本课程介绍了如何创建 CompatTab
和 TabHelper
抽象类的子类以及如何使用新 API。只要设备搭载的平台版本支持,您的应用就可以在设备上使用此实现。
使用新版 API 实现标签页
使用新版 API 的 CompatTab
和 TabHelper
的具体类是一种代理实现。由于上一课中定义的抽象类反映的是新版 API(类结构、方法签名等),因此使用这些新版 API 的具体类只是代理了方法调用及其结果。
您可以直接在这些具体类中使用新版 API,而不会在旧版设备上因为延迟加载类而导致崩溃。类会在第一次访问时加载并初始化,即首次实例化类或访问类的某个静态字段或方法时。因此,只要不在 Honeycomb 之前的设备上实例化特定于 Honeycomb 的实现,Dalvik 虚拟机就不会抛出任何 VerifyError
异常。
针对此实现的一种建议的命名惯例是,附加与这些具体类所要求的 API 相对应的 API 级别或平台版本代码名称。例如,CompatTabHoneycomb
和 TabHelperHoneycomb
类可以提供原生标签页实现,因为这两个类依赖于 Android 3.0(API 级别 11)或更高版本中提供的 API。
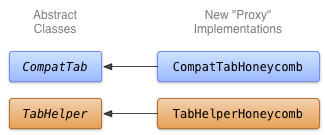
图 1.Honeycomb 标签页实现的类图表。
实现 CompatTabHoneycomb
CompatTabHoneycomb
是 TabHelperHoneycomb
用来引用各个标签页的 CompatTab
抽象类的实现。CompatTabHoneycomb
只是用其所包含的 ActionBar.Tab
对象代理所有方法调用。
开始使用新的 ActionBar.Tab
API 来实现 CompatTabHoneycomb
:
Kotlin
class CompatTabHoneycomb internal constructor(val activity: Activity, tag: String) : CompatTab(tag) { ... // The native tab object that this CompatTab acts as a proxy for. private var mTab: ActionBar.Tab = // Proxy to new ActionBar.newTab API activity.actionBar.newTab() override fun setText(@StringRes textId: Int): CompatTab { // Proxy to new ActionBar.Tab.setText API mTab.setText(textId) return this } ... // Do the same for other properties (icon, callback, etc.) }
Java
public class CompatTabHoneycomb extends CompatTab { // The native tab object that this CompatTab acts as a proxy for. ActionBar.Tab mTab; ... protected CompatTabHoneycomb(FragmentActivity activity, String tag) { ... // Proxy to new ActionBar.newTab API mTab = activity.getActionBar().newTab(); } public CompatTab setText(int resId) { // Proxy to new ActionBar.Tab.setText API mTab.setText(resId); return this; } ... // Do the same for other properties (icon, callback, etc.) }
实现 TabHelperHoneycomb
TabHelperHoneycomb
是 TabHelper
抽象类的实现,该实现用实际的 ActionBar
(从它所包含的 Activity
中获取)来代理方法调用。
实现 TabHelperHoneycomb
,即用 ActionBar
API 来代理方法调用:
Kotlin
class TabHelperHoneycomb internal constructor(activity: FragmentActivity) : TabHelper(activity) { private var mActionBar: ActionBar? = null override fun setUp() { mActionBar = mActionBar ?: mActivity.actionBar.apply { navigationMode = ActionBar.NAVIGATION_MODE_TABS } } override fun addTab(tab: CompatTab) { // Tab is a CompatTabHoneycomb instance, so its // native tab object is an ActionBar.Tab. mActionBar?.addTab(tab.getTab() as ActionBar.Tab) } }
Java
public class TabHelperHoneycomb extends TabHelper { ActionBar actionBar; ... protected void setUp() { if (actionBar == null) { actionBar = activity.getActionBar(); actionBar.setNavigationMode( ActionBar.NAVIGATION_MODE_TABS); } } public void addTab(CompatTab tab) { ... // Tab is a CompatTabHoneycomb instance, so its // native tab object is an ActionBar.Tab. actionBar.addTab((ActionBar.Tab) tab.getTab()); } // The other important method, newTab() is part of // the base implementation. }