让对象按照预设程序(例如旋转的三角形)移动有助于
但如果您想让用户与您的 OpenGL ES 图形交互,该怎么办呢?
使 OpenGL ES 应用触摸可交互的关键在于扩展
GLSurfaceView
,用于替换
onTouchEvent()
,用于监听触摸事件。
本课程介绍了如何监听触摸事件,以使用户可以旋转 OpenGL ES 对象。
设置触摸监听器
为了使您的 OpenGL ES 应用响应触摸事件,您必须实现
onTouchEvent()
方法
GLSurfaceView
类。以下示例实现展示了如何监听
MotionEvent.ACTION_MOVE
事件并将其翻译成
形状的旋转角度
Kotlin
private const val TOUCH_SCALE_FACTOR: Float = 180.0f / 320f ... private var previousX: Float = 0f private var previousY: Float = 0f override fun onTouchEvent(e: MotionEvent): Boolean { // MotionEvent reports input details from the touch screen // and other input controls. In this case, you are only // interested in events where the touch position changed. val x: Float = e.x val y: Float = e.y when (e.action) { MotionEvent.ACTION_MOVE -> { var dx: Float = x - previousX var dy: Float = y - previousY // reverse direction of rotation above the mid-line if (y > height / 2) { dx *= -1 } // reverse direction of rotation to left of the mid-line if (x < width / 2) { dy *= -1 } renderer.angle += (dx + dy) * TOUCH_SCALE_FACTOR requestRender() } } previousX = x previousY = y return true }
Java
private final float TOUCH_SCALE_FACTOR = 180.0f / 320; private float previousX; private float previousY; @Override public boolean onTouchEvent(MotionEvent e) { // MotionEvent reports input details from the touch screen // and other input controls. In this case, you are only // interested in events where the touch position changed. float x = e.getX(); float y = e.getY(); switch (e.getAction()) { case MotionEvent.ACTION_MOVE: float dx = x - previousX; float dy = y - previousY; // reverse direction of rotation above the mid-line if (y > getHeight() / 2) { dx = dx * -1 ; } // reverse direction of rotation to left of the mid-line if (x < getWidth() / 2) { dy = dy * -1 ; } renderer.setAngle( renderer.getAngle() + ((dx + dy) * TOUCH_SCALE_FACTOR)); requestRender(); } previousX = x; previousY = y; return true; }
请注意,在计算旋转角度后,此方法会调用
requestRender()
告诉
渲染程序时该渲染帧了在这个示例中,这是最高效的
因为除非旋转角度发生变化,否则无需重新绘制帧。不过,
不会对效率产生任何影响
使用 setRenderMode()
更改数据
方法,因此请确保在渲染程序中取消注释以下行:
Kotlin
class MyGlSurfaceView(context: Context) : GLSurfaceView(context) { init { // Render the view only when there is a change in the drawing data renderMode = GLSurfaceView.RENDERMODE_WHEN_DIRTY } }
Java
public MyGLSurfaceView(Context context) { ... // Render the view only when there is a change in the drawing data setRenderMode(GLSurfaceView.RENDERMODE_WHEN_DIRTY); }
公开旋转角度
上面的示例代码要求您通过以下方式通过渲染程序公开旋转角度:
添加公开成员。由于渲染程序代码在独立于主用户的线程上运行
接口线程,则必须将此公共变量声明为 volatile
。
以下是用于声明变量和公开 getter 和 setter 对的代码:
Kotlin
class MyGLRenderer4 : GLSurfaceView.Renderer { @Volatile var angle: Float = 0f }
Java
public class MyGLRenderer implements GLSurfaceView.Renderer { ... public volatile float mAngle; public float getAngle() { return mAngle; } public void setAngle(float angle) { mAngle = angle; } }
应用旋转
如需应用触控输入生成的旋转,请注释掉生成角度的代码,并 添加一个变量,该变量包含触控输入生成的角度:
Kotlin
override fun onDrawFrame(gl: GL10) { ... val scratch = FloatArray(16) // Create a rotation for the triangle // long time = SystemClock.uptimeMillis() % 4000L; // float angle = 0.090f * ((int) time); Matrix.setRotateM(rotationMatrix, 0, angle, 0f, 0f, -1.0f) // Combine the rotation matrix with the projection and camera view // Note that the mvpMatrix factor *must be first* in order // for the matrix multiplication product to be correct. Matrix.multiplyMM(scratch, 0, mvpMatrix, 0, rotationMatrix, 0) // Draw triangle triangle.draw(scratch) }
Java
public void onDrawFrame(GL10 gl) { ... float[] scratch = new float[16]; // Create a rotation for the triangle // long time = SystemClock.uptimeMillis() % 4000L; // float angle = 0.090f * ((int) time); Matrix.setRotateM(rotationMatrix, 0, mAngle, 0, 0, -1.0f); // Combine the rotation matrix with the projection and camera view // Note that the vPMatrix factor *must be first* in order // for the matrix multiplication product to be correct. Matrix.multiplyMM(scratch, 0, vPMatrix, 0, rotationMatrix, 0); // Draw triangle mTriangle.draw(scratch); }
完成上述步骤后,运行程序并将手指拖动到 屏幕旋转三角形:
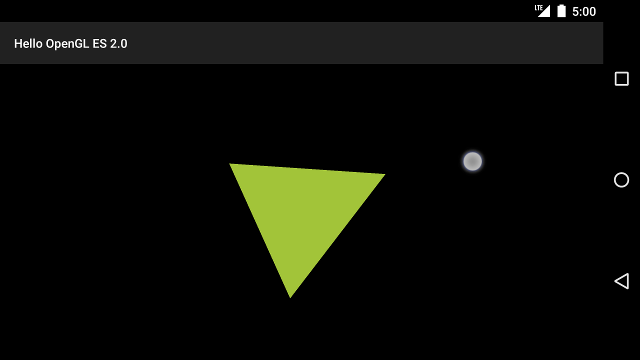
图 1. 使用触控输入旋转的三角形(圆圈表示触摸) 位置)。