本指南介绍了如何在 Compose 中创建动态顶部应用栏,以便在从列表中选择项时更改其选项。您可以根据选择状态修改顶部应用栏的标题和操作。
实现动态顶部应用栏行为
以下代码为顶部应用栏定义了一个可组合函数,该函数会根据项选择而发生变化:
@Composable fun AppBarSelectionActions( selectedItems: Set<Int>, modifier: Modifier = Modifier, ) { val hasSelection = selectedItems.isNotEmpty() val topBarText = if (hasSelection) { "Selected ${selectedItems.size} items" } else { "List of items" } TopAppBar( title = { Text(topBarText) }, colors = TopAppBarDefaults.topAppBarColors( containerColor = MaterialTheme.colorScheme.primaryContainer, titleContentColor = MaterialTheme.colorScheme.primary, ), actions = { if (hasSelection) { IconButton(onClick = { /* click action */ }) { Icon( imageVector = Icons.Filled.Share, contentDescription = "Share items" ) } } }, ) }
代码要点
AppBarSelectionActions
接受所选项索引的Set
。topBarText
会根据是否选择了任何项而发生变化。- 选择项目后,
TopAppBar
中会显示描述所选项目数量的文本。 - 如果未选择任何项,
topBarText
为“项列表”。
- 选择项目后,
actions
块用于定义顶部应用栏中显示的操作。如果hasSelection
为 true,则文本后面会显示一个分享图标。IconButton
的onClick
lambda 会在用户点击图标时处理分享操作。
结果

将可选列表集成到动态顶部应用栏中
以下示例演示了如何向动态顶部应用栏添加可选择列表:
@Composable private fun AppBarMultiSelectionExample( modifier: Modifier = Modifier, ) { val listItems by remember { mutableStateOf(listOf(1, 2, 3, 4, 5, 6)) } var selectedItems by rememberSaveable { mutableStateOf(setOf<Int>()) } Scaffold( topBar = { AppBarSelectionActions(selectedItems) } ) { innerPadding -> LazyColumn(contentPadding = innerPadding) { itemsIndexed(listItems) { _, index -> val isItemSelected = selectedItems.contains(index) ListItemSelectable( selected = isItemSelected, Modifier .combinedClickable( interactionSource = remember { MutableInteractionSource() }, indication = null, onClick = { /* click action */ }, onLongClick = { if (isItemSelected) selectedItems -= index else selectedItems += index } ) ) } } } }
代码要点
- 顶部栏会根据所选列表项的数量进行更新。
selectedItems
用于存储一组所选项的索引。AppBarMultiSelectionExample
使用Scaffold
来构建界面。topBar = { AppBarSelectionActions(selectedItems) }
将AppBarSelectionActions
可组合项设置为顶部应用栏。AppBarSelectionActions
收到selectedItems
状态。
LazyColumn
以垂直列表的形式显示项,只渲染屏幕上可见的项。ListItemSelectable
表示可选择的列表项。combinedClickable
允许同时处理点击和长按操作,以便选择项。点击会执行操作,而长按某项内容会切换其选择状态。
结果
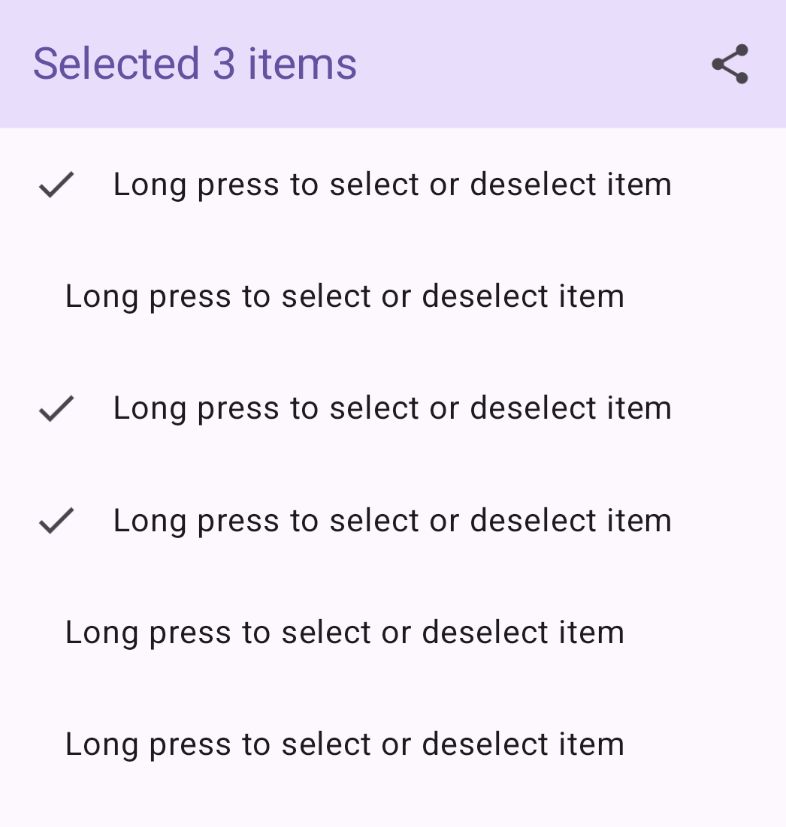