İletişim kutusu, kullanıcının bir karar verebilir veya ek bilgiler girebilirsiniz. İletişim kutusu ekranı kaplamıyor ve Normalde, kullanıcıların uygulamanızı indirmeden önce bir işlem yapmasını gerektiren kalıcı etkinlikler için devam edebilir.
ziyaret edin.Dialog
sınıfı, iletişim kutuları için temel sınıftır ancak Dialog
örneğini göstermeyin.
doğrudan ekleyebilirsiniz. Bunun yerine aşağıdaki alt sınıflardan birini kullanın:
AlertDialog
- Bir başlık, en fazla üç düğme ve seçilebilir bir liste gösteren iletişim kutusu veya özel bir düzen kullanabilirsiniz.
DatePickerDialog
. veyaTimePickerDialog
- Kullanıcının tarih seçmesini sağlayan, önceden tanımlanmış kullanıcı arayüzünün bulunduğu iletişim kutusu gerekir. ziyaret edin.
Bu sınıflar diyaloğunuzun stilini ve yapısını tanımlar. Ayrıca şunlara da ihtiyacınız vardır:
CANNOT TRANSLATE
DialogFragment
.
iletişim kutunuz için bir kapsayıcı olarak kullanın. DialogFragment
sınıfı şunları sağlar:
iletişim kutunuzu oluşturmak ve görünümünü yönetmek için ihtiyacınız olan tüm
yerine Dialog
nesnesinde yöntemler çağırmaktır.
İletişim kutusunu yönetmek için DialogFragment
kullanılması, iletişimin doğru bir şekilde yapılmasını sağlar
Kullanıcının Geri düğmesine dokunması veya cihazı döndürmesi gibi yaşam döngüsü olaylarını ele alma
ekranda görebilirsiniz. DialogFragment
sınıfı, şu öğeleri yeniden kullanmanıza da olanak tanır:
daha büyük bir kullanıcı arayüzünde yerleştirilebilir bir bileşen olarak gösteren
geleneksel
Fragment
—
Böylece, iletişim kutusu kullanıcı arayüzünün
büyük ve küçük boyutlarda
ekranları.
Bu dokümanın aşağıdaki bölümlerinde, bir
Bir AlertDialog
ile birlikte DialogFragment
nesnesini tanımlayın. Bir tarih veya saat seçici oluşturmak istiyorsanız
uygulamasında gösterilir.
İletişim kutusu parçası oluşturma
Özel alanlar da dahil olmak üzere çok çeşitli iletişim
ve aynı zamanda
Materyal Tasarım
Diyaloglar: DialogFragment
uzantısını genişletip
AlertDialog
onCreateDialog()
geri çağırma yöntemini kullanın.
Örneğin, burada yönetilen bir temel AlertDialog
DialogFragment
:
Kotlin
class StartGameDialogFragment : DialogFragment() { override fun onCreateDialog(savedInstanceState: Bundle?): Dialog { return activity?.let { // Use the Builder class for convenient dialog construction. val builder = AlertDialog.Builder(it) builder.setMessage("Start game") .setPositiveButton("Start") { dialog, id -> // START THE GAME! } .setNegativeButton("Cancel") { dialog, id -> // User cancelled the dialog. } // Create the AlertDialog object and return it. builder.create() } ?: throw IllegalStateException("Activity cannot be null") } } class OldXmlActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_old_xml) StartGameDialogFragment().show(supportFragmentManager, "GAME_DIALOG") } }
Java
public class StartGameDialogFragment extends DialogFragment { @Override public Dialog onCreateDialog(Bundle savedInstanceState) { // Use the Builder class for convenient dialog construction. AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); builder.setMessage(R.string.dialog_start_game) .setPositiveButton(R.string.start, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // START THE GAME! } }) .setNegativeButton(R.string.cancel, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // User cancels the dialog. } }); // Create the AlertDialog object and return it. return builder.create(); } } // ... StartGameDialogFragment().show(supportFragmentManager, "GAME_DIALOG");
Bu sınıfın bir örneğini oluşturup
show()
.
iletişim kutusu aşağıdaki şekilde gösterildiği gibi görünür.
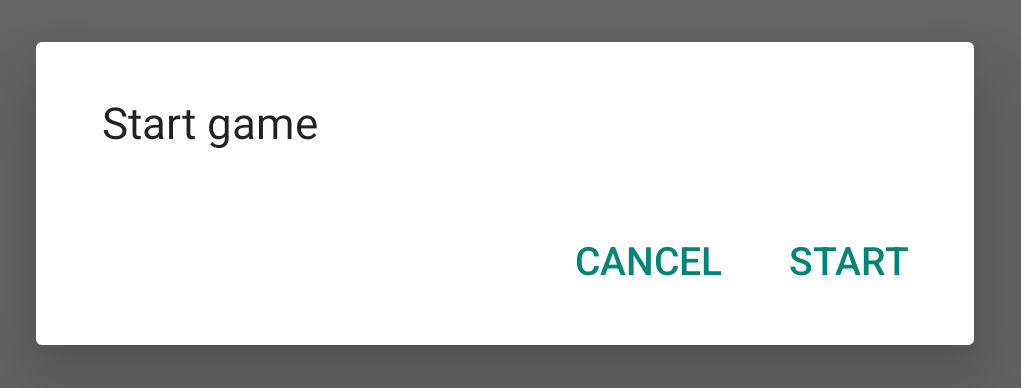
Sonraki bölümde,
AlertDialog.Builder
.
İletişim kutusunu oluşturmak için kullanılan API'ler.
Diyaloğunuzun ne kadar karmaşık olduğuna bağlı olarak, iletişim kurmanın
DialogFragment
özelliğindeki tüm diğer geri çağırma yöntemleri
temel parça yaşam döngüsü yöntemlerini ele alacağız.
Uyarı iletişim kutusu oluştur
AlertDialog
sınıfı çeşitli iletişim kutuları oluşturmanıza olanak tanır
genellikle ihtiyacınız olan tek diyalog sınıfıdır. Aşağıda gösterildiği gibi
uyarı iletişim kutusunun üç bölümü vardır:
- Başlık: Bu kısım isteğe bağlıdır ve yalnızca içerik alanı ayrıntılı bir mesaj, liste veya özel düzenle dolu. Gerekirse basit bir ileti veya soru belirtiyorsanız başlık gerekmez.
- İçerik alanı: Bir mesaj, liste veya başka bir özel alan görüntüleyebilir. kullanır.
- İşlem düğmeleri: Tek bir reklamda en fazla üç işlem düğmesi olabilir iletişim kutusu.
AlertDialog.Builder
sınıfı, aşağıdakileri oluşturmanıza olanak tanıyan API'ler sağlar:
dahil olmak üzere, bu tür içeriklere sahip AlertDialog
kullanır.
AlertDialog
oluşturmak için aşağıdakileri yapın:
Kotlin
val builder: AlertDialog.Builder = AlertDialog.Builder(context) builder .setMessage("I am the message") .setTitle("I am the title") val dialog: AlertDialog = builder.create() dialog.show()
Java
// 1. Instantiate an AlertDialog.Builder with its constructor. AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); // 2. Chain together various setter methods to set the dialog characteristics. builder.setMessage(R.string.dialog_message) .setTitle(R.string.dialog_title); // 3. Get the AlertDialog. AlertDialog dialog = builder.create();
Önceki kod snippet'i şu iletişim kutusunu oluşturur:
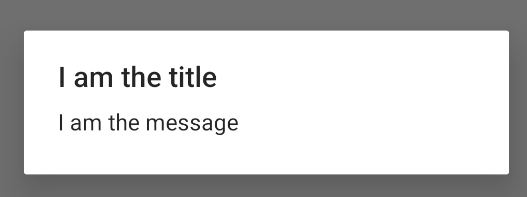
Düğme ekle
Şekil 2'dekine benzer işlem düğmeleri eklemek için
setPositiveButton()
.
ve
setNegativeButton()
yöntemleri:
Kotlin
val builder: AlertDialog.Builder = AlertDialog.Builder(context) builder .setMessage("I am the message") .setTitle("I am the title") .setPositiveButton("Positive") { dialog, which -> // Do something. } .setNegativeButton("Negative") { dialog, which -> // Do something else. } val dialog: AlertDialog = builder.create() dialog.show()
Java
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); // Add the buttons. builder.setPositiveButton(R.string.ok, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // User taps OK button. } }); builder.setNegativeButton(R.string.cancel, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // User cancels the dialog. } }); // Set other dialog properties. ... // Create the AlertDialog. AlertDialog dialog = builder.create();
set...Button()
yöntemleri,
düğmesinden; bir
dize kaynağı ve
CANNOT TRANSLATE
DialogInterface.OnClickListener
Kullanıcı düğmeye dokunduğunda yapılacak işlemi tanımlayan
Ekleyebileceğiniz üç işlem düğmesi vardır:
- Olumlu: İşlemi kabul etmek ve işleme devam etmek için bu seçeneği kullanın ( "Tamam" işlemi).
- Negatif: İşlemi iptal etmek için bunu kullanın.
- Nötr: Bu seçeneği, kullanıcı işlemi gerçekleştirmek istemeyebileceğinde iptal etmek istemediği anlamına gelir. Bu artı ve eksi düğmelerine bakalım. Örneğin, "Bana hatırlat daha sonra."
AlertDialog
öğesine her düğme türünden yalnızca bir tane ekleyebilirsiniz. Örneğin,
Örneğin, tek seferde birden fazla "olumlu" düğmesini tıklayın.
Önceki kod snippet'i, size aşağıdaki gibi bir uyarı iletişim kutusu sunar:
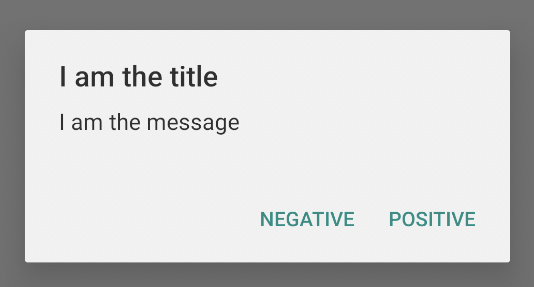
Liste ekleme
AlertDialog
ile kullanılabilen üç tür liste vardır
API'ler:
- Geleneksel tek seçimli liste.
- Kalıcı tek seçimli liste (radyo düğmeleri).
- Kalıcı bir çoktan seçmeli liste (onay kutuları).
Şekil 5'teki gibi bir tekli seçimli liste oluşturmak için
setItems()
.
yöntem:
Kotlin
val builder: AlertDialog.Builder = AlertDialog.Builder(context) builder .setTitle("I am the title") .setPositiveButton("Positive") { dialog, which -> // Do something. } .setNegativeButton("Negative") { dialog, which -> // Do something else. } .setItems(arrayOf("Item One", "Item Two", "Item Three")) { dialog, which -> // Do something on item tapped. } val dialog: AlertDialog = builder.create() dialog.show()
Java
@Override public Dialog onCreateDialog(Bundle savedInstanceState) { AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); builder.setTitle(R.string.pick_color) .setItems(R.array.colors_array, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { // The 'which' argument contains the index position of the selected item. } }); return builder.create(); }
Bu kod snippet'i aşağıdakine benzer bir iletişim kutusu oluşturur:
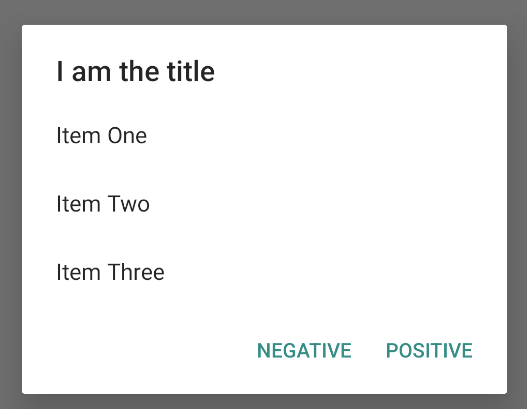
Liste, iletişim kutusunun içerik alanında göründüğünden iletişim kutusu gösterilemiyor
hem ileti hem de liste oluşturabilirsiniz. Şununla iletişim kutusu için bir başlık belirleyin:
setTitle()
Listenin öğelerini belirtmek için setItems()
öğesini çağırın,
dizisidir. Alternatif olarak, şunu kullanarak bir liste belirtebilirsiniz:
setAdapter()
Bu, listeyi dinamik verilerle (örneğin, bir cihazdan
kullanarak bir
ListAdapter
Listenizi bir ListAdapter
ile yedeklerseniz her zaman bir
Loader
içerik eşzamansız olarak yüklenir. Bu konu,
Derleme düzenleri
ve bir adaptörle
Yükleyiciler.
Kalıcı bir çoktan seçmeli veya tekli seçim listesi ekleme
Çoktan seçmeli öğeler (onay kutuları) veya tekli seçimli öğeler listesi eklemek için
(radyo düğmeleri) kullanıyorsanız
setMultiChoiceItems()
.
veya
setSingleChoiceItems()
yöntemlerine bakalım.
Örneğin, Arkadaş Bitkiler projenizde çoktan seçmeli bir listeyi nasıl
Şekil 6'da gösterilmiştir. Bu örnek öğeler,
ArrayList
:
Kotlin
val builder: AlertDialog.Builder = AlertDialog.Builder(context) builder .setTitle("I am the title") .setPositiveButton("Positive") { dialog, which -> // Do something. } .setNegativeButton("Negative") { dialog, which -> // Do something else. } .setMultiChoiceItems( arrayOf("Item One", "Item Two", "Item Three"), null) { dialog, which, isChecked -> // Do something. } val dialog: AlertDialog = builder.create() dialog.show()
Java
@Override public Dialog onCreateDialog(Bundle savedInstanceState) { selectedItems = new ArrayList(); // Where we track the selected items AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); // Set the dialog title. builder.setTitle(R.string.pick_toppings) // Specify the list array, the items to be selected by default (null for // none), and the listener through which to receive callbacks when items // are selected. .setMultiChoiceItems(R.array.toppings, null, new DialogInterface.OnMultiChoiceClickListener() { @Override public void onClick(DialogInterface dialog, int which, boolean isChecked) { if (isChecked) { // If the user checks the item, add it to the selected // items. selectedItems.add(which); } else if (selectedItems.contains(which)) { // If the item is already in the array, remove it. selectedItems.remove(which); } } }) // Set the action buttons .setPositiveButton(R.string.ok, new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int id) { // User taps OK, so save the selectedItems results // somewhere or return them to the component that opens the // dialog. ... } }) .setNegativeButton(R.string.cancel, new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int id) { ... } }); return builder.create(); }
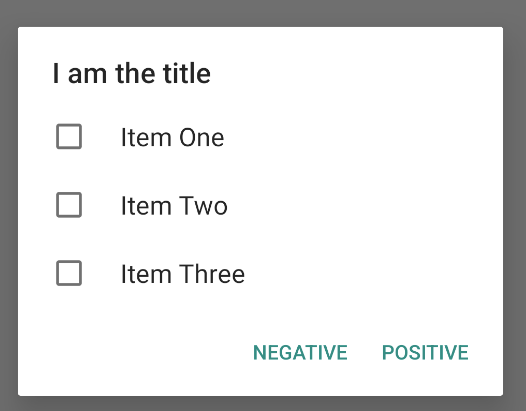
Şu şekilde tek seçimli uyarı iletişim kutusu elde edilebilir:
Kotlin
val builder: AlertDialog.Builder = AlertDialog.Builder(context) builder .setTitle("I am the title") .setPositiveButton("Positive") { dialog, which -> // Do something. } .setNegativeButton("Negative") { dialog, which -> // Do something else. } .setSingleChoiceItems( arrayOf("Item One", "Item Two", "Item Three"), 0 ) { dialog, which -> // Do something. } val dialog: AlertDialog = builder.create() dialog.show()
Java
String[] choices = {"Item One", "Item Two", "Item Three"}; AlertDialog.Builder builder = AlertDialog.Builder(context); builder .setTitle("I am the title") .setPositiveButton("Positive", (dialog, which) -> { }) .setNegativeButton("Negative", (dialog, which) -> { }) .setSingleChoiceItems(choices, 0, (dialog, which) -> { }); AlertDialog dialog = builder.create(); dialog.show();
Bu, aşağıdaki örnekle sonuçlanır:
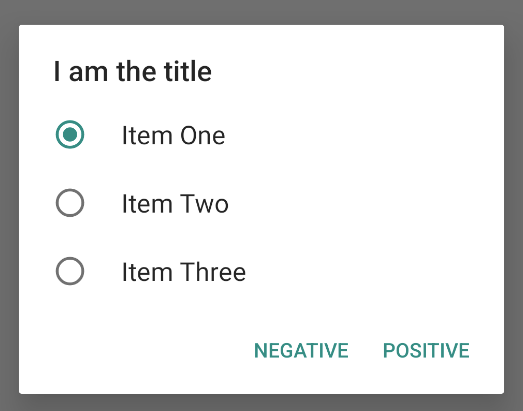
Özel düzen oluşturma
Bir iletişim kutusunda özel düzen isterseniz bir düzen oluşturun ve
Telefon ederek AlertDialog
setView()
AlertDialog.Builder
nesnenizde.
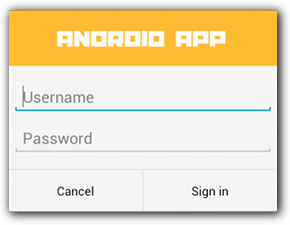
Özel düzen, varsayılan olarak iletişim kutusunu doldurur ancak
Düğme ve başlık eklemek için AlertDialog.Builder
yöntem.
Örneğin, önceki özel iletişim kutusunun düzen dosyası düzen:
res/layout/dialog_signin.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="wrap_content" android:layout_height="wrap_content"> <ImageView android:src="@drawable/header_logo" android:layout_width="match_parent" android:layout_height="64dp" android:scaleType="center" android:background="#FFFFBB33" android:contentDescription="@string/app_name" /> <EditText android:id="@+id/username" android:inputType="textEmailAddress" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="16dp" android:layout_marginLeft="4dp" android:layout_marginRight="4dp" android:layout_marginBottom="4dp" android:hint="@string/username" /> <EditText android:id="@+id/password" android:inputType="textPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="4dp" android:layout_marginLeft="4dp" android:layout_marginRight="4dp" android:layout_marginBottom="16dp" android:fontFamily="sans-serif" android:hint="@string/password"/> </LinearLayout>
DialogFragment
metriğinizde düzeni artırmak için
LayoutInflater
şununla:
getLayoutInflater()
arayın
inflate()
.
İlk parametre, düzen kaynağı kimliği, ikinci parametre ise
üst görünüm oluşturabilirsiniz. Ardından
setView()
.
tıklayın. Bu, aşağıdaki örnekte gösterilmektedir.
Kotlin
override fun onCreateDialog(savedInstanceState: Bundle?): Dialog { return activity?.let { val builder = AlertDialog.Builder(it) // Get the layout inflater. val inflater = requireActivity().layoutInflater; // Inflate and set the layout for the dialog. // Pass null as the parent view because it's going in the dialog // layout. builder.setView(inflater.inflate(R.layout.dialog_signin, null)) // Add action buttons. .setPositiveButton(R.string.signin, DialogInterface.OnClickListener { dialog, id -> // Sign in the user. }) .setNegativeButton(R.string.cancel, DialogInterface.OnClickListener { dialog, id -> getDialog().cancel() }) builder.create() } ?: throw IllegalStateException("Activity cannot be null") }
Java
@Override public Dialog onCreateDialog(Bundle savedInstanceState) { AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); // Get the layout inflater. LayoutInflater inflater = requireActivity().getLayoutInflater(); // Inflate and set the layout for the dialog. // Pass null as the parent view because it's going in the dialog layout. builder.setView(inflater.inflate(R.layout.dialog_signin, null)) // Add action buttons .setPositiveButton(R.string.signin, new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int id) { // Sign in the user. } }) .setNegativeButton(R.string.cancel, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { LoginDialogFragment.this.getDialog().cancel(); } }); return builder.create(); }
Özel bir iletişim kutusu istiyorsanız bunun yerine
Activity
iletişim kutusunu işaretleyin.Dialog
Etkinlik oluşturun ve
temasını şu şekilde ayarla:
Theme.Holo.Dialog
.
<activity>
manifest öğesi:
<activity android:theme="@android:style/Theme.Holo.Dialog" >
Etkinlik artık tam ekran yerine bir iletişim penceresinde görüntülenir.
Etkinlikleri iletişim kutusunun ana makinesine geri iletme
Kullanıcı iletişim kutusundaki işlem düğmelerinden birine dokunduğunda veya bir öğe seçtiğinde
işlemini kendi listesinden çıkardığınızda, DialogFragment
cihazınız
fakat genellikle etkinliği etkinliğe teslim etmek veya
parçayı tıklayın. Bunun için arayüz ve yöntem tanımlayın
tıklayın. Ardından, bu arayüzü ana makineye uygulayın
iletişim kutusundan işlem etkinliklerini alan bileşen
Örneğin, aşağıda bir arayüzü tanımlayan DialogFragment
verilmiştir
Bu işlemden sonra etkinlikler, düzenleyen kişinin etkinliğine geri gönderilir:
Kotlin
class NoticeDialogFragment : DialogFragment() { // Use this instance of the interface to deliver action events. internal lateinit var listener: NoticeDialogListener // The activity that creates an instance of this dialog fragment must // implement this interface to receive event callbacks. Each method passes // the DialogFragment in case the host needs to query it. interface NoticeDialogListener { fun onDialogPositiveClick(dialog: DialogFragment) fun onDialogNegativeClick(dialog: DialogFragment) } // Override the Fragment.onAttach() method to instantiate the // NoticeDialogListener. override fun onAttach(context: Context) { super.onAttach(context) // Verify that the host activity implements the callback interface. try { // Instantiate the NoticeDialogListener so you can send events to // the host. listener = context as NoticeDialogListener } catch (e: ClassCastException) { // The activity doesn't implement the interface. Throw exception. throw ClassCastException((context.toString() + " must implement NoticeDialogListener")) } } }
Java
public class NoticeDialogFragment extends DialogFragment { // The activity that creates an instance of this dialog fragment must // implement this interface to receive event callbacks. Each method passes // the DialogFragment in case the host needs to query it. public interface NoticeDialogListener { public void onDialogPositiveClick(DialogFragment dialog); public void onDialogNegativeClick(DialogFragment dialog); } // Use this instance of the interface to deliver action events. NoticeDialogListener listener; // Override the Fragment.onAttach() method to instantiate the // NoticeDialogListener. @Override public void onAttach(Context context) { super.onAttach(context); // Verify that the host activity implements the callback interface. try { // Instantiate the NoticeDialogListener so you can send events to // the host. listener = (NoticeDialogListener) context; } catch (ClassCastException e) { // The activity doesn't implement the interface. Throw exception. throw new ClassCastException(activity.toString() + " must implement NoticeDialogListener"); } } ... }
İletişim kutusunu barındıran etkinlik
iletişim kutusu parçasının oluşturucusudur ve iletişim kutusunun etkinliklerini bir
NoticeDialogListener
arayüzünün uygulanması:
Kotlin
class MainActivity : FragmentActivity(), NoticeDialogFragment.NoticeDialogListener { fun showNoticeDialog() { // Create an instance of the dialog fragment and show it. val dialog = NoticeDialogFragment() dialog.show(supportFragmentManager, "NoticeDialogFragment") } // The dialog fragment receives a reference to this Activity through the // Fragment.onAttach() callback, which it uses to call the following // methods defined by the NoticeDialogFragment.NoticeDialogListener // interface. override fun onDialogPositiveClick(dialog: DialogFragment) { // User taps the dialog's positive button. } override fun onDialogNegativeClick(dialog: DialogFragment) { // User taps the dialog's negative button. } }
Java
public class MainActivity extends FragmentActivity implements NoticeDialogFragment.NoticeDialogListener{ ... public void showNoticeDialog() { // Create an instance of the dialog fragment and show it. DialogFragment dialog = new NoticeDialogFragment(); dialog.show(getSupportFragmentManager(), "NoticeDialogFragment"); } // The dialog fragment receives a reference to this Activity through the // Fragment.onAttach() callback, which it uses to call the following // methods defined by the NoticeDialogFragment.NoticeDialogListener // interface. @Override public void onDialogPositiveClick(DialogFragment dialog) { // User taps the dialog's positive button. ... } @Override public void onDialogNegativeClick(DialogFragment dialog) { // User taps the dialog's negative button. ... } }
Çünkü düzenleyen kullanıcı etkinliği,
NoticeDialogListener
(
onAttach()
önceki örnekte gösterilen geri çağırma yöntemi. İletişim kutusu parçası,
Tıklama etkinliklerini etkinliğe iletmek için arayüz geri çağırma yöntemlerini kullanın:
Kotlin
override fun onCreateDialog(savedInstanceState: Bundle): Dialog { return activity?.let { // Build the dialog and set up the button click handlers. val builder = AlertDialog.Builder(it) builder.setMessage(R.string.dialog_start_game) .setPositiveButton(R.string.start, DialogInterface.OnClickListener { dialog, id -> // Send the positive button event back to the // host activity. listener.onDialogPositiveClick(this) }) .setNegativeButton(R.string.cancel, DialogInterface.OnClickListener { dialog, id -> // Send the negative button event back to the // host activity. listener.onDialogNegativeClick(this) }) builder.create() } ?: throw IllegalStateException("Activity cannot be null") }
Java
public class NoticeDialogFragment extends DialogFragment { ... @Override public Dialog onCreateDialog(Bundle savedInstanceState) { // Build the dialog and set up the button click handlers. AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); builder.setMessage(R.string.dialog_start_game) .setPositiveButton(R.string.start, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // Send the positive button event back to the host activity. listener.onDialogPositiveClick(NoticeDialogFragment.this); } }) .setNegativeButton(R.string.cancel, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // Send the negative button event back to the host activity. listener.onDialogNegativeClick(NoticeDialogFragment.this); } }); return builder.create(); } }
İletişim kutusu göster
Diyaloğunuzu göstermek istediğinizde
DialogFragment
ve telefon et
show()
,
yalnızca
FragmentManager
iletişim kutusu parçası için bir etiket adı ekleyin.
Şu numarayı arayarak FragmentManager
alabilirsiniz:
getSupportFragmentManager()
şuradan
FragmentActivity
veya şu numarayı arayarak
getParentFragmentManager()
Fragment
adlı cihazdan. Örnek için aşağıdaki bilgilere bakın:
Kotlin
fun confirmStartGame() { val newFragment = StartGameDialogFragment() newFragment.show(supportFragmentManager, "game") }
Java
public void confirmStartGame() { DialogFragment newFragment = new StartGameDialogFragment(); newFragment.show(getSupportFragmentManager(), "game"); }
İkinci bağımsız değişken olan "game"
,
sistem, gerektiğinde parça durumunu kaydedip geri yüklemek için kullanır. Etiket ayrıca
öğesini çağırarak parçaya bir herkese açık kullanıcı adı eklemenizi sağlar
findFragmentByTag()
İletişim kutusunu tam ekran veya yerleştirilmiş parça olarak göster
Kullanıcı arayüzü tasarımınızın bir parçasının
bazı durumlarda iletişim kutusu olarak
ve diğerlerinde tam ekran ya da yerleşik bir parça olarak kullanılabilir. Ayrıca
cihazın ekran boyutuna bağlı olarak farklı görünmesini
istemeyebilirsiniz. İlgili içeriği oluşturmak için kullanılan
DialogFragment
sınıfı bunu başarma esnekliği sunar.
çünkü yerleştirilebilir Fragment
olarak davranabilir.
Ancak AlertDialog.Builder
veya diğer
Bu örnekte iletişim kutusunu oluşturmak için Dialog
nesne. URL'nin
DialogFragment
yerleştirilebilir, iletişim kutusunun kullanıcı arayüzünü
düzen, ardından düzeni
onCreateView()
geri arama.
İletişim kutusu olarak gösterilebilecek bir DialogFragment
örneğini burada bulabilirsiniz.
adlı bir düzen kullanan yerleştirilebilir bir parça
purchase_items.xml
:
Kotlin
class CustomDialogFragment : DialogFragment() { // The system calls this to get the DialogFragment's layout, regardless of // whether it's being displayed as a dialog or an embedded fragment. override fun onCreateView( inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle? ): View { // Inflate the layout to use as a dialog or embedded fragment. return inflater.inflate(R.layout.purchase_items, container, false) } // The system calls this only when creating the layout in a dialog. override fun onCreateDialog(savedInstanceState: Bundle): Dialog { // The only reason you might override this method when using // onCreateView() is to modify the dialog characteristics. For example, // the dialog includes a title by default, but your custom layout might // not need it. Here, you can remove the dialog title, but you must // call the superclass to get the Dialog. val dialog = super.onCreateDialog(savedInstanceState) dialog.requestWindowFeature(Window.FEATURE_NO_TITLE) return dialog } }
Java
public class CustomDialogFragment extends DialogFragment { // The system calls this to get the DialogFragment's layout, regardless of // whether it's being displayed as a dialog or an embedded fragment. @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout to use as a dialog or embedded fragment. return inflater.inflate(R.layout.purchase_items, container, false); } // The system calls this only when creating the layout in a dialog. @Override public Dialog onCreateDialog(Bundle savedInstanceState) { // The only reason you might override this method when using // onCreateView() is to modify the dialog characteristics. For example, // the dialog includes a title by default, but your custom layout might // not need it. Here, you can remove the dialog title, but you must // call the superclass to get the Dialog. Dialog dialog = super.onCreateDialog(savedInstanceState); dialog.requestWindowFeature(Window.FEATURE_NO_TITLE); return dialog; } }
Aşağıdaki örnek, parçanın iletişim kutusu olarak mı yoksa bir iletişim kutusu olarak mı gösterileceğini belirler ekran boyutuna göre tam ekran kullanıcı arayüzü:
Kotlin
fun showDialog() { val fragmentManager = supportFragmentManager val newFragment = CustomDialogFragment() if (isLargeLayout) { // The device is using a large layout, so show the fragment as a // dialog. newFragment.show(fragmentManager, "dialog") } else { // The device is smaller, so show the fragment fullscreen. val transaction = fragmentManager.beginTransaction() // For a polished look, specify a transition animation. transaction.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN) // To make it fullscreen, use the 'content' root view as the container // for the fragment, which is always the root view for the activity. transaction .add(android.R.id.content, newFragment) .addToBackStack(null) .commit() } }
Java
public void showDialog() { FragmentManager fragmentManager = getSupportFragmentManager(); CustomDialogFragment newFragment = new CustomDialogFragment(); if (isLargeLayout) { // The device is using a large layout, so show the fragment as a // dialog. newFragment.show(fragmentManager, "dialog"); } else { // The device is smaller, so show the fragment fullscreen. FragmentTransaction transaction = fragmentManager.beginTransaction(); // For a polished look, specify a transition animation. transaction.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN); // To make it fullscreen, use the 'content' root view as the container // for the fragment, which is always the root view for the activity. transaction.add(android.R.id.content, newFragment) .addToBackStack(null).commit(); } }
Parça işlemleri gerçekleştirme hakkında daha fazla bilgi için bkz. Parçalar.
Bu örnekte mIsLargeLayout
boole değeri,
geçerli cihaz, uygulamanın geniş düzen tasarımını kullanmalıdır.
Böylece bu seçenek,
parçasına dönüştürmeyin. Bu tür bir ayarı yapmanın en iyi yolu
boole değeri
bool kaynağı
değeri
alternatif
kaynak değerini doğrulayın. Örneğin, burada
farklı ekran boyutları için bool kaynağının sürümleri şunlardır:
res/values/bools.xml
<!-- Default boolean values --> <resources> <bool name="large_layout">false</bool> </resources>
res/values-large/bools.xml
<!-- Large screen boolean values --> <resources> <bool name="large_layout">true</bool> </resources>
Daha sonra mIsLargeLayout
değerini
etkinlik
onCreate()
yöntemini çağırın:
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) isLargeLayout = resources.getBoolean(R.bool.large_layout) }
Java
boolean isLargeLayout; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); isLargeLayout = getResources().getBoolean(R.bool.large_layout); }
Bir etkinliği büyük ekranlarda iletişim kutusu olarak göster
Bir iletişim kutusunu küçük ekranlarda tam ekran kullanıcı arayüzü olarak göstermek yerine
Activity
öğesini büyük bir iletişim kutusu olarak göstererek aynı sonucu
ekranları. Seçtiğiniz yaklaşım, uygulamanızın tasarımına bağlıdır ancak
küçük bir iletişim kutusu olarak tasarlanan uygulamalar genellikle
ve tabletlerdeki deneyimi iyileştirmek istiyorsanız,
bir iletişim kutusu olarak görebiliriz.
Bir etkinliği yalnızca büyük ekranlarda iletişim kutusu olarak göstermek için
Theme.Holo.DialogWhenLarge
.
<activity>
manifest öğesi için bir tema oluşturun:
<activity android:theme="@android:style/Theme.Holo.DialogWhenLarge" >
Etkinliklerinizin stilini temalarla düzenleme hakkında daha fazla bilgi için Stiller ve temalar.
İletişim kutusunu kapatma
Kullanıcı,
AlertDialog.Builder
, sistem iletişim kutusunu sizin yerinize kapatır.
Kullanıcı, iletişim kutusundaki bir öğeye dokunduğunda sistem iletişim kutusunu da kapatır
(Listenin radyo düğmelerini veya onay kutularını kullandığı durumlar dışında) Aksi takdirde
şunu çağırarak iletişiminizi manuel olarak kapatın:
dismiss()
.
DialogFragment
cihazınızda.
İletişim kutusu kaybolduğunda belirli işlemleri gerçekleştirmeniz gerekiyorsa şunları yapabilirsiniz:
onDismiss()
.
yöntemini DialogFragment
ile değiştirebilirsiniz.
Ayrıca iletişim kutusunu iptal edebilirsiniz. Bu özel bir etkinliktir
kullanıcının görevi tamamlamadan iletişim kutusundan ayrıldığını gösterir. Bu
kullanıcı Geri düğmesine dokunduğunda veya iletişim kutusu dışında ekrana dokunduğunda gerçekleşir
yoksa açıkça aradıktan sonra
cancel()
.
Dialog
üzerinde (örneğin, "İptal"e yanıt olarak) düğmesini
iletişim kutusu.
Önceki örnekte gösterildiği gibi iptal etkinliğine şu şekilde yanıt verebilirsiniz:
uygulamak
onCancel()
.
DialogFragment
sınıfınızda.