CameraX cung cấp API Extensions dùng cho việc truy cập vào các tiện ích mà nhà sản xuất thiết bị đã triển khai trên nhiều thiết bị Android. Để biết danh sách các chế độ được hỗ trợ của tiện ích, hãy xem bài viết Tiện ích dành cho máy ảnh.
Để biết danh sách các thiết bị hỗ trợ tiện ích, hãy xem bài viết Thiết bị được hỗ trợ.
Cấu trúc tiện ích
Hình ảnh sau đây cho thấy cấu trúc của các tiện ích máy ảnh.
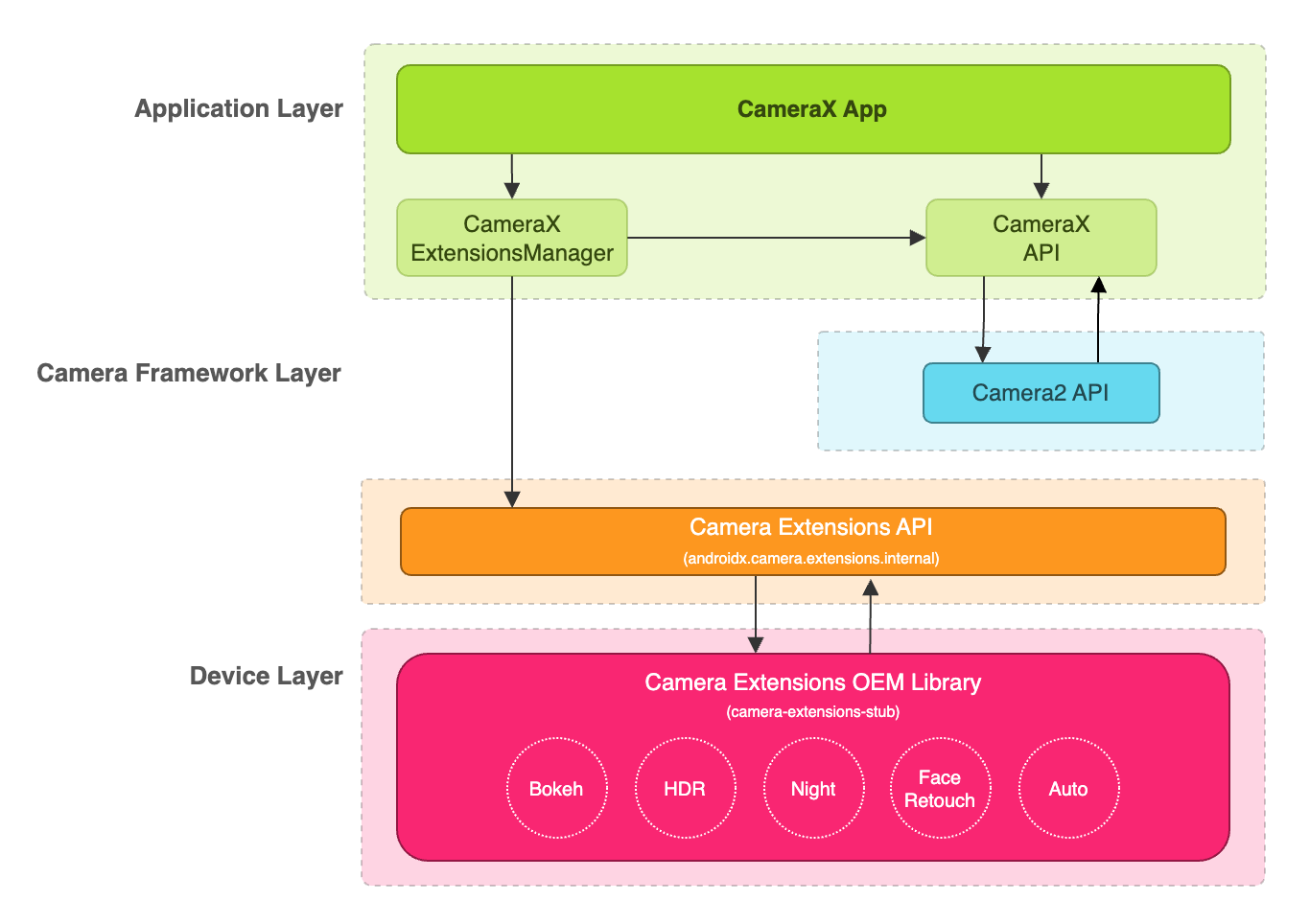
Ứng dụng CameraX có thể sử dụng các tiện ích thông qua CameraX Extensions API (API tiện ích CameraX). CameraX Extensions API (API tiện ích CameraX) quản lý việc truy vấn các tiện ích có sẵn, định cấu hình một phiên máy ảnh tiện ích và giao tiếp với thư viện OEM của Tiện ích máy ảnh. Điều này cho phép ứng dụng của bạn dùng các chức năng như Ban đêm, HDR, Tự động, Hiệu ứng Bokeh hoặc Làm đẹp khuôn mặt.
Bật tiện ích khi chụp và xem trước ảnh
Trước khi sử dụng Extensions API (API tiện ích), hãy truy xuất thực thể ExtensionsManager
bằng cách sử dụng phương thức ExtensionsManager#getInstanceAsync(Context, CameraProvider). Hành động này sẽ cho phép bạn truy vấn thông tin về tình trạng sẵn có của tiện ích. Sau đó, truy xuất tiện ích đã bật CameraSelector
. Chế độ tiện ích sẽ được áp dụng trong các trường hợp sử dụng chụp và xem trước ảnh khi gọi phương thức bindToLifecycle() và tiện ích CameraSelector
đã bật.
Để triển khai tiện ích cho trường hợp sử dụng chụp và xem trước ảnh, hãy tham khảo đoạn mã mẫu sau:
Kotlin
import androidx.camera.extensions.ExtensionMode import androidx.camera.extensions.ExtensionsManager override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) val lifecycleOwner = this val cameraProviderFuture = ProcessCameraProvider.getInstance(applicationContext) cameraProviderFuture.addListener({ // Obtain an instance of a process camera provider // The camera provider provides access to the set of cameras associated with the device. // The camera obtained from the provider will be bound to the activity lifecycle. val cameraProvider = cameraProviderFuture.get() val extensionsManagerFuture = ExtensionsManager.getInstanceAsync(applicationContext, cameraProvider) extensionsManagerFuture.addListener({ // Obtain an instance of the extensions manager // The extensions manager enables a camera to use extension capabilities available on // the device. val extensionsManager = extensionsManagerFuture.get() // Select the camera val cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA // Query if extension is available. // Not all devices will support extensions or might only support a subset of // extensions. if (extensionsManager.isExtensionAvailable(cameraSelector, ExtensionMode.NIGHT)) { // Unbind all use cases before enabling different extension modes. try { cameraProvider.unbindAll() // Retrieve a night extension enabled camera selector val nightCameraSelector = extensionsManager.getExtensionEnabledCameraSelector( cameraSelector, ExtensionMode.NIGHT ) // Bind image capture and preview use cases with the extension enabled camera // selector. val imageCapture = ImageCapture.Builder().build() val preview = Preview.Builder().build() // Connect the preview to receive the surface the camera outputs the frames // to. This will allow displaying the camera frames in either a TextureView // or SurfaceView. The SurfaceProvider can be obtained from the PreviewView. preview.setSurfaceProvider(surfaceProvider) // Returns an instance of the camera bound to the lifecycle // Use this camera object to control various operations with the camera // Example: flash, zoom, focus metering etc. val camera = cameraProvider.bindToLifecycle( lifecycleOwner, nightCameraSelector, imageCapture, preview ) } catch (e: Exception) { Log.e(TAG, "Use case binding failed", e) } } }, ContextCompat.getMainExecutor(this)) }, ContextCompat.getMainExecutor(this)) }
Java
import androidx.camera.extensions.ExtensionMode; import androidx.camera.extensions.ExtensionsManager; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); final LifecycleOwner lifecycleOwner = this; final ListenableFuturecameraProviderFuture = ProcessCameraProvider.getInstance(getApplicationContext()); cameraProviderFuture.addListener(() -> { try { // Obtain an instance of a process camera provider // The camera provider provides access to the set of cameras associated with the // device. The camera obtained from the provider will be bound to the activity // lifecycle. final ProcessCameraProvider cameraProvider = cameraProviderFuture.get(); final ListenableFuture extensionsManagerFuture = ExtensionsManager.getInstanceAsync(getApplicationContext(), cameraProvider); extensionsManagerFuture.addListener(() -> { // Obtain an instance of the extensions manager // The extensions manager enables a camera to use extension capabilities available // on the device. try { final ExtensionsManager extensionsManager = extensionsManagerFuture.get(); // Select the camera final CameraSelector cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA; // Query if extension is available. // Not all devices will support extensions or might only support a subset of // extensions. if (extensionsManager.isExtensionAvailable( cameraSelector, ExtensionMode.NIGHT )) { // Unbind all use cases before enabling different extension modes. cameraProvider.unbindAll(); // Retrieve extension enabled camera selector final CameraSelector nightCameraSelector = extensionsManager .getExtensionEnabledCameraSelector(cameraSelector, ExtensionMode.NIGHT); // Bind image capture and preview use cases with the extension enabled camera // selector. final ImageCapture imageCapture = new ImageCapture.Builder().build(); final Preview preview = new Preview.Builder().build(); // Connect the preview to receive the surface the camera outputs the frames // to. This will allow displaying the camera frames in either a TextureView // or SurfaceView. The SurfaceProvider can be obtained from the PreviewView. preview.setSurfaceProvider(surfaceProvider); cameraProvider.bindToLifecycle( lifecycleOwner, nightCameraSelector, imageCapture, preview ); } } catch (ExecutionException | InterruptedException e) { throw new RuntimeException(e); } }, ContextCompat.getMainExecutor(this)); } catch (ExecutionException | InterruptedException e) { throw new RuntimeException(e); } }, ContextCompat.getMainExecutor(this)); }
Tắt tiện ích
Để tắt tiện ích của nhà cung cấp, hãy huỷ liên kết mọi trường hợp sử dụng và liên kết lại các trường hợp sử dụng tính năng chụp và xem trước ảnh bằng bộ chọn máy ảnh thông thường. Ví dụ: Liên kết lại với camera sau bằng CameraSelector.DEFAULT_BACK_CAMERA
.
Phần phụ thuộc
API Extensions của CameraX được triển khai trong thư viện camera-extensions
.
Các tiện ích phụ thuộc vào các mô-đun lõi của CameraX (core
, camera2
, lifecycle
).
Groovy
dependencies { def camerax_version = "1.2.0-rc01" implementation "androidx.camera:camera-core:${camerax_version}" implementation "androidx.camera:camera-camera2:${camerax_version}" implementation "androidx.camera:camera-lifecycle:${camerax_version}" //the CameraX Extensions library implementation "androidx.camera:camera-extensions:${camerax_version}" ... }
Kotlin
dependencies { val camerax_version = "1.2.0-rc01" implementation("androidx.camera:camera-core:${camerax_version}") implementation("androidx.camera:camera-camera2:${camerax_version}") implementation("androidx.camera:camera-lifecycle:${camerax_version}") // the CameraX Extensions library implementation("androidx.camera:camera-extensions:${camerax_version}") ... }
Xoá API cũ
Với API Extensions mới được phát hành trong 1.0.0-alpha26
, API Extensions cũ phát hành vào tháng 8 năm 2019 hiện không còn được dùng nữa. Kể từ phiên bản 1.0.0-alpha28
, API Extensions cũ đã bị xoá khỏi thư viện. Các ứng dụng sử dụng Extensions API (API tiện ích) mới hiện phải nhận được CameraSelector
đã bật tiện ích và sử dụng lớp này để liên kết các trường hợp sử dụng.
Các ứng dụng sử dụng API Extensions cũ phải chuyển sang API Extensions mới để đảm bảo khả năng tương thích trong tương lai với các bản phát hành CameraX sắp tới.
Tài nguyên khác
Để tìm hiểu thêm về CameraX, hãy tham khảo các tài liệu bổ sung sau đây.
Lớp học lập trình
Mã mẫu
Ứng dụng mẫu của CameraX Extensions
Tài liệu tham khảo khác
Tiện ích của nhà cung cấp CameraX
Công cụ xác thực tiện ích của nhà cung cấp CameraX