Starting in Android Studio Koala Feature Drop, you can view snapshots of your Wear OS app's tiles. This panel is particularly useful if your tile's appearance changes in response to conditions, such as different content depending on the device's display size, or a sports event reaching halftime.
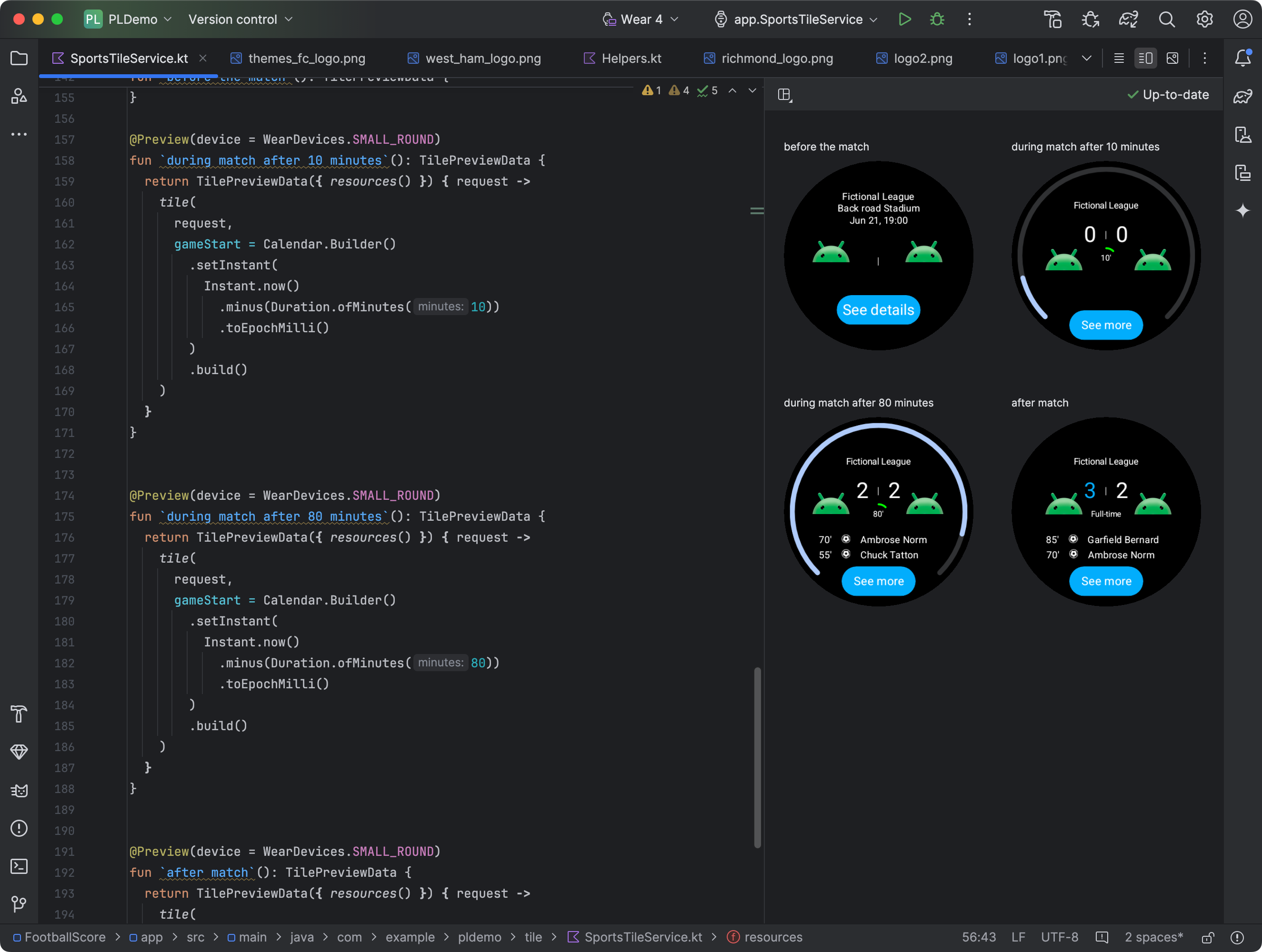
Add dependencies
Include the following dependencies in your app's build.gradle.kts
or
build.gradle
file:
dependencies {
implementation("androidx.wear.tiles:tiles-tooling-preview:1.5.0-alpha04")
debugImplementation("androidx.wear.tiles:tiles-tooling:1.5.0-alpha04")
implementation("androidx.wear:wear-tooling-preview:1.0.0")
}
Configure tile previews
To see a preview of your tile's appearance on different Wear OS display
sizes, add the @Preview
annotation, and pass in the device
parameter. Note
that this @Preview
annotation is from a different package than the one you
use for composable previews.
import androidx.wear.tiles.tooling.preview.Preview
@Preview(device = WearDevices.SMALL_ROUND)
@Preview(device = WearDevices.LARGE_ROUND)
fun tilePreview(context: Context) = TilePreviewData(
onTileRequest = { request ->
TilePreviewHelper.singleTimelineEntryTileBuilder(
buildMyTileLayout()
).build()
}
)
Add and register resources
If your tile uses Android resources, you will need to register them within the
onTileResourceRequest
parameter of TilePreviewData
, as shown in the
following code snippet:
import androidx.wear.tiles.tooling.preview.Preview @Preview(device = WearDevices.SMALL_ROUND) fun previewWithResources(context: Context) = TilePreviewData( onTileResourceRequest = { request -> Resources.Builder() .setVersion(myResourcesVersion) .addIdToImageMapping( myImageId, getImageById(R.drawable.myDrawableImageId)) .build() }, onTileRequest = { request -> TilePreviewHelper.singleTimelineEntryTileBuilder( buildMyTileLayout() ).build() } ) fun getImageById( @DrawableRes id: Int, ): ImageResource = ImageResource.Builder() .setAndroidResourceByResId( AndroidImageResourceByResId.Builder() .setResourceId(id) .build(), ) .build()
Show specific values from platform data sources
If your tile uses platform data—such as heart rate, calories, distance, and steps—the tile shows default values for them.
To show a specific value, set the platformDataValues
parameter when creating
the TilePreviewData
object, as shown in the following code snippet:
import androidx.wear.tiles.tooling.preview.Preview @Preview(device = WearDevices.SMALL_ROUND) fun previewWithPlatformOverride(context: Context) = TilePreviewData( platformDataValues = PlatformDataValues.of( PlatformHealthSources.Keys.HEART_RATE_BPM, DynamicDataBuilders.DynamicDataValue.fromFloat(160f) ), onTileRequest = { request -> TilePreviewHelper.singleTimelineEntryTileBuilder( buildMyTileLayout() ).build() } )