텍스트의 모양을 문자별로 애니메이션 처리하여 타자기에서 생성하는 것과 유사한 스트리밍 입력 효과를 줄 수 있습니다.
버전 호환성
이 구현을 사용하려면 프로젝트 minSDK를 API 수준 21 이상으로 설정해야 합니다.
종속 항목
텍스트 문자별로 애니메이션 처리
이 코드는 텍스트를 문자별로 애니메이션 처리합니다. 색인을 추적하여 텍스트가 표시되는 정도를 제어합니다. 표시된 텍스트는 현재 색인까지의 문자만 표시되도록 동적으로 업데이트됩니다. 마지막으로 변수가 변경되면 애니메이션이 실행됩니다.
@Composable private fun AnimatedText() { val text = "This text animates as though it is being typed \uD83E\uDDDE\u200D♀\uFE0F \uD83D\uDD10 \uD83D\uDC69\u200D❤\uFE0F\u200D\uD83D\uDC68 \uD83D\uDC74\uD83C\uDFFD" // Use BreakIterator as it correctly iterates over characters regardless of how they are // stored, for example, some emojis are made up of multiple characters. // You don't want to break up an emoji as it animates, so using BreakIterator will ensure // this is correctly handled! val breakIterator = remember(text) { BreakIterator.getCharacterInstance() } // Define how many milliseconds between each character should pause for. This will create the // illusion of an animation, as we delay the job after each character is iterated on. val typingDelayInMs = 50L var substringText by remember { mutableStateOf("") } LaunchedEffect(text) { // Initial start delay of the typing animation delay(1000) breakIterator.text = StringCharacterIterator(text) var nextIndex = breakIterator.next() // Iterate over the string, by index boundary while (nextIndex != BreakIterator.DONE) { substringText = text.subSequence(0, nextIndex).toString() // Go to the next logical character boundary nextIndex = breakIterator.next() delay(typingDelayInMs) } } Text(substringText)
코드 관련 핵심 사항
BreakIterator
는 문자가 저장된 방식과 관계없이 문자를 올바르게 반복합니다. 예를 들어 애니메이션 이모티콘은 여러 문자로 구성됩니다.BreakIterator
는 애니메이션이 중단되지 않도록 단일 문자로 처리되도록 합니다.LaunchedEffect
는 코루틴을 시작하여 문자 간에 지연을 적용합니다. 코드 블록을 클릭 리스너 또는 다른 이벤트로 대체하여 애니메이션을 트리거할 수 있습니다.Text
컴포저블은substringText
값이 업데이트될 때마다 다시 렌더링됩니다.
결과
이 가이드가 포함된 컬렉션
이 가이드는 더 광범위한 Android 개발 목표를 다루는 선별된 빠른 가이드 모음의 일부입니다.
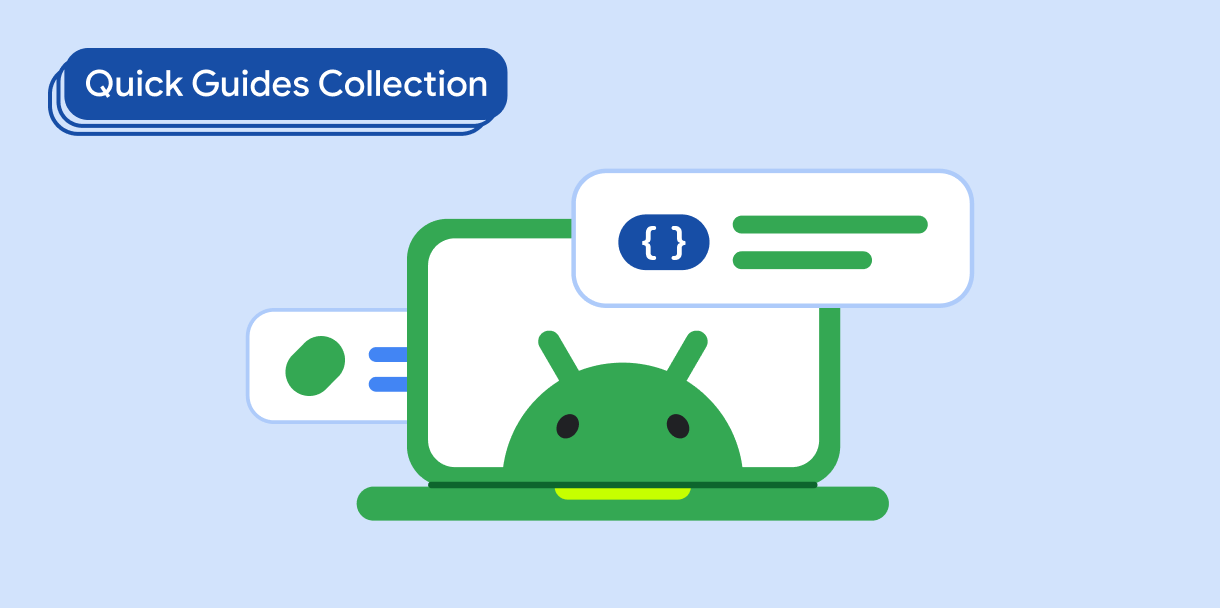
표시 텍스트
텍스트는 모든 UI의 핵심 요소입니다. 만족도 높은 사용자 환경을 제공하기 위해 앱에서 텍스트를 표시하는 다양한 방법을 알아보세요.
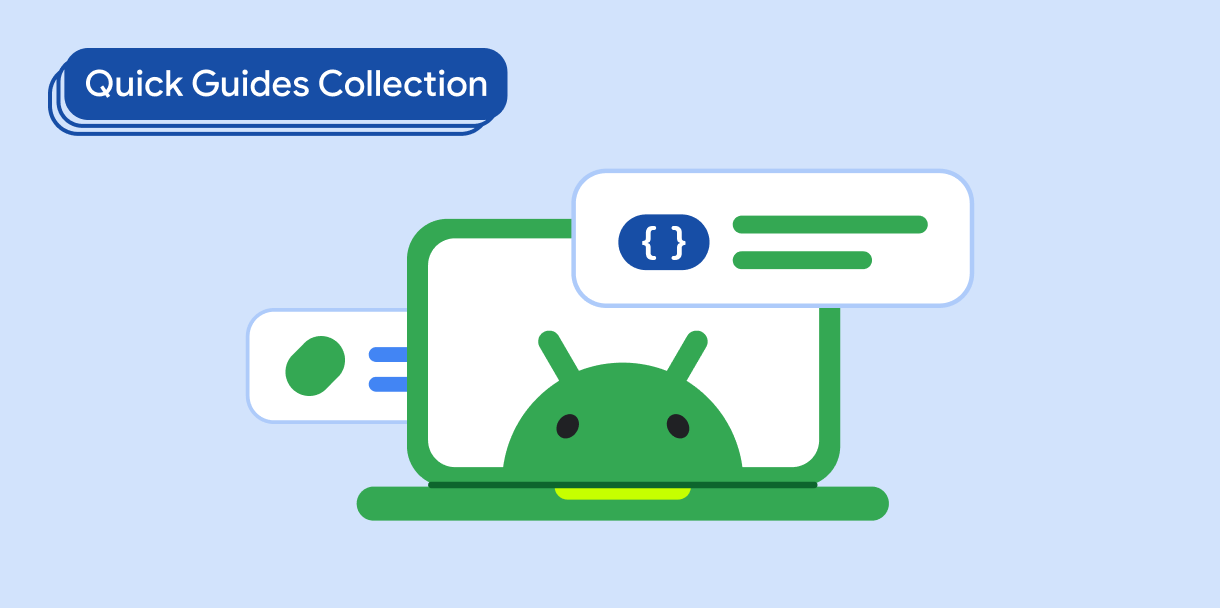
Compose 기초 (동영상 모음)
이 동영상 시리즈에서는 다양한 Compose API를 소개하고 사용 가능한 API와 사용 방법을 빠르게 보여줍니다.
질문이나 의견이 있으신가요?
자주 묻는 질문(FAQ) 페이지로 이동하여 빠른 가이드를 알아보거나 문의하여 의견을 보내주세요.