すべてのテキスト フィールドには、メールアドレス、電話番号、書式なしテキストなど、特定のタイプのテキスト入力が想定されます。適切なソフト入力方法(画面キーボードなど)が表示されるように、アプリの各テキスト フィールドの入力タイプを指定する必要があります。
入力方法で使用できるボタンのタイプ以外にも、入力方法でスペル候補を表示する、新しい文を大文字にする、改行ボタンを [Done] や [Next] などのアクション ボタンに置き換えるかどうかなどの動作を指定できます。このページでは、これらの特性を指定する方法について説明します。
キーボード タイプの指定
テキスト フィールドの入力方法を常に宣言するには、<EditText>
要素に android:inputType
属性を追加します。
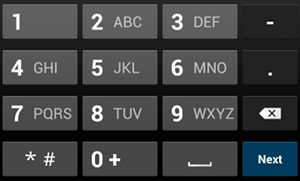
phone
入力タイプ。たとえば、電話番号を入力する入力方法が必要な場合は、"phone"
の値を使用します。
<EditText android:id="@+id/phone" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="@string/phone_hint" android:inputType="phone" />
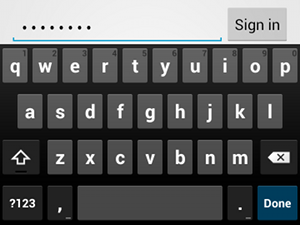
textPassword
入力タイプ。テキスト フィールドがパスワード用の場合は、"textPassword"
値を使用して、テキスト フィールドでユーザーの入力が隠されるようにします。
<EditText android:id="@+id/password" android:hint="@string/password_hint" android:inputType="textPassword" ... />
android:inputType
属性には、使用可能な値がいくつかあります。値の一部を組み合わせて、入力メソッドの外観やその他の動作を指定できます。
スペルの候補と他の動作の有効化
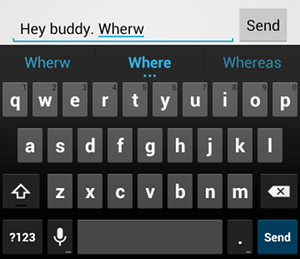
textAutoCorrect
を追加すると、スペルミスが自動修正されます。android:inputType
属性を使用すると、インプット メソッドのさまざまな動作を指定できます。最も重要な点は、テキスト フィールドが基本的なテキスト入力(テキスト メッセージなど)を目的とした場合は、"textAutoCorrect"
値を使用して自動スペル修正を有効にすることです。
android:inputType
属性を使用すると、さまざまな動作と入力方法のスタイルを組み合わせることができます。たとえば、文の最初の単語を大文字にし、スペルミスを自動修正するテキスト フィールドの作成方法は次のとおりです。
<EditText android:id="@+id/message" android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType= "textCapSentences|textAutoCorrect" ... />
入力方法のアクションの指定
ほとんどのソフト入力方法では、現在のテキスト フィールドに適したユーザー アクション ボタンが下隅に用意されています。デフォルトでは、Next または Done のアクションに対してこのボタンが使用されます。ただし、テキスト フィールドが複数行テキスト(android:inputType="textMultiLine"
など)をサポートしている場合、アクション ボタンは改行です。ただし、Send や Go など、テキスト フィールドに適した他のアクションを指定できます。
キーボード アクション ボタンを指定するには、"actionSend"
や "actionSearch"
などのアクション値を指定した android:imeOptions
属性を使用します。たとえば、以下の場合です。

android:imeOptions="actionSend"
を宣言すると、[Send] ボタンが表示されます。<EditText android:id="@+id/search" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="@string/search_hint" android:inputType="text" android:imeOptions="actionSend" />
EditText
要素に TextView.OnEditorActionListener
を定義すると、アクション ボタンの押下をリッスンできます。リスナーで、EditorInfo
クラスで定義された適切な IME アクション ID(IME_ACTION_SEND
など)に応答します。次に例を示します。
Kotlin
findViewById<EditText>(R.id.search).setOnEditorActionListener { v, actionId, event -> return@setOnEditorActionListener when (actionId) { EditorInfo.IME_ACTION_SEND -> { sendMessage() true } else -> false } }
Java
EditText editText = (EditText) findViewById(R.id.search); editText.setOnEditorActionListener(new OnEditorActionListener() { @Override public boolean onEditorAction(TextView v, int actionId, KeyEvent event) { boolean handled = false; if (actionId == EditorInfo.IME_ACTION_SEND) { sendMessage(); handled = true; } return handled; } });
オートコンプリート候補の提供
ユーザーの入力時に候補を提示する場合は、AutoCompleteTextView
という EditText
のサブクラスを使用できます。オートコンプリートを実装するには、テキストの候補を表示する Adapter
を指定する必要があります。データの取得元(データベースやアレイなど)に応じて、複数のアダプタを使用できます。
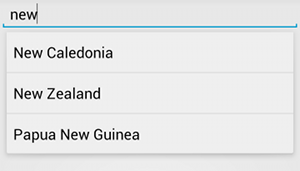
AutoCompleteTextView
の例次の手順では、ArrayAdapter
を使用して配列からの提案を提供する AutoCompleteTextView
を設定する方法について説明します。
AutoCompleteTextView
をレイアウトに追加します。テキスト フィールドのみを含むレイアウトを次に示します。<?xml version="1.0" encoding="utf-8"?> <AutoCompleteTextView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/autocomplete_country" android:layout_width="fill_parent" android:layout_height="wrap_content" />
- すべてのテキスト候補を含む配列を定義します。たとえば、国名の配列は以下のようになります。
<?xml version="1.0" encoding="utf-8"?> <resources> <string-array name="countries_array"> <item>Afghanistan</item> <item>Albania</item> <item>Algeria</item> <item>American Samoa</item> <item>Andorra</item> <item>Angola</item> <item>Anguilla</item> <item>Antarctica</item> ... </string-array> </resources>
Activity
またはFragment
で、次のコードを使用して、候補を提供するアダプターを指定します。Kotlin
// Get a reference to the AutoCompleteTextView in the layout. val textView = findViewById(R.id.autocomplete_country) as AutoCompleteTextView // Get the string array. val countries: Array<out String> = resources.getStringArray(R.array.countries_array) // Create the adapter and set it to the AutoCompleteTextView. ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, countries).also { adapter -> textView.setAdapter(adapter) }
Java
// Get a reference to the AutoCompleteTextView in the layout. AutoCompleteTextView textView = (AutoCompleteTextView) findViewById(R.id.autocomplete_country); // Get the string array. String[] countries = getResources().getStringArray(R.array.countries_array); // Create the adapter and set it to the AutoCompleteTextView. ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, countries); textView.setAdapter(adapter);
上記の例では、新しい
ArrayAdapter
が初期化され、countries_array
文字列配列内の各アイテムがsimple_list_item_1
レイアウトに存在するTextView
にバインドされます。これは Android が提供するレイアウトで、リスト内のテキストの標準的な外観を実現します。-
setAdapter()
を呼び出して、アダプターをAutoCompleteTextView
に割り当てます。