CameraX 提供擴充功能 API,可用於存取裝置製造商在不同 Android 裝置上實作的擴充功能。如需支援的擴充功能模式清單,請參閱「相機擴充功能」。
如需支援擴充功能的裝置清單,請參閱「支援的裝置」。
擴充功能架構
下圖顯示相機擴充功能的架構。
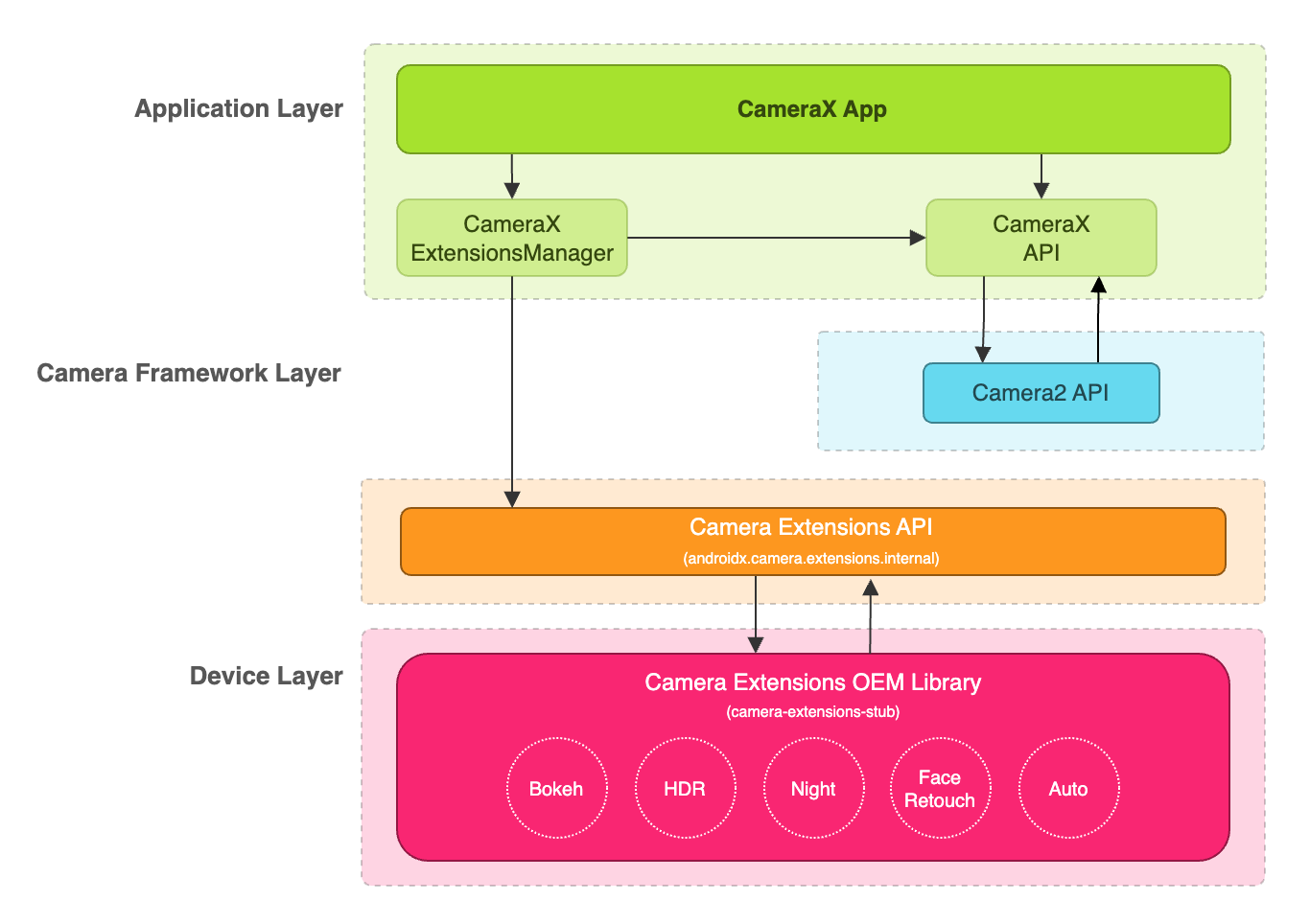
CameraX 應用程式可透過 CameraX Extensions API 使用擴充功能。CameraX Extensions API 會管理以下作業:查詢可用擴充功能、設定擴充功能相機工作階段,以及與 Camera Extensions OEM 程式庫通訊。這可讓應用程式使用「夜晚」、「高動態範圍」、「自動」、「散景」或「修容」等功能。
啟用拍照和預覽的擴充功能
使用 Extensions API 前,請先用 ExtensionsManager#getInstanceAsync(Context, CameraProvider) 方法擷取 ExtensionsManager
執行個體。如此一來,即可查詢擴充功能的可用性資訊。然後擷取已啟用擴充功能的 CameraSelector
。搭配擴充功能已啟用的 CameraSelector
,呼叫 bindToLifecycle() 方法後,擴充功能模式便會套用在拍照和預覽用途。
如要導入拍照與預覽用途的擴充功能,請參閱以下程式碼範例:
Kotlin
import androidx.camera.extensions.ExtensionMode import androidx.camera.extensions.ExtensionsManager override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) val lifecycleOwner = this val cameraProviderFuture = ProcessCameraProvider.getInstance(applicationContext) cameraProviderFuture.addListener({ // Obtain an instance of a process camera provider // The camera provider provides access to the set of cameras associated with the device. // The camera obtained from the provider will be bound to the activity lifecycle. val cameraProvider = cameraProviderFuture.get() val extensionsManagerFuture = ExtensionsManager.getInstanceAsync(applicationContext, cameraProvider) extensionsManagerFuture.addListener({ // Obtain an instance of the extensions manager // The extensions manager enables a camera to use extension capabilities available on // the device. val extensionsManager = extensionsManagerFuture.get() // Select the camera val cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA // Query if extension is available. // Not all devices will support extensions or might only support a subset of // extensions. if (extensionsManager.isExtensionAvailable(cameraSelector, ExtensionMode.NIGHT)) { // Unbind all use cases before enabling different extension modes. try { cameraProvider.unbindAll() // Retrieve a night extension enabled camera selector val nightCameraSelector = extensionsManager.getExtensionEnabledCameraSelector( cameraSelector, ExtensionMode.NIGHT ) // Bind image capture and preview use cases with the extension enabled camera // selector. val imageCapture = ImageCapture.Builder().build() val preview = Preview.Builder().build() // Connect the preview to receive the surface the camera outputs the frames // to. This will allow displaying the camera frames in either a TextureView // or SurfaceView. The SurfaceProvider can be obtained from the PreviewView. preview.setSurfaceProvider(surfaceProvider) // Returns an instance of the camera bound to the lifecycle // Use this camera object to control various operations with the camera // Example: flash, zoom, focus metering etc. val camera = cameraProvider.bindToLifecycle( lifecycleOwner, nightCameraSelector, imageCapture, preview ) } catch (e: Exception) { Log.e(TAG, "Use case binding failed", e) } } }, ContextCompat.getMainExecutor(this)) }, ContextCompat.getMainExecutor(this)) }
Java
import androidx.camera.extensions.ExtensionMode; import androidx.camera.extensions.ExtensionsManager; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); final LifecycleOwner lifecycleOwner = this; final ListenableFuturecameraProviderFuture = ProcessCameraProvider.getInstance(getApplicationContext()); cameraProviderFuture.addListener(() -> { try { // Obtain an instance of a process camera provider // The camera provider provides access to the set of cameras associated with the // device. The camera obtained from the provider will be bound to the activity // lifecycle. final ProcessCameraProvider cameraProvider = cameraProviderFuture.get(); final ListenableFuture extensionsManagerFuture = ExtensionsManager.getInstanceAsync(getApplicationContext(), cameraProvider); extensionsManagerFuture.addListener(() -> { // Obtain an instance of the extensions manager // The extensions manager enables a camera to use extension capabilities available // on the device. try { final ExtensionsManager extensionsManager = extensionsManagerFuture.get(); // Select the camera final CameraSelector cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA; // Query if extension is available. // Not all devices will support extensions or might only support a subset of // extensions. if (extensionsManager.isExtensionAvailable( cameraSelector, ExtensionMode.NIGHT )) { // Unbind all use cases before enabling different extension modes. cameraProvider.unbindAll(); // Retrieve extension enabled camera selector final CameraSelector nightCameraSelector = extensionsManager .getExtensionEnabledCameraSelector(cameraSelector, ExtensionMode.NIGHT); // Bind image capture and preview use cases with the extension enabled camera // selector. final ImageCapture imageCapture = new ImageCapture.Builder().build(); final Preview preview = new Preview.Builder().build(); // Connect the preview to receive the surface the camera outputs the frames // to. This will allow displaying the camera frames in either a TextureView // or SurfaceView. The SurfaceProvider can be obtained from the PreviewView. preview.setSurfaceProvider(surfaceProvider); cameraProvider.bindToLifecycle( lifecycleOwner, nightCameraSelector, imageCapture, preview ); } } catch (ExecutionException | InterruptedException e) { throw new RuntimeException(e); } }, ContextCompat.getMainExecutor(this)); } catch (ExecutionException | InterruptedException e) { throw new RuntimeException(e); } }, ContextCompat.getMainExecutor(this)); }
停用擴充功能
如要停用廠商擴充功能,請取消繫結所有用途,然後利用一般相機選取器,重新繫結拍照與預覽用途。例如,使用 CameraSelector.DEFAULT_BACK_CAMERA
重新繫結至後置鏡頭。
依附元件
CameraX Extensions API 已導入到 camera-extensions
程式庫。擴充功能與 CameraX 核心模組 (core
、camera2
、lifecycle
) 具有相依關係。
Groovy
dependencies { def camerax_version = "1.2.0-rc01" implementation "androidx.camera:camera-core:${camerax_version}" implementation "androidx.camera:camera-camera2:${camerax_version}" implementation "androidx.camera:camera-lifecycle:${camerax_version}" //the CameraX Extensions library implementation "androidx.camera:camera-extensions:${camerax_version}" ... }
Kotlin
dependencies { val camerax_version = "1.2.0-rc01" implementation("androidx.camera:camera-core:${camerax_version}") implementation("androidx.camera:camera-camera2:${camerax_version}") implementation("androidx.camera:camera-lifecycle:${camerax_version}") // the CameraX Extensions library implementation("androidx.camera:camera-extensions:${camerax_version}") ... }
移除舊版 API
發布新版 Extensions API (1.0.0-alpha26
版本) 後,我們目前已淘汰於 2019 年 8 月發布的舊版 Extensions API。自 1.0.0-alpha28
版本起,舊版 Extensions API 已從程式庫中移除。現在,使用新版 Extensions API 的應用程式,必須取得已啟用擴充功能的 CameraSelector
,並用其繫結用途。
使用舊版 Extensions API 的應用程式,則應遷移到新版 Extensions API,以確保日後與即將發布的 CameraX 版本相容。
其他資源
如要進一步瞭解 CameraX,請參閱下列其他資源。
程式碼研究室