應用程式列可讓您新增使用者動作按鈕。這項功能可讓您針對目前結構定義,將最重要的「動作」放在應用程式頂端。舉例來說,相片瀏覽應用程式可能會在使用者查看相片膠卷時,在頂端顯示「分享」和「建立專輯」按鈕。使用者查看個別相片時,應用程式可能會顯示「裁剪」和「濾鏡」按鈕。
應用程式列空間有限,如果應用程式宣告的動作超過應用程式列所能容納的動作數量,應用程式列會將多餘的動作傳送至「溢位」選單。應用程式也可以指定動作一律顯示在溢位選單中,而不是在應用程式列顯示。
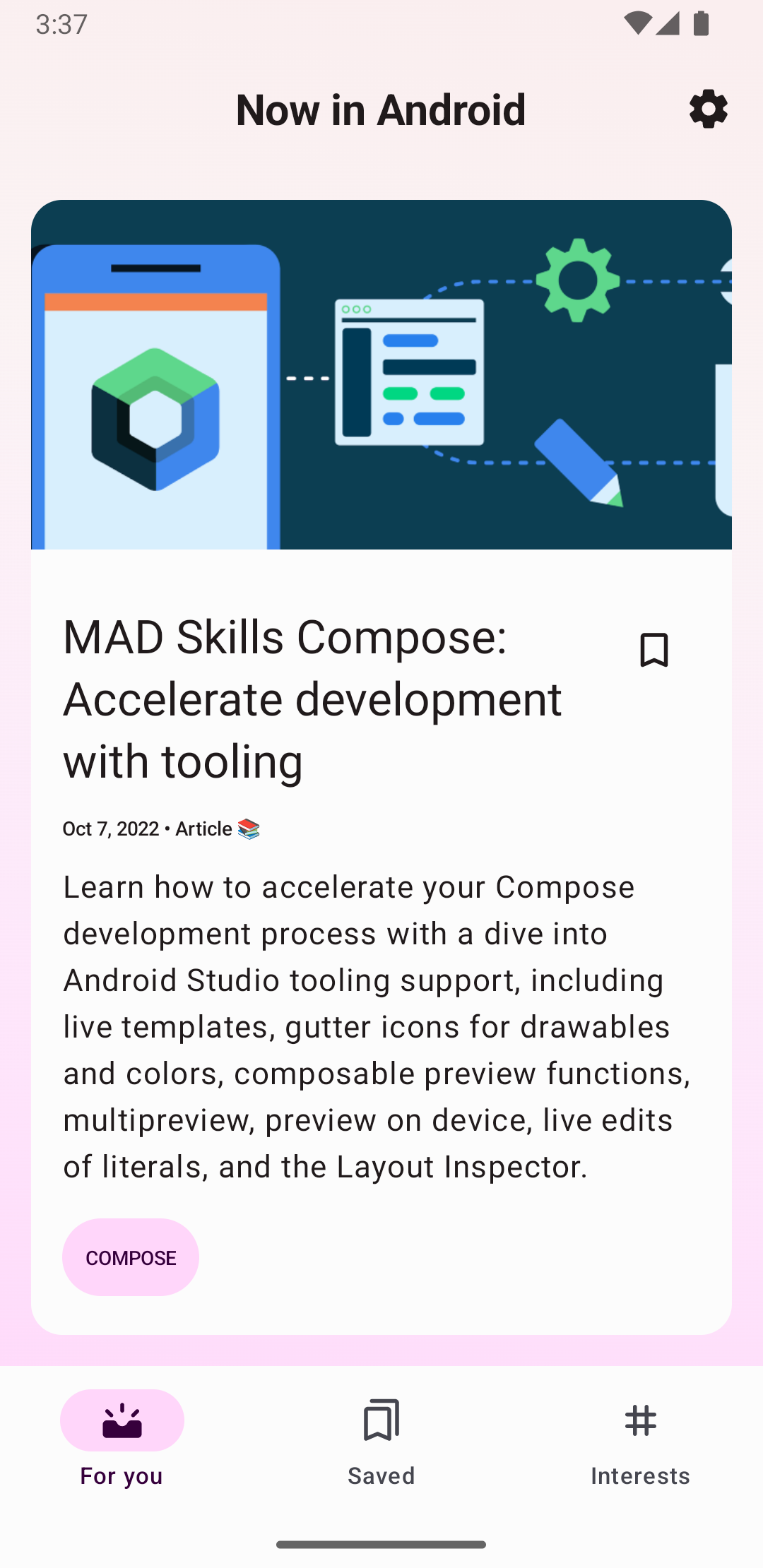
新增動作按鈕
動作溢位中的所有動作按鈕和其他項目都是在 XML 選單資源中定義。如要在動作列新增動作,請在專案的 res/menu/
目錄中建立新的 XML 檔案。
請為要加入動作列的每個項目新增 <item>
元素,如以下選單 XML 檔案範例所示:
<menu xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto"> <!-- "Mark Favorite", must appear as action button if possible. --> <item android:id="@+id/action_favorite" android:icon="@drawable/ic_favorite_black_48dp" android:title="@string/action_favorite" app:showAsAction="ifRoom"/> <!-- Settings, must always be in the overflow. --> <item android:id="@+id/action_settings" android:title="@string/action_settings" app:showAsAction="never"/> </menu>
app:showAsAction
屬性可指定動作是否在應用程式列上顯示為按鈕。如果您設定了 app:showAsAction="ifRoom"
(如範例程式碼的 favorite 動作所示),如果應用程式列中有空間,動作就會顯示為按鈕。如果空間不足,系統會將超額操作傳送至溢位選單。如果您在程式碼範例的 設定 動作中設定了 app:showAsAction="never"
,該動作一律會列在溢位選單中,不會顯示在應用程式列中。
如果動作顯示在應用程式列中,系統就會使用動作圖示做為動作按鈕。您可以在 Material Design 圖示中找到許多實用圖示。
回應動作
當使用者選取其中一個應用程式列項目時,系統會呼叫活動的 onOptionsItemSelected()
回呼方法,並傳遞 MenuItem
物件來指出輕觸的項目。在 onOptionsItemSelected()
實作中,呼叫 MenuItem.getItemId()
方法來判斷輕觸的項目。傳回的 ID 與您在對應 <item>
元素的 android:id
屬性中宣告的值相符。
舉例來說,下列程式碼片段會檢查使用者選取的動作。如果這個方法無法辨識使用者的動作,會叫用父類別方法:
Kotlin
override fun onOptionsItemSelected(item: MenuItem) = when (item.itemId) { R.id.action_settings -> { // User chooses the "Settings" item. Show the app settings UI. true } R.id.action_favorite -> { // User chooses the "Favorite" action. Mark the current item as a // favorite. true } else -> { // The user's action isn't recognized. // Invoke the superclass to handle it. super.onOptionsItemSelected(item) } }
Java
@Override public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()) { case R.id.action_settings: // User chooses the "Settings" item. Show the app settings UI. return true; case R.id.action_favorite: // User chooses the "Favorite" action. Mark the current item as a // favorite. return true; default: // The user's action isn't recognized. // Invoke the superclass to handle it. return super.onOptionsItemSelected(item); } }