在 Android 12.0(API 级别 31)及更高版本中,系统提供
CallStyle
通知模板,用于区分来电通知和
其他类型的通知使用此模板创建传入或
进行中的通话通知。该模板支持大幅面通知
包含来电者信息和所需操作(例如接听或
拒接来电。
由于来电和正在进行的通话属于高优先级活动,因此这些通知 在通知栏中收到最高优先级通知。这种排名还会使 用于将优先级优先的呼叫转接到其他设备。
CallStyle
通知模板包含以下必需操作:
- 接听或拒接来电。
- 挂断正在进行通话。
- 接听或挂断以进行来电过滤。
此样式的操作显示为按钮,系统会自动添加 适当的图标和文字。不支持手动标记按钮。 如需详细了解通知设计原则,请参阅 通知。
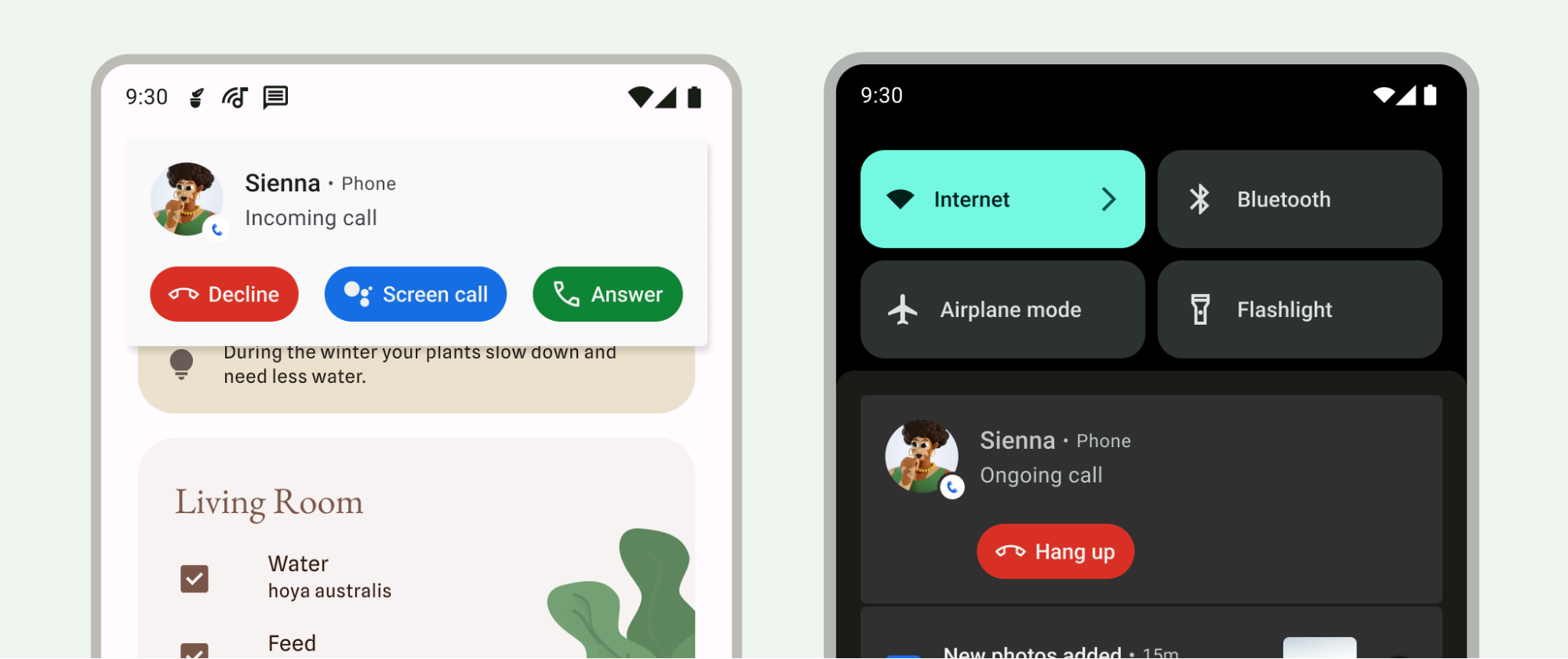
所需操作以 intent 形式传递,例如 hangupIntent
和
answerIntent
。以上每个参数都是对
由系统维护的令牌令牌是一个轻量级对象,
可在不同的应用和进程之间传递系统
负责管理令牌的生命周期并确保
即使创建 PendingIntent
的应用不再
。如果您向另一个应用提供 PendingIntent
,
拥有执行指定操作(例如拒绝或接听)的权限。
即使创建 intent 的应用也会授予此权限
目前没有运行。如需了解详情,请参阅参考文档。
(针对PendingIntent
)。
从 Android 14(API 级别 34)开始,您可以配置来电通知
不可关闭。为此,请结合使用 CallStyle
通知和
Notification.FLAG_ONGOING_EVENT
到
Notification.Builder#setOngoing(true)
。
以下是将各种方法与 CallStyle
搭配使用的示例
通知。
Kotlin
// Create a new call, setting the user as the caller. val incomingCaller = Person.Builder() .setName("Jane Doe") .setImportant(true) .build()
Java
// Create a new call with the user as the caller. Person incomingCaller = new Person.Builder() .setName("Jane Doe") .setImportant(true) .build();
来电
使用 forIncomingCall()
方法为
来电。
Kotlin
// Create a call style notification for an incoming call. val builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forIncomingCall(caller, declineIntent, answerIntent)) .addPerson(incomingCaller)
Java
// Create a call style notification for an incoming call. Notification.Builder builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forIncomingCall(caller, declineIntent, answerIntent)) .addPerson(incomingCaller);
当前通话
使用 forOngoingCall()
方法为
通话中。
Kotlin
// Create a call style notification for an ongoing call. val builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forOngoingCall(caller, hangupIntent)) .addPerson(second_caller)
Java
// Create a call style notification for an ongoing call. Notification.Builder builder = new Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forOngoingCall(caller, hangupIntent)) .addPerson(second_caller);
过滤来电
使用 forScreeningCall()
方法为以下事件创建通话样式通知:
来电过滤
Kotlin
// Create a call style notification for screening a call. val builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forScreeningCall(caller, hangupIntent, answerIntent)) .addPerson(second_caller)
Java
// Create a call style notification for screening a call. Notification.Builder builder = new Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forScreeningCall(caller, hangupIntent, answerIntent)) .addPerson(second_caller);
与更多 Android 版本兼容
将 API 版本 30 或更低版本的 CallStyle
通知与
前台服务,以便为他们指定在 API 中授予的较高排名
31 或更高级别。此外,API 版本 30 上会有 CallStyle
通知
或更早版本
使用 setColorized()
方法着色。
将 Telecom API 与 CallStyle
通知搭配使用。如需了解详情,请参阅
Telecom 框架概览。