Dialog
元件會在主要應用程式內容上方的圖層顯示彈出式訊息,或要求使用者輸入內容。這會造成中斷的 UI 體驗,吸引使用者的注意力。
對話方塊的用途包括:
- 確認使用者動作,例如刪除檔案時。
- 要求使用者輸入內容,例如在待辦事項清單應用程式中。
- 提供使用者選項清單,例如在設定個人資料時選擇國家/地區。
快訊對話方塊
AlertDialog
可組合函式提供方便的 API,可用來建立以 Material Design 為主題的對話方塊。AlertDialog
具有專用參數
處理對話方塊的特定元素。包括:
title
:對話方塊頂端顯示的文字。text
:對話方塊中以居中顯示的文字。icon
:對話方塊頂端顯示的圖片。onDismissRequest
:使用者關閉對話方塊時呼叫的函式。 例如在外碰觸碰dismissButton
:做為關閉按鈕的可組合函式。confirmButton
:做為確認按鈕的可組合函式。
以下範例會在警告對話方塊中實作兩個按鈕,一個用於關閉對話方塊,另一個則用於確認要求。
@Composable fun AlertDialogExample( onDismissRequest: () -> Unit, onConfirmation: () -> Unit, dialogTitle: String, dialogText: String, icon: ImageVector, ) { AlertDialog( icon = { Icon(icon, contentDescription = "Example Icon") }, title = { Text(text = dialogTitle) }, text = { Text(text = dialogText) }, onDismissRequest = { onDismissRequest() }, confirmButton = { TextButton( onClick = { onConfirmation() } ) { Text("Confirm") } }, dismissButton = { TextButton( onClick = { onDismissRequest() } ) { Text("Dismiss") } } ) }
這個實作方式暗示父項可組合項會以這種方式將引數傳遞至子項可組合項:
@Composable fun DialogExamples() { // ... val openAlertDialog = remember { mutableStateOf(false) } // ... when { // ... openAlertDialog.value -> { AlertDialogExample( onDismissRequest = { openAlertDialog.value = false }, onConfirmation = { openAlertDialog.value = false println("Confirmation registered") // Add logic here to handle confirmation. }, dialogTitle = "Alert dialog example", dialogText = "This is an example of an alert dialog with buttons.", icon = Icons.Default.Info ) } } } }
實作內容如下所示:
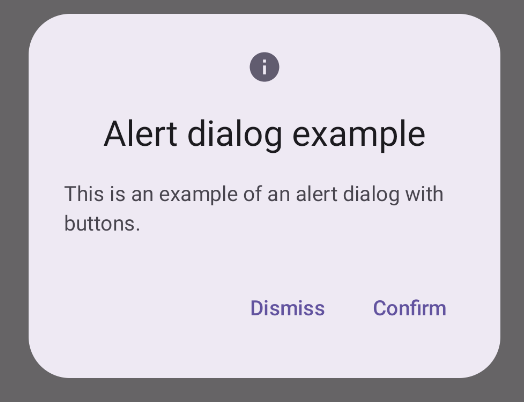
對話方塊可組合函式
Dialog
是基本的可組合項,不提供任何樣式或
預先定義的版位這是一個相對簡單的容器,您應使用 Card
等容器填入資料。以下是對話方塊的部分重要參數:
onDismissRequest
:使用者關閉對話方塊時呼叫的 lambda。properties
:DialogProperties
的例項,可提供一些額外的自訂範圍。
基本範例
以下範例為 Dialog
可組合項的基本實作。注意事項
以 Card
做為次要容器如果沒有 Card
,Text
則會顯示在主要應用程式內容上方。
@Composable fun MinimalDialog(onDismissRequest: () -> Unit) { Dialog(onDismissRequest = { onDismissRequest() }) { Card( modifier = Modifier .fillMaxWidth() .height(200.dp) .padding(16.dp), shape = RoundedCornerShape(16.dp), ) { Text( text = "This is a minimal dialog", modifier = Modifier .fillMaxSize() .wrapContentSize(Alignment.Center), textAlign = TextAlign.Center, ) } } }
實作內容如下所示。請注意,開啟對話方塊後,下方的應用程式主要內容會變暗並顯示為灰色:
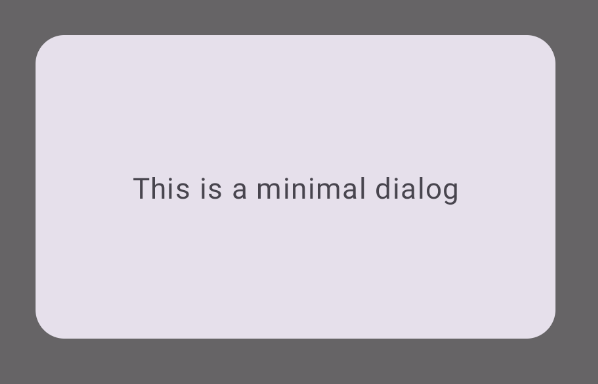
進階範例
以下是實作 Dialog
可組合項的進階實作。在這種情況下,元件會手動實作與上述 AlertDialog
範例相似的介面。
@Composable fun DialogWithImage( onDismissRequest: () -> Unit, onConfirmation: () -> Unit, painter: Painter, imageDescription: String, ) { Dialog(onDismissRequest = { onDismissRequest() }) { // Draw a rectangle shape with rounded corners inside the dialog Card( modifier = Modifier .fillMaxWidth() .height(375.dp) .padding(16.dp), shape = RoundedCornerShape(16.dp), ) { Column( modifier = Modifier .fillMaxSize(), verticalArrangement = Arrangement.Center, horizontalAlignment = Alignment.CenterHorizontally, ) { Image( painter = painter, contentDescription = imageDescription, contentScale = ContentScale.Fit, modifier = Modifier .height(160.dp) ) Text( text = "This is a dialog with buttons and an image.", modifier = Modifier.padding(16.dp), ) Row( modifier = Modifier .fillMaxWidth(), horizontalArrangement = Arrangement.Center, ) { TextButton( onClick = { onDismissRequest() }, modifier = Modifier.padding(8.dp), ) { Text("Dismiss") } TextButton( onClick = { onConfirmation() }, modifier = Modifier.padding(8.dp), ) { Text("Confirm") } } } } } }
此實作方式如下所示:
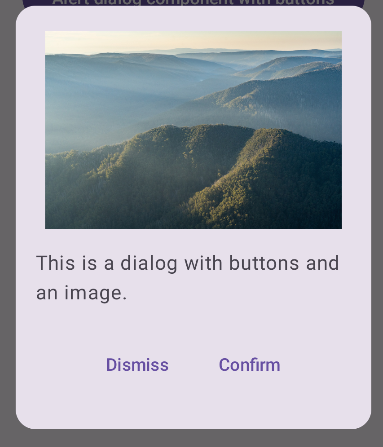