图标有助于用户识别您的应用和应用中的操作。您可以使用 Compose 访问 Material 图标套件,并直接将这些图标添加到应用中。如要使用 View 为应用创建自定义图标或一般图标,不妨试试 Android Studio 提供的 Image Asset Studio。
使用 Compose 在应用中添加图标
您可以使用 Compose 导入 Compose Material 库或 Compose Material 3 库来访问任何 Material 图标。然后,使用 Icon
可组合项将图标添加到应用中。Material 图标最适合创建操作栏图标、标签页图标或通知图标。如需了解详情,请参阅 Material 图标。
如果您需要创建自定义图标,例如应用启动器图标,请使用 Image Asset Studio。对于启动器图标,AndroidManifest.xml
文件必须引用 mipmap/
位置。Image Asset Studio 会自动添加此代码。以下清单文件代码引用了 mipmap/
目录中的 ic_launcher
图标:
<application android:name="ApplicationTitle" android:label="@string/app_label" android:icon="@mipmap/ic_launcher" >
关于 Image Asset Studio
Android Studio 包含一个名为 Image Asset Studio 的工具,可帮助您根据 Material 图标、自定义图片和文本字符串生成自己的应用图标。它会针对应用支持的每种像素密度以适当的分辨率生成一组图标。Image Asset Studio 会将新生成的图标放置在项目中 res/
目录下的密度专用文件夹中。在运行时,Android 将根据运行应用的设备的屏幕密度来使用适当的资源。
Image Asset Studio 可帮助您生成以下类型的图标:
- 启动器图标
- 操作栏和标签页图标
- 通知图标
下面几部分将说明您可以创建的图标类型以及您可以使用的图片和文本输入。
自适应和旧版启动器图标
启动器图标是一个图形,用来向用户表示您的应用。它可以:
- 显示在设备上已安装应用的列表中和主屏幕上。
- 表示进入您的应用的快捷方式(例如,点击联系人快捷方式图标可以打开联系人的详细信息)。
- 由启动器应用使用。
- 帮助用户在 Google Play 上查找您的应用。
Android 8.0(API 级别 26)及更高版本提供了自适应启动器图标,这些图标可在不同型号的设备上显示为各种不同的形状。Android Studio 3.0 支持使用 Image Asset Studio 创建自适应图标。Image Asset Studio 会生成自适应图标的圆形、方圆形、圆角方形和方形预览,以及图标的全宽预览。Image Asset Studio 还会生成图标的旧版、圆形和 Google Play 商店预览。旧版启动器图标是一个图形,用来在设备的主屏幕上以及启动器窗口中表示您的应用。旧版启动器图标适用于搭载 Android 7.1(API 级别 25)或更低版本的设备(这些设备不支持自适应图标),它们不会在不同型号的设备上显示为各种不同的形状。
Image Asset Studio 会将图标放置在 res/mipmap-density/
目录中的适当位置。它还会创建一张 512 x 512 像素的图片,该图片适合在 Google Play 商店中使用。
我们建议您对启动器图标使用 Material Design 样式,即使您的应用支持较低的 Android 版本。
如需了解详情,请参阅自适应启动器图标和产品图标 - Material Design。
操作栏和标签页图标
操作栏图标是置于操作栏中的图形元素,它们表示各个操作项。如需了解详情,请参阅添加和处理操作、应用栏 - Material Design 和操作栏设计。
标签页图标是用于在多标签页界面中表示各个标签页的图形元素。每个标签页图标都有两种状态:未选中和选中。如需了解详情,请参阅使用标签页创建滑动视图和标签页 - Material Design。
Image Asset Studio 会将图标放置在 res/drawable-density/
目录中的适当位置。
建议您对操作栏和标签页图标使用 Material Design 样式,即使您的应用支持较低的 Android 版本。使用 appcompat
和其他支持库可以向较低版本的平台提供您的 Material Design 界面。
除了使用 Image Asset Studio,您还可以使用 Vector Asset Studio 创建操作栏和标签页图标。矢量可绘制对象适合用来创建简单图标,并且可以减小应用的大小。
通知图标
通知是您可以在应用的正常界面之外向用户显示的消息。Image Asset Studio 会将通知图标放置在 res/drawable-density/
目录中的适当位置:
- 对于 Android 2.2(API 级别 8)及更低版本,图标放置在
res/drawable-density/
目录中。 - 对于 Android 2.3 到 2.3.7(API 级别 9 到 10),图标放置在
res/drawable-density-v9/
目录中。 - 对于 Android 3(API 级别 11)及更高版本,图标放置在
res/drawable-density-v11/
目录中。
如果您的应用支持 Android 2.3 到 2.3.7(API 级别 9 到 10),Image Asset Studio 会生成灰色版本的图标。更高版本的 Android 使用 Image Asset Studio 生成的白色图标。
如需了解详情,请参阅通知、通知 Material Design、Android 5.0 中的通知变更、Android 4.4 及更低版本中的通知和 Android 3.0 及更低版本中的状态栏图标。
剪贴画
利用 Image Asset Studio,您可以轻松地导入 VectorDrawable 和 PNG 格式的 Google Material 图标,只需从对话框中选择一个图标即可。如需了解详情,请参阅 Material 图标。
图片
您可以导入自己的图片并针对图标类型对其进行调整。Image Asset Studio 支持以下文件类型:PNG(首选)、JPG(可接受)和 GIF(不推荐)。
文本字符串
Image Asset Studio 支持您输入各种字体的文本字符串,并将其放置在图标上。它会针对不同的密度将基于文本的图标转换为 PNG 文件。您可以使用计算机上安装的字体。
运行 Image Asset Studio
如需启动 Image Asset Studio,请按以下步骤操作:
- 在 Project 窗口中,选择 Android 视图。
- 右键点击 res 文件夹,然后依次选择 New > Image Asset。
- 继续执行以下操作步骤:
- 创建自适应和旧版启动器图标。
- 创建操作栏或标签页图标。
- 创建通知图标。
创建自适应和旧版启动器图标
打开 Image Asset Studio 后,您可以按照以下步骤添加自适应和旧版图标:
- 在 Icon Type 字段中,选择 Launcher Icons (Adaptive & Legacy)。
- 在 Foreground Layer 标签页的 Asset Type 中选择一种资源类型,然后在下面的字段中指定资源:
- 选择 Image 以指定图片文件的路径。
- 选择 Clip Art 以指定 Material Design 图标集中的一张图片。
- 选择 Text 以指定文本字符串并选择字体。
- 在 Background Layer 标签页的 Asset Type 中选择一种资源类型,然后在下面的字段中指定资源。您可以选择一种颜色或指定一张图片作为背景图层。
- 在 Legacy 标签页中,查看默认设置并确认您要生成旧版、圆形和 Google Play 商店中使用的图标。
- (可选)在 Foreground Layer 和 Background Layer 标签页中更改每个图标的名称和显示设置:
- Name - 如果您不想使用默认名称,请输入新名称。如果项目中已存在该资源名称(由向导底部的错误来指示),它将被覆盖。名称只能包含小写字符、下划线和数字。
- Trim - 要调整源资源中图标图形与边框之间的边距,请选择 Yes。此操作将移除透明空间,同时让宽高比保持不变。要让源资源保持不变,请选择 No。
- Color - 要更改 Clip Art 或 Text 图标的颜色,请点击该字段。在 Select Color 对话框中,指定一种颜色,然后点击 Choose。该字段中会显示新值。
- Resize - 使用滑块指定缩放比例(以百分比表示)以调整 Image、Clip Art 或 Text 图标的大小。如果指定了 Color 资源类型,系统会为背景图层停用此控件。
- 点击 Next。
- (可选)更改资源目录。选择想在其中添加图片资源的资源源代码集:src/main/res、src/debug/res、src/release/res 或自定义源代码集。主源代码集适用于所有构建变体,包括调试和发布。调试和发布源代码集将替换主源代码集,并应用于构建的一个版本。调试源代码集仅用于调试。要定义新源代码集,请依次选择 File > Project Structure > app > Build Types。例如,您可以定义一个 Beta 版源代码集,并创建一个版本的图标,使其右下角包含文本“BETA”。如需了解详情,请参阅配置构建变体。
- 点击 Finish。Image Asset Studio 会针对不同的密度将图片添加到 mipmap 文件夹。
预览带主题的应用图标
Android Studio 允许您预览带主题的应用图标,并测试该图标如何适应用户壁纸的颜色。若要预览带主题的应用图标,请打开用于定义图标的 launcher.xml
文件,然后使用工具栏上的 System UI Mode 选择器切换壁纸并查看图标显示效果。
如需详细了解如何创建带主题的应用图标,请参阅自适应图标。
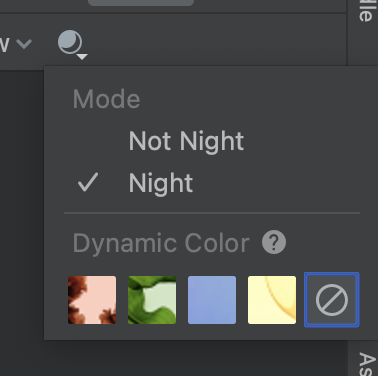
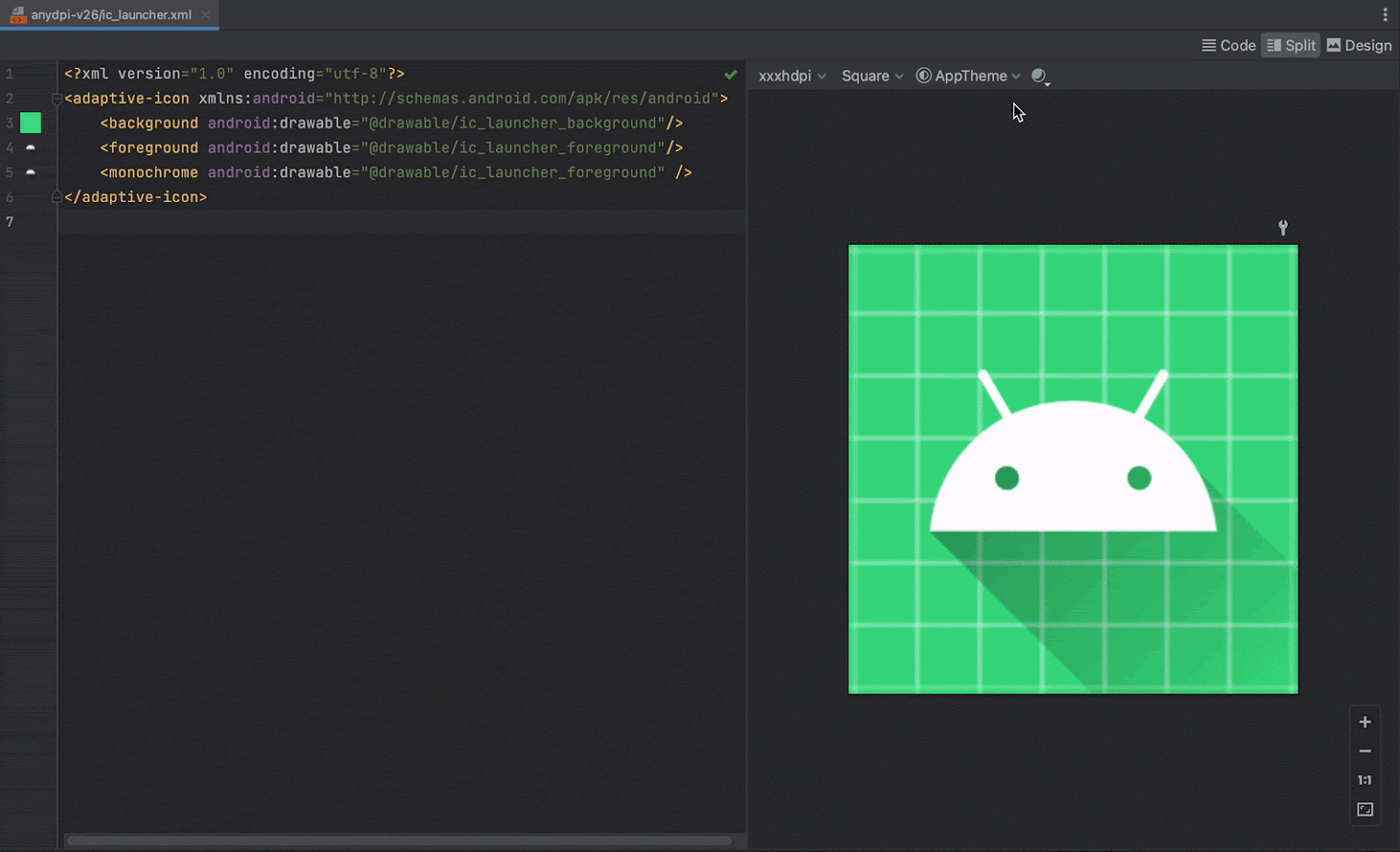
创建操作栏或标签页图标
打开 Image Asset Studio 后,您可以按照以下步骤添加操作栏或标签页图标:
- 在 Icon Type 字段中,选择 Action Bar and Tab Icons。
- 在 Asset Type 中选择一种资源类型,然后在下面的字段中指定资源:
- 在 Clip Art 字段中,点击相应按钮。
- 在 Path 字段中,指定图片的路径和文件名。点击 … 以使用对话框。
- 在 Text 字段中,输入文本字符串并选择字体。
- (可选)更改名称和显示选项:
- Name - 如果您不想使用默认名称,请输入新名称。如果项目中已存在该资源名称(由向导底部的错误来指示),它将被覆盖。名称只能包含小写字符、下划线和数字。
- Trim - 要调整源资源中图标图形与边框之间的边距,请选择 Yes。此操作将移除透明空间,同时让宽高比保持不变。要让源资源保持不变,请选择 No。
- Padding - 如果要调整全部四面的源资源内边距,请移动滑块。选择一个介于 -10% 和 50% 之间的值。如果也选择了 Trim,则先进行剪裁。
- Theme - 选择 HOLO_LIGHT 或 HOLO_DARK。或者,要在 Select Color 对话框中指定颜色,请选择 CUSTOM,然后点击 Custom color 字段。
Image Asset Studio 会在透明的正方形内创建图标,所以边缘上有一些内边距。内边距为标准的阴影图标效果提供了充足的空间。
- 点击 Next。
- (可选)更改资源目录:
- Res Directory - 选择想在其中添加图片资源的资源源代码集:src/main/res、src/debug/res、src/release/res 或用户定义的源代码集。主源代码集适用于所有 build 变体,包括调试和发布 build。调试和发布源代码集将替换主源代码集,并应用于 build 的一个版本。调试源代码集仅用于调试。要定义新源代码集,请依次选择 File > Project Structure > app > Build Types。例如,您可以定义一个 Beta 版源代码集,并创建一个图标版本,在其右下角添加“BETA”字样。如需了解详情,请参阅配置构建变体。
- 点击 Finish。
在 Select Icon 对话框中,选择一个素材图标,然后点击 OK。
图标会显示在右侧的 Source Asset 区域以及向导底部的预览区域中。
Output Directories 区域会显示图片以及它们将出现在 Project 窗口的“Project Files”视图中的哪些文件夹内。
Image Asset Studio 会针对不同的密度将图片添加到 drawable 文件夹。
创建通知图标
打开 Image Asset Studio 后,您可以按照以下步骤添加通知图标:
- 在 Icon Type 字段中,选择 Notification Icons。
- 在 Asset Type 中选择一种资源类型,然后在下面的字段中指定资源:
- 在 Clip Art 字段中,点击相应按钮。
- 在 Path 字段中,指定图片的路径和文件名。点击 … 以使用对话框。
- 在 Text 字段中,输入文本字符串并选择字体。
- (可选)更改名称和显示选项:
- Name - 如果您不想使用默认名称,请输入新名称。如果项目中已存在该资源名称(由向导底部的错误来指示),它将被覆盖。名称只能包含小写字符、下划线和数字。
- Trim - 要调整源资源中图标图形与边框之间的边距,请选择 Yes。此操作将移除透明空间,同时让宽高比保持不变。要让源资源保持不变,请选择 No。
- Padding - 如果要调整全部四面的源资源内边距,请移动滑块。选择一个介于 -10% 和 50% 之间的值。如果也选择了 Trim,则先进行剪裁。
Image Asset Studio 会在透明的正方形内创建图标,所以边缘上有一些内边距。内边距为标准的阴影图标效果提供了充足的空间。
- 点击 Next。
- (可选)更改资源目录:
- Res Directory - 选择想在其中添加图片资源的资源源代码集:src/main/res、src/debug/res、src/release/res 或用户定义的源代码集。主源代码集适用于所有 build 变体,包括调试和发布 build。调试和发布源代码集将替换主源代码集,并应用于 build 的一个版本。调试源代码集仅用于调试。要定义新源代码集,请依次选择 File > Project Structure > app > Build Types。例如,您可以定义一个 Beta 版源代码集,并创建一个图标版本,在其右下角添加“BETA”字样。如需了解详情,请参阅配置构建变体。
- 点击 Finish。
在 Select Icon 对话框中,选择一个素材图标,然后点击 OK。
图标会显示在右侧的 Source Asset 区域以及向导底部的预览区域中。
Output Directories 区域会显示图片以及它们将出现在 Project 窗口的“Project Files”视图中的哪些文件夹内。
Image Asset Studio 会针对不同的密度和版本将图片添加到 drawable 文件夹。
使用 View 在代码中引用图片资源
您通常可以在代码中以通用方式引用图片资源,当应用运行时,系统会根据设备自动显示对应的图片:
- 大多数情况下,您可以在 XML 代码中以
@drawable
的形式引用图片资源,或在 Java 代码中以Drawable
的形式引用图片资源。 - 如果您的应用使用支持库,您可以通过
app:srcCompat
语句在 XML 代码中引用图片资源。例如:
例如,以下布局 XML 代码会在 ImageView 中显示可绘制对象:
<ImageView android:layout_height="wrap_content" android:layout_width="wrap_content" android:src="@drawable/myimage" />
以下 Java 代码会以 Drawable
的形式检索图片:
Kotlin
val drawable = resources.getDrawable(R.drawable.myimage, theme)
Java
Resources res = getResources(); Drawable drawable = res.getDrawable(R.drawable.myimage, getTheme());
getResources()
方法位于 Context
类中,它适用于界面对象,如 Activity、Fragment、布局和视图等。
<ImageView android:layout_height="wrap_content" android:layout_width="wrap_content" app:srcCompat="@drawable/myimage" />
您只能从主线程访问图片资源。
项目的 res/
目录中有了某个图片资源后,您可以使用其资源 ID 从 Java 代码或 XML 布局中对其进行引用。以下 Java 代码将 ImageView 设置为使用 drawable/myimage.png
资源:
Kotlin
findViewById<ImageView>(R.id.myimageview).apply { setImageResource(R.drawable.myimage) }
Java
ImageView imageView = (ImageView) findViewById(R.id.myimageview); imageView.setImageResource(R.drawable.myimage);
如需了解详情,请参阅访问资源。
从项目中删除图标
如需从项目中移除图标,请执行以下操作:
- 在 Project 窗口中,选择 Android 视图。
- 展开启动器图标的 res/mipmap 文件夹,或其他类型图标的 res/drawable 文件夹。
- 找到具有您要删除的图标名称的子文件夹。
- 选择该文件夹,然后按 Delete 键。
- (可选)选择适当的选项以在项目中查找使用该图标的位置,然后点击 OK。
- 依次选择 Build > Clean Project。
- 如果需要,请更正由引用资源的代码部分引起的其余所有错误。
此文件夹包含不同密度的图标。
或者,依次选择 Edit > Delete。又或右键点击相应的文件,然后选择 Delete。
此时将显示 Safe Delete 对话框。
Android Studio 会从项目和驱动器中删除这些文件。不过,如果您选择在项目中搜索使用这些文件的位置,在找到部分位置后,您可以查看这些位置,再决定是否删除这些文件。您必须删除或替换这些引用,才能成功编译项目。
Android Studio 会移除所有生成的与已删除图片资源对应的图片文件。它会从项目和驱动器中移除这些文件。
Android Studio 会在您的代码中突出显示这些错误。从您的代码中移除所有引用后,您便可以成功地重新构建项目。