여러 Android 버전에서 알림이 최적의 상태로 표시되도록 하려면 표준 알림 사용 템플릿을 사용하여 있습니다. 알림에 더 많은 콘텐츠를 제공하려면 확장 가능한 알림 기능을 사용해 보세요. 템플릿을 참조하세요.
하지만 시스템 템플릿이 필요에 맞지 않는 경우 자체 템플릿을 나타냅니다.
콘텐츠 영역의 맞춤 레이아웃 만들기
맞춤 레이아웃이 필요한 경우
NotificationCompat.DecoratedCustomViewStyle
드림
추가할 수 있습니다. 이 API를 사용하면 콘텐츠의 맞춤 레이아웃을 제공할 수 있습니다.
일반적으로 제목과 텍스트 콘텐츠가 차지하고
알림 아이콘, 타임스탬프, 하위 텍스트, 작업 버튼의 장식입니다.
이 API는 기본 알림을 기반으로 작성되어 확장 가능한 알림 템플릿과 유사하게 작동합니다. 레이아웃은 다음과 같습니다.
- 기본 알림 빌드
다음 코드로 교체합니다.
NotificationCompat.Builder
- 전화걸기
setStyle()
님, 인코더-디코더 아키텍처를NotificationCompat.DecoratedCustomViewStyle
- 맞춤 레이아웃을
RemoteViews
- 전화걸기
setCustomContentView()
드림 접힌 알림의 레이아웃을 설정합니다. - 원하는 경우
setCustomBigContentView()
드림 확장된 알림에 다른 레이아웃을 설정할 수 있습니다.
레이아웃 준비
small
및 large
레이아웃이 필요합니다. 이 예에서 small
레이아웃은
다음과 같을 수 있습니다.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/notification_title"
style="@style/TextAppearance.Compat.Notification.Title"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_weight="1"
android:text="Small notification, showing only a title" />
</LinearLayout>
large
레이아웃은 다음과 같을 수 있습니다.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="300dp"
android:orientation="vertical">
<TextView
android:id="@+id/notification_title"
style="@style/TextAppearance.Compat.Notification.Title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Large notification, showing a title and a body." />
<TextView
android:id="@+id/notification_body"
style="@style/TextAppearance.Compat.Notification.Line2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="This is the body. The height is manually forced to 300dp." />
</LinearLayout>
알림 빌드 및 표시
레이아웃이 준비되면 다음 예와 같이 사용할 수 있습니다.
Kotlin
val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager // Get the layouts to use in the custom notification. val notificationLayout = RemoteViews(packageName, R.layout.notification_small) val notificationLayoutExpanded = RemoteViews(packageName, R.layout.notification_large) // Apply the layouts to the notification. val customNotification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setStyle(NotificationCompat.DecoratedCustomViewStyle()) .setCustomContentView(notificationLayout) .setCustomBigContentView(notificationLayoutExpanded) .build() notificationManager.notify(666, customNotification)
자바
NotificationManager notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); // Get the layouts to use in the custom notification RemoteViews notificationLayout = new RemoteViews(getPackageName(), R.layout.notification_small); RemoteViews notificationLayoutExpanded = new RemoteViews(getPackageName(), R.layout.notification_large); // Apply the layouts to the notification. Notification customNotification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setStyle(new NotificationCompat.DecoratedCustomViewStyle()) .setCustomContentView(notificationLayout) .setCustomBigContentView(notificationLayoutExpanded) .build(); notificationManager.notify(666, customNotification);
알림의 배경 색상은 기기마다 다를 수 있습니다.
확인할 수 있습니다 다음과 같은 지원 라이브러리 스타일을 적용합니다.
TextAppearance_Compat_Notification
: 텍스트
맞춤 레이아웃의 제목: TextAppearance_Compat_Notification_Title
다음 예와 같이 됩니다. 이 스타일은 색상 변화에 맞춰 조정되므로
검은색 바탕에 검은색 바탕에 검은색 바탕에 흰색 바탕에 흰색 텍스트로 표시되지 않습니다.
<TextView android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_weight="1" android:text="@string/notification_title" android:id="@+id/notification_title" style="@style/TextAppearance.Compat.Notification.Title" />
RemoteViews
객체에 배경 이미지를 설정하지 마세요.
읽을 수 없게 됩니다.
사용자가 앱을 사용하는 동안 알림을 트리거하면 결과는 다음과 같습니다. 그림 1과 유사합니다.
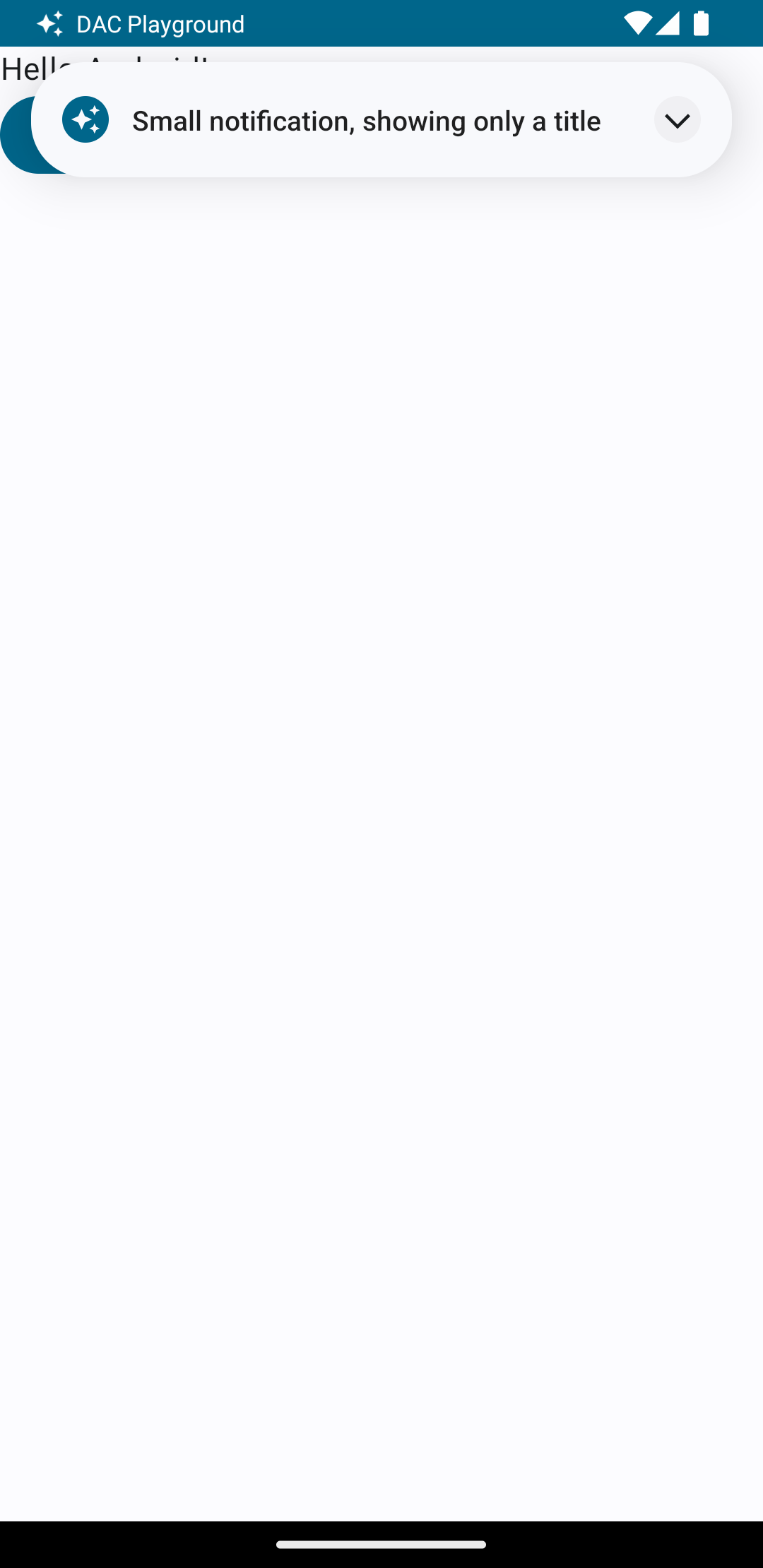
확장 화살표를 탭하면 그림 2와 같이 알림이 펼쳐집니다.
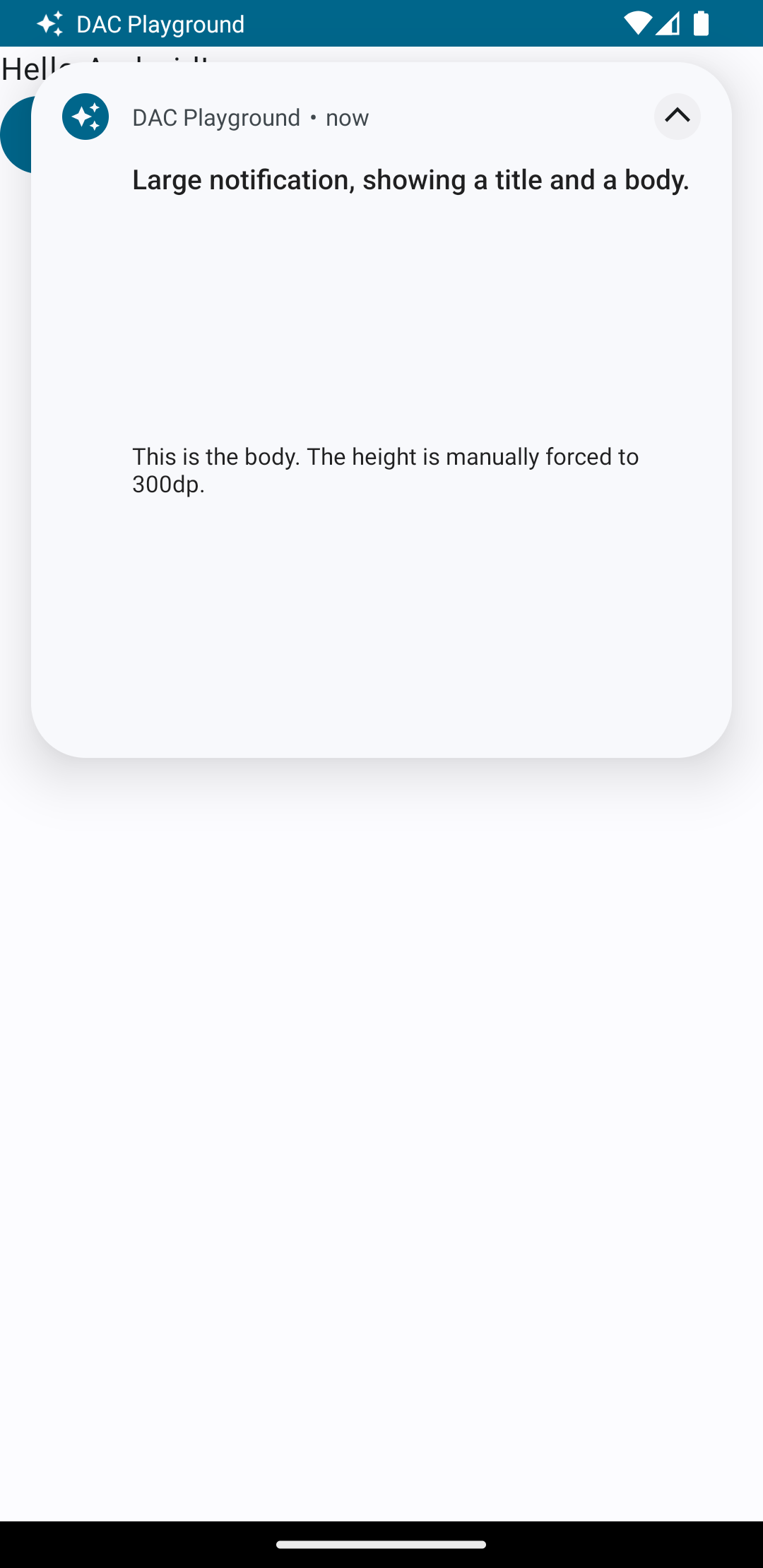
알림 제한 시간이 초과되면 알림은 시스템 표시줄(그림 3 참고)
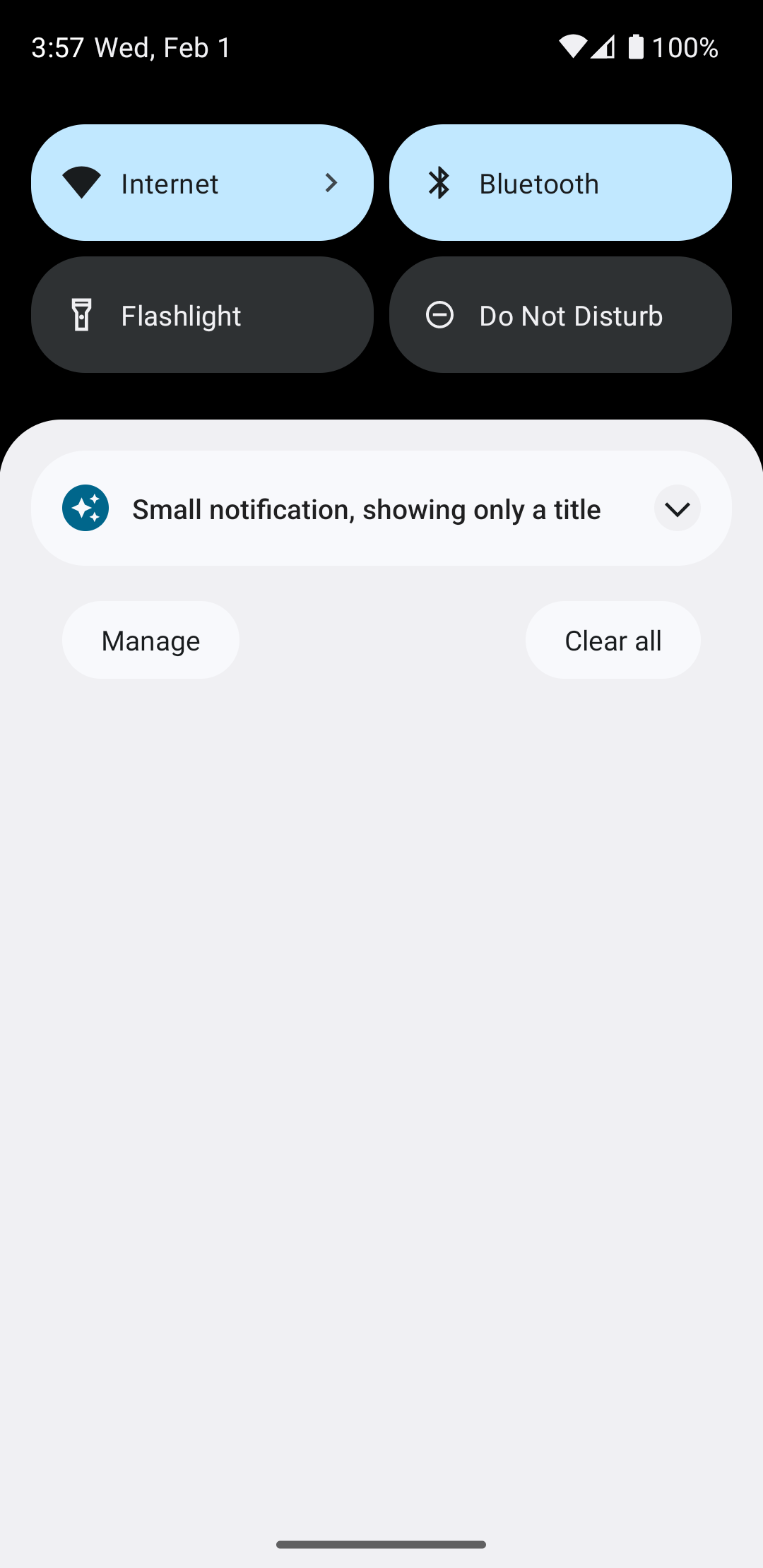
확장 화살표를 탭하면 그림 4와 같이 알림이 펼쳐집니다.
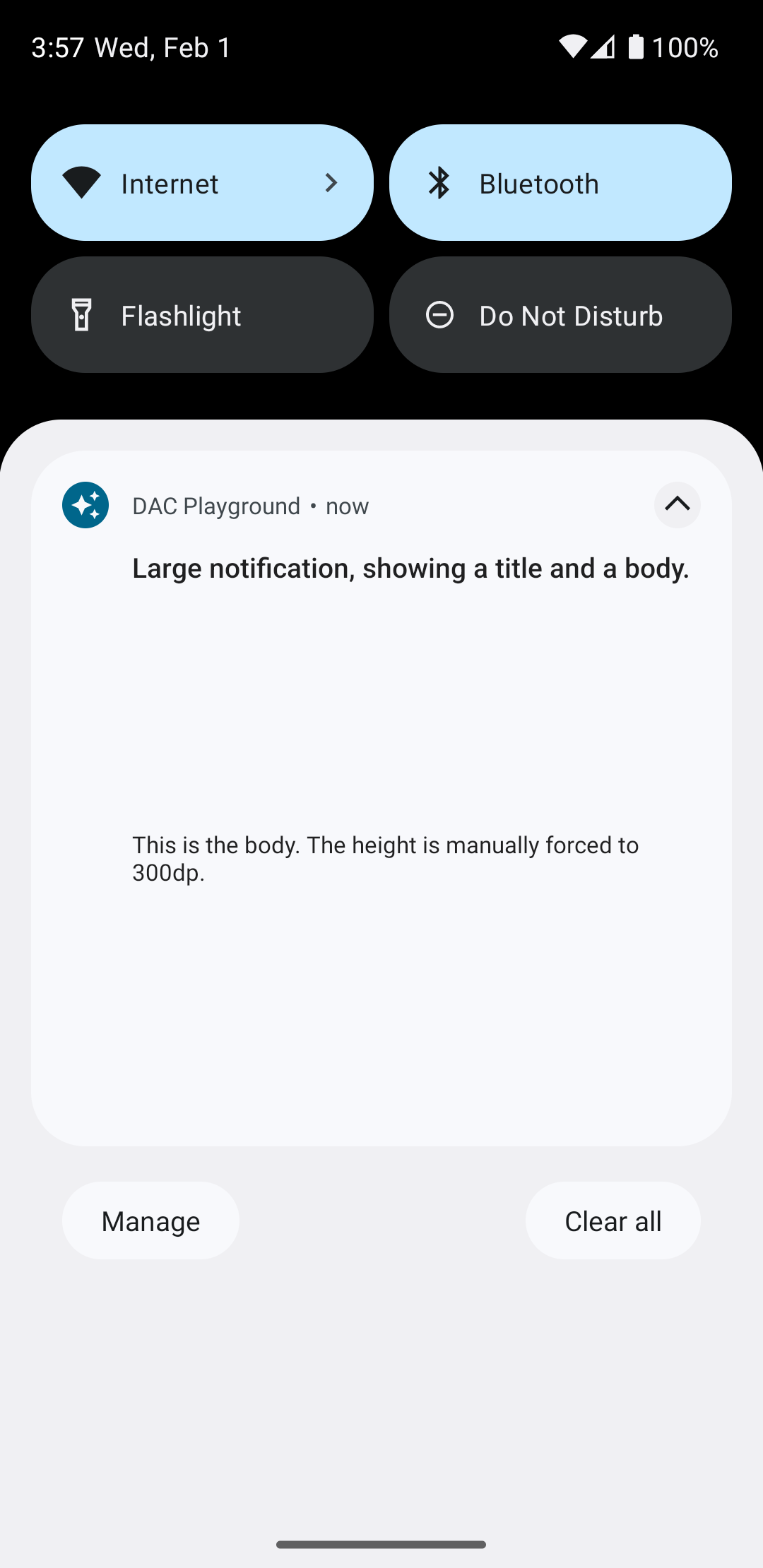
완전한 맞춤 알림 레이아웃 만들기
알림을 표준 알림으로 장식하고 싶지 않은 경우
아이콘 및 헤더가 있는 경우 이전 단계를 따르되 setStyle()
를 호출하지 마세요.