Google-এর মাধ্যমে সাইন ইন করুন আপনাকে আপনার Android অ্যাপের সাথে ব্যবহারকারীর প্রমাণীকরণ দ্রুত সংহত করতে সাহায্য করে। ব্যবহারকারীরা তাদের Google অ্যাকাউন্ট ব্যবহার করে আপনার অ্যাপে সাইন ইন করতে, সম্মতি দিতে এবং আপনার অ্যাপের সাথে তাদের প্রোফাইল তথ্য নিরাপদে শেয়ার করতে পারে। অ্যান্ড্রয়েডের ক্রেডেনশিয়াল ম্যানেজার জেটপ্যাক লাইব্রেরি এই ইন্টিগ্রেশনটিকে মসৃণ করে তোলে, একটি একক API ব্যবহার করে অ্যান্ড্রয়েড ডিভাইস জুড়ে সামঞ্জস্যপূর্ণ অভিজ্ঞতা প্রদান করে।
এই দস্তাবেজটি আপনাকে অ্যান্ড্রয়েড অ্যাপ্লিকেশানগুলিতে Google এর সাথে সাইন ইন করার মাধ্যমে, আপনি কীভাবে Google বোতাম UI দিয়ে সাইন ইন সেট আপ করতে পারেন এবং অ্যাপ-অপ্টিমাইজড ওয়ান ট্যাপ সাইন-আপ এবং সাইন-ইন অভিজ্ঞতাগুলি কনফিগার করার মাধ্যমে আপনাকে গাইড করে৷ মসৃণ ডিভাইস মাইগ্রেশনের জন্য, Google এর মাধ্যমে সাইন ইন স্বয়ংক্রিয় সাইন-ইন সমর্থন করে এবং এর ক্রস-প্ল্যাটফর্ম প্রকৃতি Android, iOS এবং ওয়েব সারফেস জুড়ে আপনাকে যেকোনো ডিভাইসে আপনার অ্যাপের জন্য সাইন-ইন অ্যাক্সেস প্রদান করতে সহায়তা করে। আপনি যদি আপনার অ্যাপ্লিকেশনের জন্য Firebase প্রমাণীকরণ ব্যবহার করেন, তাহলে আপনি Google-এর সাথে সাইন ইন ইন্টিগ্রেট করার বিষয়ে আরও জানতে পারবেন এবং ক্রেডেনশিয়াল ম্যানেজার তাদের Android-এ Google-এর সাথে প্রমাণীকরণ করুন ।
Google এর সাথে সাইন ইন সেট আপ করতে, এই দুটি প্রধান পদক্ষেপ অনুসরণ করুন:
ক্রেডেনশিয়াল ম্যানেজারের নীচের শীট UI এর জন্য একটি বিকল্প হিসাবে Google এর সাথে সাইন ইন করুন কনফিগার করুন ৷ ব্যবহারকারীকে সাইন ইন করার জন্য স্বয়ংক্রিয়ভাবে প্রম্পট করার জন্য এটি কনফিগার করা যেতে পারে৷ আপনি যদি পাসকি বা পাসওয়ার্ড প্রয়োগ করে থাকেন তবে আপনি একই সাথে সমস্ত প্রাসঙ্গিক শংসাপত্রের জন্য অনুরোধ করতে পারেন, যাতে ব্যবহারকারীকে সাইন ইন করার জন্য আগে ব্যবহার করা বিকল্পটি মনে রাখতে না হয়৷
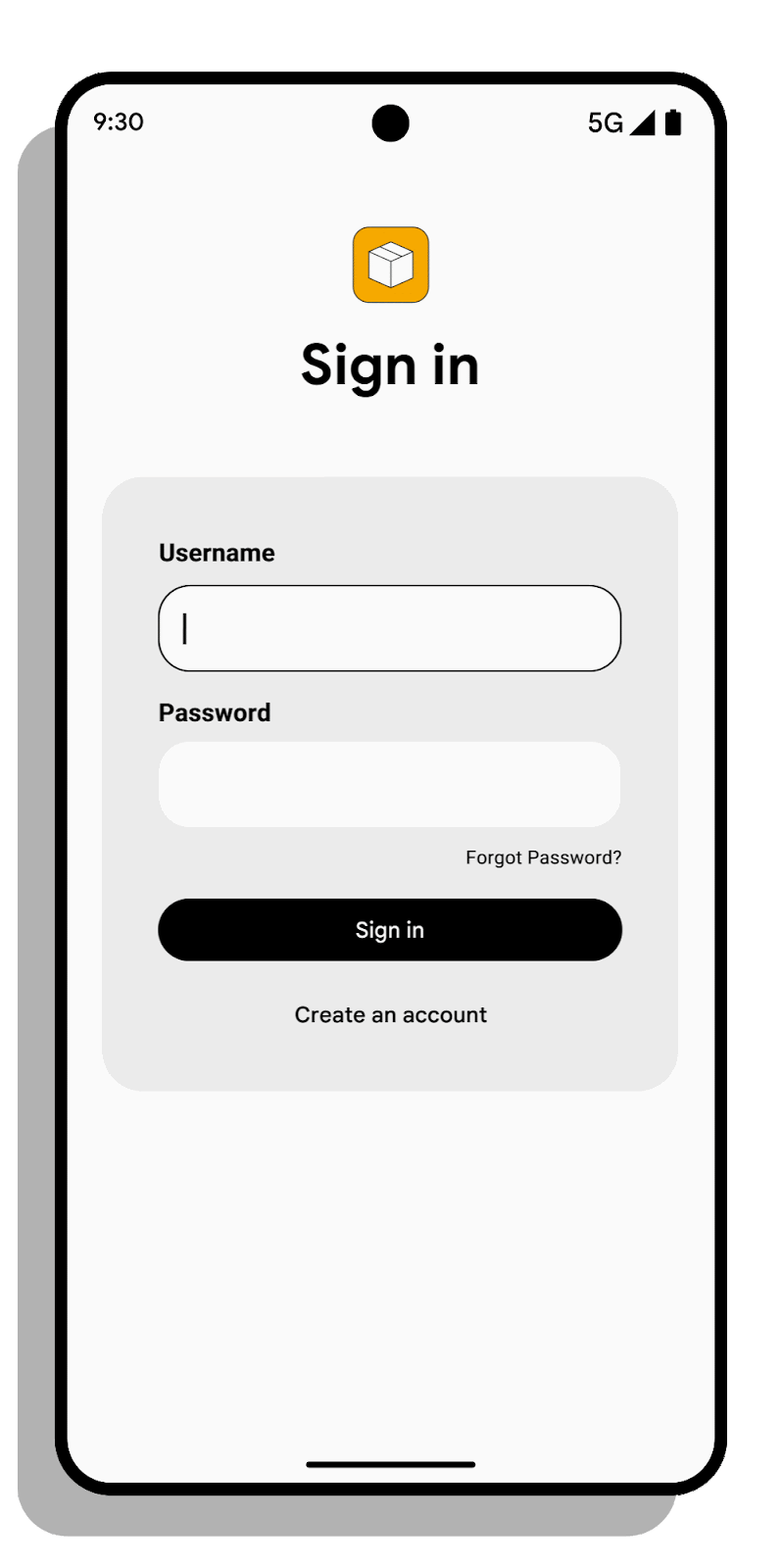
আপনার অ্যাপের UI-তে Google এর সাথে সাইন ইন বোতামটি যোগ করুন । Google এর সাথে সাইন ইন বোতামটি ব্যবহারকারীদের তাদের বিদ্যমান Google অ্যাকাউন্টগুলিকে সাইন আপ করতে বা Android অ্যাপগুলিতে সাইন ইন করার জন্য একটি সুগমিত উপায় অফার করে৷ ব্যবহারকারীরা যদি নীচের শীট UI খারিজ করে দেন, অথবা যদি তারা স্পষ্টভাবে সাইন আপ এবং সাইন ইন করার জন্য তাদের Google অ্যাকাউন্ট ব্যবহার করতে চান তাহলে Google এর সাথে সাইন ইন বোতামে ক্লিক করবেন৷ বিকাশকারীদের জন্য, এর অর্থ হল ব্যবহারকারীর অনবোর্ডিং সহজ এবং সাইন-আপের সময় ঘর্ষণ হ্রাস৷
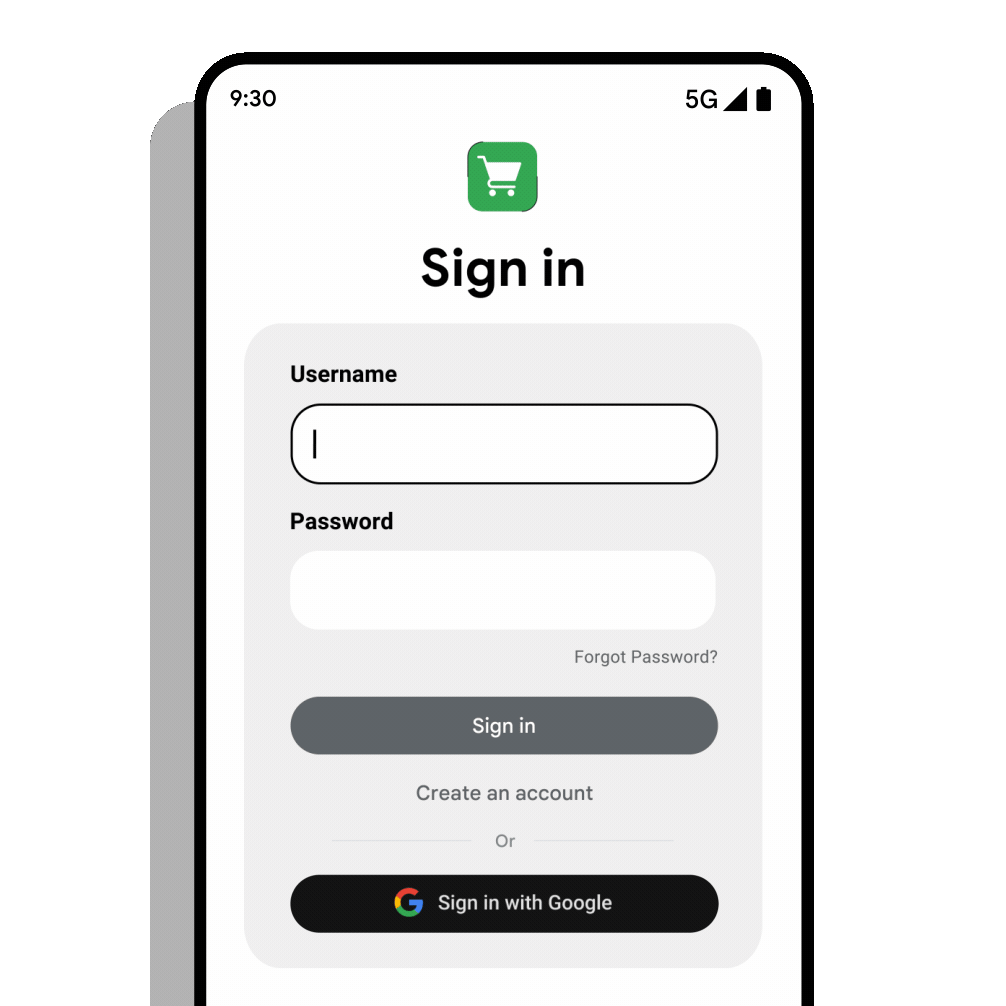
এই নথিটি ব্যাখ্যা করে যে কীভাবে Google আইডি সাহায্যকারী লাইব্রেরি ব্যবহার করে ক্রেডেনশিয়াল ম্যানেজার API-এর সাথে Google বোতাম এবং নীচের শীট ডায়ালগের সাথে সাইন ইনকে একীভূত করতে হয়৷
আপনার সেট আপ প্রকল্প
- আপনার প্রকল্প খুলুন , অথবা আপনার কাছে ইতিমধ্যে একটি না থাকলে একটি প্রকল্প তৈরি করুন৷
- উপর , নিশ্চিত করুন যে সমস্ত তথ্য সম্পূর্ণ এবং সঠিক।
- নিশ্চিত করুন যে আপনার অ্যাপে একটি সঠিক অ্যাপের নাম, অ্যাপ লোগো এবং অ্যাপ হোমপেজ অ্যাসাইন করা আছে। এই মানগুলি সাইন আপে Google-এর সম্মতি দিয়ে সাইন ইন স্ক্রিনে এবং থার্ড-পার্টি অ্যাপ ও পরিষেবার স্ক্রিনে ব্যবহারকারীদের কাছে উপস্থাপন করা হবে।
- নিশ্চিত করুন যে আপনি আপনার অ্যাপের গোপনীয়তা নীতি এবং পরিষেবার শর্তাবলীর URLগুলি নির্দিষ্ট করেছেন৷
- মধ্যে , আপনার অ্যাপের জন্য একটি অ্যান্ড্রয়েড ক্লায়েন্ট আইডি তৈরি করুন যদি আপনার কাছে ইতিমধ্যে একটি না থাকে। আপনাকে আপনার অ্যাপের প্যাকেজের নাম এবং SHA-1 স্বাক্ষর উল্লেখ করতে হবে।
- মধ্যে , একটি নতুন "ওয়েব অ্যাপ্লিকেশন" ক্লায়েন্ট আইডি তৈরি করুন যদি আপনার ইতিমধ্যে না থাকে। আপনি আপাতত "অনুমোদিত জাভাস্ক্রিপ্ট অরিজিনস" এবং "অনুমোদিত রিডাইরেক্ট ইউআরআই" ক্ষেত্রগুলিকে উপেক্ষা করতে পারেন৷ এই ক্লায়েন্ট আইডিটি আপনার ব্যাকএন্ড সার্ভার সনাক্ত করতে ব্যবহার করা হবে যখন এটি Google এর প্রমাণীকরণ পরিষেবাগুলির সাথে যোগাযোগ করবে৷
নির্ভরতা ঘোষণা করুন
আপনার মডিউলের build.gradle ফাইলে, ক্রেডেনশিয়াল ম্যানেজারের সর্বশেষ সংস্করণ ব্যবহার করে নির্ভরতা ঘোষণা করুন:
dependencies {
// ... other dependencies
implementation "androidx.credentials:credentials:<latest version>"
implementation "androidx.credentials:credentials-play-services-auth:<latest version>"
implementation "com.google.android.libraries.identity.googleid:googleid:<latest version>"
}
একটি Google সাইন-ইন অনুরোধ তাত্ক্ষণিক করুন৷
আপনার বাস্তবায়ন শুরু করতে, একটি Google সাইন-ইন অনুরোধ ইনস্ট্যান্টিয়েট করুন । ব্যবহারকারীর Google আইডি টোকেন পুনরুদ্ধার করতে GetGoogleIdOption
ব্যবহার করুন।
val googleIdOption: GetGoogleIdOption = GetGoogleIdOption.Builder()
.setFilterByAuthorizedAccounts(true)
.setServerClientId(WEB_CLIENT_ID)
.setAutoSelectEnabled(true)
.setNonce(<nonce string to use when generating a Google ID token>)
.build()
প্রথমে, ব্যবহারকারীর এমন কোনো অ্যাকাউন্ট আছে কি না যা পূর্বে আপনার অ্যাপে সাইন ইন করার জন্য ব্যবহার করা হয়েছে true
পরীক্ষা করে দেখুন setFilterByAuthorizedAccounts
ব্যবহারকারীরা সাইন ইন করার জন্য উপলব্ধ অ্যাকাউন্টগুলির মধ্যে বেছে নিতে পারেন৷
যদি কোনো অনুমোদিত Google অ্যাকাউন্ট উপলব্ধ না হয়, ব্যবহারকারীকে তাদের উপলব্ধ অ্যাকাউন্টগুলির মধ্যে সাইন আপ করার জন্য অনুরোধ করা উচিত। এটি করার জন্য, API-কে আবার কল করে এবং setFilterByAuthorizedAccounts
false
এ সেট করে ব্যবহারকারীকে প্রম্পট করুন। সাইন আপ সম্পর্কে আরও জানুন ।
ফিরে আসা ব্যবহারকারীদের জন্য স্বয়ংক্রিয় সাইন-ইন সক্ষম করুন (প্রস্তাবিত)
ডেভেলপারদের উচিত স্বয়ংক্রিয় সাইন-ইন সক্ষম করা ব্যবহারকারীদের জন্য যারা তাদের একক অ্যাকাউন্টের সাথে নিবন্ধন করে। এটি ডিভাইস জুড়ে একটি নির্বিঘ্ন অভিজ্ঞতা প্রদান করে, বিশেষ করে ডিভাইস মাইগ্রেশনের সময়, যেখানে ব্যবহারকারীরা শংসাপত্রগুলি পুনরায় প্রবেশ না করে দ্রুত তাদের অ্যাকাউন্টে অ্যাক্সেস পুনরুদ্ধার করতে পারে। আপনার ব্যবহারকারীদের জন্য, এটি অপ্রয়োজনীয় ঘর্ষণকে সরিয়ে দেয় যখন তারা আগে থেকেই সাইন ইন ছিল।
স্বয়ংক্রিয় সাইন-ইন সক্ষম করতে, setAutoSelectEnabled(true)
ব্যবহার করুন। স্বয়ংক্রিয় সাইন ইন শুধুমাত্র তখনই সম্ভব যখন নিম্নলিখিত মানদণ্ডগুলি পূরণ করা হয়:
- অনুরোধের সাথে মিলে একটি একক শংসাপত্র আছে, যা হতে পারে একটি Google অ্যাকাউন্ট বা একটি পাসওয়ার্ড, এবং এই শংসাপত্রটি Android-চালিত ডিভাইসে ডিফল্ট অ্যাকাউন্টের সাথে মেলে৷
- ব্যবহারকারী স্পষ্টভাবে সাইন আউট করেননি।
- ব্যবহারকারী তাদের Google অ্যাকাউন্ট সেটিংসে স্বয়ংক্রিয় সাইন-ইন অক্ষম করেনি৷
val googleIdOption: GetGoogleIdOption = GetGoogleIdOption.Builder()
.setFilterByAuthorizedAccounts(true)
.setServerClientId(WEB_CLIENT_ID)
.setAutoSelectEnabled(true)
.setNonce(<nonce string to use when generating a Google ID token>)
.build()
স্বয়ংক্রিয় সাইন-ইন প্রয়োগ করার সময় সঠিকভাবে সাইন-আউট পরিচালনা করতে মনে রাখবেন, যাতে ব্যবহারকারীরা আপনার অ্যাপ থেকে স্পষ্টভাবে সাইন আউট করার পরে সর্বদা সঠিক অ্যাকাউন্ট বেছে নিতে পারেন।
নিরাপত্তা উন্নত করতে একটি নন্স সেট করুন
সাইন-ইন নিরাপত্তা উন্নত করতে এবং রিপ্লে আক্রমণ এড়াতে, প্রতিটি অনুরোধে একটি নন্স অন্তর্ভুক্ত করতে setNonce
যোগ করুন। একটি ননস তৈরি করা সম্পর্কে আরও জানুন ।
val googleIdOption: GetGoogleIdOption = GetGoogleIdOption.Builder()
.setFilterByAuthorizedAccounts(true)
.setServerClientId(WEB_CLIENT_ID)
.setAutoSelectEnabled(true)
.setNonce(<nonce string to use when generating a Google ID token>)
.build()
Google ফ্লো দিয়ে সাইন ইন তৈরি করুন
Google ফ্লো দিয়ে সাইন ইন সেট আপ করার ধাপগুলি নিম্নরূপ:
- একটি
GetCredentialRequest
ইনস্ট্যান্টিয়েট করুন, তারপর শংসাপত্রগুলি পুনরুদ্ধার করতেaddCredentialOption()
ব্যবহার করে পূর্বে তৈরি করাgoogleIdOption
যোগ করুন। - ব্যবহারকারীর উপলব্ধ শংসাপত্রগুলি পুনরুদ্ধার করতে
getCredential()
(Kotlin) বাgetCredentialAsync()
(Java) কলে এই অনুরোধটি পাস করুন৷ - একবার API সফল হলে,
CustomCredential
বের করুন যাGoogleIdTokenCredential
ডেটার ফলাফল ধারণ করে। -
CustomCredential
এর ধরনGoogleIdTokenCredential.TYPE_GOOGLE_ID_TOKEN_CREDENTIAL
এর মানের সমান হওয়া উচিত।TYPE_GOOGLE_ID_TOKEN_CREDENTIAL।GoogleIdTokenCredential.createFrom
পদ্ধতি ব্যবহার করে বস্তুটিকে একটিGoogleIdTokenCredential
এ রূপান্তর করুন। রূপান্তর সফল হলে,
GoogleIdTokenCredential
ID বের করুন, এটি যাচাই করুন এবং আপনার সার্ভারে প্রমাণপত্রাদি প্রমাণীকরণ করুন।যদি রূপান্তরটি
GoogleIdTokenParsingException
সাথে ব্যর্থ হয়, তাহলে আপনাকে Google লাইব্রেরি সংস্করণের সাথে আপনার সাইন ইন আপডেট করতে হতে পারে৷কোনো অচেনা কাস্টম শংসাপত্র ধরন ধরুন.
val request: GetCredentialRequest = Builder()
.addCredentialOption(googleIdOption)
.build()
coroutineScope.launch {
try {
val result = credentialManager.getCredential(
request = request,
context = activityContext,
)
handleSignIn(result)
} catch (e: GetCredentialException) {
handleFailure(e)
}
}
fun handleSignIn(result: GetCredentialResponse) {
// Handle the successfully returned credential.
val credential = result.credential
when (credential) {
// Passkey credential
is PublicKeyCredential -> {
// Share responseJson such as a GetCredentialResponse on your server to
// validate and authenticate
responseJson = credential.authenticationResponseJson
}
// Password credential
is PasswordCredential -> {
// Send ID and password to your server to validate and authenticate.
val username = credential.id
val password = credential.password
}
// GoogleIdToken credential
is CustomCredential -> {
if (credential.type == GoogleIdTokenCredential.TYPE_GOOGLE_ID_TOKEN_CREDENTIAL) {
try {
// Use googleIdTokenCredential and extract the ID to validate and
// authenticate on your server.
val googleIdTokenCredential = GoogleIdTokenCredential
.createFrom(credential.data)
// You can use the members of googleIdTokenCredential directly for UX
// purposes, but don't use them to store or control access to user
// data. For that you first need to validate the token:
// pass googleIdTokenCredential.getIdToken() to the backend server.
GoogleIdTokenVerifier verifier = ... // see validation instructions
GoogleIdToken idToken = verifier.verify(idTokenString);
// To get a stable account identifier (e.g. for storing user data),
// use the subject ID:
idToken.getPayload().getSubject()
} catch (e: GoogleIdTokenParsingException) {
Log.e(TAG, "Received an invalid google id token response", e)
}
} else {
// Catch any unrecognized custom credential type here.
Log.e(TAG, "Unexpected type of credential")
}
}
else -> {
// Catch any unrecognized credential type here.
Log.e(TAG, "Unexpected type of credential")
}
}
}
Google বোতাম ফ্লো দিয়ে একটি সাইন ইন ট্রিগার করুন
Google বোতাম ফ্লো দিয়ে সাইন ইন ট্রিগার করতে, GetGoogleIdOption
এর পরিবর্তে GetSignInWithGoogleOption
ব্যবহার করুন:
val signInWithGoogleOption: GetSignInWithGoogleOption = GetSignInWithGoogleOption.Builder()
.setServerClientId(WEB_CLIENT_ID)
.setNonce(<nonce string to use when generating a Google ID token>)
.build()
নিম্নোক্ত কোড উদাহরণে বর্ণিত GoogleIdTokenCredential
ফেরত পরিচালনা করুন।
fun handleSignIn(result: GetCredentialResponse) {
// Handle the successfully returned credential.
val credential = result.credential
when (credential) {
is CustomCredential -> {
if (credential.type == GoogleIdTokenCredential.TYPE_GOOGLE_ID_TOKEN_CREDENTIAL) {
try {
// Use googleIdTokenCredential and extract id to validate and
// authenticate on your server.
val googleIdTokenCredential = GoogleIdTokenCredential
.createFrom(credential.data)
} catch (e: GoogleIdTokenParsingException) {
Log.e(TAG, "Received an invalid google id token response", e)
}
}
else -> {
// Catch any unrecognized credential type here.
Log.e(TAG, "Unexpected type of credential")
}
}
else -> {
// Catch any unrecognized credential type here.
Log.e(TAG, "Unexpected type of credential")
}
}
}
একবার আপনি Google সাইন ইন অনুরোধটি ইনস্ট্যান্টিশিয়েট করলে, Google এর সাথে সাইন ইন বিভাগে উল্লিখিত অনুরূপভাবে প্রমাণীকরণ প্রবাহ চালু করুন।
নতুন ব্যবহারকারীদের জন্য সাইন আপ সক্ষম করুন (প্রস্তাবিত)
Google-এর মাধ্যমে সাইন ইন করা হল ব্যবহারকারীদের জন্য আপনার অ্যাপ বা পরিষেবা দিয়ে একটি নতুন অ্যাকাউন্ট তৈরি করার সবচেয়ে সহজ উপায় মাত্র কয়েকটি ট্যাপে৷
যদি কোনো সংরক্ষিত শংসাপত্র পাওয়া না যায় ( getGoogleIdOption
দ্বারা কোনো Google অ্যাকাউন্ট ফেরত দেওয়া হয় না), আপনার ব্যবহারকারীকে সাইন আপ করতে অনুরোধ করুন। আগে, আগে ব্যবহার করা অ্যাকাউন্ট আছে কিনা তা দেখতে setFilterByAuthorizedAccounts(true)
কিনা তা পরীক্ষা করুন। যদি কোনটি পাওয়া না যায়, ব্যবহারকারীকে setFilterByAuthorizedAccounts(false)
উদাহরণ:
val googleIdOption: GetGoogleIdOption = GetGoogleIdOption.Builder()
.setFilterByAuthorizedAccounts(false)
.setServerClientId(WEB_CLIENT_ID)
.build()
একবার আপনি Google সাইন আপ অনুরোধটি ইনস্ট্যান্টিশিয়েট করলে, প্রমাণীকরণ প্রবাহ চালু করুন। ব্যবহারকারীরা সাইন আপের জন্য Google এর সাথে সাইন ইন ব্যবহার করতে না চাইলে, স্বয়ংক্রিয়ভাবে পূরণের জন্য আপনার অ্যাপটি অপ্টিমাইজ করার কথা বিবেচনা করুন৷ একবার আপনার ব্যবহারকারী একটি অ্যাকাউন্ট তৈরি করে ফেললে, অ্যাকাউন্ট তৈরির চূড়ান্ত পদক্ষেপ হিসাবে পাসকিগুলিতে তাদের নথিভুক্ত করার কথা বিবেচনা করুন।
সাইন-আউট পরিচালনা করুন
যখন একজন ব্যবহারকারী আপনার অ্যাপ থেকে সাইন আউট করেন, তখন সমস্ত শংসাপত্র প্রদানকারী থেকে বর্তমান ব্যবহারকারীর শংসাপত্রের অবস্থা সাফ করতে API clearCredentialState()
পদ্ধতিতে কল করুন। এটি সমস্ত শংসাপত্র প্রদানকারীকে অবহিত করবে যে প্রদত্ত অ্যাপের জন্য যেকোন সঞ্চিত শংসাপত্রের অধিবেশন সাফ করা উচিত।
একটি শংসাপত্র প্রদানকারী একটি সক্রিয় শংসাপত্রের অধিবেশন সঞ্চয় করে থাকতে পারে এবং ভবিষ্যতে প্রাপ্ত-শংসাপত্র কলগুলির জন্য সাইন-ইন বিকল্পগুলি সীমিত করতে এটি ব্যবহার করতে পারে৷ উদাহরণস্বরূপ, এটি অন্য কোনো উপলব্ধ শংসাপত্রের চেয়ে সক্রিয় শংসাপত্রকে অগ্রাধিকার দিতে পারে। যখন আপনার ব্যবহারকারী স্পষ্টভাবে আপনার অ্যাপ থেকে সাইন আউট করে এবং পরের বার সামগ্রিক সাইন-ইন বিকল্পগুলি পেতে, প্রদানকারীকে যেকোন সঞ্চিত শংসাপত্রের অধিবেশন সাফ করার জন্য আপনাকে এই API কল করতে হবে।