Compose 具有许多内置的动画机制, 不知道该选择哪一个以下是常见的动画用例列表。如需详细了解可供您使用的一整套不同的 API 选项,请参阅完整的 Compose 动画文档。
为常见可组合项属性添加动画效果
Compose 提供了便捷的 API,可让您解决许多常见的动画用例。本部分演示了如何为可组合项的常见属性添加动画效果。
添加出现/消失动画
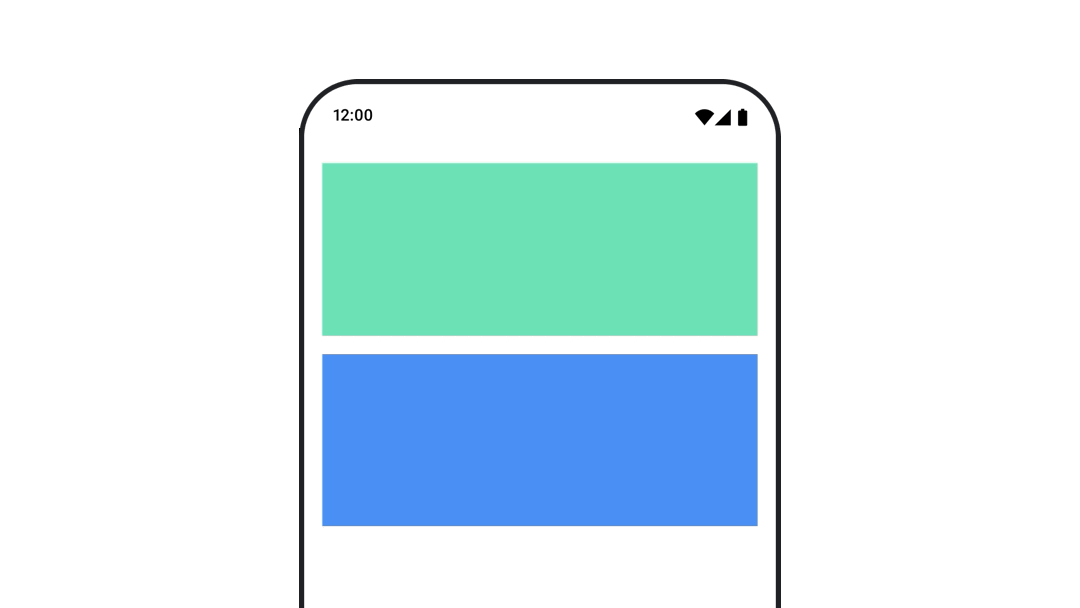
使用 AnimatedVisibility
隐藏或显示可组合项。内部儿童
AnimatedVisibility
可以使用Modifier.animateEnterExit()
作为自己的参赛项目
或退出过渡。
var visible by remember { mutableStateOf(true) } // Animated visibility will eventually remove the item from the composition once the animation has finished. AnimatedVisibility(visible) { // your composable here // ... }
借助 AnimatedVisibility
的进入和退出参数,您可以配置
可组合项在出现和消失时的行为。如需了解详情,请参阅完整文档。
为可组合项添加可见性动画的另一种方法是使用 animateFloatAsState
在时间轴上为 Alpha 值添加动画效果:
var visible by remember { mutableStateOf(true) } val animatedAlpha by animateFloatAsState( targetValue = if (visible) 1.0f else 0f, label = "alpha" ) Box( modifier = Modifier .size(200.dp) .graphicsLayer { alpha = animatedAlpha } .clip(RoundedCornerShape(8.dp)) .background(colorGreen) .align(Alignment.TopCenter) ) { }
不过,更改 Alpha 值后,需要注意的是,可组合项仍会保留
,并且继续占据其布局空间。这个
可能会导致屏幕阅读器和其他无障碍机制仍然考虑
屏幕上呈现的内容另一方面,AnimatedVisibility
最终会移除
组合中的项。
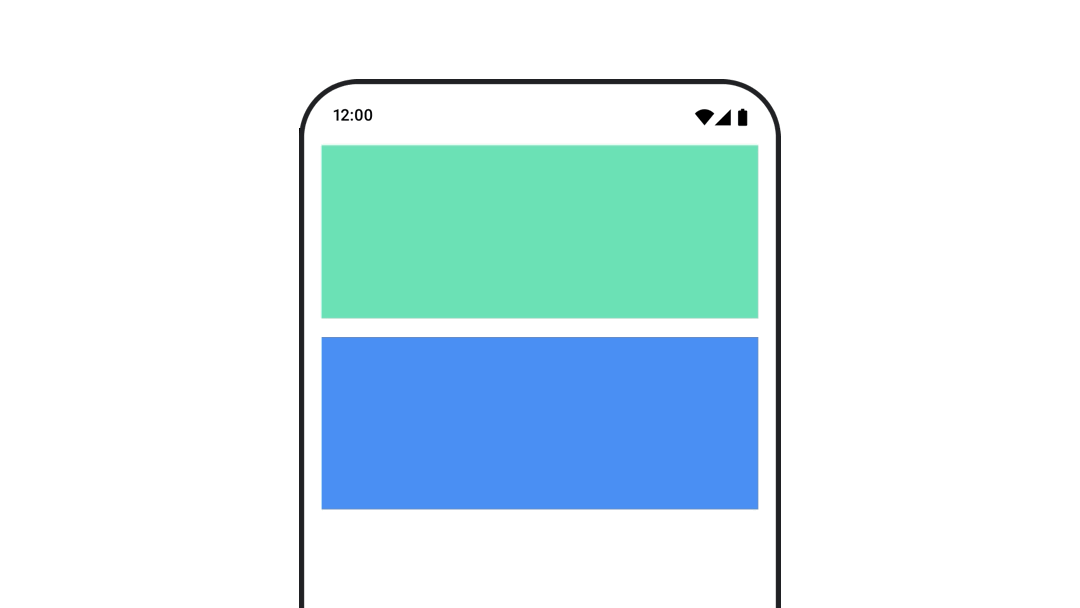
以动画形式呈现背景颜色
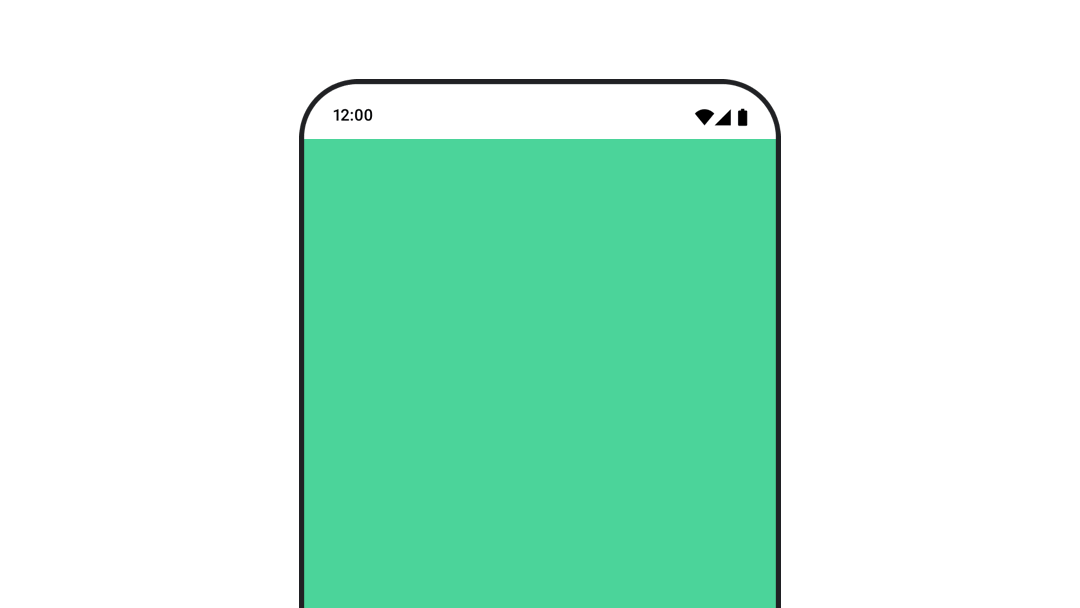
val animatedColor by animateColorAsState( if (animateBackgroundColor) colorGreen else colorBlue, label = "color" ) Column( modifier = Modifier.drawBehind { drawRect(animatedColor) } ) { // your composable here }
此选项的性能高于使用 Modifier.background()
。Modifier.background()
适用于单次色彩设置,
为颜色添加动画效果,可能会导致重组次数
。
如需为背景颜色添加无限动画效果,请参阅“重复动画”部分。
为可组合项的大小添加动画效果
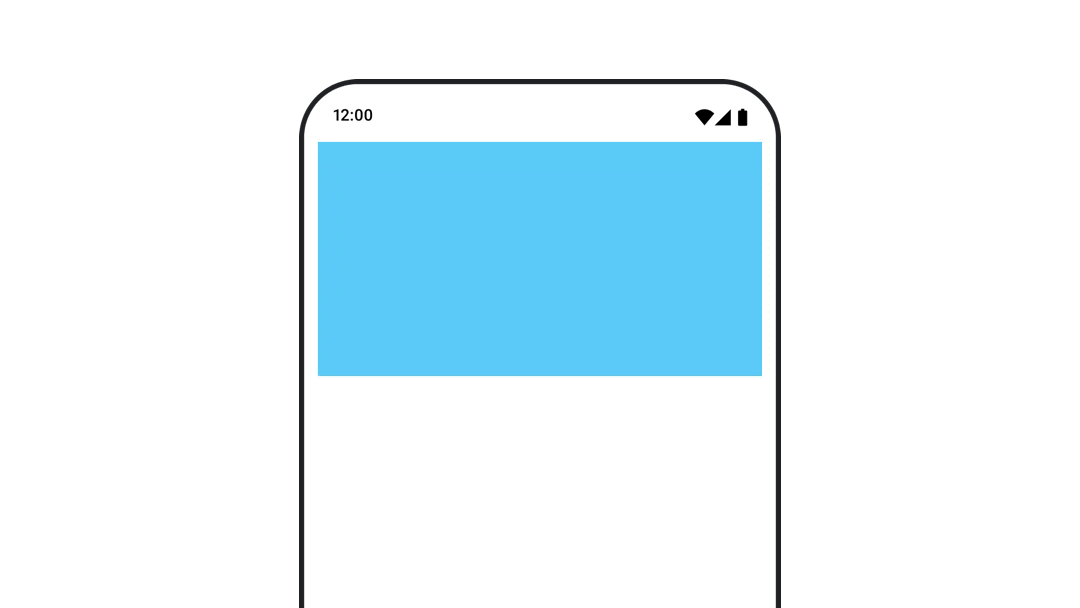
借助 Compose,您可以通过几种不同的方式为可组合项的大小添加动画效果。在可组合项大小发生变化时,使用 animateContentSize()
实现动画效果。
例如,如果您有一个包含文本的方框,该文本可以从一
那么可以使用 Modifier.animateContentSize()
来实现更流畅的
转换:
var expanded by remember { mutableStateOf(false) } Box( modifier = Modifier .background(colorBlue) .animateContentSize() .height(if (expanded) 400.dp else 200.dp) .fillMaxWidth() .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { expanded = !expanded } ) { }
您还可以使用 AnimatedContent
和 SizeTransform
来描述大小变化的发生方式。
为可组合项的位置添加动画效果
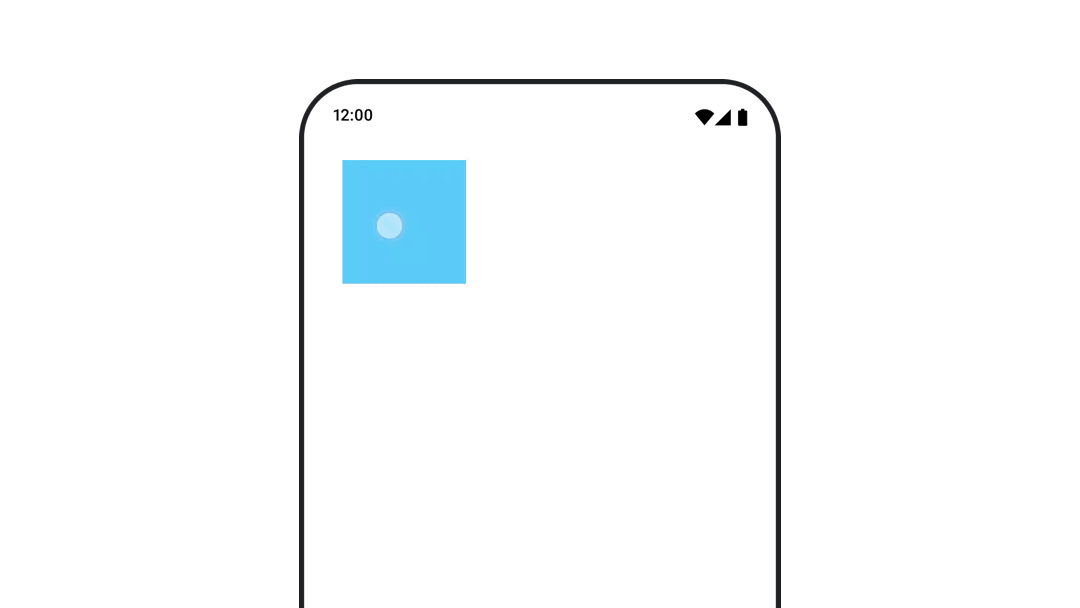
如需为可组合项的位置添加动画效果,请将 Modifier.offset{ }
与
animateIntOffsetAsState()
。
var moved by remember { mutableStateOf(false) } val pxToMove = with(LocalDensity.current) { 100.dp.toPx().roundToInt() } val offset by animateIntOffsetAsState( targetValue = if (moved) { IntOffset(pxToMove, pxToMove) } else { IntOffset.Zero }, label = "offset" ) Box( modifier = Modifier .offset { offset } .background(colorBlue) .size(100.dp) .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { moved = !moved } )
如果您想确保可组合项不会在其他
为位置或大小添加动画效果时,请使用 Modifier.layout{ }
。这个
修饰符会将尺寸和位置更改传播到父视图,而这随后会影响
儿童。
例如,如果您在 Column
中移动 Box
,并且其他子项需要在 Box
移动时移动,请将偏移信息添加到 Modifier.layout{ }
,如下所示:
var toggled by remember { mutableStateOf(false) } val interactionSource = remember { MutableInteractionSource() } Column( modifier = Modifier .padding(16.dp) .fillMaxSize() .clickable(indication = null, interactionSource = interactionSource) { toggled = !toggled } ) { val offsetTarget = if (toggled) { IntOffset(150, 150) } else { IntOffset.Zero } val offset = animateIntOffsetAsState( targetValue = offsetTarget, label = "offset" ) Box( modifier = Modifier .size(100.dp) .background(colorBlue) ) Box( modifier = Modifier .layout { measurable, constraints -> val offsetValue = if (isLookingAhead) offsetTarget else offset.value val placeable = measurable.measure(constraints) layout(placeable.width + offsetValue.x, placeable.height + offsetValue.y) { placeable.placeRelative(offsetValue) } } .size(100.dp) .background(colorGreen) ) Box( modifier = Modifier .size(100.dp) .background(colorBlue) ) }
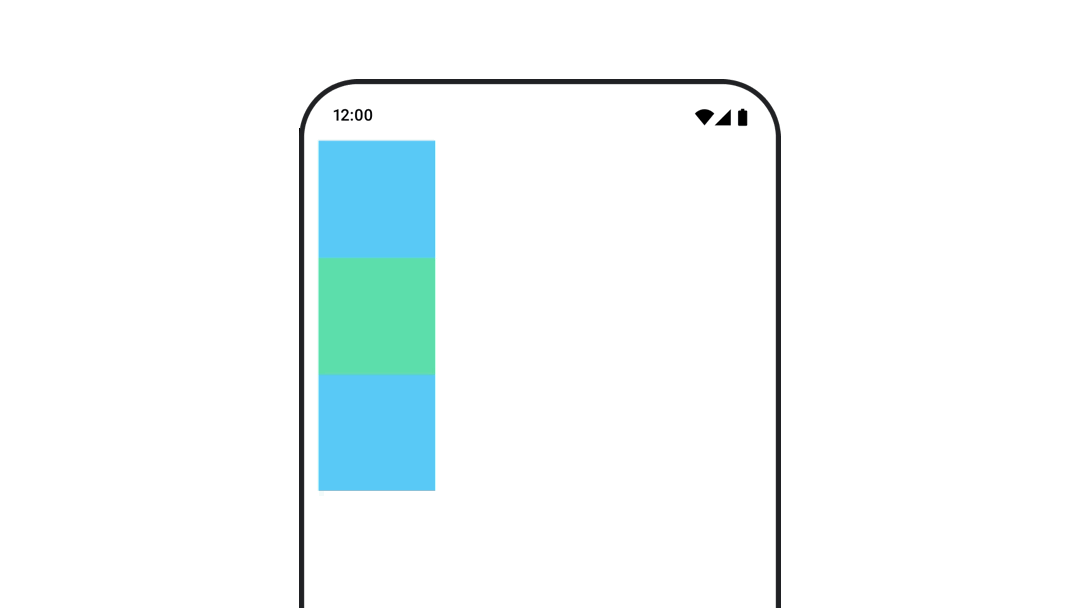
Modifier.layout{ }
添加动画效果为可组合项的内边距添加动画效果
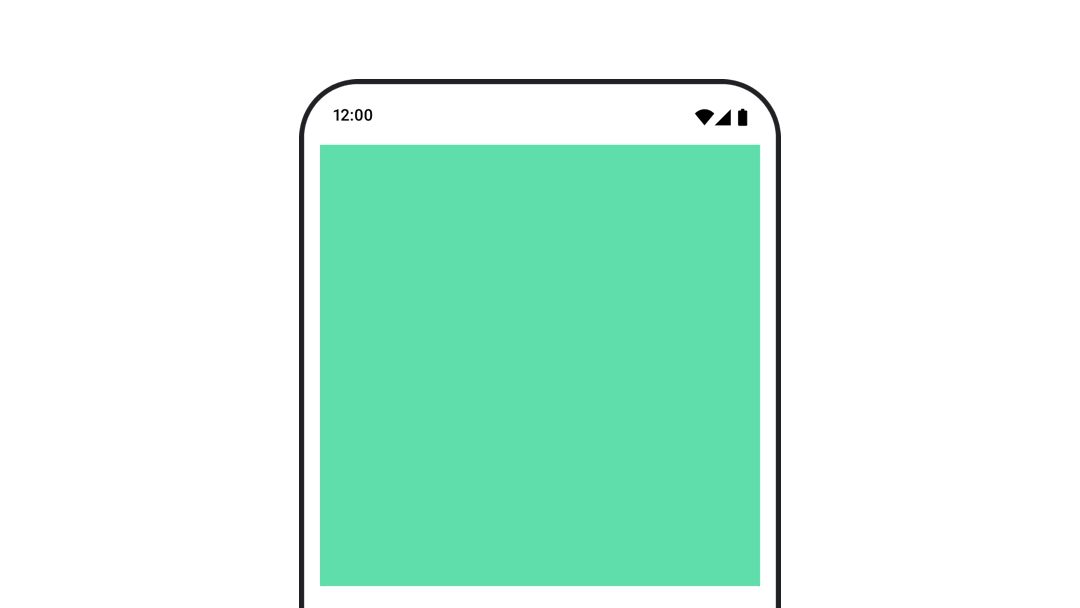
如需为可组合项的内边距添加动画效果,请将 animateDpAsState
与 Modifier.padding()
结合使用:
var toggled by remember { mutableStateOf(false) } val animatedPadding by animateDpAsState( if (toggled) { 0.dp } else { 20.dp }, label = "padding" ) Box( modifier = Modifier .aspectRatio(1f) .fillMaxSize() .padding(animatedPadding) .background(Color(0xff53D9A1)) .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { toggled = !toggled } )
为可组合项的高度添加动画效果
如需为可组合项的高度添加动画效果,请将 animateDpAsState
与
Modifier.graphicsLayer{ }
。对于一次性的高度更改,请使用
Modifier.shadow()
。如果您要为阴影添加动画效果,使用 Modifier.graphicsLayer{ }
修饰符是性能更高的选项。
val mutableInteractionSource = remember { MutableInteractionSource() } val pressed = mutableInteractionSource.collectIsPressedAsState() val elevation = animateDpAsState( targetValue = if (pressed.value) { 32.dp } else { 8.dp }, label = "elevation" ) Box( modifier = Modifier .size(100.dp) .align(Alignment.Center) .graphicsLayer { this.shadowElevation = elevation.value.toPx() } .clickable(interactionSource = mutableInteractionSource, indication = null) { } .background(colorGreen) ) { }
或者,使用 Card
可组合项,并将 elevation 属性设置为每种状态的不同值。
为文本缩放、平移或旋转添加动画效果
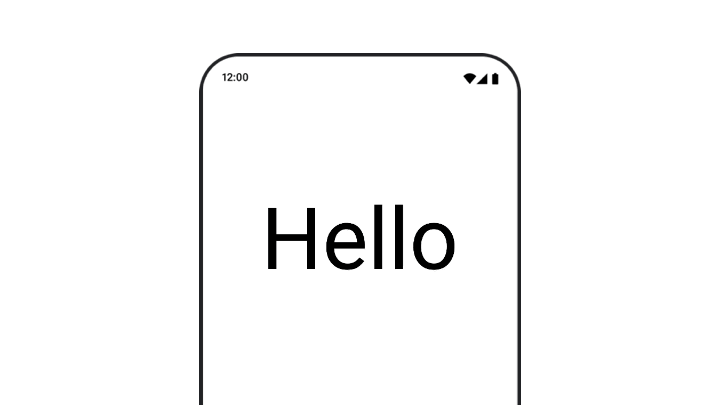
在为文本的缩放、平移或旋转添加动画效果时,请设置 textMotion
将 TextStyle
的参数设置为 TextMotion.Animated
。这样可以确保文本动画之间的转换更流畅。使用 Modifier.graphicsLayer{ }
平移、旋转或缩放文本。
val infiniteTransition = rememberInfiniteTransition(label = "infinite transition") val scale by infiniteTransition.animateFloat( initialValue = 1f, targetValue = 8f, animationSpec = infiniteRepeatable(tween(1000), RepeatMode.Reverse), label = "scale" ) Box(modifier = Modifier.fillMaxSize()) { Text( text = "Hello", modifier = Modifier .graphicsLayer { scaleX = scale scaleY = scale transformOrigin = TransformOrigin.Center } .align(Alignment.Center), // Text composable does not take TextMotion as a parameter. // Provide it via style argument but make sure that we are copying from current theme style = LocalTextStyle.current.copy(textMotion = TextMotion.Animated) ) }
为文本颜色添加动画效果
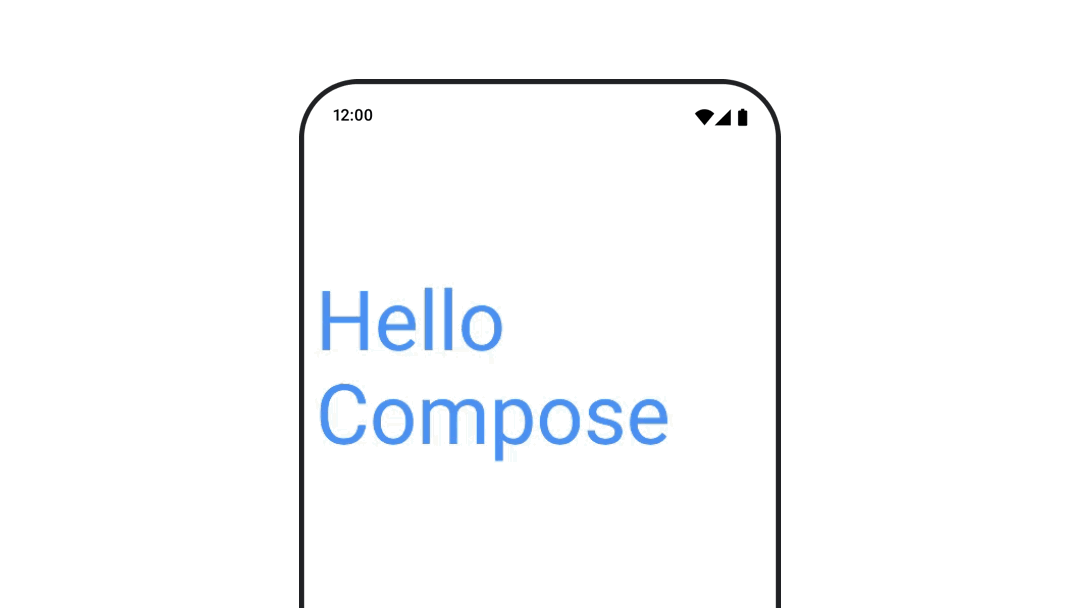
如需为文本颜色添加动画效果,请在 BasicText
可组合项中使用 color
lambda:
val infiniteTransition = rememberInfiniteTransition(label = "infinite transition") val animatedColor by infiniteTransition.animateColor( initialValue = Color(0xFF60DDAD), targetValue = Color(0xFF4285F4), animationSpec = infiniteRepeatable(tween(1000), RepeatMode.Reverse), label = "color" ) BasicText( text = "Hello Compose", color = { animatedColor }, // ... )
在不同类型的内容之间切换
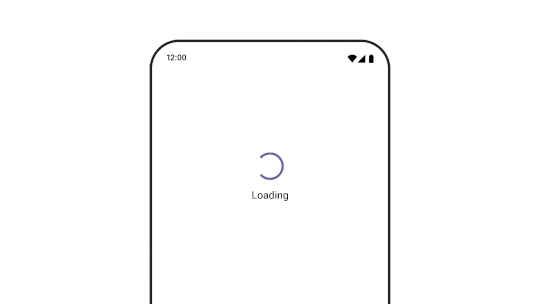
使用 AnimatedContent
在不同的可组合项之间添加动画效果;如果您只希望在可组合项之间呈现标准淡出效果,请使用 Crossfade
。
var state by remember { mutableStateOf(UiState.Loading) } AnimatedContent( state, transitionSpec = { fadeIn( animationSpec = tween(3000) ) togetherWith fadeOut(animationSpec = tween(3000)) }, modifier = Modifier.clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { state = when (state) { UiState.Loading -> UiState.Loaded UiState.Loaded -> UiState.Error UiState.Error -> UiState.Loading } }, label = "Animated Content" ) { targetState -> when (targetState) { UiState.Loading -> { LoadingScreen() } UiState.Loaded -> { LoadedScreen() } UiState.Error -> { ErrorScreen() } } }
您可以对AnimatedContent
进行自定义,以显示多种不同的输入和
退出过渡。有关详情,请参阅
AnimatedContent
或阅读此博文
AnimatedContent
。
在导航到不同目的地时呈现动画效果
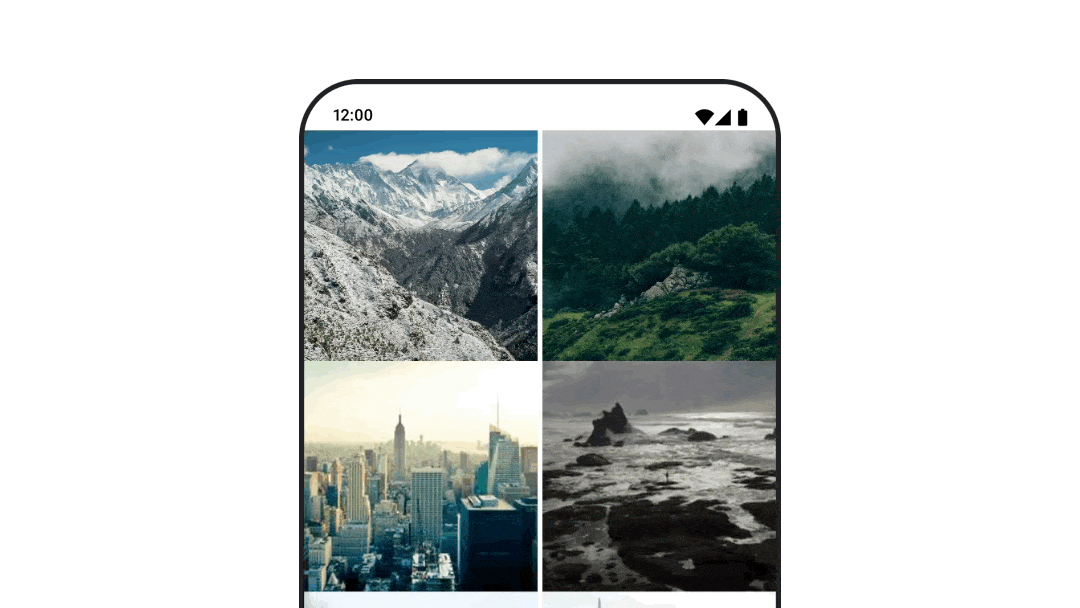
如需在使用
navigation-compose 工件,指定 enterTransition
并
针对可组合项的 exitTransition
。您还可以将默认动画设置为
用于顶级 NavHost
中的所有目的地:
val navController = rememberNavController() NavHost( navController = navController, startDestination = "landing", enterTransition = { EnterTransition.None }, exitTransition = { ExitTransition.None } ) { composable("landing") { ScreenLanding( // ... ) } composable( "detail/{photoUrl}", arguments = listOf(navArgument("photoUrl") { type = NavType.StringType }), enterTransition = { fadeIn( animationSpec = tween( 300, easing = LinearEasing ) ) + slideIntoContainer( animationSpec = tween(300, easing = EaseIn), towards = AnimatedContentTransitionScope.SlideDirection.Start ) }, exitTransition = { fadeOut( animationSpec = tween( 300, easing = LinearEasing ) ) + slideOutOfContainer( animationSpec = tween(300, easing = EaseOut), towards = AnimatedContentTransitionScope.SlideDirection.End ) } ) { backStackEntry -> ScreenDetails( // ... ) } }
有许多不同类型的进入和退出转场效果,可对传入和传出内容应用不同的效果,如需了解详情,请参阅文档。
重复动画
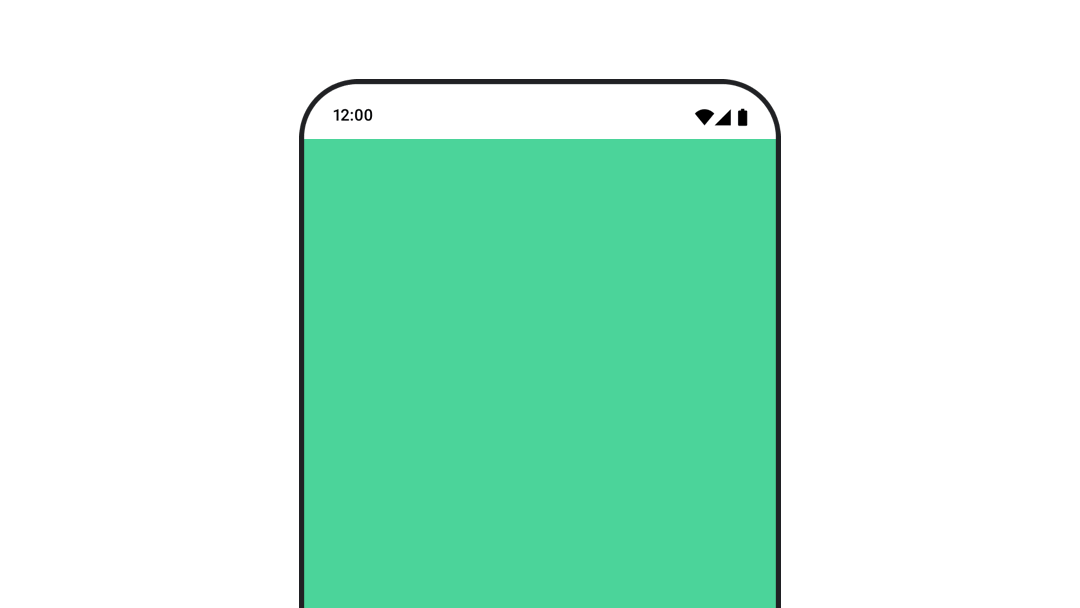
将 rememberInfiniteTransition
与 infiniteRepeatable
animationSpec
搭配使用,可让动画不断重复播放。将“RepeatModes
”更改为
指定它的往返方式
使用 finiteRepeatable
重复设置的次数。
val infiniteTransition = rememberInfiniteTransition(label = "infinite") val color by infiniteTransition.animateColor( initialValue = Color.Green, targetValue = Color.Blue, animationSpec = infiniteRepeatable( animation = tween(1000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "color" ) Column( modifier = Modifier.drawBehind { drawRect(color) } ) { // your composable here }
在启动可组合项时启动动画
当可组合项进入组合时,系统会运行 LaunchedEffect
。它会在可组合项启动时启动动画,您可以使用它来驱动动画状态更改。结合使用 Animatable
和 animateTo
方法启动
启动时的动画:
val alphaAnimation = remember { Animatable(0f) } LaunchedEffect(Unit) { alphaAnimation.animateTo(1f) } Box( modifier = Modifier.graphicsLayer { alpha = alphaAnimation.value } )
创建顺序动画
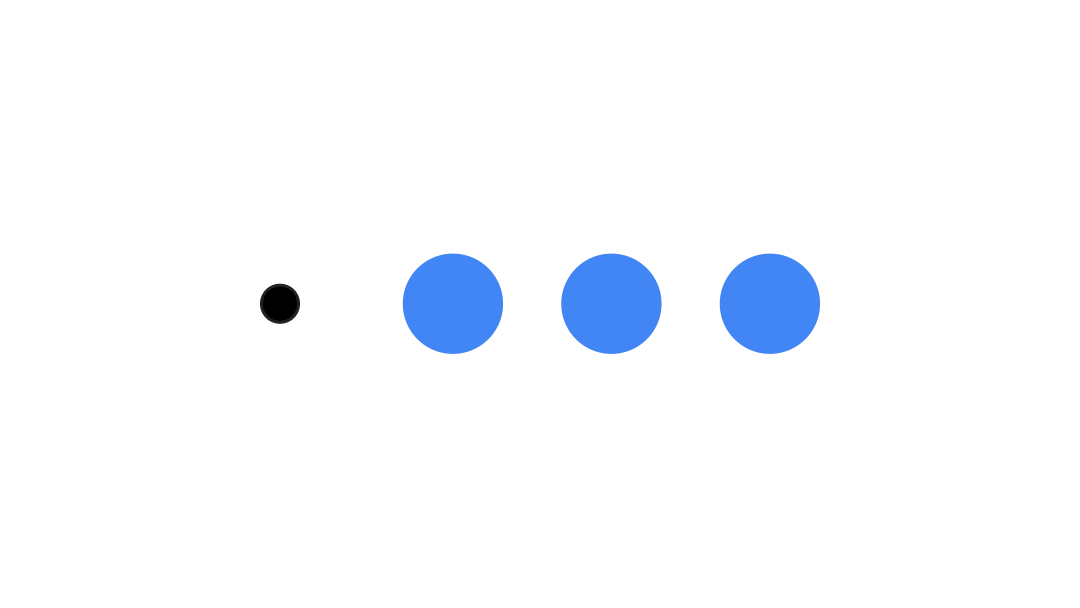
使用 Animatable
协程 API 执行顺序或并发动画。依次对 Animatable
调用 animateTo
让每个动画都等待前一个动画播放完毕后再继续 。
这是因为它是一个挂起函数。
val alphaAnimation = remember { Animatable(0f) } val yAnimation = remember { Animatable(0f) } LaunchedEffect("animationKey") { alphaAnimation.animateTo(1f) yAnimation.animateTo(100f) yAnimation.animateTo(500f, animationSpec = tween(100)) }
创建并发动画
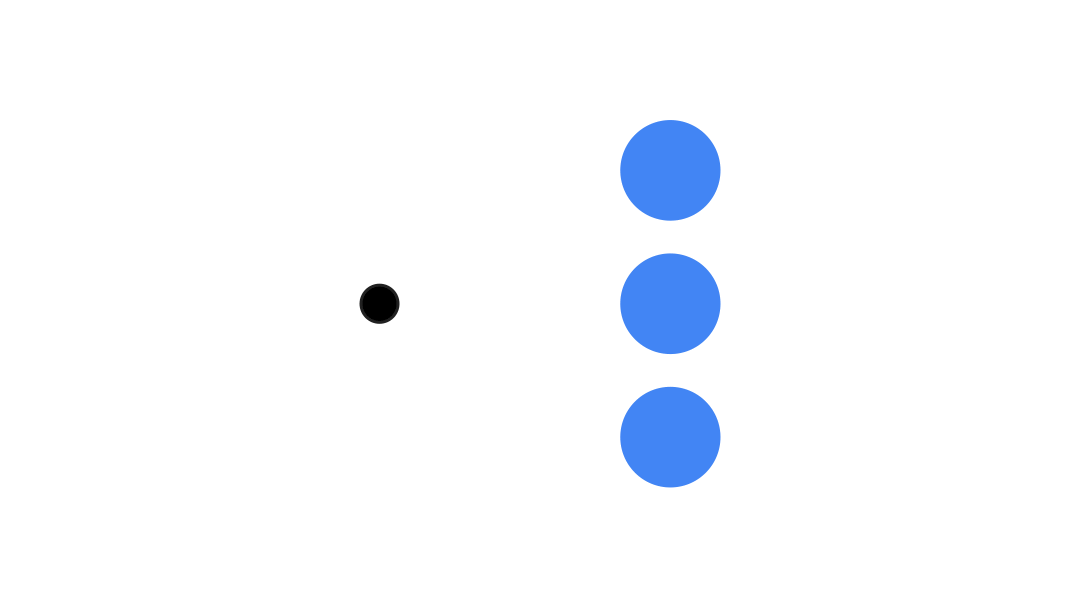
使用协程 API(Animatable#animateTo()
或 animate
),或
Transition
API 来实现并发动画。如果您在协程上下文中使用多个启动函数,系统会同时启动动画:
val alphaAnimation = remember { Animatable(0f) } val yAnimation = remember { Animatable(0f) } LaunchedEffect("animationKey") { launch { alphaAnimation.animateTo(1f) } launch { yAnimation.animateTo(100f) } }
您可以使用 updateTransition
API 使用相同的状态来推动
多个不同的属性动画。以下示例为由状态更改控制的两个属性(rect
和 borderWidth
)添加了动画效果:
var currentState by remember { mutableStateOf(BoxState.Collapsed) } val transition = updateTransition(currentState, label = "transition") val rect by transition.animateRect(label = "rect") { state -> when (state) { BoxState.Collapsed -> Rect(0f, 0f, 100f, 100f) BoxState.Expanded -> Rect(100f, 100f, 300f, 300f) } } val borderWidth by transition.animateDp(label = "borderWidth") { state -> when (state) { BoxState.Collapsed -> 1.dp BoxState.Expanded -> 0.dp } }
优化动画性能
Compose 中的动画可能会导致性能问题。这是由于动画的性质所致:逐帧快速移动或更改屏幕上的像素,以营造移动的错觉。
考虑 Compose 的不同阶段:组合、布局和绘制。如果 您的动画会更改布局阶段,因此需要所有受影响的可组合项 重新布局和绘制。如果动画在绘制阶段发生,则默认情况下,其性能会比在布局阶段运行动画更好,因为总体工作量会更少。
为了确保应用在呈现动画时尽可能少执行操作,请尽可能选择 Modifier
的 lambda 版本。这会跳过重组并在组合阶段之外执行动画,否则请使用 Modifier.graphicsLayer{ }
,因为此修饰符始终在绘制阶段运行。如需了解详情,请参阅性能文档中的推迟读取部分。
更改动画时间设置
默认情况下,Compose 会对大多数动画使用弹簧动画。Springs 或
基于物理特性的动画,让您感觉更自然。它们还可中断,因为它们会考虑物体的当前速度,而不是固定时间。如果要替换默认值,可以使用上面演示的所有动画 API
能够设置 animationSpec
以自定义动画的运行方式,
是希望它在一定的时间内执行,还是提高跳动性
以下简要介绍了不同的 animationSpec
选项:
spring
:基于物理特性的动画,所有动画的默认设置。您 通过改变刚度或 dampingRatio 外观。tween
(简称“between”):基于时长的动画,使用Easing
函数在两个值之间添加动画效果。keyframes
:用于在 动画。repeatable
:基于时长的规范,会运行RepeatMode
指定的次数。infiniteRepeatable
:基于时长的规范,会一直运行。snap
:立即贴靠结束值,而不使用任何动画。
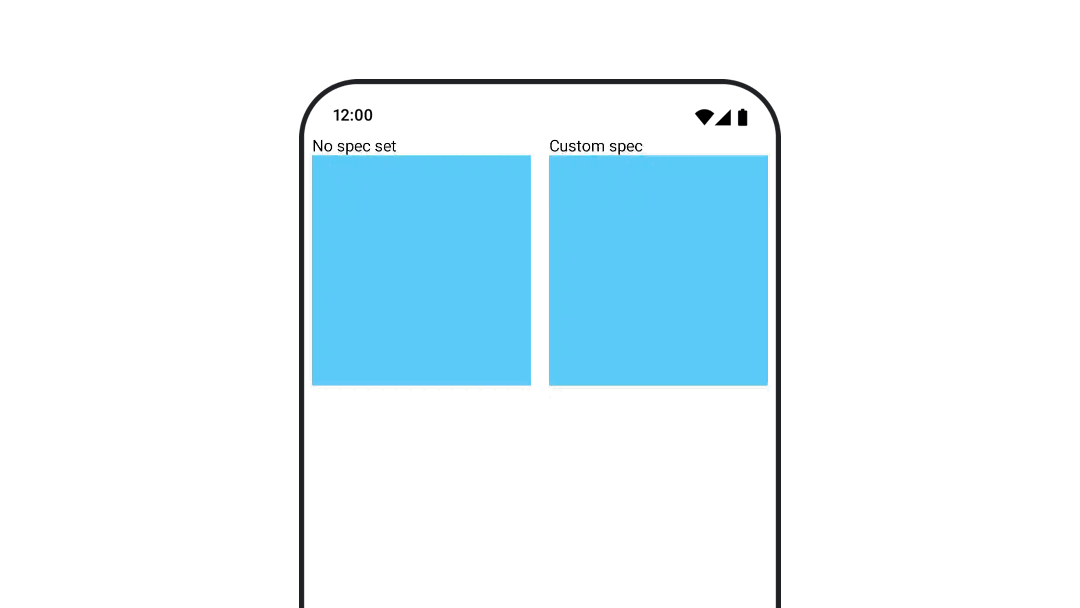
如需详细了解 animationSpec,请参阅完整文档。
其他资源
如需查看 Compose 中更多有趣动画的示例,请参阅以下内容: