من خلال القوائم، يمكن للمستخدمين اختيار سلعة من بين مجموعة من الخيارات بسهولة على أجهزة Wear OS.
تتضمّن "مكتبة واجهة المستخدم القابلة للارتداء" فئة
WearableRecyclerView
، وهي فئة من تطبيقات
RecyclerView
تهدف إلى إنشاء قوائم محسَّنة للأجهزة القابلة للارتداء. يمكنك استخدام هذه
الواجهة في تطبيقك القابل للارتداء عن طريق إنشاء حاوية WearableRecyclerView
جديدة.
يمكنك استخدام WearableRecyclerView
للحصول على قائمة طويلة من العناصر البسيطة، مثل مشغّل التطبيقات أو قائمة جهات الاتصال. وقد يحتوي كل عنصر
على سلسلة قصيرة ورمز مرتبط بها. وبدلاً من ذلك، يمكن أن يحتوي كل عنصر على سلسلة أو رمز فقط.
ملاحظة: تجنَّب التصاميم المعقدة. ويجب ألا يحتاج المستخدمون إلا إلى إلقاء نظرة سريعة على العنصر لفهمه، لا سيما إذا كان حجم الشاشة المحدود للأجهزة القابلة للارتداء.
من خلال توسيع فئة RecyclerView
الحالية، تعرض واجهات برمجة التطبيقات WearableRecyclerView
تلقائيًا قائمة بالعناصر قابلة للتمرير عموديًا في قائمة مستقيمة. يمكنك أيضًا استخدام
واجهات برمجة التطبيقات WearableRecyclerView
لتفعيل التصميم المنحني
وإيماءة التمرير الدائري
في تطبيقاتك القابلة للارتداء.
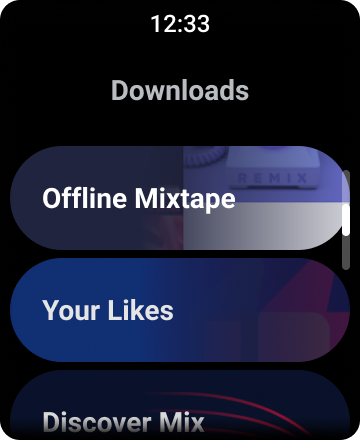
الشكل 1. عرض القائمة التلقائي على نظام التشغيل Wear OS
يشرح لك هذا الدليل كيفية استخدام الصف WearableRecyclerView
لإنشاء
قوائم في تطبيقات Wear OS، وكيفية تفعيل التنسيق المنحني
للعناصر القابلة للتمرير، وكيفية تخصيص مظهر
الأطفال أثناء التمرير.
إضافة WearableRecyclerView إلى نشاط باستخدام تنسيق XML
يضيف التنسيق التالي WearableRecyclerView
إلى نشاط:
<androidx.wear.widget.WearableRecyclerView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/recycler_launcher_view" android:layout_width="match_parent" android:layout_height="match_parent" android:scrollbars="vertical" />
يوضّح المثال التالي سمة WearableRecyclerView
التي تم تطبيقها على نشاط:
Kotlin
class MainActivity : Activity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) } ... }
Java
public class MainActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } ... }
إنشاء تصميم مقوّس
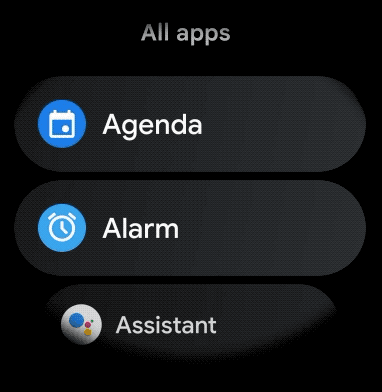
لإنشاء تصميم منحني للعناصر القابلة للتمرير في تطبيقك القابل للارتداء، عليك إجراء ما يلي:
-
استخدِم
WearableRecyclerView
كحاويتك الرئيسية في تنسيق XML المناسب. -
اضبط طريقة
setEdgeItemsCenteringEnabled(boolean)
علىtrue
. يؤدي هذا الإجراء إلى توسيط العنصرَين الأول والأخير عموديًا في القائمة التي تظهر على الشاشة. -
استخدِم الطريقة
WearableRecyclerView.setLayoutManager()
لضبط تنسيق العناصر التي تظهر على الشاشة.
Kotlin
wearableRecyclerView.apply { // To align the edge children (first and last) with the center of the screen. isEdgeItemsCenteringEnabled = true ... layoutManager = WearableLinearLayoutManager(this@MainActivity) }
Java
// To align the edge children (first and last) with the center of the screen. wearableRecyclerView.setEdgeItemsCenteringEnabled(true); ... wearableRecyclerView.setLayoutManager( new WearableLinearLayoutManager(this));
إذا كان لتطبيقك متطلبات محدّدة لتخصيص مظهر الأطفال أثناء التنقّل، مثلاً،
تغيير حجم الرموز والنص أثناء تمرير العناصر بعيدًا عن المركز، يمكنك توسيع فئة
WearableLinearLayoutManager.LayoutCallback
وإلغاء طريقة
onLayoutFinished
.
تعرض مقتطفات الرمز التالية مثالاً على تخصيص تمرير العناصر لتوسيع نطاقه بعيدًا عن المركز من خلال توسيع فئة WearableLinearLayoutManager.LayoutCallback
:
Kotlin
/** How much icons should scale, at most. */ private const val MAX_ICON_PROGRESS = 0.65f class CustomScrollingLayoutCallback : WearableLinearLayoutManager.LayoutCallback() { private var progressToCenter: Float = 0f override fun onLayoutFinished(child: View, parent: RecyclerView) { child.apply { // Figure out % progress from top to bottom. val centerOffset = height.toFloat() / 2.0f / parent.height.toFloat() val yRelativeToCenterOffset = y / parent.height + centerOffset // Normalize for center. progressToCenter = Math.abs(0.5f - yRelativeToCenterOffset) // Adjust to the maximum scale. progressToCenter = Math.min(progressToCenter, MAX_ICON_PROGRESS) scaleX = 1 - progressToCenter scaleY = 1 - progressToCenter } } }
Java
public class CustomScrollingLayoutCallback extends WearableLinearLayoutManager.LayoutCallback { /** How much icons should scale, at most. */ private static final float MAX_ICON_PROGRESS = 0.65f; private float progressToCenter; @Override public void onLayoutFinished(View child, RecyclerView parent) { // Figure out % progress from top to bottom. float centerOffset = ((float) child.getHeight() / 2.0f) / (float) parent.getHeight(); float yRelativeToCenterOffset = (child.getY() / parent.getHeight()) + centerOffset; // Normalize for center. progressToCenter = Math.abs(0.5f - yRelativeToCenterOffset); // Adjust to the maximum scale. progressToCenter = Math.min(progressToCenter, MAX_ICON_PROGRESS); child.setScaleX(1 - progressToCenter); child.setScaleY(1 - progressToCenter); } }
Kotlin
wearableRecyclerView.layoutManager = WearableLinearLayoutManager(this, CustomScrollingLayoutCallback())
Java
CustomScrollingLayoutCallback customScrollingLayoutCallback = new CustomScrollingLayoutCallback(); wearableRecyclerView.setLayoutManager( new WearableLinearLayoutManager(this, customScrollingLayoutCallback));