רשימות מאפשרות למשתמשים לבחור בקלות פריט מתוך קבוצת אפשרויות במכשירי Wear OS.
ספריית ממשק המשתמש הלביש כוללת את
WearableRecyclerView
, שהיא
RecyclerView
להטמעת רשימות שמותאמות למכשירים לבישים. אפשר להשתמש
בממשק של האפליקציה הלבישת באמצעות יצירה של מאגר WearableRecyclerView
חדש.
שימוש ב-WearableRecyclerView
עבור
רשימה ארוכה של פריטים פשוטים, כמו מרכז האפליקציות או רשימה של אנשי קשר. כל פריט
יש מחרוזת קצרה וסמל משויך. לחלופין, לכל פריט יכול להיות רק מחרוזת
או סמל.
הערה: יש להימנע מפריסות מורכבות. המשתמשים צריכים רק להביט בפריט כדי להבין במה מדובר, במיוחד עם גאדג'טים לבישים גודל המסך מוגבל.
על ידי הרחבה של כיתה קיימת RecyclerView
, WearableRecyclerView
כברירת מחדל, ממשקי API מציגים רשימת פריטים שאפשר לגלול אנכית ברשימה ישרה. אפשר גם להשתמש
את ממשקי ה-API של WearableRecyclerView
כדי להביע הסכמה לפריסה מעוקלת
תנועת גלילה מעגלית
באפליקציות הלבישות.
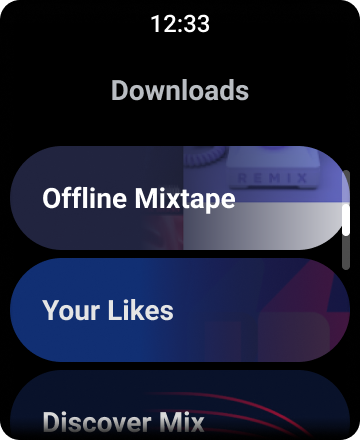
איור 1. תצוגת הרשימה שמוגדרת כברירת מחדל ב-Wear OS.
במדריך הזה מוסבר איך להשתמש בכיתה WearableRecyclerView
כדי ליצור
רשימות באפליקציות ל-Wear OS, איך מצטרפים לפריסה מעוקלת
של הפריטים שניתנים לגלילה, ואיך להתאים אישית את המראה שלהם
הילדים גוללים.
הוספת WearableRecyclerView לפעילות באמצעות XML
הפריסה הבאה מוסיפה WearableRecyclerView
לפעילות:
<androidx.wear.widget.WearableRecyclerView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/recycler_launcher_view" android:layout_width="match_parent" android:layout_height="match_parent" android:scrollbars="vertical" />
הדוגמה הבאה מראה את שדה ה-WearableRecyclerView
הוחלו על פעילות:
Kotlin
class MainActivity : Activity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) } ... }
Java
public class MainActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } ... }
יצירת פריסה מעוקלת
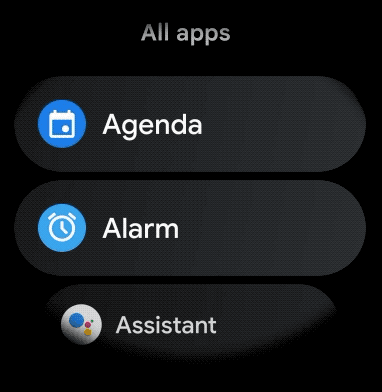
כדי ליצור פריסה מעוקלת של פריטים שניתנים לגלילה באפליקציה הלבישה, צריך לבצע את הפעולות הבאות:
-
שימוש ב-
WearableRecyclerView
בתור הקונטיינר הראשי בפריסת ה-XML הרלוונטית. -
מגדירים את
setEdgeItemsCenteringEnabled(boolean)
לtrue
. הזה הפריט הראשון והאחרון מופיעים ברשימה וממרכזים אותם באופן אנכי. -
משתמשים בשיטה
WearableRecyclerView.setLayoutManager()
כדי להגדיר את הפריסה של פריטים במסך.
Kotlin
wearableRecyclerView.apply { // To align the edge children (first and last) with the center of the screen. isEdgeItemsCenteringEnabled = true ... layoutManager = WearableLinearLayoutManager(this@MainActivity) }
Java
// To align the edge children (first and last) with the center of the screen. wearableRecyclerView.setEdgeItemsCenteringEnabled(true); ... wearableRecyclerView.setLayoutManager( new WearableLinearLayoutManager(this));
אם לאפליקציה שלך יש דרישות ספציפיות להתאמה אישית של המראה של הילדים בזמן גלילה – לדוגמה,
שינוי קנה המידה של הסמלים והטקסט בזמן שהפריטים נגללים מהמרכז — הרחבה
ה
הכיתה WearableLinearLayoutManager.LayoutCallback
ומשנים את הערך
onLayoutFinished
.
קטעי הקוד הבאים מציגים דוגמה להתאמה אישית של גלילה של פריטים בקנה מידה נרחב
רחוקים מהמרכז על ידי הרחבת
כיתה אחת (WearableLinearLayoutManager.LayoutCallback
):
Kotlin
/** How much icons should scale, at most. */ private const val MAX_ICON_PROGRESS = 0.65f class CustomScrollingLayoutCallback : WearableLinearLayoutManager.LayoutCallback() { private var progressToCenter: Float = 0f override fun onLayoutFinished(child: View, parent: RecyclerView) { child.apply { // Figure out % progress from top to bottom. val centerOffset = height.toFloat() / 2.0f / parent.height.toFloat() val yRelativeToCenterOffset = y / parent.height + centerOffset // Normalize for center. progressToCenter = Math.abs(0.5f - yRelativeToCenterOffset) // Adjust to the maximum scale. progressToCenter = Math.min(progressToCenter, MAX_ICON_PROGRESS) scaleX = 1 - progressToCenter scaleY = 1 - progressToCenter } } }
Java
public class CustomScrollingLayoutCallback extends WearableLinearLayoutManager.LayoutCallback { /** How much icons should scale, at most. */ private static final float MAX_ICON_PROGRESS = 0.65f; private float progressToCenter; @Override public void onLayoutFinished(View child, RecyclerView parent) { // Figure out % progress from top to bottom. float centerOffset = ((float) child.getHeight() / 2.0f) / (float) parent.getHeight(); float yRelativeToCenterOffset = (child.getY() / parent.getHeight()) + centerOffset; // Normalize for center. progressToCenter = Math.abs(0.5f - yRelativeToCenterOffset); // Adjust to the maximum scale. progressToCenter = Math.min(progressToCenter, MAX_ICON_PROGRESS); child.setScaleX(1 - progressToCenter); child.setScaleY(1 - progressToCenter); } }
Kotlin
wearableRecyclerView.layoutManager = WearableLinearLayoutManager(this, CustomScrollingLayoutCallback())
Java
CustomScrollingLayoutCallback customScrollingLayoutCallback = new CustomScrollingLayoutCallback(); wearableRecyclerView.setLayoutManager( new WearableLinearLayoutManager(this, customScrollingLayoutCallback));