Asset Links 将应用与网站相关联。
Android Studio 中的 App Links Assistant 以分步向导的方式简化了该流程,如下所述。
如需详细了解应用链接的运作方式及其提供的优势,请参阅处理 Android App Links。
添加 intent 过滤器
Android Studio 中的 App Links Assistant 可帮助您在清单中创建 intent 过滤器,并将您网站中的现有网址映射到应用中的 activity。App Links Assistant 还会在每个对应的 activity 中添加处理 intent 的模板代码。
若要添加 intent 过滤器和网址处理功能,请按以下步骤操作:
- 依次选择 Tools > App Links Assistant。
- 点击 Open URL Mapping Editor,然后点击 URL Mapping 列表底部的 Add
以添加新的网址映射。
添加新网址映射的详细信息:
图 1. 添加有关您网站的链接结构的基本详情,以将网址映射到应用中的 activity。
- 在 Host 字段中输入您网站的网址。
为您要映射的网址添加
path
、pathPrefix
或pathPattern
。例如,如果您有一个食谱分享应用,所有食谱都在同一个 activity 中,并且对应网站的食谱都在同一个“/recipe”目录中,请使用 pathPrefix 并输入“/recipe”。这样,网址 http://www.recipe-app.com/recipe/grilled-potato-salad 就会映射到您在下一步中选择的 activity。
- 选择网址应将用户转至的 Activity。
- 点击 OK。
此时会显示 URL Mapping Editor(网址映射编辑器)窗口。App Links Assistant 会根据您映射到
AndroidManifest.xml
文件的网址添加 intent 过滤器,并在 Preview 字段中突出显示更改。如果您要进行任何更改,请点击 Open AndroidManifest.xml 以修改 intent 过滤器。如需了解详情,请参阅为传入链接添加 intent 过滤器。主 App Links Assistant 工具窗口还会显示
AndroidManifest.xml
文件中的所有现有深层链接,并且您可以通过点击 Fix All Manifest Issues 快速修复任何错误配置。注意:若要在不更新应用的情况下支持更多链接,请定义一个网址映射,让该映射支持您计划添加的网址。此外,请为您的应用主屏幕添加一个网址,使其包含在搜索结果中。
如需验证网址映射是否正常运行,请在 Check URL Mapping 字段中输入网址。
如果网址映射正常运行,成功消息会显示您输入的网址映射到您选择的 activity。
处理传入链接
确认网址映射正常运行后,请添加逻辑以处理您创建的 intent:
- 点击 App Links Assistant 中的 Select Activity。
- 从列表中选择一个 activity,然后点击 Insert Code。
App Links Assistant 会将代码添加到 activity 中,类似于以下代码段:
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) ... // ATTENTION: This was auto-generated to handle app links. val appLinkIntent: Intent = intent val appLinkAction: String? = appLinkIntent.action val appLinkData: Uri? = appLinkIntent.data ... }
Java
@Override void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); ... // ATTENTION: This was auto-generated to handle app links. Intent appLinkIntent = getIntent(); String appLinkAction = appLinkIntent.getAction(); Uri appLinkData = appLinkIntent.getData(); ... }
上述代码并不完整。您现在必须根据 appLinkData
中的 URI 执行一项操作,例如显示相应内容。例如,对于食谱分享应用,您的代码可能如以下示例所示:
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) ... handleIntent(intent) } override fun onNewIntent(intent: Intent) { super.onNewIntent(intent) handleIntent(intent) } private fun handleIntent(intent: Intent) { val appLinkAction = intent.action val appLinkData: Uri? = intent.data if (Intent.ACTION_VIEW == appLinkAction) { appLinkData?.lastPathSegment?.also { recipeId -> Uri.parse("content://com.recipe_app/recipe/") .buildUpon() .appendPath(recipeId) .build().also { appData -> showRecipe(appData) } } } }
Java
protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); ... handleIntent(getIntent()); } protected void onNewIntent(Intent intent) { super.onNewIntent(intent); handleIntent(intent); } private void handleIntent(Intent intent) { String appLinkAction = intent.getAction(); Uri appLinkData = intent.getData(); if (Intent.ACTION_VIEW.equals(appLinkAction) && appLinkData != null){ String recipeId = appLinkData.getLastPathSegment(); Uri appData = Uri.parse("content://com.recipe_app/recipe/").buildUpon() .appendPath(recipeId).build(); showRecipe(appData); } }
将应用与网站相关联
在为您的应用设置网址支持后,App Links Assistant 会生成一个 Digital Asset Links 文件,您可以使用该文件将网站与应用相关联。
除了使用 Digital Asset Links 文件,您还可以在 Search Console 中将您的网站与应用相关联。
如果您的应用使用的是 Play 应用签名,则 App Links Assistant 生成的证书指纹通常与用户设备上的证书指纹不匹配。在这种情况下,您可以在 Play 管理中心开发者账号的 Release > Setup > App signing 下找到正确的 Digital Asset Links JSON 代码段。
如需使用 App Links Assistant 将您的应用与网站相关联,请点击 App Links Assistant 中的 Open Digital Asset Links File Generator,然后按照以下步骤操作:
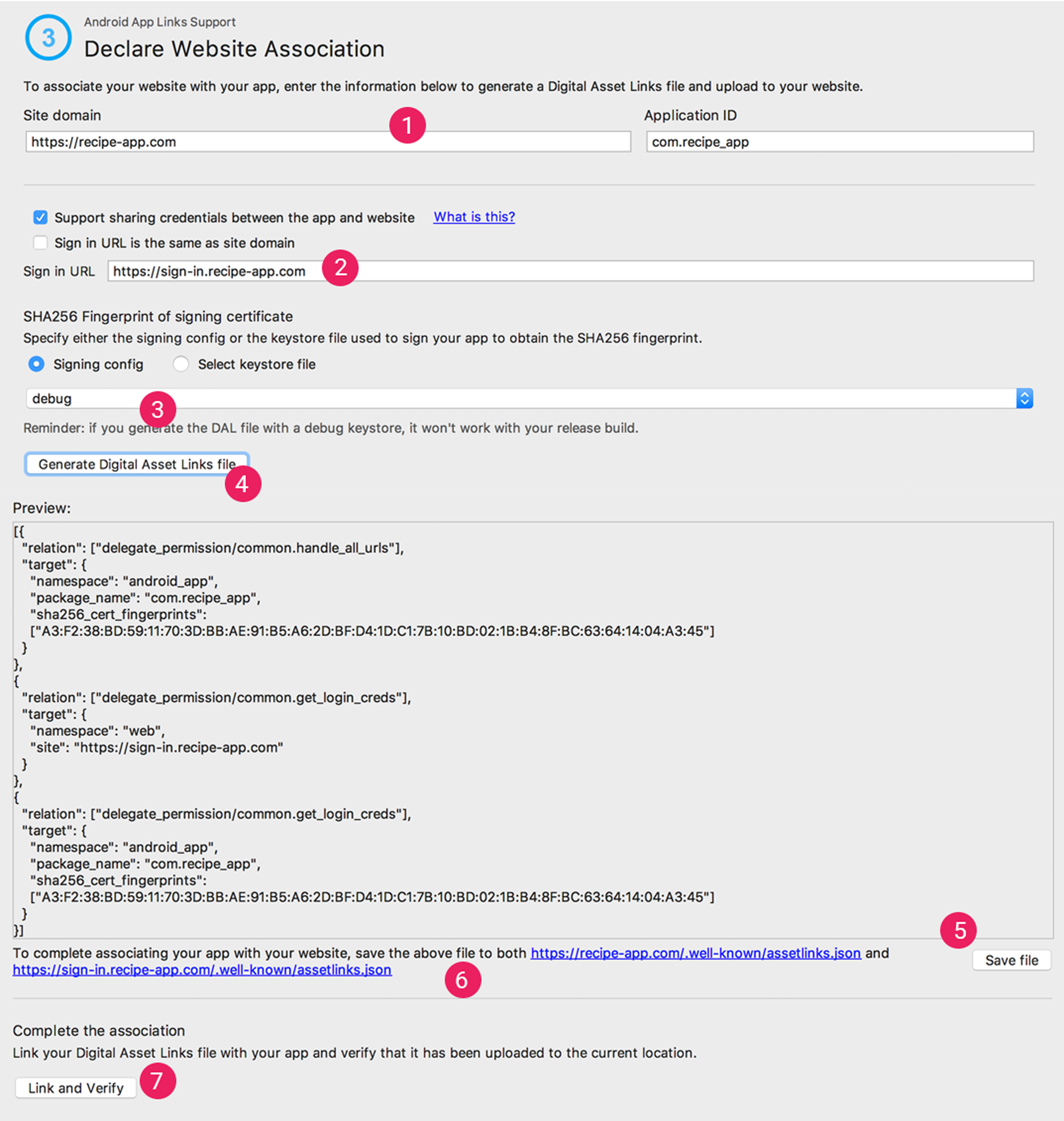
图 2. 输入有关您的网站和应用的详细信息,以生成 Digital Asset Links 文件。
- 输入您的 Site domain 和 Application ID。
如需在 Digital Asset Links 文件中添加对一键登录的支持,请选择 Support sharing credentials between the app and the website,输入网站的登录网址。此操作会将以下字符串添加到 Digital Asset Links 文件中,声明应用和网站共享登录凭据:
delegate_permission/common.get_login_creds
。-
请确保为应用的发布 build 选择正确的发布配置或密钥库文件,或为应用的调试 build 选择调试配置或密钥库文件。如果要设置正式版 build,请使用发布配置。如果要测试 build,请使用调试配置。
- 点击 Generate Digital Asset Links file。
- Android Studio 生成文件后,点击 Save file 进行下载。
- 将
assetlinks.json
文件上传到您的网站并允许所有人读取,网址为https://yoursite/.well-known/assetlinks.json
。重要提示:系统会通过加密的 HTTPS 协议验证 Digital Asset Links 文件。请确保无论应用的 intent 过滤器是否包括
https
,均可通过 HTTPS 连接访问assetlinks.json
文件。 - 点击 Link and Verify 以确认您已将正确的 Digital Asset Links 文件上传到适当的位置。
App Link Assistant 可以验证应发布到的 Digital Asset Links 文件 。对于清单文件中声明的每个网域,Google 助理会解析相应文件 执行验证检查,并详细说明如何修正 错误。
如需详细了解如何通过 Digital Asset Links 文件将网站与应用相关联,请访问声明网站关联。
测试 Android App Links
如需验证您的链接是否会打开正确的 activity,请按以下步骤操作:
- 在 App Links Assistant 中,点击 Test App Links。
- 在 URL 字段中输入要测试的网址,例如 http://recipe-app.com/recipe/grilled-potato-salad。
- 点击 Run Test。
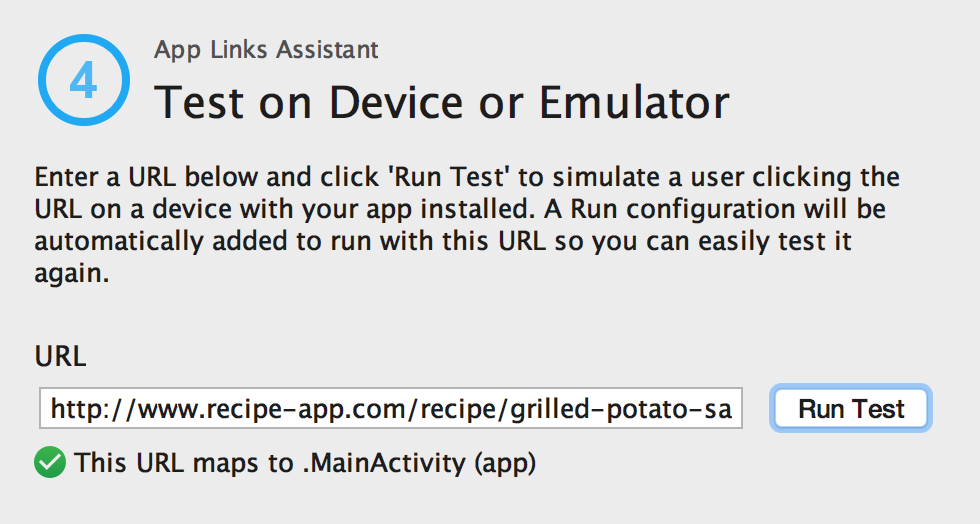
图 3. Test App Links 对话框,其中显示正在测试的网址及成功消息。
如果网址映射未正确设置或不存在,Test App Links 对话框中的网址下会显示一条错误消息。如果存在正确设置的网址映射,Android Studio 会在设备或模拟器的指定 activity 中启动您的应用,而不显示消除歧义对话框(“应用选择器”),并在 App Link Testing 对话框中显示一条成功消息,如图 3 所示。
如果 Android Studio 无法启动该应用,Android Studio 的 Run 窗口中就会显示一条错误消息。
如需通过 App Links Assistant 测试 Android App Links,您必须有一个搭载 Android 6.0(API 级别 23)或更高版本的已连接设备或虚拟设备。如需了解详情,请参阅介绍如何连接设备或创建 AVD 的相关文档。