借助 Compose,您可以创建由多边形组成的形状。例如,您可以制作以下类型的形状:
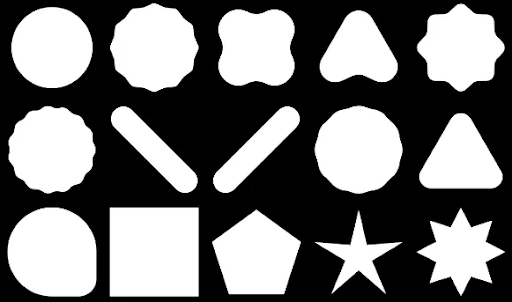
如需在 Compose 中创建自定义圆角多边形,请将 graphics-shapes
依赖项添加到 app/build.gradle
:
implementation "androidx.graphics:graphics-shapes:1.0.0-alpha05"
借助此库,您可以创建由多边形制成的形状。虽然多边形只有直边和尖角,但这些形状允许使用可选的圆角。您可以轻松地在两种不同的形状之间进行变形。任意形状之间的变形很难处理,并且往往是一个设计时问题。但这个库通过在这些具有类似多边形结构的形状之间进行变形,来简化操作。
创建多边形
以下代码段会创建一个以绘制区域的中心为 6 个点的基本多边形形状:
Box( modifier = Modifier .drawWithCache { val roundedPolygon = RoundedPolygon( numVertices = 6, radius = size.minDimension / 2, centerX = size.width / 2, centerY = size.height / 2 ) val roundedPolygonPath = roundedPolygon.toPath().asComposePath() onDrawBehind { drawPath(roundedPolygonPath, color = Color.Blue) } } .fillMaxSize() )
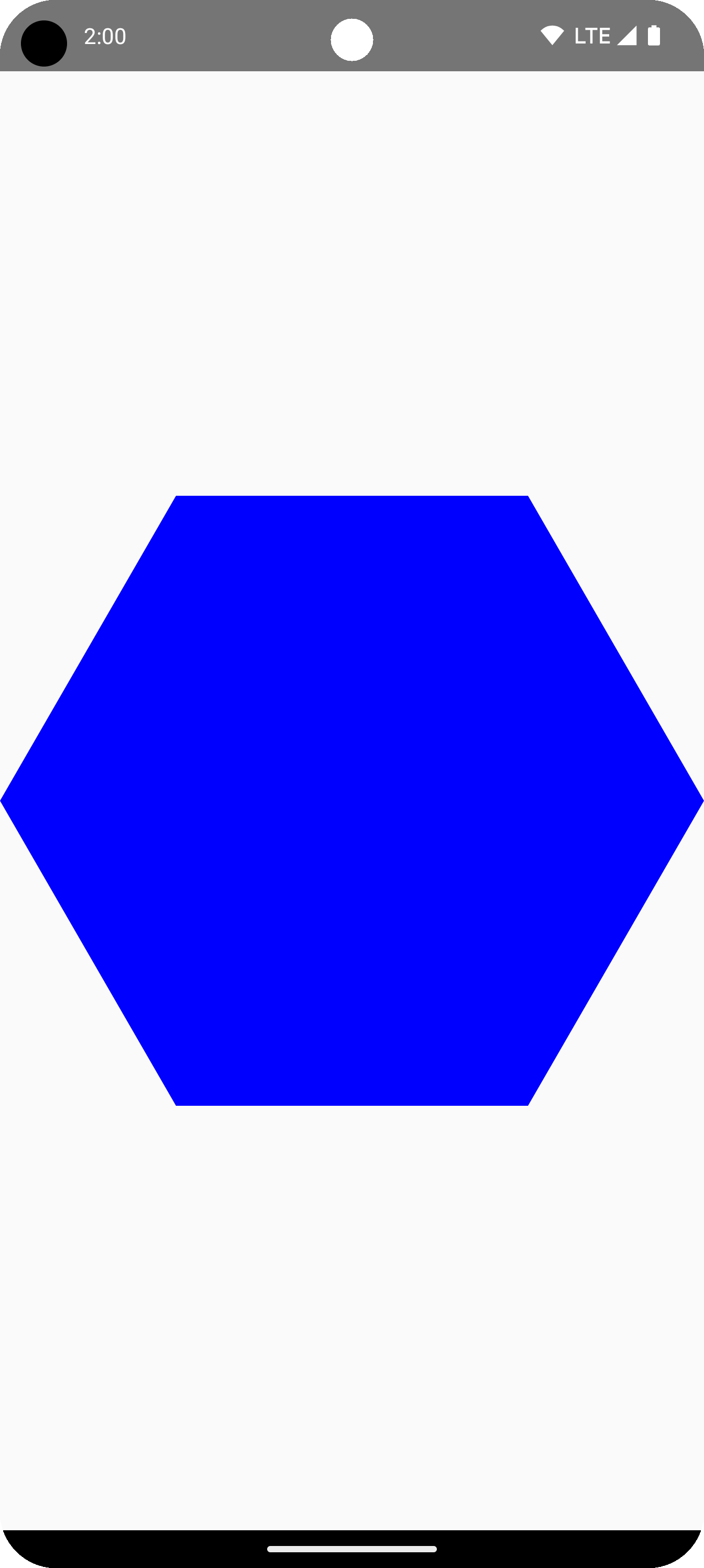
在此示例中,该库会创建一个 RoundedPolygon
,其中包含表示所请求形状的几何图形。如需在 Compose 应用中绘制该形状,您必须从中获取 Path
对象,以将形状转换为 Compose 知道如何绘制的形式。
将多边形的角设置为圆角
如需对多边形的角进行圆角处理,请使用 CornerRounding
参数。这接受两个参数:radius
和 smoothing
。每个圆角由 1-3 个立方曲线组成,其中心为圆弧,而两条边(“侧置”)曲线从形状的边缘过渡到中心曲线。
半径
radius
是用于取整顶点的圆的半径。
例如,以下圆角三角形如下所示:
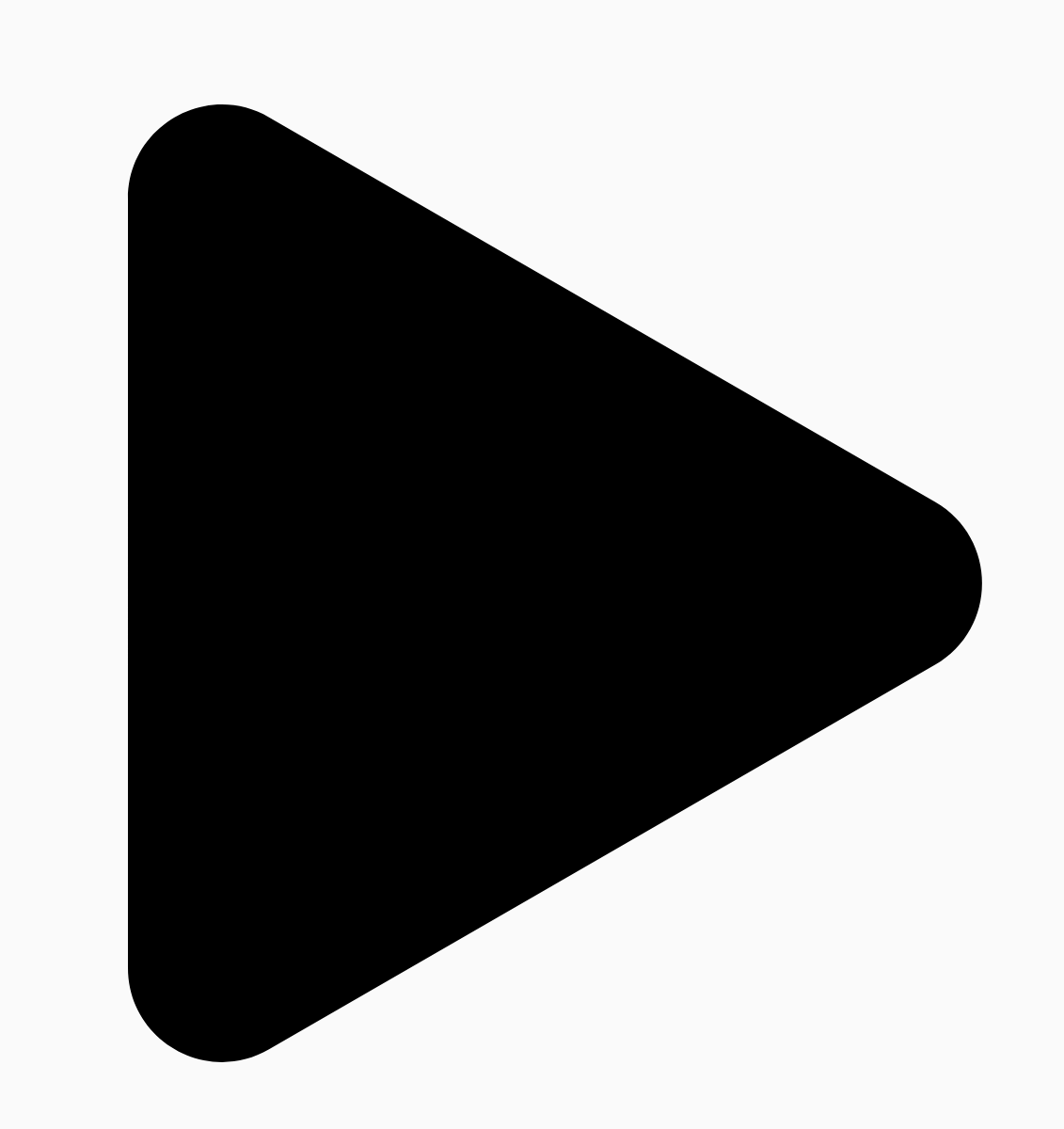
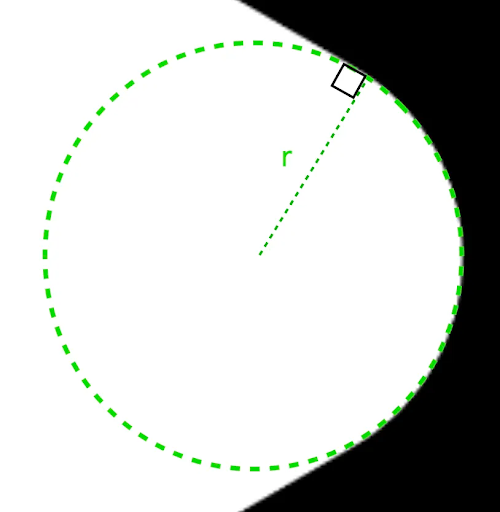
r
决定了圆角的圆角大小。平滑
平滑是决定从角的圆形圆角部分到边缘所需的时间的因素。平滑因数为 0(未平滑,CornerRounding
的默认值)会导致纯圆角舍入。非零平滑因数(最大值为 1.0)会导致角被三条单独的曲线圆角。
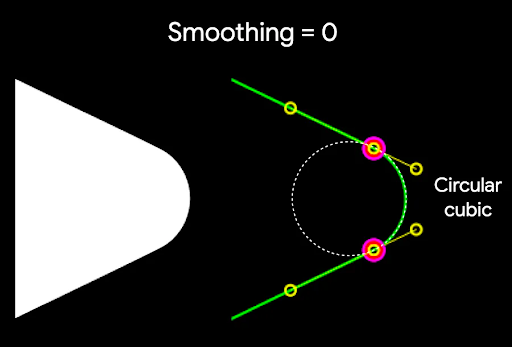
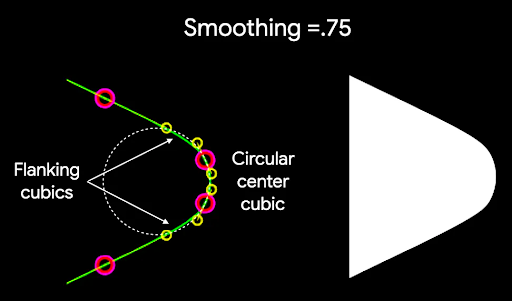
例如,以下代码段展示了将平滑处理设置为 0 与 1 之间的细微差别:
Box( modifier = Modifier .drawWithCache { val roundedPolygon = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2, centerX = size.width / 2, centerY = size.height / 2, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val roundedPolygonPath = roundedPolygon.toPath().asComposePath() onDrawBehind { drawPath(roundedPolygonPath, color = Color.Black) } } .size(100.dp) )
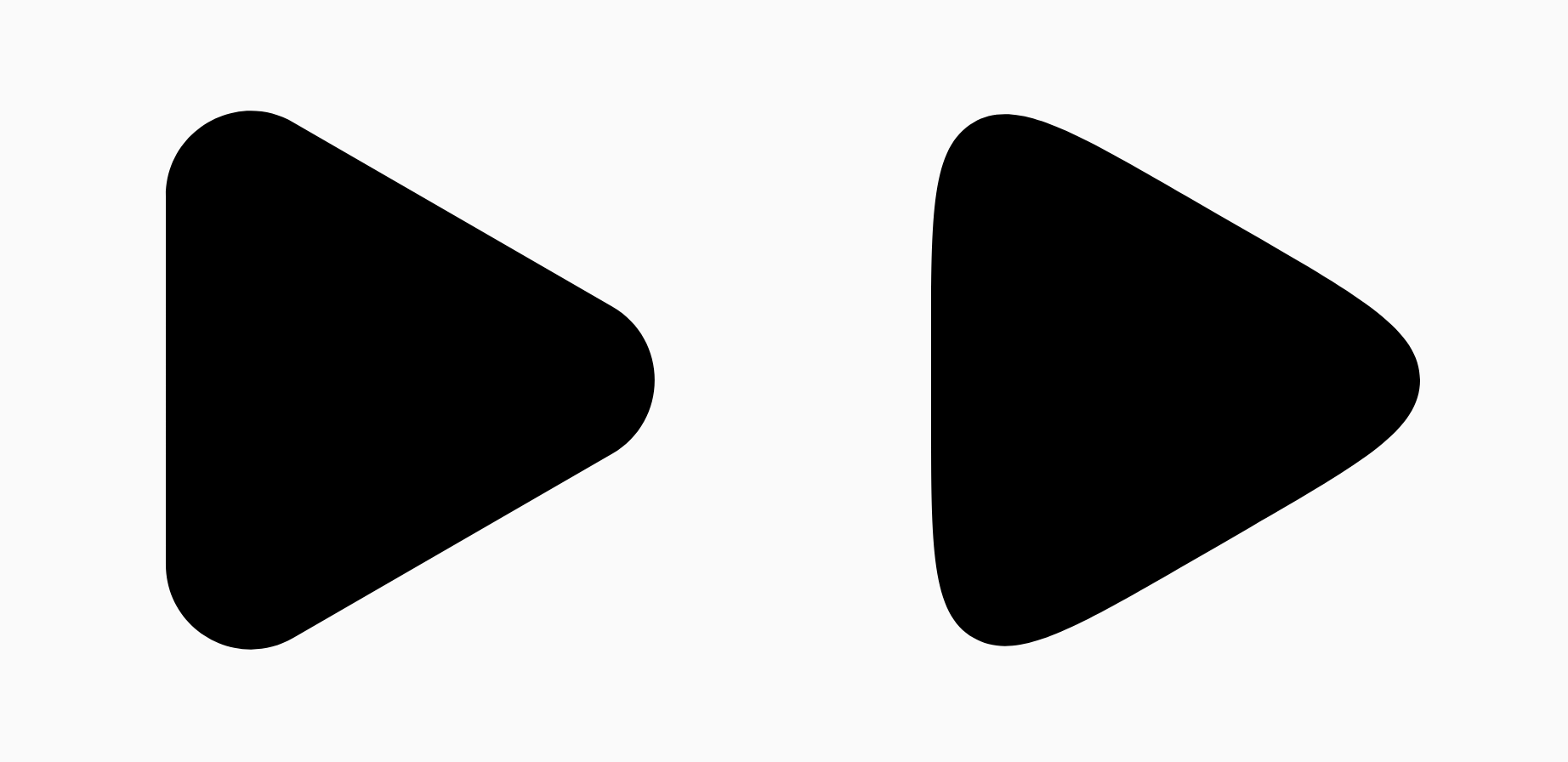
尺寸和位置
默认情况下,创建一个以中心 (0, 0
) 为中心的半径为 1
。这个半径表示形状所基于的多边形的中心与外部顶点之间的距离。请注意,对角进行圆角生成的形状较小,因为圆角比圆角的顶点更接近中心。若要调整多边形的大小,请调整 radius
值。如需调整位置,请更改多边形的 centerX
或 centerY
。或者,使用标准 DrawScope
转换函数(例如 DrawScope#translate()
)转换对象,以更改其大小、位置和旋转角度。
变形形状
Morph
对象是一种新形状,表示两个多边形形状之间的动画。如需在两种形状之间变形,请创建两个 RoundedPolygons
和一个采用这两种形状的 Morph
对象。如需计算起始形状和结束形状之间的形状,请提供介于 0 到 1 之间的 progress
值,以确定起始形状 (0) 和结束形状 (1) 之间的形状:
Box( modifier = Modifier .drawWithCache { val triangle = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val square = RoundedPolygon( numVertices = 4, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f ) val morph = Morph(start = triangle, end = square) val morphPath = morph .toPath(progress = 0.5f).asComposePath() onDrawBehind { drawPath(morphPath, color = Color.Black) } } .fillMaxSize() )
在上面的示例中,进度正好位于两个形状(圆角三角形和方形)的中间,生成的结果如下:
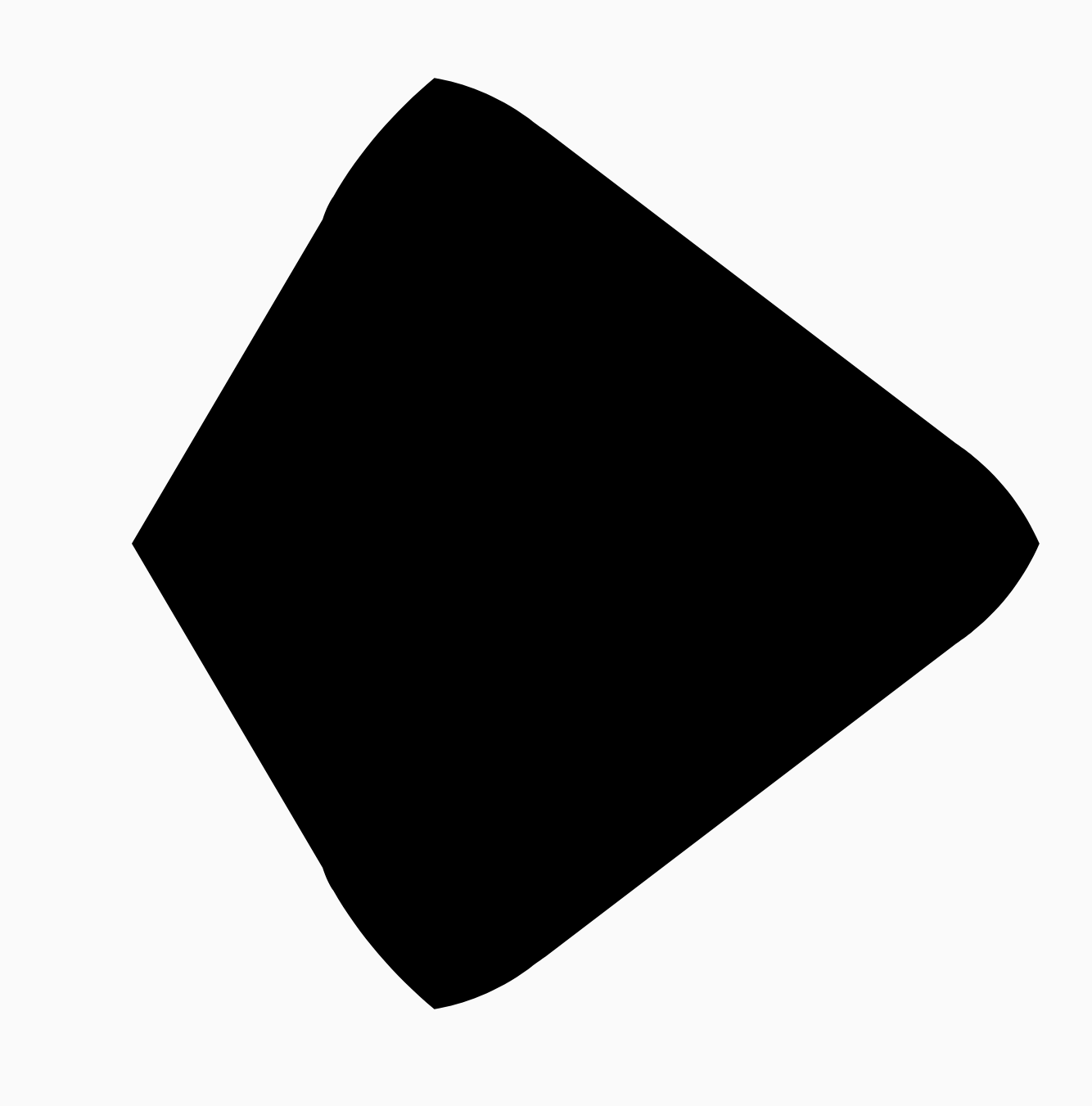
在大多数情况下,变形作为动画的一部分(而不只是静态渲染)完成。如需在两者之间添加动画效果,您可以使用 Compose 中的标准动画 API 随时间更改进度值。例如,您可以为这两个形状之间的变形无限添加动画效果,如下所示:
val infiniteAnimation = rememberInfiniteTransition(label = "infinite animation") val morphProgress = infiniteAnimation.animateFloat( initialValue = 0f, targetValue = 1f, animationSpec = infiniteRepeatable( tween(500), repeatMode = RepeatMode.Reverse ), label = "morph" ) Box( modifier = Modifier .drawWithCache { val triangle = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val square = RoundedPolygon( numVertices = 4, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f ) val morph = Morph(start = triangle, end = square) val morphPath = morph .toPath(progress = morphProgress.value) .asComposePath() onDrawBehind { drawPath(morphPath, color = Color.Black) } } .fillMaxSize() )
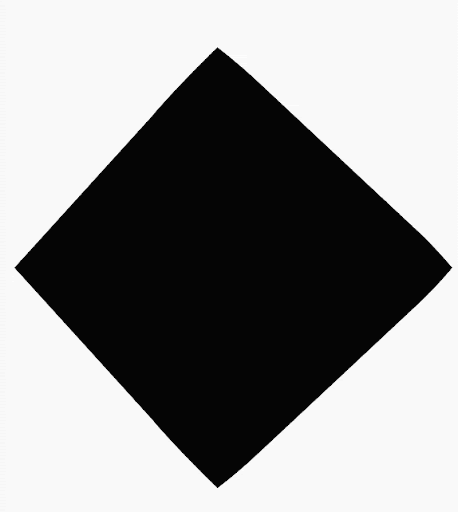
使用多边形作为裁剪
通常在 Compose 中使用 clip
修饰符来更改可组合项的渲染方式,并利用在剪裁区域周围绘制的阴影:
fun RoundedPolygon.getBounds() = calculateBounds().let { Rect(it[0], it[1], it[2], it[3]) } class RoundedPolygonShape( private val polygon: RoundedPolygon, private var matrix: Matrix = Matrix() ) : Shape { private var path = Path() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { path.rewind() path = polygon.toPath().asComposePath() matrix.reset() val bounds = polygon.getBounds() val maxDimension = max(bounds.width, bounds.height) matrix.scale(size.width / maxDimension, size.height / maxDimension) matrix.translate(-bounds.left, -bounds.top) path.transform(matrix) return Outline.Generic(path) } }
然后,您可以将多边形用作裁剪,如以下代码段所示:
val hexagon = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val clip = remember(hexagon) { RoundedPolygonShape(polygon = hexagon) } Box( modifier = Modifier .clip(clip) .background(MaterialTheme.colorScheme.secondary) .size(200.dp) ) { Text( "Hello Compose", color = MaterialTheme.colorScheme.onSecondary, modifier = Modifier.align(Alignment.Center) ) }
这会导致出现以下情况:
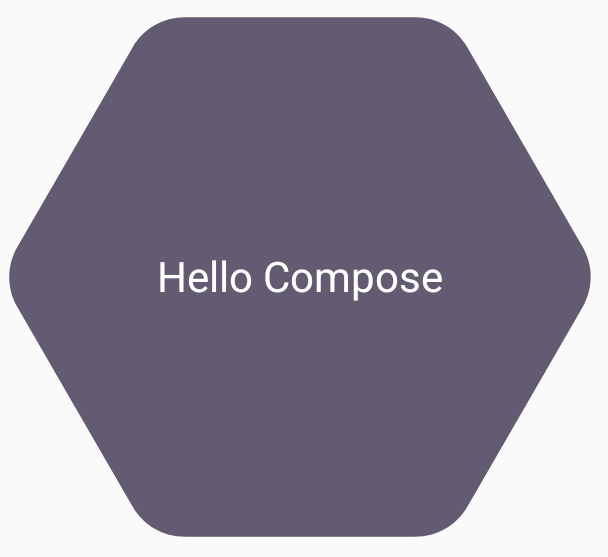
这看起来可能与之前渲染的内容没有什么不同,但可让您利用 Compose 中的其他功能。例如,以下方法可用于裁剪图片并在裁剪区域周围应用阴影:
val hexagon = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val clip = remember(hexagon) { RoundedPolygonShape(polygon = hexagon) } Box( modifier = Modifier.fillMaxSize(), contentAlignment = Alignment.Center ) { Image( painter = painterResource(id = R.drawable.dog), contentDescription = "Dog", contentScale = ContentScale.Crop, modifier = Modifier .graphicsLayer { this.shadowElevation = 6.dp.toPx() this.shape = clip this.clip = true this.ambientShadowColor = Color.Black this.spotShadowColor = Color.Black } .size(200.dp) ) }
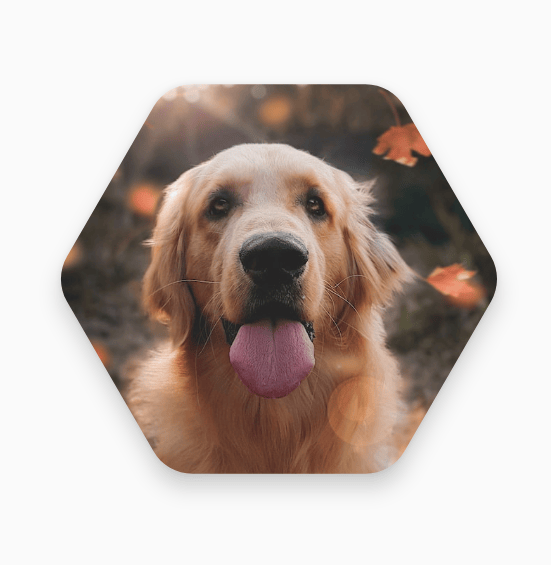
点击时变形按钮
您可以使用 graphics-shape
库创建一个按钮,该按钮会在按下时在两个形状之间变形。首先,创建一个扩展 Shape
的 MorphPolygonShape
,并缩放和平移该元素以适应相应大小。请注意传入的进度,以便能够为形状添加动画效果:
class MorphPolygonShape( private val morph: Morph, private val percentage: Float ) : Shape { private val matrix = Matrix() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { // Below assumes that you haven't changed the default radius of 1f, nor the centerX and centerY of 0f // By default this stretches the path to the size of the container, if you don't want stretching, use the same size.width for both x and y. matrix.scale(size.width / 2f, size.height / 2f) matrix.translate(1f, 1f) val path = morph.toPath(progress = percentage).asComposePath() path.transform(matrix) return Outline.Generic(path) } }
如需使用此变形形状,请创建两个多边形:shapeA
和 shapeB
。创建并记住 Morph
。然后,将变形作为剪辑轮廓应用于按钮,并将按下时的 interactionSource
用作动画背后的驱动力:
val shapeA = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val shapeB = remember { RoundedPolygon.star( 6, rounding = CornerRounding(0.1f) ) } val morph = remember { Morph(shapeA, shapeB) } val interactionSource = remember { MutableInteractionSource() } val isPressed by interactionSource.collectIsPressedAsState() val animatedProgress = animateFloatAsState( targetValue = if (isPressed) 1f else 0f, label = "progress", animationSpec = spring(dampingRatio = 0.4f, stiffness = Spring.StiffnessMedium) ) Box( modifier = Modifier .size(200.dp) .padding(8.dp) .clip(MorphPolygonShape(morph, animatedProgress.value)) .background(Color(0xFF80DEEA)) .size(200.dp) .clickable(interactionSource = interactionSource, indication = null) { } ) { Text("Hello", modifier = Modifier.align(Alignment.Center)) }
这样一来,当用户点按该框时,会生成以下动画:
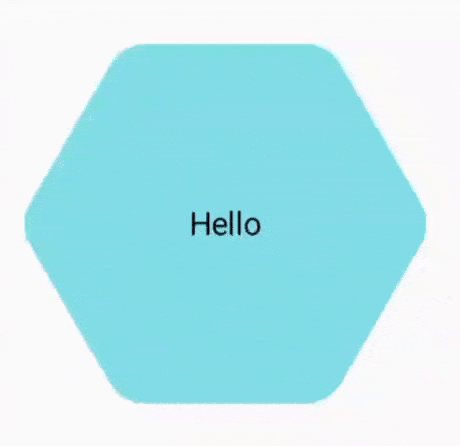
为形状无限变形添加动画效果
如需无限地为变形形状添加动画效果,请使用 rememberInfiniteTransition
。下面的示例展示了形状会随着时间无限改变(和旋转)的个人资料照片。此方法对上面显示的 MorphPolygonShape
稍作调整:
class CustomRotatingMorphShape( private val morph: Morph, private val percentage: Float, private val rotation: Float ) : Shape { private val matrix = Matrix() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { // Below assumes that you haven't changed the default radius of 1f, nor the centerX and centerY of 0f // By default this stretches the path to the size of the container, if you don't want stretching, use the same size.width for both x and y. matrix.scale(size.width / 2f, size.height / 2f) matrix.translate(1f, 1f) matrix.rotateZ(rotation) val path = morph.toPath(progress = percentage).asComposePath() path.transform(matrix) return Outline.Generic(path) } } @Preview @Composable private fun RotatingScallopedProfilePic() { val shapeA = remember { RoundedPolygon( 12, rounding = CornerRounding(0.2f) ) } val shapeB = remember { RoundedPolygon.star( 12, rounding = CornerRounding(0.2f) ) } val morph = remember { Morph(shapeA, shapeB) } val infiniteTransition = rememberInfiniteTransition("infinite outline movement") val animatedProgress = infiniteTransition.animateFloat( initialValue = 0f, targetValue = 1f, animationSpec = infiniteRepeatable( tween(2000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "animatedMorphProgress" ) val animatedRotation = infiniteTransition.animateFloat( initialValue = 0f, targetValue = 360f, animationSpec = infiniteRepeatable( tween(6000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "animatedMorphProgress" ) Box( modifier = Modifier.fillMaxSize(), contentAlignment = Alignment.Center ) { Image( painter = painterResource(id = R.drawable.dog), contentDescription = "Dog", contentScale = ContentScale.Crop, modifier = Modifier .clip( CustomRotatingMorphShape( morph, animatedProgress.value, animatedRotation.value ) ) .size(200.dp) ) } }
此代码会给出以下有趣的结果:
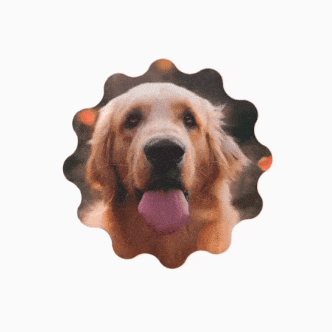
自定义多边形
如果通过正多边形创建的形状无法涵盖您的用例,您可以使用顶点列表创建更自定义的形状。例如,您可能需要创建一个如下所示的心形:
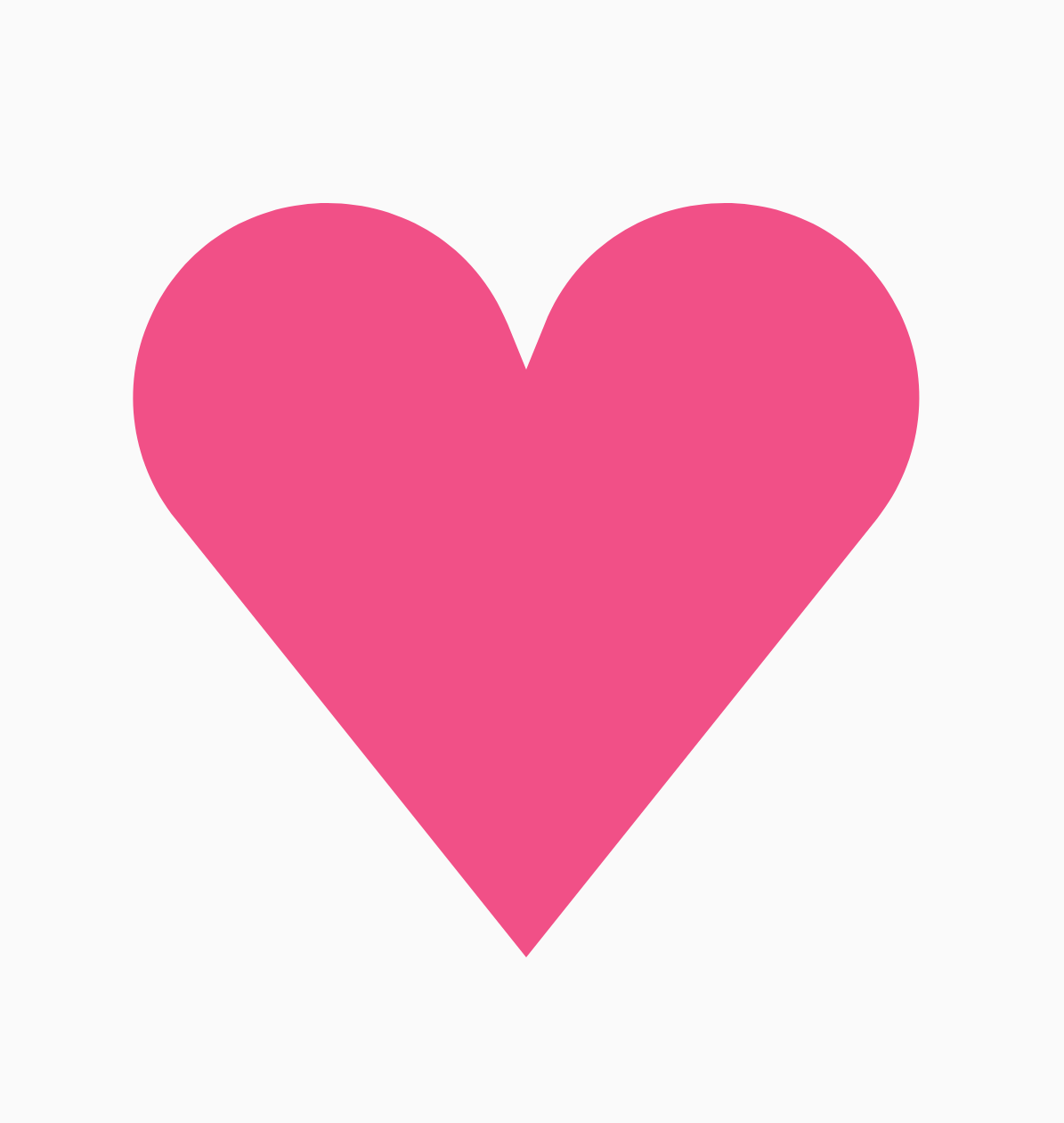
您可以使用接受 x、y 坐标的浮点数组的 RoundedPolygon
重载来指定此形状的各个顶点。
如需拆分心形多边形,请注意用于指定点的极坐标系比使用笛卡尔 (x,y) 坐标系更容易实现,其中 0°
从右侧开始,顺时针前进,270°
位于 12 点钟位置:
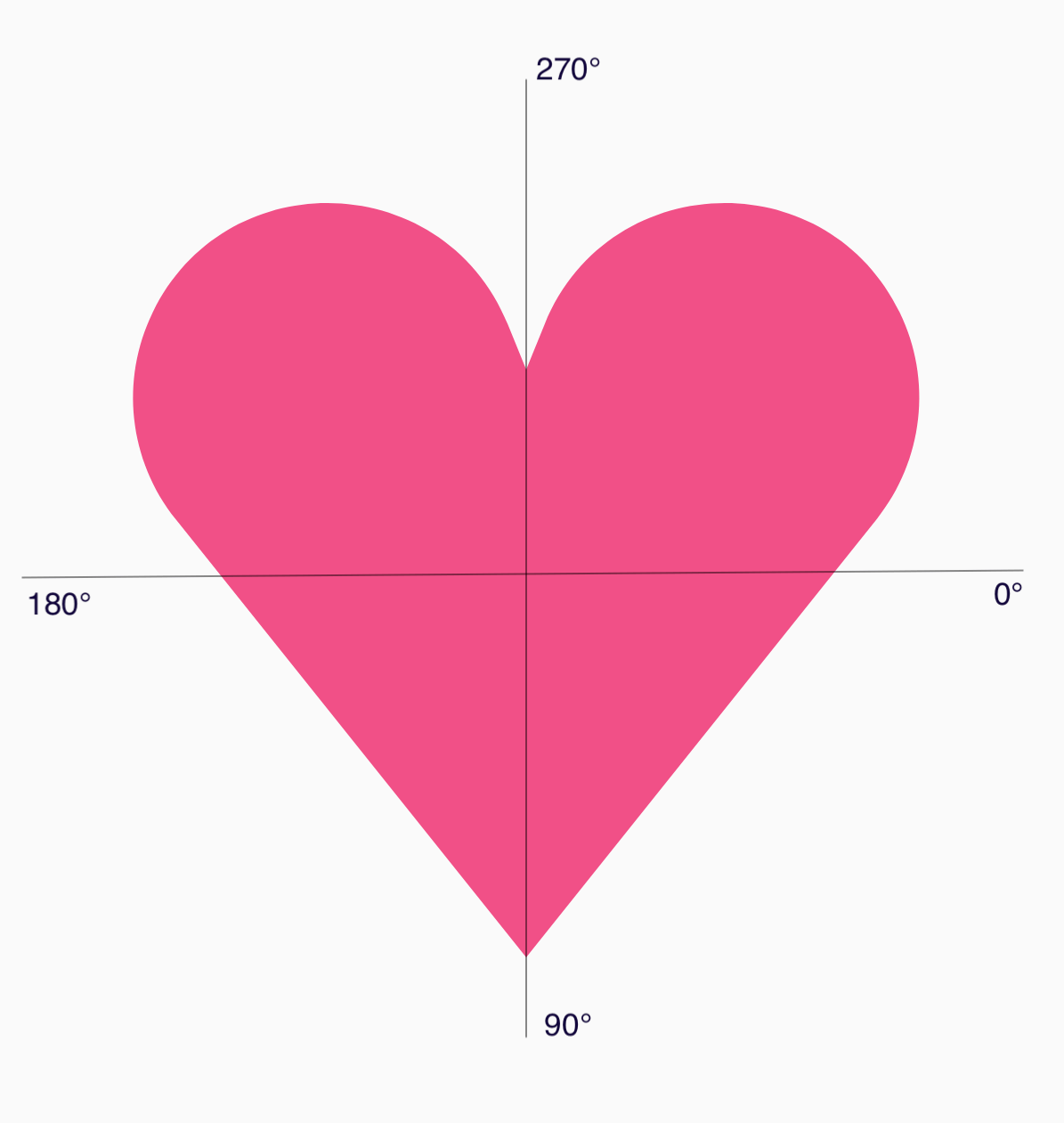
现在,通过指定每个点的中心的角度 (?) 和半径,可以更轻松地定义形状:
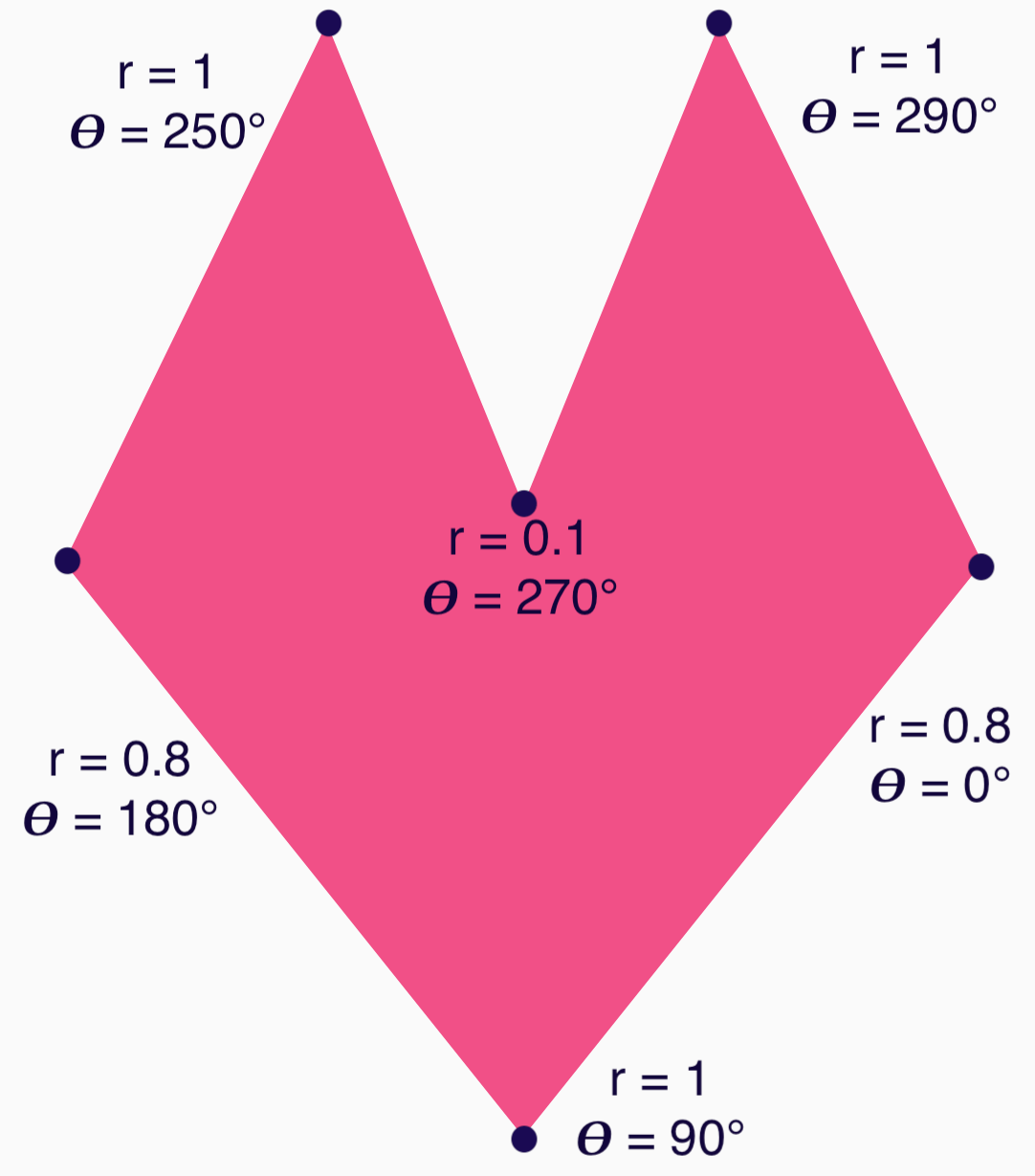
现在可以创建顶点并将其传递给 RoundedPolygon
函数:
val vertices = remember { val radius = 1f val radiusSides = 0.8f val innerRadius = .1f floatArrayOf( radialToCartesian(radiusSides, 0f.toRadians()).x, radialToCartesian(radiusSides, 0f.toRadians()).y, radialToCartesian(radius, 90f.toRadians()).x, radialToCartesian(radius, 90f.toRadians()).y, radialToCartesian(radiusSides, 180f.toRadians()).x, radialToCartesian(radiusSides, 180f.toRadians()).y, radialToCartesian(radius, 250f.toRadians()).x, radialToCartesian(radius, 250f.toRadians()).y, radialToCartesian(innerRadius, 270f.toRadians()).x, radialToCartesian(innerRadius, 270f.toRadians()).y, radialToCartesian(radius, 290f.toRadians()).x, radialToCartesian(radius, 290f.toRadians()).y, ) }
您需要使用以下 radialToCartesian
函数将顶点转换为笛卡尔坐标:
internal fun Float.toRadians() = this * PI.toFloat() / 180f internal val PointZero = PointF(0f, 0f) internal fun radialToCartesian( radius: Float, angleRadians: Float, center: PointF = PointZero ) = directionVectorPointF(angleRadians) * radius + center internal fun directionVectorPointF(angleRadians: Float) = PointF(cos(angleRadians), sin(angleRadians))
上述代码为您提供了心形的原始顶点,但您需要对特定角进行圆角处理才能得到所选心形的形状。90°
和 270°
的角没有圆角,但其他角没有圆角。如需为各个角实现自定义圆角,请使用 perVertexRounding
参数:
val rounding = remember { val roundingNormal = 0.6f val roundingNone = 0f listOf( CornerRounding(roundingNormal), CornerRounding(roundingNone), CornerRounding(roundingNormal), CornerRounding(roundingNormal), CornerRounding(roundingNone), CornerRounding(roundingNormal), ) } val polygon = remember(vertices, rounding) { RoundedPolygon( vertices = vertices, perVertexRounding = rounding ) } Box( modifier = Modifier .drawWithCache { val roundedPolygonPath = polygon.toPath().asComposePath() onDrawBehind { scale(size.width * 0.5f, size.width * 0.5f) { translate(size.width * 0.5f, size.height * 0.5f) { drawPath(roundedPolygonPath, color = Color(0xFFF15087)) } } } } .size(400.dp) )
这会产生粉色心形:
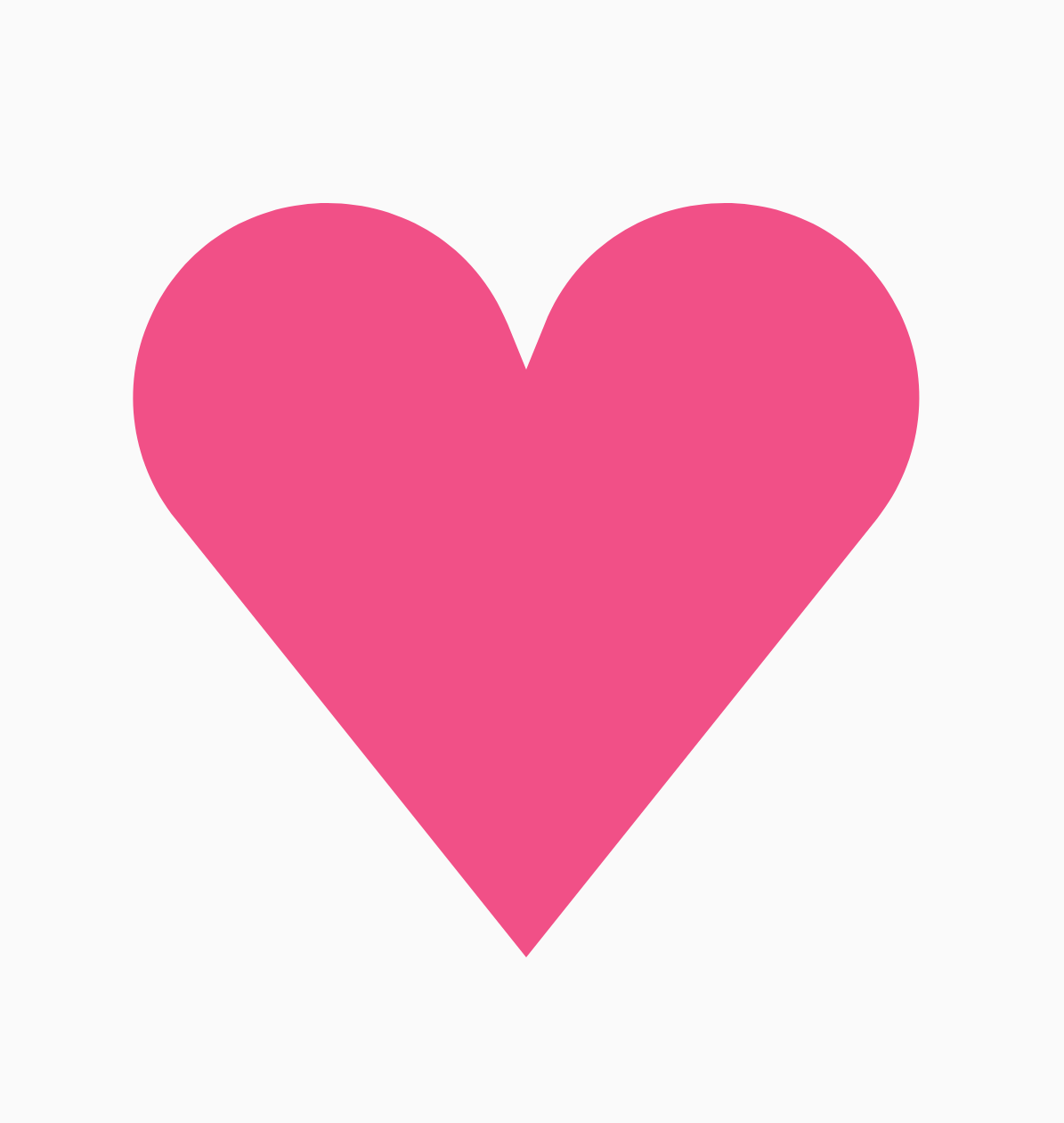
如果上述形状无法涵盖您的用例,请考虑使用 Path
类绘制自定义形状,或从磁盘加载 ImageVector
文件。graphics-shapes
库并非用于任意形状,而专门用于简化圆角多边形的创建以及圆角多边形之间的变形动画。
其他资源
如需了解详情并查看示例,请参阅以下资源: