アプリで静止画像を表示する必要がある場合は、Drawable
クラスとそのサブクラスを使用してシェイプを描画し、
作成します。Drawable
は、Pod を
描画できるもの。さまざまなサブクラスが特定の画像処理をサポートする
それらを拡張して独自のドローアブル オブジェクトを定義したり、
異なる動作をします。
クラス コンストラクタを使用する以外に、Drawable
を定義しインスタンス化する方法には次の 2 つがあります。
- プロジェクトに保存されている画像リソース(ビットマップ ファイル)をインフレートする。
- ドローアブル プロパティを定義する XML リソースをインフレートする。
注: 代わりにベクター型ドローアブルを使用することをおすすめします。このドローアブルでは、 点、線、曲線、および関連する色情報。これにより ベクター型ドローアブルや 品質を損なうことなくさまざまなサイズに スケーリングできます詳細については、ベクトル ドローアブルの概要をご覧ください。
リソースの画像からドローアブルを作成する
アプリの画像ファイルを参照して、アプリにグラフィックスを プロジェクト リソースを管理できます。サポートされているファイル形式は、PNG(推奨)、JPG(許容)、 GIF(使用禁止)。アプリのアイコン、ロゴ、その他のグラフィック(使用されているものなど) 使用する場合、この手法は適しています。
画像リソースを使用するには、ファイルを res/drawable/
に追加します。
ディレクトリに配置します。プロジェクトに追加されたら、画像を参照して
XML レイアウトから参照できます。いずれにしても
リソース ID は、ファイル形式の拡張子を除いたファイル名です。対象
たとえば、my_image.png
を my_image
として参照します。
注:
res/drawable/
ディレクトリは、
ビルド中に aapt
ツールによる可逆画像圧縮
プロセスです例: 256 色以上を必要としないトゥルーカラーの PNG
カラーパレットを使用する 8 ビットの PNG に変換できます。画像が生成されます。
同じ画質ですが、必要なメモリは少なくなります。その結果、画像バイナリは
このディレクトリに配置されたディレクトリは、ビルド時に変更される可能性があります。本コースの最初のモジュールは、
ビットマップに変換するには、ファイルを
res/raw/
フォルダ(aapt
ツールが実行されない)
変更できます。
次のコード スニペットは、ImageView
ドローアブル リソースから画像を作成し、レイアウトに追加します。
Kotlin
private lateinit var constraintLayout: ConstraintLayout override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) // Instantiate an ImageView and define its properties val i = ImageView(this).apply { setImageResource(R.drawable.my_image) contentDescription = resources.getString(R.string.my_image_desc) // set the ImageView bounds to match the Drawable's dimensions adjustViewBounds = true layoutParams = ViewGroup.LayoutParams( ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT) } // Create a ConstraintLayout in which to add the ImageView constraintLayout = ConstraintLayout(this).apply { // Add the ImageView to the layout. addView(i) } // Set the layout as the content view. setContentView(constraintLayout) }
Java
ConstraintLayout constraintLayout; protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Create a ConstraintLayout in which to add the ImageView constraintLayout = new ConstraintLayout(this); // Instantiate an ImageView and define its properties ImageView i = new ImageView(this); i.setImageResource(R.drawable.my_image); i.setContentDescription(getResources().getString(R.string.my_image_desc)); // set the ImageView bounds to match the Drawable's dimensions i.setAdjustViewBounds(true); i.setLayoutParams(new ViewGroup.LayoutParams( ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT)); // Add the ImageView to the layout and set the layout as the content view. constraintLayout.addView(i); setContentView(constraintLayout); }
その他に、以下に示すように、画像リソースを Drawable
オブジェクトとして処理することもできます。
例:
Kotlin
val myImage: Drawable = ResourcesCompat.getDrawable(context.resources, R.drawable.my_image, null)
Java
Resources res = context.getResources(); Drawable myImage = ResourcesCompat.getDrawable(res, R.drawable.my_image, null);
警告: プロジェクト内の一意のリソースごとに、
オブジェクトの数に関係なく、1 つの状態しか維持できません。
インスタンスを作成します。たとえば、同じ画像リソースから 2 つの Drawable
オブジェクトをインスタンス化し、
あるオブジェクトのプロパティ(alpha など)を変更すると、
定義します。画像リソースの複数のインスタンスを扱う場合は、
Drawable
オブジェクトを直接変換する場合は、
9 ~ 12 歳の子ども
アニメーション。
次の XML スニペットは、XML レイアウトの ImageView
にドローアブル リソースを追加する方法を示しています。
<ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/my_image" android:contentDescription="@string/my_image_desc" />
プロジェクト リソースの使用方法の詳細については、リソースとアセットをご覧ください。
注: 画像リソースをドローアブルのソースとして使用する場合は、 さまざまなピクセル密度に適した画像サイズになるようにしてください。もし 正しくない画像は収まるように拡大されるため、ドローアブルでアーティファクトが生じることがあります。 詳しくは、 ピクセル密度。
XML リソースからドローアブルを作成する
Drawable
がある場合
オブジェクトを定義します。最初は、変数に
XML で Drawable
を定義することをおすすめします。均等
ユーザーの操作中に Drawable
がプロパティを変更する場合
XML でオブジェクトを定義することをおすすめします。プロパティは XML で定義できます。
オブジェクトがインスタンス化されました
Drawable
を XML で定義したら、ファイルを
プロジェクトの res/drawable/
ディレクトリ。次の例は、トレーニング コードを
は、
TransitionDrawable
このリソースは、Drawable
から継承します。
<!-- res/drawable/expand_collapse.xml --> <transition xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@drawable/image_expand"/> <item android:drawable="@drawable/image_collapse"/> </transition>
次に、API 呼び出しによってオブジェクトを取得し、
Resources#getDrawable()
XML ファイルのリソース ID を渡します。制限なし
Drawable
サブクラス
inflate()
メソッドをサポートする API は、XML で定義してインスタンス化できます。
自動的に最適化されます。
XML インフレーションをサポートする各ドローアブル クラスは、特定の XML 属性を利用します。
オブジェクトのプロパティを定義します。次のコードは、
TransitionDrawable
それをコンテンツの
ImageView
オブジェクト:
Kotlin
val transition= ResourcesCompat.getDrawable( context.resources, R.drawable.expand_collapse, null ) as TransitionDrawable val image: ImageView = findViewById(R.id.toggle_image) image.setImageDrawable(transition) // Description of the initial state that the drawable represents. image.contentDescription = resources.getString(R.string.collapsed) // Then you can call the TransitionDrawable object's methods. transition.startTransition(1000) // After the transition is complete, change the image's content description // to reflect the new state.
Java
Resources res = context.getResources(); TransitionDrawable transition = (TransitionDrawable) ResourcesCompat.getDrawable(res, R.drawable.expand_collapse, null); ImageView image = (ImageView) findViewById(R.id.toggle_image); image.setImageDrawable(transition); // Description of the initial state that the drawable represents. image.setContentDescription(getResources().getString(R.string.collapsed)); // Then you can call the TransitionDrawable object's methods. transition.startTransition(1000); // After the transition is complete, change the image's content description // to reflect the new state.
サポートされている XML 属性の詳細については、このモジュールのコースリソースに記載の 使用できます。
シェイプ ドローアブル
ShapeDrawable
オブジェクトの使用をおすすめします。
を使用すると、2 次元のグラフィックを動的に描画できます。Google Chat では
ShapeDrawable
オブジェクトにプリミティブ シェイプをプログラムで描画する
アプリに必要なスタイルを適用します。
ShapeDrawable
は Drawable
のサブクラスです。このため、
Drawable
が想定される場所で ShapeDrawable
。対象
たとえば、ShapeDrawable
オブジェクトを使用して背景を
ビューを setBackgroundDrawable()
メソッドに渡します。また、シェイプをそのシェイプを
アプリのレイアウトに追加できます。
ShapeDrawable
には独自の draw()
メソッドがあるため、
ShapeDrawable
を描画する View
のサブクラス
これは、onDraw()
イベント中に
次のコード例をご覧ください。
Kotlin
class CustomDrawableView(context: Context) : View(context) { private val drawable: ShapeDrawable = run { val x = 10 val y = 10 val width = 300 val height = 50 contentDescription = context.resources.getString(R.string.my_view_desc) ShapeDrawable(OvalShape()).apply { // If the color isn't set, the shape uses black as the default. paint.color = 0xff74AC23.toInt() // If the bounds aren't set, the shape can't be drawn. setBounds(x, y, x + width, y + height) } } override fun onDraw(canvas: Canvas) { drawable.draw(canvas) } }
Java
public class CustomDrawableView extends View { private ShapeDrawable drawable; public CustomDrawableView(Context context) { super(context); int x = 10; int y = 10; int width = 300; int height = 50; setContentDescription(context.getResources().getString( R.string.my_view_desc)); drawable = new ShapeDrawable(new OvalShape()); // If the color isn't set, the shape uses black as the default. drawable.getPaint().setColor(0xff74AC23); // If the bounds aren't set, the shape can't be drawn. drawable.setBounds(x, y, x + width, y + height); } protected void onDraw(Canvas canvas) { drawable.draw(canvas); } }
コードサンプルの CustomDrawableView
クラスを使用すると、
他のカスタムビューを使用する場合と同じです。たとえば
アプリのアクティビティにプログラムで追加します。コードは次のようになります。
例:
Kotlin
private lateinit var customDrawableView: CustomDrawableView override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) customDrawableView = CustomDrawableView(this) setContentView(customDrawableView) }
Java
CustomDrawableView customDrawableView; protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); customDrawableView = new CustomDrawableView(this); setContentView(customDrawableView); }
代わりに XML レイアウトでカスタムビューを使用する場合は、
CustomDrawableView
クラスは、クラスが呼び出されたときに呼び出される View(Context, AttributeSet)
コンストラクタをオーバーライドする必要があります。
XML からインフレートできます次の例は、Pod を
XML レイアウト内の CustomDrawableView
:
<com.example.shapedrawable.CustomDrawableView android:layout_width="fill_parent" android:layout_height="wrap_content" />
ShapeDrawable
クラス(他の多くのクラスと同様)
android.graphics.drawable
パッケージのドローアブル タイプでは、
public メソッドを使用してオブジェクトのさまざまなプロパティを定義します。例
調整するプロパティには、アルファ透明度、カラーフィルタ、
調整できます
XML リソースを使用して基本的なドローアブル図形を定義することもできます。詳細 詳しくは、をご覧ください。 のシェイプ ドローアブル ドローアブル リソースのタイプ。
NinePatch ドローアブル
NinePatchDrawable
グラフィックは、
ビューの背景として使用できる伸縮可能なビットマップ画像。Android
は、ビューのコンテンツに合わせてグラフィックのサイズを自動的に変更します。「
NinePatch イメージの使用例は、標準の Android で使用される背景
ボタン。ボタンは、さまざまな長さの文字列に対応できるように伸ばさなければなりません。
NinePatch グラフィックは、1 ピクセルの枠線を含む標準の PNG 画像です。
9.png
拡張子を使用して、
プロジェクトの res/drawable/
ディレクトリ。
枠線を使用して、画像の拡大可能な領域と静的な領域を定義します。 伸縮可能セクションを指定するには、1 ピクセル幅を 1 つ(または複数)描画します。 枠線の左側と上部に黒い線(他の枠線ピクセル)がある は完全に透明または白色です)。伸縮可能なセクションをいくつでも できます。伸縮可能なセクションの相対的なサイズは変わらないため、 最も大きいセクションが常に最大となります。
画像のオプションのドローアブル セクションを定義することもできます(実際には、
パディング線など)を、右側に線、下部に線を引きます。もし
View
オブジェクトは、NinePatch グラフィックを背景として設定します。
ビューのテキストを指定すると、すべてのテキストが
右端と下線(存在する場合)で指定された領域のみを占有します。
パディング行が含まれていない場合、Android は左と上の行を使用して、
このドローアブル領域を定義します。
これらの行の違いを明確にするために、左と上の行は、 引き延ばすために複製できる画像のピクセル 説明します。下と右の線は、画像内の相対的な領域を定義します。 ビューのコンテンツを占有できます。
図 1 は、ボタンの定義に使用する NinePatch グラフィックの例を示しています。
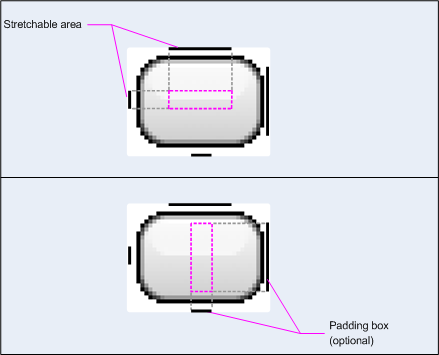
図 1: NinePatch グラフィックの例 ボタンを定義する
この NinePatch グラフィックは、伸縮可能な領域(左と上)を 1 つ定義しています。 下と右の線が描かれたドローアブル領域です。上の図では、 グレーの点線は、複製されたイメージの領域を示します。 画像を引き伸ばします下の画像のピンク色の長方形は、 ビューのコンテンツを許可するリージョン。コンテンツが表示されない場合は、 この領域に収まると、画像が収まるように引き伸ばされます。
[Draw 9-patch] ツールには、 WYSIWYG グラフィックスを使用して NinePatch 画像を簡単に作成できます。 クリックします。さらに、伸縮可能ゾーンに定義したリージョンが 画像領域に、ピクセル レイヤが原因で描画アーティファクトが生じるリスクがある 必要があります。
次のサンプル レイアウト XML は、NinePatch グラフィックの追加方法を示しています。
ボタンを押したままにしますNinePatch 画像は
res/drawable/my_button_background.9.png
。
<Button android:id="@+id/tiny" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:layout_centerInParent="true" android:text="Tiny" android:textSize="8sp" android:background="@drawable/my_button_background"/> <Button android:id="@+id/big" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_centerInParent="true" android:text="Biiiiiiig text!" android:textSize="30sp" android:background="@drawable/my_button_background"/>
layout_width
と layout_height
は
属性が wrap_content
に設定されているため、ボタンがきれいに収まります。
必要があります。
図 2 は、XML と NinePatch の画像からレンダリングされた 2 つのボタンを示しています。 表示されます。ボタンの幅と高さがテキストによって異なることに注目してください。 それに合わせて背景画像が引き伸ばされます
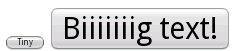
図 2: XML を使用してレンダリングされたボタン リソースと NinePatch グラフィックが含まれています。
カスタム ドローアブル
カスタムの図形描画を作成する場合は、Drawable
クラス(またはそのサブクラス)を拡張します。
実装する最も重要なメソッドは draw(Canvas)
です。
これにより、Terraform で必要な Canvas
オブジェクトが、
描画の指示を与えます。
次のコードは、Drawable
の単純なサブクラスを示しています。
この関数では、次のように円を描画します。
Kotlin
class MyDrawable : Drawable() { private val redPaint: Paint = Paint().apply { setARGB(255, 255, 0, 0) } override fun draw(canvas: Canvas) { // Get the drawable's bounds val width: Int = bounds.width() val height: Int = bounds.height() val radius: Float = Math.min(width, height).toFloat() / 2f // Draw a red circle in the center canvas.drawCircle((width / 2).toFloat(), (height / 2).toFloat(), radius, redPaint) } override fun setAlpha(alpha: Int) { // This method is required } override fun setColorFilter(colorFilter: ColorFilter?) { // This method is required } override fun getOpacity(): Int = // Must be PixelFormat.UNKNOWN, TRANSLUCENT, TRANSPARENT, or OPAQUE PixelFormat.OPAQUE }
Java
public class MyDrawable extends Drawable { private final Paint redPaint; public MyDrawable() { // Set up color and text size redPaint = new Paint(); redPaint.setARGB(255, 255, 0, 0); } @Override public void draw(Canvas canvas) { // Get the drawable's bounds int width = getBounds().width(); int height = getBounds().height(); float radius = Math.min(width, height) / 2; // Draw a red circle in the center canvas.drawCircle(width/2, height/2, radius, redPaint); } @Override public void setAlpha(int alpha) { // This method is required } @Override public void setColorFilter(ColorFilter colorFilter) { // This method is required } @Override public int getOpacity() { // Must be PixelFormat.UNKNOWN, TRANSLUCENT, TRANSPARENT, or OPAQUE return PixelFormat.OPAQUE; } }
その後、ドローアブルを任意の場所(
次に示すように、ImageView
を使用します。
Kotlin
val myDrawing = MyDrawable() val image: ImageView = findViewById(R.id.imageView) image.setImageDrawable(myDrawing) image.contentDescription = resources.getString(R.string.my_image_desc)
Java
MyDrawable mydrawing = new MyDrawable(); ImageView image = findViewById(R.id.imageView); image.setImageDrawable(mydrawing); image.setContentDescription(getResources().getString(R.string.my_image_desc));
Android 7.0(API レベル 24)以降では、カスタム ドローアブルのインスタンスも定義できます。 XML に置き換える必要があります。
- XML 要素名として完全修飾クラス名を使用する。この方法では、カスタムの
ドローアブル クラスはパブリック トップレベル クラスである必要があります。
<com.myapp.MyDrawable xmlns:android="http://schemas.android.com/apk/res/android" android:color="#ffff0000" />
- XML タグ名として
drawable
を使用し、完全修飾クラスを指定する 指定します。この方法は、パブリック トップレベル クラスと public 静的内部クラス:<drawable xmlns:android="http://schemas.android.com/apk/res/android" class="com.myapp.MyTopLevelClass$MyDrawable" android:color="#ffff0000" />
ドローアブルに色合いを追加する
Android 5.0(API レベル 21)以降では、次のように定義されたビットマップと 9-patch の色合いを調整できます。
使用できます。カラーリソースまたはカラーに解決されるテーマ属性で色合いを調整できます。
リソース(例: ?android:attr/colorPrimary
)通常これらのアセットは
テーマに合わせて自動的に色を付けることができます。
色合いは BitmapDrawable
、NinePatchDrawable
、VectorDrawable
に適用できます
setTint()
メソッドを使って取得します。Google Chat では
また、android:tint
と
android:tintMode
属性。
画像から代表色を抽出する
Android サポート ライブラリには、画像から代表色を抽出できる Palette
クラスが含まれています。
ドローアブルを Bitmap
として読み込み、Palette
に渡してその色にアクセスできます。
詳しくは、
Palette API を使用します。