Compose には多くのアニメーション メカニズムが組み込まれており、どれを選択すればよいか判断するのは難しい場合があります。一般的なアニメーションのユースケースは次のとおりです。利用可能なさまざまな API オプションの詳細については、Compose Animation のドキュメントをご覧ください。
一般的なコンポーザブル プロパティをアニメーション化する
Compose には、一般的なアニメーションのユースケースを解決できる便利な API が用意されています。このセクションでは、コンポーザブルの一般的なプロパティをアニメーション化する方法について説明します。
表示 / 非表示をアニメーション化する
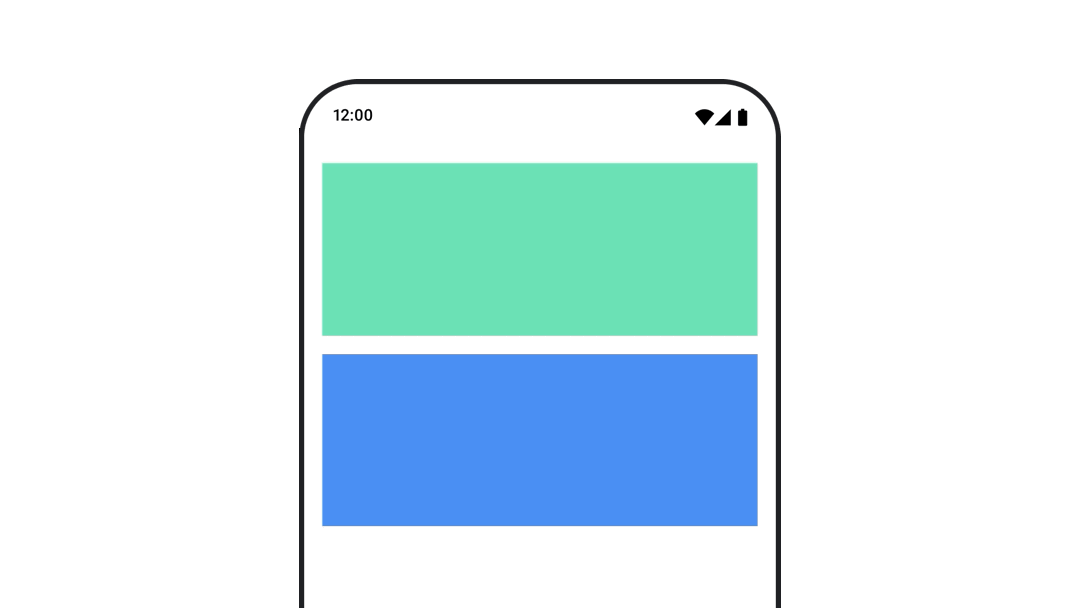
AnimatedVisibility
を使用して、コンポーザブルを非表示または表示します。AnimatedVisibility
内の子は、独自の開始遷移または終了遷移に Modifier.animateEnterExit()
を使用できます。
var visible by remember { mutableStateOf(true) } // Animated visibility will eventually remove the item from the composition once the animation has finished. AnimatedVisibility(visible) { // your composable here // ... }
AnimatedVisibility
の enter パラメータと exit パラメータを使用すると、コンポーザブルの表示と非表示の動作を構成できます。詳細については、完全なドキュメントをご覧ください。
コンポーザブルの可視性をアニメーション化するもう 1 つの方法は、animateFloatAsState
を使用して時間の経過とともにアルファをアニメーション化することです。
var visible by remember { mutableStateOf(true) } val animatedAlpha by animateFloatAsState( targetValue = if (visible) 1.0f else 0f, label = "alpha" ) Box( modifier = Modifier .size(200.dp) .graphicsLayer { alpha = animatedAlpha } .clip(RoundedCornerShape(8.dp)) .background(colorGreen) .align(Alignment.TopCenter) ) { }
ただし、アルファを変更すると、コンポーザブルはコンポジション内に残り、レイアウトされているスペースを占有し続けるという注意点があります。これにより、スクリーン リーダーやその他のユーザー補助メカニズムによって、画面上のアイテムが引き続き考慮される可能性があります。一方、AnimatedVisibility
は最終的にアイテムをコンポジションから削除します。
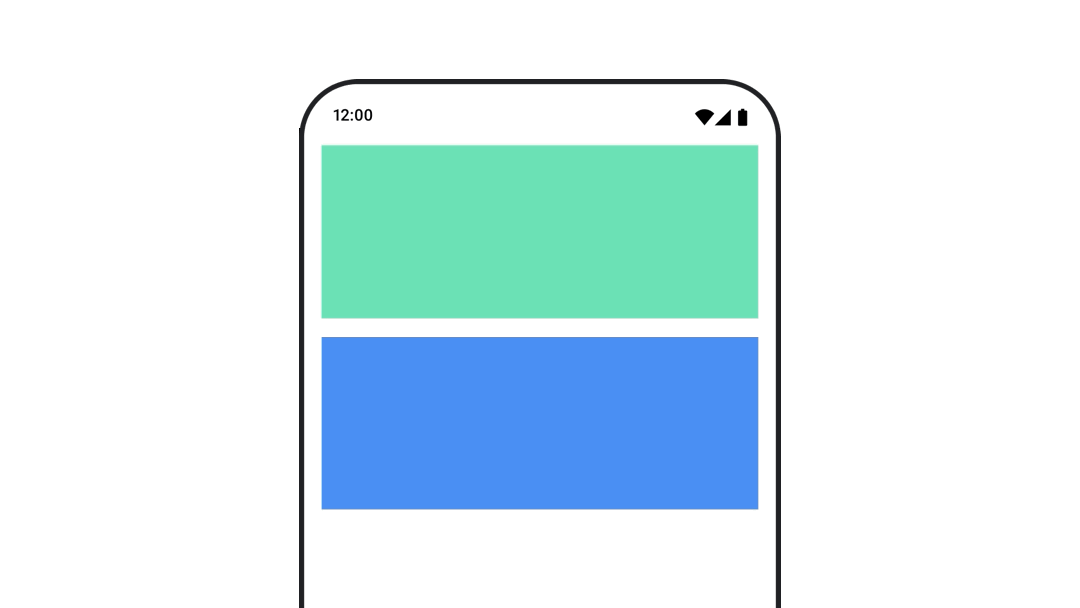
背景色をアニメーション化する
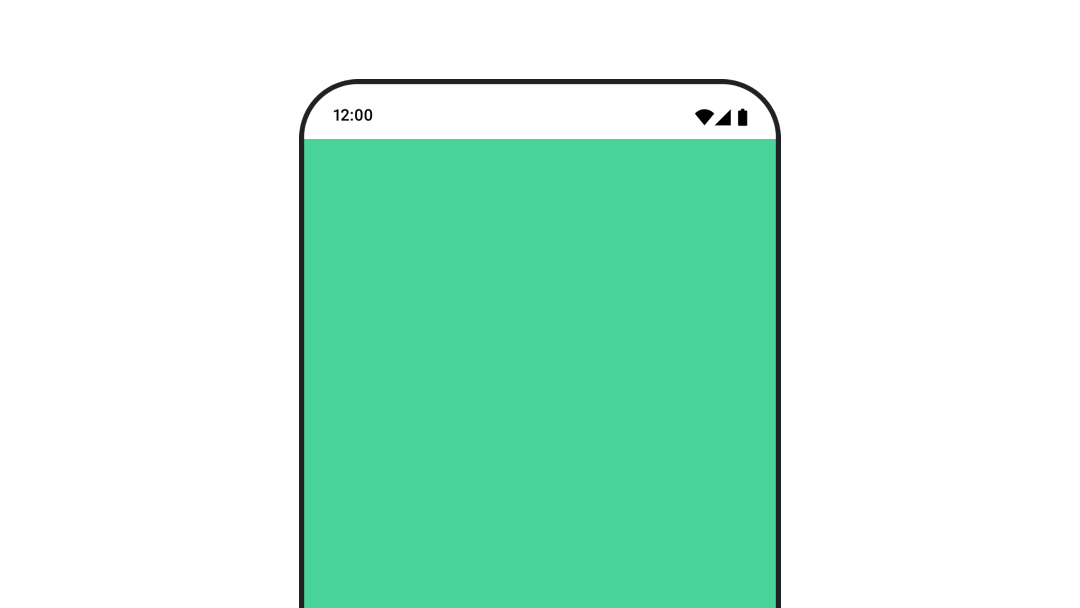
val animatedColor by animateColorAsState( if (animateBackgroundColor) colorGreen else colorBlue, label = "color" ) Column( modifier = Modifier.drawBehind { drawRect(animatedColor) } ) { // your composable here }
このオプションは、Modifier.background()
を使用するよりもパフォーマンスが高くなります。Modifier.background()
は 1 回限りの色設定には適していますが、時間の経過とともに色をアニメーション化する場合は、必要以上に再コンポーズが発生する可能性があります。
背景色を無限にアニメーション化する方法については、アニメーションの繰り返しをご覧ください。
コンポーザブルのサイズをアニメーション化する
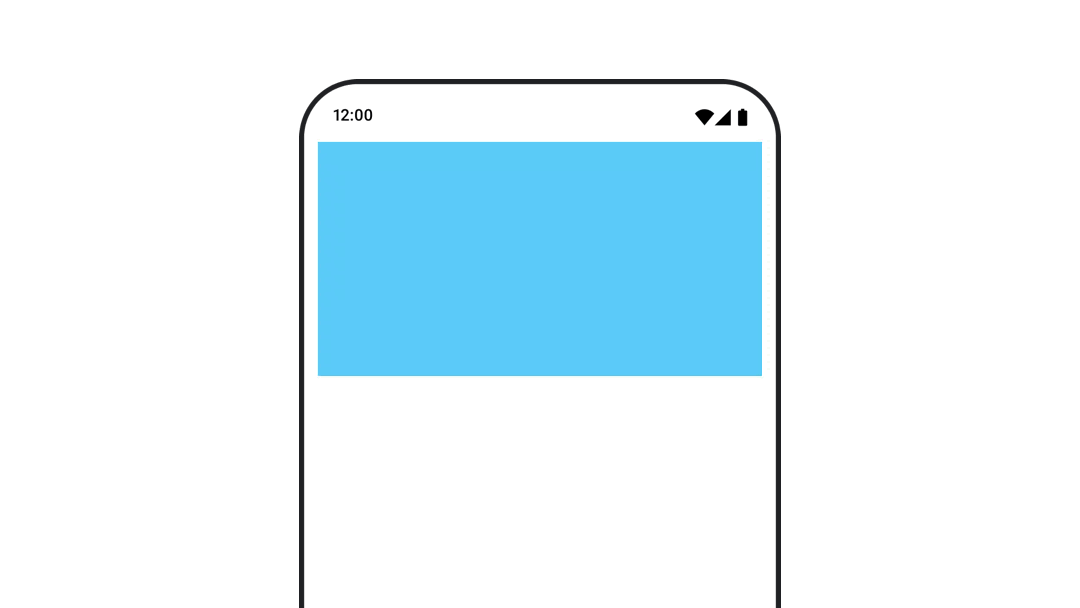
Compose では、コンポーザブルのサイズをいくつかの方法でアニメーション化できます。コンポーザブルのサイズ変更間のアニメーションには animateContentSize()
を使用します。
たとえば、1 行から複数行に拡張できるテキストを含むボックスがある場合は、Modifier.animateContentSize()
を使用してスムーズな遷移を実現できます。
var expanded by remember { mutableStateOf(false) } Box( modifier = Modifier .background(colorBlue) .animateContentSize() .height(if (expanded) 400.dp else 200.dp) .fillMaxWidth() .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { expanded = !expanded } ) { }
AnimatedContent
と SizeTransform
を使用して、サイズ変更を行う方法を記述することもできます。
コンポーザブルの位置をアニメーション化する
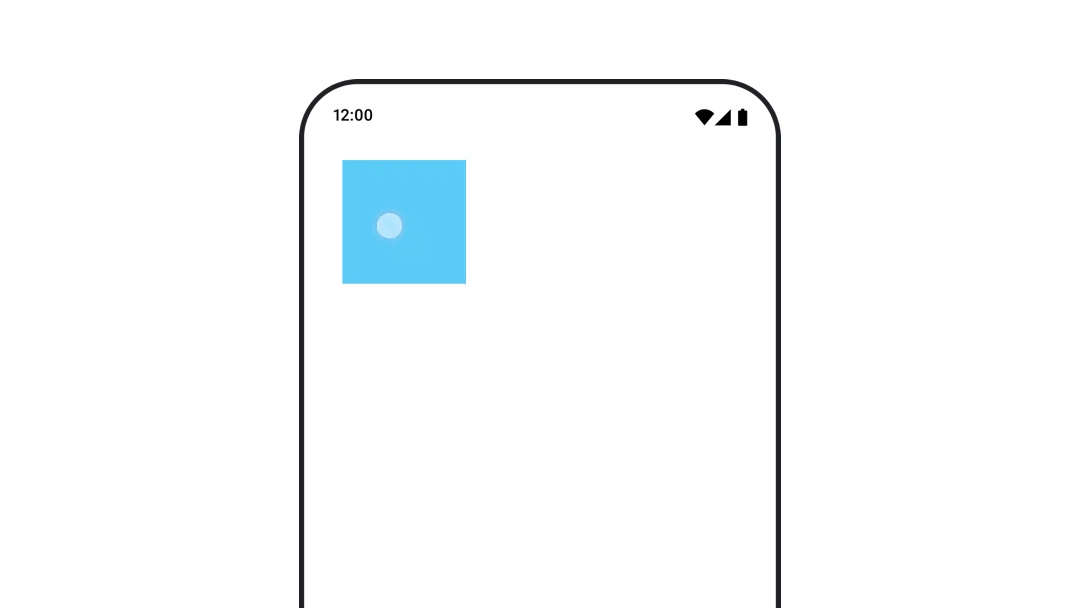
コンポーザブルの位置をアニメーション化するには、Modifier.offset{ }
と animateIntOffsetAsState()
を組み合わせて使用します。
var moved by remember { mutableStateOf(false) } val pxToMove = with(LocalDensity.current) { 100.dp.toPx().roundToInt() } val offset by animateIntOffsetAsState( targetValue = if (moved) { IntOffset(pxToMove, pxToMove) } else { IntOffset.Zero }, label = "offset" ) Box( modifier = Modifier .offset { offset } .background(colorBlue) .size(100.dp) .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { moved = !moved } )
位置やサイズをアニメーション化するときに、コンポーザブルが他のコンポーザブルの上に重ねて描画されないようにするには、Modifier.layout{ }
を使用します。この修飾子は、サイズと位置の変更を親に伝播し、他の子に影響します。
たとえば、Column
内で Box
を移動し、Box
の移動時に他の子を移動する必要がある場合は、次のように Modifier.layout{ }
にオフセット情報を含めます。
var toggled by remember { mutableStateOf(false) } val interactionSource = remember { MutableInteractionSource() } Column( modifier = Modifier .padding(16.dp) .fillMaxSize() .clickable(indication = null, interactionSource = interactionSource) { toggled = !toggled } ) { val offsetTarget = if (toggled) { IntOffset(150, 150) } else { IntOffset.Zero } val offset = animateIntOffsetAsState( targetValue = offsetTarget, label = "offset" ) Box( modifier = Modifier .size(100.dp) .background(colorBlue) ) Box( modifier = Modifier .layout { measurable, constraints -> val offsetValue = if (isLookingAhead) offsetTarget else offset.value val placeable = measurable.measure(constraints) layout(placeable.width + offsetValue.x, placeable.height + offsetValue.y) { placeable.placeRelative(offsetValue) } } .size(100.dp) .background(colorGreen) ) Box( modifier = Modifier .size(100.dp) .background(colorBlue) ) }
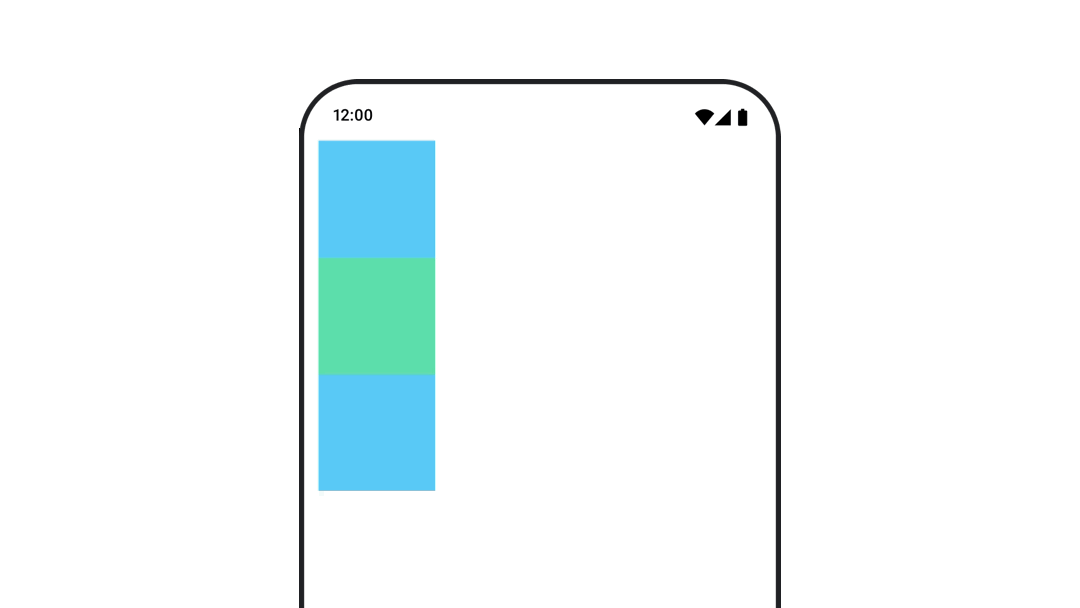
Modifier.layout{ }
によるアニメーション化コンポーザブルのパディングをアニメーション化する
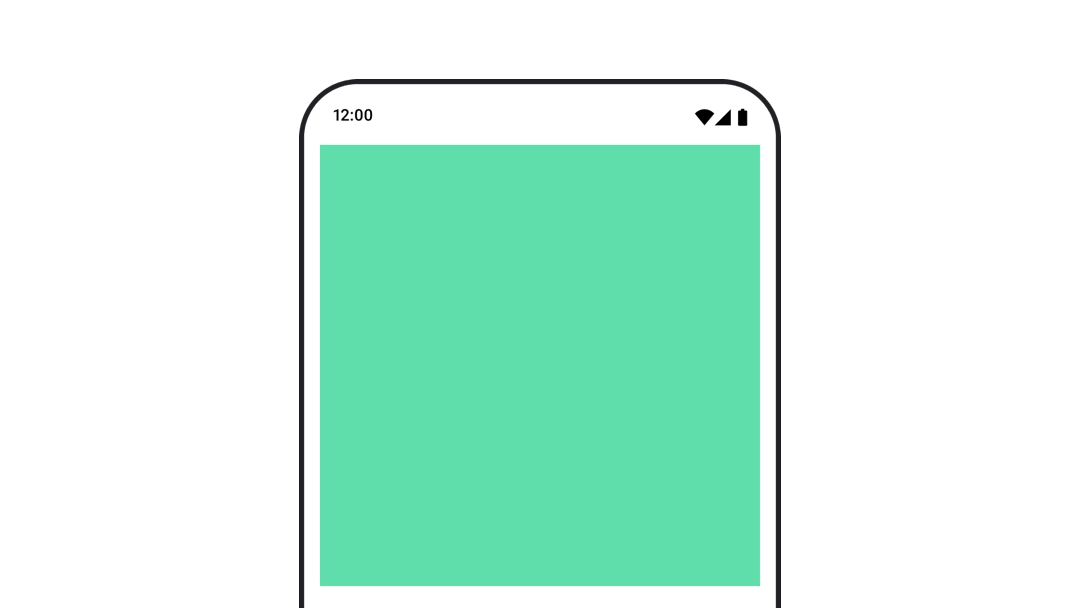
コンポーザブルのパディングをアニメーション化するには、animateDpAsState
と Modifier.padding()
を組み合わせて使用します。
var toggled by remember { mutableStateOf(false) } val animatedPadding by animateDpAsState( if (toggled) { 0.dp } else { 20.dp }, label = "padding" ) Box( modifier = Modifier .aspectRatio(1f) .fillMaxSize() .padding(animatedPadding) .background(Color(0xff53D9A1)) .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { toggled = !toggled } )
コンポーザブルのアニメーション化
コンポーザブルのエレベーションをアニメーション化するには、animateDpAsState
と Modifier.graphicsLayer{ }
を組み合わせて使用します。標高の 1 回限りの変更には Modifier.shadow()
を使用します。シャドウをアニメーション化する場合は、Modifier.graphicsLayer{ }
修飾子を使用するとパフォーマンスが向上します。
val mutableInteractionSource = remember { MutableInteractionSource() } val pressed = mutableInteractionSource.collectIsPressedAsState() val elevation = animateDpAsState( targetValue = if (pressed.value) { 32.dp } else { 8.dp }, label = "elevation" ) Box( modifier = Modifier .size(100.dp) .align(Alignment.Center) .graphicsLayer { this.shadowElevation = elevation.value.toPx() } .clickable(interactionSource = mutableInteractionSource, indication = null) { } .background(colorGreen) ) { }
または、Card
コンポーザブルを使用して、状態ごとに異なる値にエレベーション プロパティを設定します。
テキストの拡大縮小、変換、回転をアニメーション化する
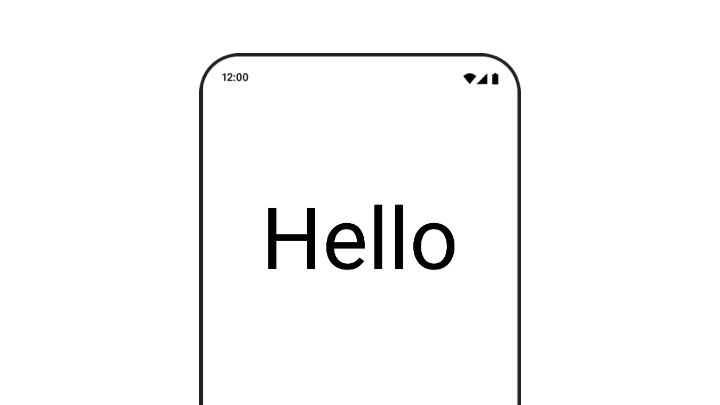
テキストのスケール、移動、回転をアニメーション化する場合は、TextStyle
の textMotion
パラメータを TextMotion.Animated
に設定します。これにより、テキスト アニメーション間の遷移がスムーズになります。Modifier.graphicsLayer{ }
を使用して、テキストを移動、回転、拡大縮小します。
val infiniteTransition = rememberInfiniteTransition(label = "infinite transition") val scale by infiniteTransition.animateFloat( initialValue = 1f, targetValue = 8f, animationSpec = infiniteRepeatable(tween(1000), RepeatMode.Reverse), label = "scale" ) Box(modifier = Modifier.fillMaxSize()) { Text( text = "Hello", modifier = Modifier .graphicsLayer { scaleX = scale scaleY = scale transformOrigin = TransformOrigin.Center } .align(Alignment.Center), // Text composable does not take TextMotion as a parameter. // Provide it via style argument but make sure that we are copying from current theme style = LocalTextStyle.current.copy(textMotion = TextMotion.Animated) ) }
テキストの色をアニメーション化する
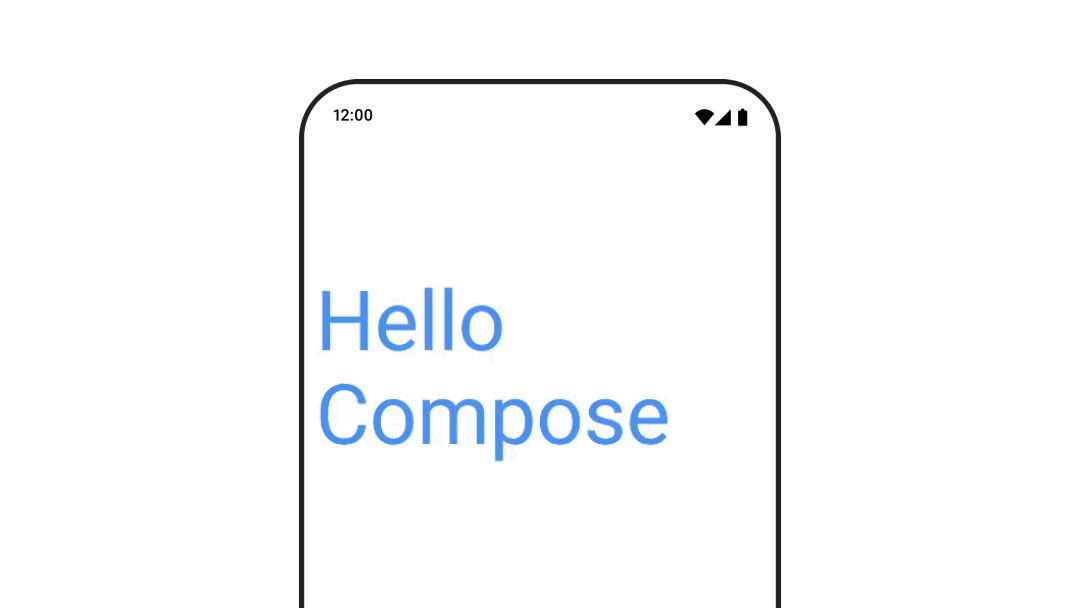
テキストの色をアニメーション化するには、BasicText
コンポーザブルで color
ラムダを使用します。
val infiniteTransition = rememberInfiniteTransition(label = "infinite transition") val animatedColor by infiniteTransition.animateColor( initialValue = Color(0xFF60DDAD), targetValue = Color(0xFF4285F4), animationSpec = infiniteRepeatable(tween(1000), RepeatMode.Reverse), label = "color" ) BasicText( text = "Hello Compose", color = { animatedColor }, // ... )
さまざまな種類のコンテンツを切り替える
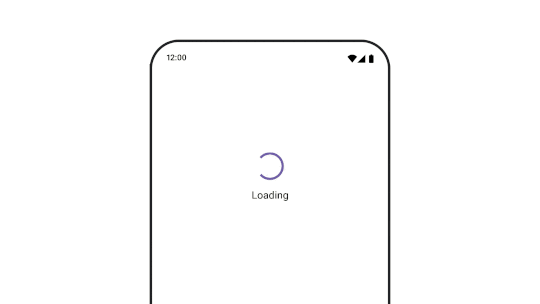
異なるコンポーザブル間でアニメーション化するには AnimatedContent
を使用します。コンポーザブル間で標準的なフェードだけを希望する場合は、Crossfade
を使用します。
var state by remember { mutableStateOf(UiState.Loading) } AnimatedContent( state, transitionSpec = { fadeIn( animationSpec = tween(3000) ) togetherWith fadeOut(animationSpec = tween(3000)) }, modifier = Modifier.clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { state = when (state) { UiState.Loading -> UiState.Loaded UiState.Loaded -> UiState.Error UiState.Error -> UiState.Loading } }, label = "Animated Content" ) { targetState -> when (targetState) { UiState.Loading -> { LoadingScreen() } UiState.Loaded -> { LoadedScreen() } UiState.Error -> { ErrorScreen() } } }
AnimatedContent
は、さまざまな種類の開始遷移と終了遷移を表示するようにカスタマイズできます。詳しくは、AnimatedContent
のドキュメントまたは、AnimatedContent
に関するこちらのブログ投稿をご覧ください。
別のデスティネーションに移動するときにアニメーション化する
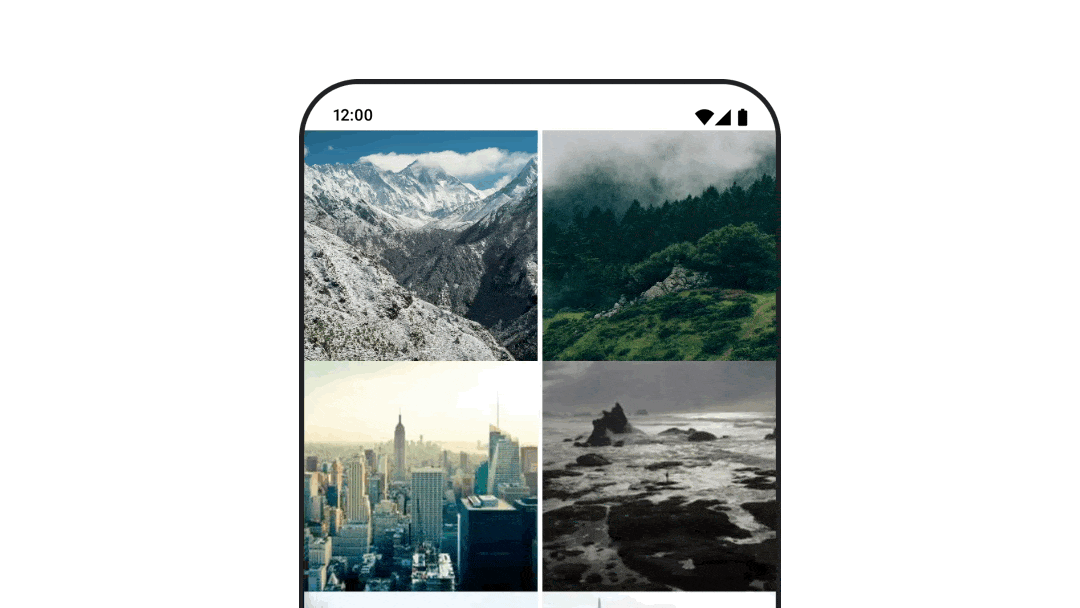
navigation-compose アーティファクトを使用するときにコンポーザブル間の遷移をアニメーション化するには、コンポーザブルに enterTransition
と exitTransition
を指定します。最上位の NavHost
で、すべてのデスティネーションに使用するデフォルトのアニメーションを設定することもできます。
val navController = rememberNavController() NavHost( navController = navController, startDestination = "landing", enterTransition = { EnterTransition.None }, exitTransition = { ExitTransition.None } ) { composable("landing") { ScreenLanding( // ... ) } composable( "detail/{photoUrl}", arguments = listOf(navArgument("photoUrl") { type = NavType.StringType }), enterTransition = { fadeIn( animationSpec = tween( 300, easing = LinearEasing ) ) + slideIntoContainer( animationSpec = tween(300, easing = EaseIn), towards = AnimatedContentTransitionScope.SlideDirection.Start ) }, exitTransition = { fadeOut( animationSpec = tween( 300, easing = LinearEasing ) ) + slideOutOfContainer( animationSpec = tween(300, easing = EaseOut), towards = AnimatedContentTransitionScope.SlideDirection.End ) } ) { backStackEntry -> ScreenDetails( // ... ) } }
入場と退場の切り替えにはさまざまな種類があり、受信コンテンツと送信コンテンツに異なる効果を適用できます。詳しくは、ドキュメントをご覧ください。
アニメーションを繰り返す
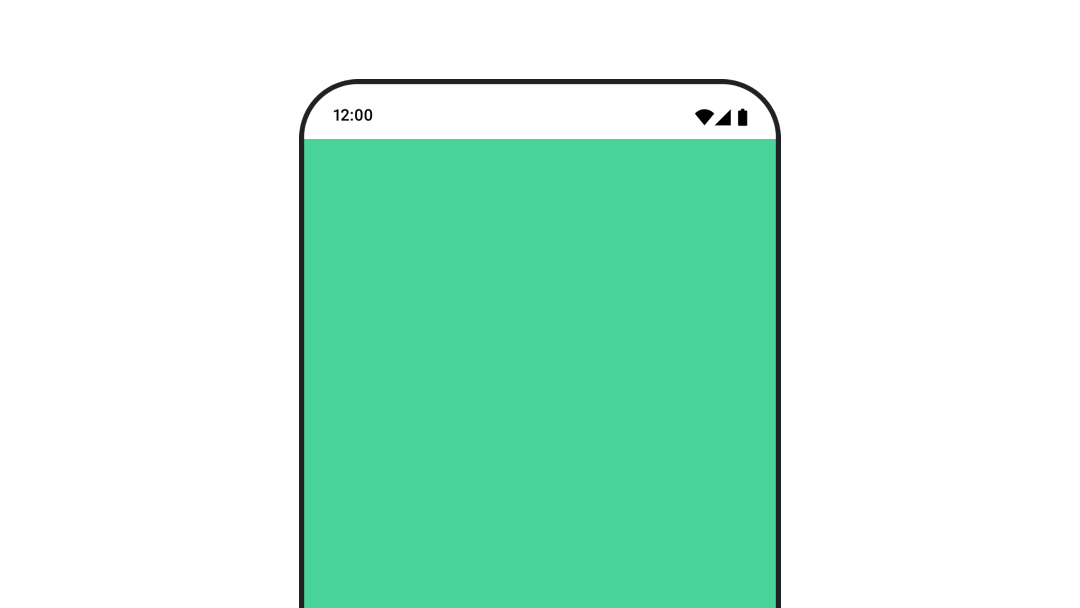
infiniteRepeatable
animationSpec
で rememberInfiniteTransition
を使用して、アニメーションを連続で繰り返します。RepeatModes
を変更して、前後に移動する方法を指定します。
finiteRepeatable
を使用して、指定した回数だけ繰り返します。
val infiniteTransition = rememberInfiniteTransition(label = "infinite") val color by infiniteTransition.animateColor( initialValue = Color.Green, targetValue = Color.Blue, animationSpec = infiniteRepeatable( animation = tween(1000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "color" ) Column( modifier = Modifier.drawBehind { drawRect(color) } ) { // your composable here }
コンポーザブルの起動時にアニメーションを開始する
LaunchedEffect
は、コンポーザブルがコンポジションに入るときに実行されます。コンポーザブルの起動時にアニメーションを開始します。これを使用して、アニメーションの状態変化を駆動できます。animateTo
メソッドで Animatable
を使用して、起動時にアニメーションを開始します。
val alphaAnimation = remember { Animatable(0f) } LaunchedEffect(Unit) { alphaAnimation.animateTo(1f) } Box( modifier = Modifier.graphicsLayer { alpha = alphaAnimation.value } )
連続アニメーションを作成する
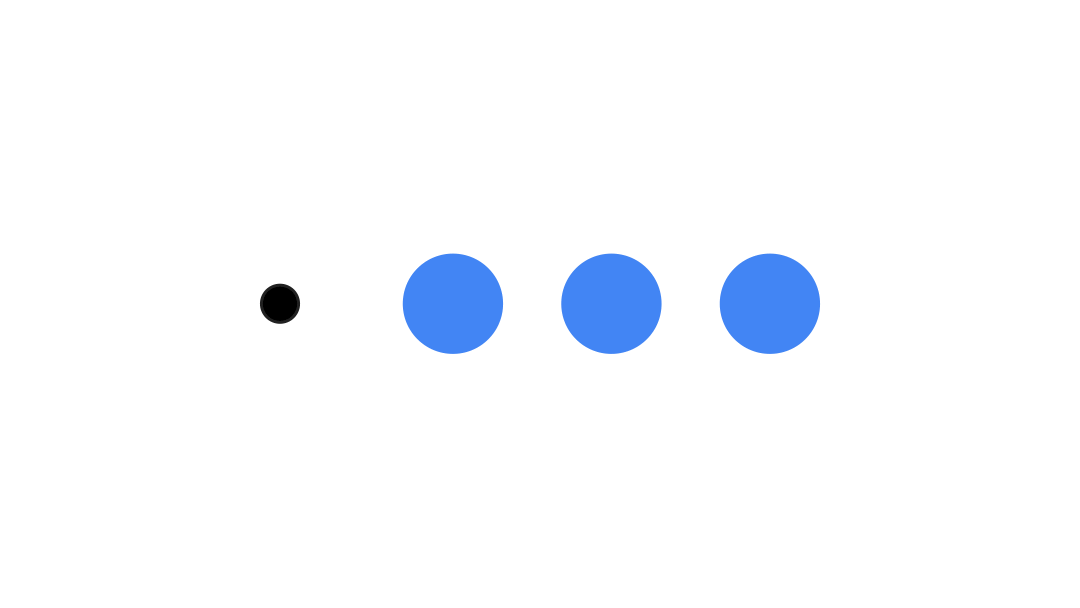
Animatable
コルーチン API を使用して、連続または同時のアニメーションを実行します。Animatable
で animateTo
を順番に呼び出すと、各アニメーションは前のアニメーションが完了するまで待ってから続行します。これは、suspend 関数であるためです。
val alphaAnimation = remember { Animatable(0f) } val yAnimation = remember { Animatable(0f) } LaunchedEffect("animationKey") { alphaAnimation.animateTo(1f) yAnimation.animateTo(100f) yAnimation.animateTo(500f, animationSpec = tween(100)) }
同時実行アニメーションを作成する
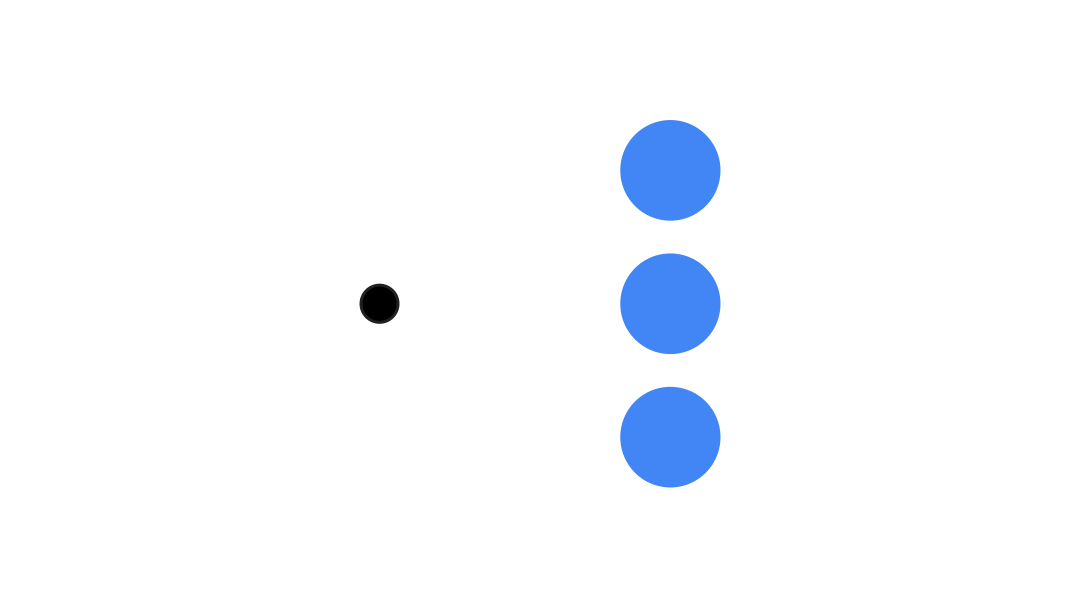
コルーチン API(Animatable#animateTo()
または animate
)または Transition
API を使用して、アニメーションを同時に実行します。コルーチン コンテキストで複数の起動関数を使用すると、アニメーションが同時に起動します。
val alphaAnimation = remember { Animatable(0f) } val yAnimation = remember { Animatable(0f) } LaunchedEffect("animationKey") { launch { alphaAnimation.animateTo(1f) } launch { yAnimation.animateTo(100f) } }
updateTransition
API を使用すると、同じ状態を使用して、さまざまなプロパティ アニメーションを同時に駆動できます。次の例では、状態変化によって制御される 2 つのプロパティ(rect
と borderWidth
)をアニメーション化します。
var currentState by remember { mutableStateOf(BoxState.Collapsed) } val transition = updateTransition(currentState, label = "transition") val rect by transition.animateRect(label = "rect") { state -> when (state) { BoxState.Collapsed -> Rect(0f, 0f, 100f, 100f) BoxState.Expanded -> Rect(100f, 100f, 300f, 300f) } } val borderWidth by transition.animateDp(label = "borderWidth") { state -> when (state) { BoxState.Collapsed -> 1.dp BoxState.Expanded -> 0.dp } }
アニメーションのパフォーマンスを最適化する
Compose のアニメーションはパフォーマンスの問題を引き起こす可能性があります。これは、アニメーションの性質によるものです。アニメーションとは、動きの錯覚を作り出すために、画面上のピクセルをフレームごとにすばやく移動または変更することです。
Compose のさまざまなフェーズ(コンポーズ、レイアウト、描画)について考えてみましょう。アニメーションでレイアウト フェーズが変更される場合は、影響を受けるすべてのコンポーザブルを再レイアウトして再描画する必要があります。アニメーションが描画フェーズで発生する場合、デフォルトでは、レイアウト フェーズでアニメーションを実行する場合よりもパフォーマンスが向上します。これは、全体的な作業量が減るためです。
アニメーション中にアプリが行う処理を最小限に抑えるため、可能であれば Modifier
のラムダ バージョンを選択してください。これにより、再コンポーズがスキップされ、コンポーズ フェーズ外でアニメーションが実行されます。そうでない場合は、この修飾子は常に描画フェーズで実行されるため、Modifier.graphicsLayer{ }
を使用します。詳細については、パフォーマンス ドキュメントの読み取りの延期をご覧ください。
アニメーションのタイミングを変更する
Compose では、デフォルトでほとんどのアニメーションにスプリング アニメーションが使用されます。バネや物理ベースのアニメーションはより自然に感じられます。また、一定の時間ではなくオブジェクトの現在の速度を考慮するため、中断することもできます。デフォルトをオーバーライドする場合は、上記のアニメーション API すべてで animationSpec
を設定して、アニメーションの実行方法をカスタマイズできます。たとえば、特定の期間にわたって実行する、弾むようにするなどです。
さまざまな animationSpec
オプションの概要は次のとおりです。
spring
: 物理ベースのアニメーション。すべてのアニメーションのデフォルトです。stiffness または dampingRatio を変更して、アニメーションの外観を変更できます。tween
(between の略): 時間ベースのアニメーション。Easing
関数を使用して 2 つの値間でアニメーション化します。keyframes
: アニメーションの特定のキーポイントの値を指定する仕様。repeatable
:RepeatMode
で指定された一定回数実行される時間ベースの spec。infiniteRepeatable
: 永続的に実行される時間ベースの spec。snap
: アニメーションなしで最終値に即座にスナップします。
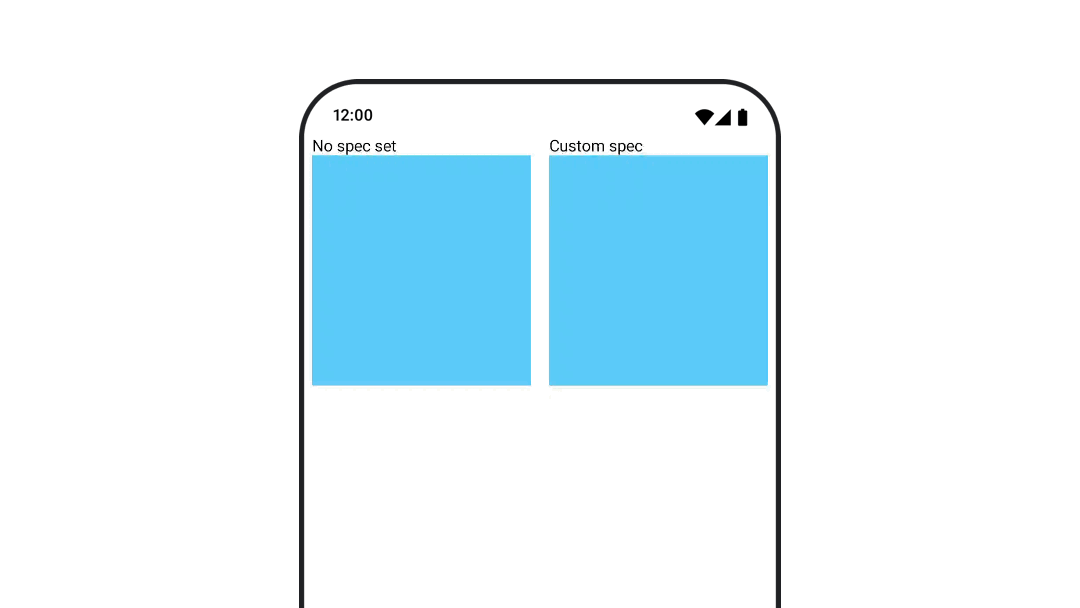
animationSpecs の詳細については、ドキュメントをご覧ください。
参考情報
Compose で作成できる楽しいアニメーションの例については、以下をご覧ください。
- Compose の 5 つのクイック アニメーション
- Compose で Jellyfish を動かす
- Compose での
AnimatedContent
のカスタマイズ - Compose でのイージング関数のイージング