การเขียนมีกลไกภาพเคลื่อนไหวในตัวมากมาย และอาจทำให้ รู้ว่าต้องเลือกอันไหน ด้านล่างนี้คือรายการ Use Case ทั่วไปของแอนิเมชัน อ่านเอกสารประกอบเกี่ยวกับการสร้างภาพเคลื่อนไหวฉบับเต็มเพื่อดูรายละเอียดเพิ่มเติมเกี่ยวกับตัวเลือก API ต่างๆ ทั้งหมดที่ใช้ได้
สร้างภาพเคลื่อนไหวของพร็อพเพอร์ตี้คอมโพสิเบิลทั่วไป
Compose มี API ที่สะดวกซึ่งช่วยให้คุณแก้ปัญหากรณีการใช้งานภาพเคลื่อนไหวที่พบได้ทั่วไปได้หลายกรณี ส่วนนี้จะสาธิตวิธีสร้างภาพเคลื่อนไหวให้กับพร็อพเพอร์ตี้ทั่วไปของคอมโพสิเบิล
ภาพเคลื่อนไหวที่ปรากฏ / หายไป
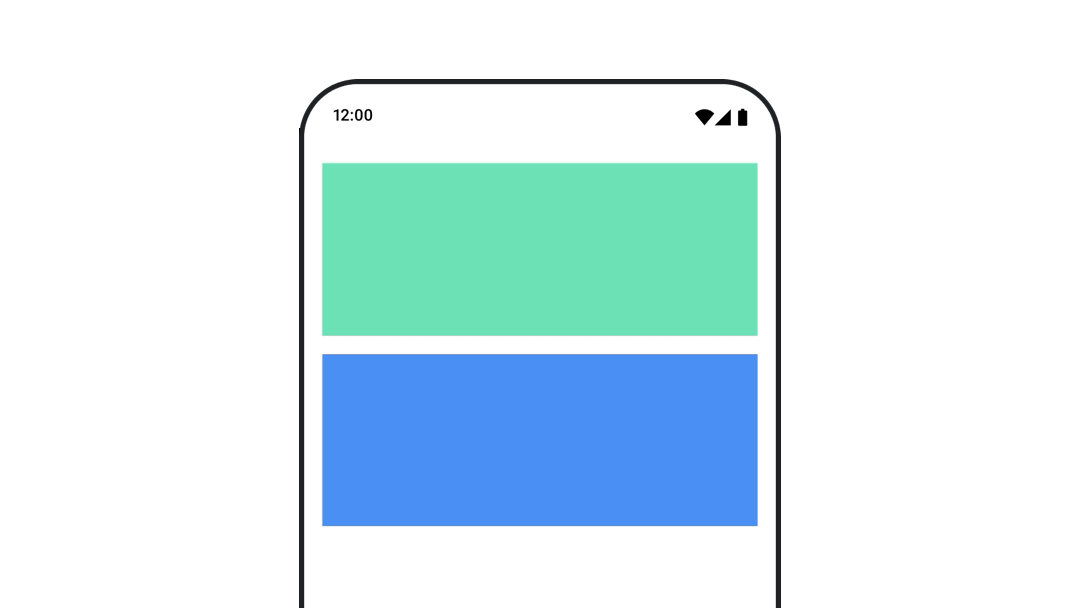
ใช้ AnimatedVisibility
เพื่อซ่อนหรือแสดง Composable เด็กที่อยู่ใน AnimatedVisibility
สามารถใช้ Modifier.animateEnterExit()
สำหรับทรานซิชันเข้าหรือออกของตนเอง
var visible by remember { mutableStateOf(true) } // Animated visibility will eventually remove the item from the composition once the animation has finished. AnimatedVisibility(visible) { // your composable here // ... }
พารามิเตอร์ "เข้า" และ "ออก" ของ AnimatedVisibility
ช่วยให้คุณกําหนดค่าลักษณะการทํางานของคอมโพสิเบิลเมื่อปรากฏขึ้นและหายไป อ่านข้อมูลเพิ่มเติมในเอกสารประกอบฉบับเต็ม
อีกตัวเลือกหนึ่งสำหรับการทำให้การมองเห็น Composable เคลื่อนไหวคือการทำให้แท็ก
เมื่อเวลาผ่านไปโดยใช้ animateFloatAsState
:
var visible by remember { mutableStateOf(true) } val animatedAlpha by animateFloatAsState( targetValue = if (visible) 1.0f else 0f, label = "alpha" ) Box( modifier = Modifier .size(200.dp) .graphicsLayer { alpha = animatedAlpha } .clip(RoundedCornerShape(8.dp)) .background(colorGreen) .align(Alignment.TopCenter) ) { }
อย่างไรก็ตาม การเปลี่ยนอัลฟ่ามาพร้อมกับข้อควรระวังว่า Composable นั้นยังคงอยู่
ในการเรียบเรียงและยังคงครอบคลุมพื้นที่ที่วางองค์ประกอบไว้ ช่วงเวลานี้
อาจทำให้โปรแกรมอ่านหน้าจอและกลไกการเข้าถึงอื่นๆ
รายการบนหน้าจอ ในทางกลับกัน AnimatedVisibility
จะนํารายการออกจากการคอมโพสในที่สุด
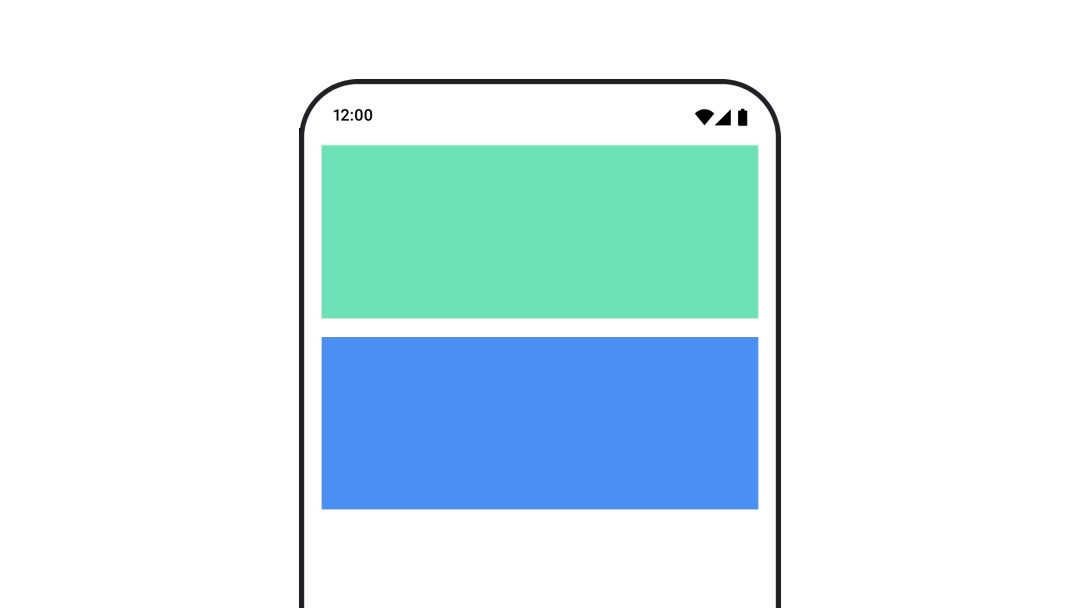
เคลื่อนไหวสีพื้นหลัง
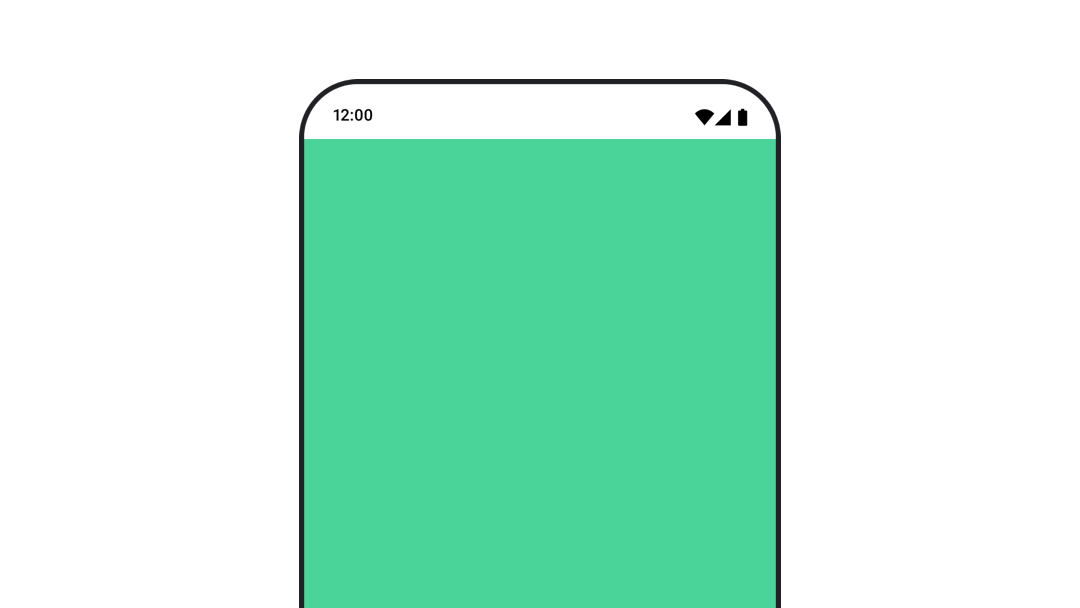
val animatedColor by animateColorAsState( if (animateBackgroundColor) colorGreen else colorBlue, label = "color" ) Column( modifier = Modifier.drawBehind { drawRect(animatedColor) } ) { // your composable here }
ตัวเลือกนี้มีประสิทธิภาพมากกว่าการใช้ Modifier.background()
Modifier.background()
ยอมรับได้สำหรับการตั้งค่าสีแบบครั้งเดียว แต่เมื่อสร้างภาพเคลื่อนไหวของสีเมื่อเวลาผ่านไป การตั้งค่านี้อาจทําให้ต้องจัดองค์ประกอบใหม่มากกว่าที่จําเป็น
หากต้องการให้สีพื้นหลังเคลื่อนไหวได้ไม่รู้จบ โปรดดูการสร้างภาพเคลื่อนไหวซ้ำๆ
ทำให้ขนาดของ Composable เคลื่อนไหว
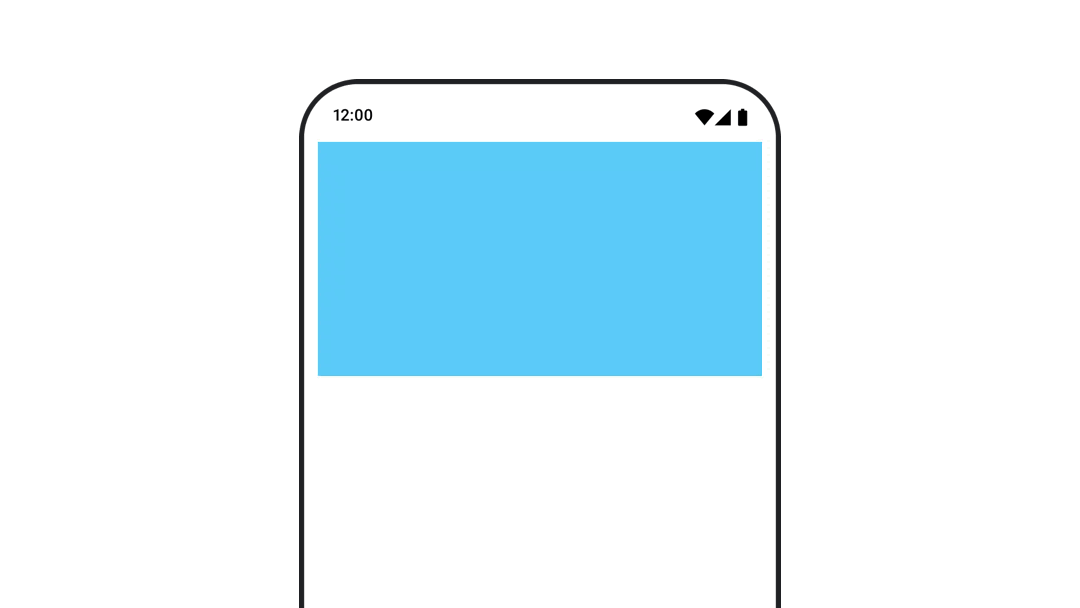
คอมโพสิชันช่วยให้คุณสร้างภาพเคลื่อนไหวขนาดของคอมโพสิเบิลได้หลายวิธี ใช้
animateContentSize()
สำหรับภาพเคลื่อนไหวระหว่างการเปลี่ยนขนาดที่เขียนได้ด้วยที่ประกอบกันได้
ตัวอย่างเช่น หากคุณมีช่องที่มีข้อความซึ่งสามารถขยายจาก 1 เป็น
หลายบรรทัดที่คุณสามารถใช้ Modifier.animateContentSize()
เพื่อให้
การเปลี่ยน:
var expanded by remember { mutableStateOf(false) } Box( modifier = Modifier .background(colorBlue) .animateContentSize() .height(if (expanded) 400.dp else 200.dp) .fillMaxWidth() .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { expanded = !expanded } ) { }
คุณยังใช้ AnimatedContent
ร่วมกับ SizeTransform
เพื่ออธิบายวิธีเปลี่ยนขนาดได้ด้วย
ภาพเคลื่อนไหวตำแหน่งของ Composable
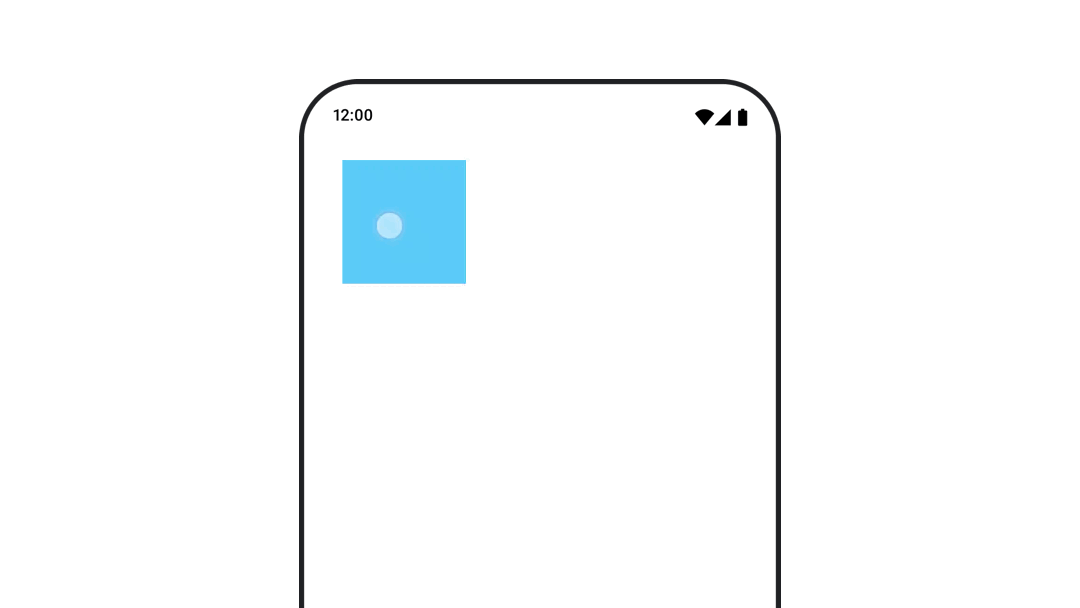
หากต้องการทำให้ตำแหน่งของ Composable เคลื่อนไหว ให้ใช้ Modifier.offset{ }
ร่วมกับ
animateIntOffsetAsState()
var moved by remember { mutableStateOf(false) } val pxToMove = with(LocalDensity.current) { 100.dp.toPx().roundToInt() } val offset by animateIntOffsetAsState( targetValue = if (moved) { IntOffset(pxToMove, pxToMove) } else { IntOffset.Zero }, label = "offset" ) Box( modifier = Modifier .offset { offset } .background(colorBlue) .size(100.dp) .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { moved = !moved } )
หากต้องการให้แน่ใจว่า Composable ไม่ได้วาดทับหรืออยู่ใต้ส่วนอื่น
Composable เมื่อทำให้ตำแหน่งหรือขนาดเคลื่อนไหว ให้ใช้ Modifier.layout{ }
ตัวแก้ไขนี้จะส่งต่อการเปลี่ยนแปลงขนาดและตําแหน่งไปยังองค์ประกอบหลัก ซึ่งจะส่งผลต่อองค์ประกอบย่อยอื่นๆ
เช่น หากคุณย้าย Box
ภายใน Column
และแท็กย่อยอื่นๆ
ที่ต้องเคลื่อนที่เมื่อ Box
ขยับ ให้ใส่ข้อมูลออฟเซ็ตด้วย
Modifier.layout{ }
ดังนี้
var toggled by remember { mutableStateOf(false) } val interactionSource = remember { MutableInteractionSource() } Column( modifier = Modifier .padding(16.dp) .fillMaxSize() .clickable(indication = null, interactionSource = interactionSource) { toggled = !toggled } ) { val offsetTarget = if (toggled) { IntOffset(150, 150) } else { IntOffset.Zero } val offset = animateIntOffsetAsState( targetValue = offsetTarget, label = "offset" ) Box( modifier = Modifier .size(100.dp) .background(colorBlue) ) Box( modifier = Modifier .layout { measurable, constraints -> val offsetValue = if (isLookingAhead) offsetTarget else offset.value val placeable = measurable.measure(constraints) layout(placeable.width + offsetValue.x, placeable.height + offsetValue.y) { placeable.placeRelative(offsetValue) } } .size(100.dp) .background(colorGreen) ) Box( modifier = Modifier .size(100.dp) .background(colorBlue) ) }
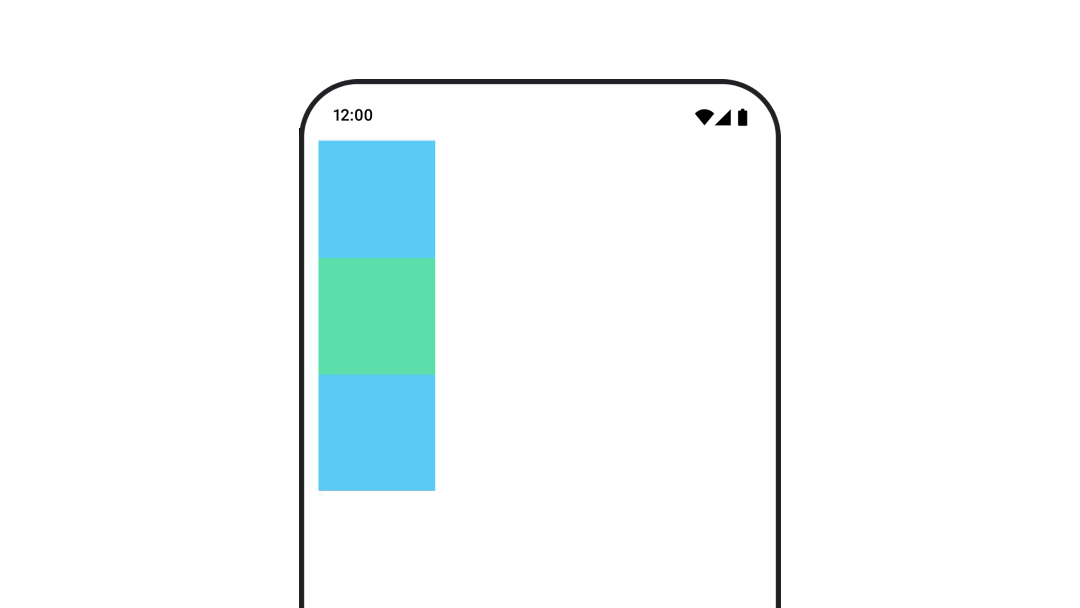
Modifier.layout{ }
สร้างภาพเคลื่อนไหวของระยะห่างจากขอบของคอมโพเนนต์ที่เขียนด้วย Compose
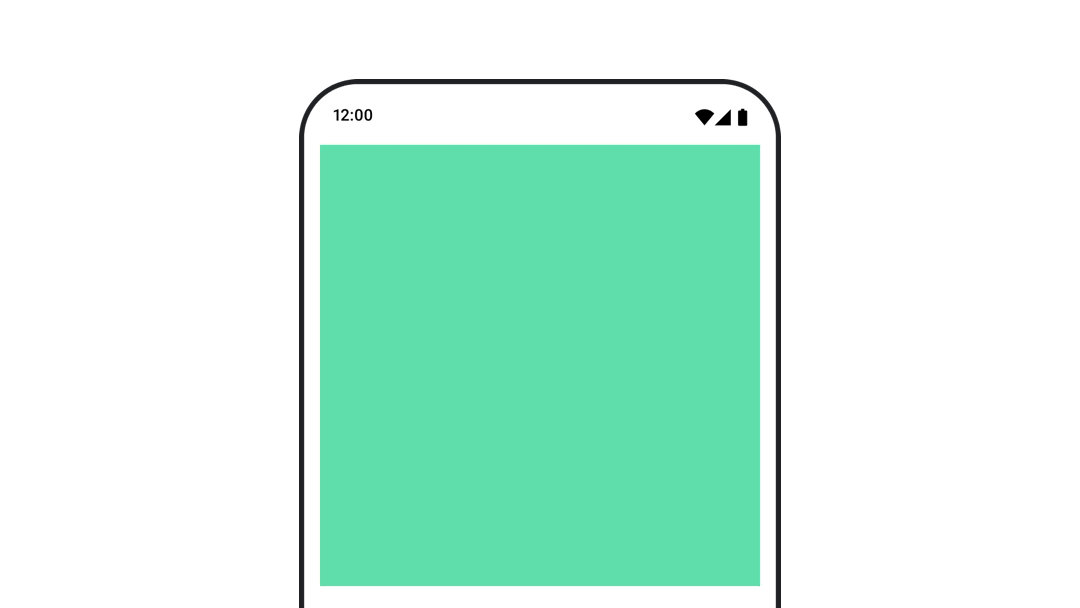
หากต้องการสร้างภาพเคลื่อนไหวให้กับระยะห่างจากขอบของ Composable ให้ใช้ animateDpAsState
ร่วมกับ
Modifier.padding()
:
var toggled by remember { mutableStateOf(false) } val animatedPadding by animateDpAsState( if (toggled) { 0.dp } else { 20.dp }, label = "padding" ) Box( modifier = Modifier .aspectRatio(1f) .fillMaxSize() .padding(animatedPadding) .background(Color(0xff53D9A1)) .clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { toggled = !toggled } )
แสดงภาพเคลื่อนไหวการยกระดับคอมโพเนนต์
หากต้องการสร้างการเคลื่อนไหวของ Composable ให้ใช้ animateDpAsState
ร่วมกับ
Modifier.graphicsLayer{ }
สำหรับการเปลี่ยนแปลงระดับความสูงครั้งเดียว ให้ใช้
Modifier.shadow()
หากต้องการสร้างภาพเคลื่อนไหวเงา การใช้ตัวแก้ไข Modifier.graphicsLayer{ }
เป็นตัวเลือกที่มีประสิทธิภาพมากกว่า
val mutableInteractionSource = remember { MutableInteractionSource() } val pressed = mutableInteractionSource.collectIsPressedAsState() val elevation = animateDpAsState( targetValue = if (pressed.value) { 32.dp } else { 8.dp }, label = "elevation" ) Box( modifier = Modifier .size(100.dp) .align(Alignment.Center) .graphicsLayer { this.shadowElevation = elevation.value.toPx() } .clickable(interactionSource = mutableInteractionSource, indication = null) { } .background(colorGreen) ) { }
หรือใช้ Card
Composable และตั้งค่าพร็อพเพอร์ตี้ระดับความสูงเป็น
ค่าที่ต่างกันต่อรัฐ
ทำให้การปรับขนาด การเปลี่ยนตำแหน่ง หรือการหมุนข้อความเคลื่อนไหว
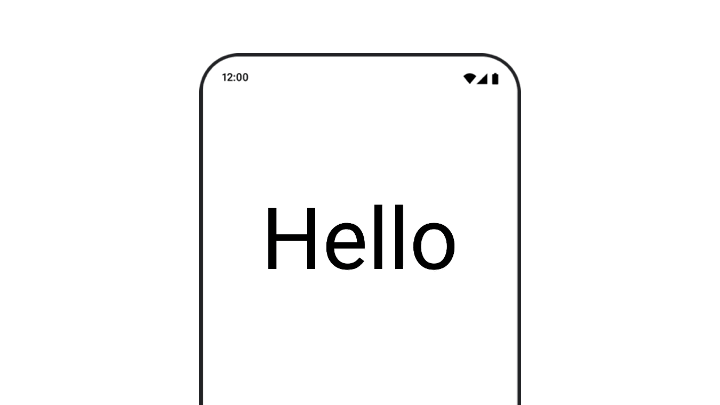
เมื่อแสดงภาพเคลื่อนไหวการปรับขนาด การเปลี่ยนตำแหน่ง หรือการหมุนข้อความ ให้ตั้งค่าพารามิเตอร์ textMotion
ใน TextStyle
เป็น TextMotion.Animated
วิธีนี้ช่วยให้การเปลี่ยนระหว่างภาพเคลื่อนไหวของข้อความเป็นไปอย่างราบรื่น ใช้ Modifier.graphicsLayer{ }
เพื่อแปล หมุน หรือปรับขนาดข้อความ
val infiniteTransition = rememberInfiniteTransition(label = "infinite transition") val scale by infiniteTransition.animateFloat( initialValue = 1f, targetValue = 8f, animationSpec = infiniteRepeatable(tween(1000), RepeatMode.Reverse), label = "scale" ) Box(modifier = Modifier.fillMaxSize()) { Text( text = "Hello", modifier = Modifier .graphicsLayer { scaleX = scale scaleY = scale transformOrigin = TransformOrigin.Center } .align(Alignment.Center), // Text composable does not take TextMotion as a parameter. // Provide it via style argument but make sure that we are copying from current theme style = LocalTextStyle.current.copy(textMotion = TextMotion.Animated) ) }
ทำให้สีข้อความเคลื่อนไหว
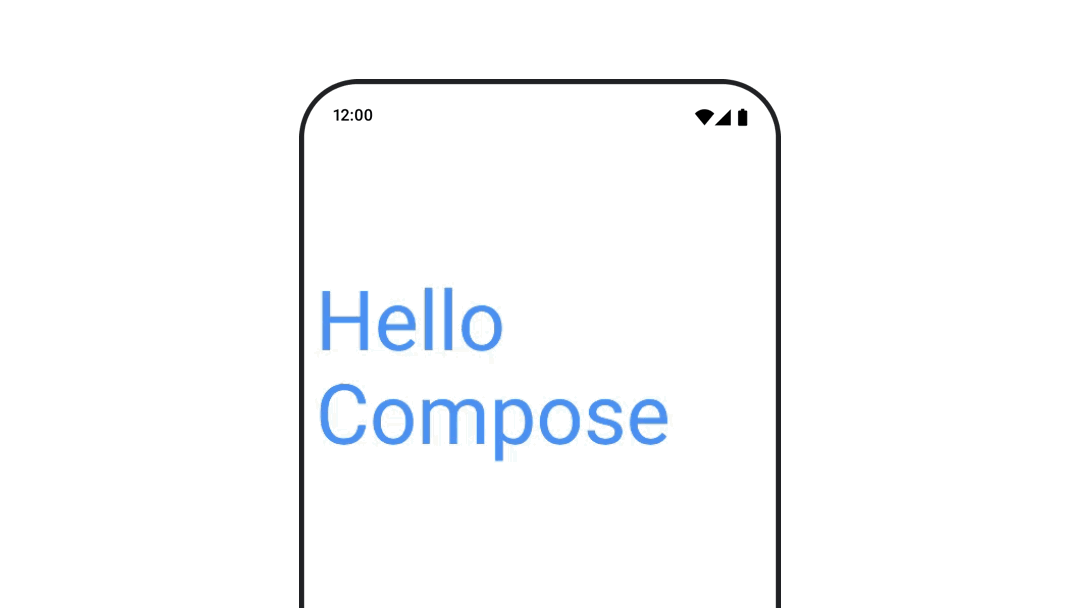
หากต้องการทำให้สีข้อความเคลื่อนไหว ให้ใช้ color
lambda ในคอมโพสิชัน BasicText
ดังนี้
val infiniteTransition = rememberInfiniteTransition(label = "infinite transition") val animatedColor by infiniteTransition.animateColor( initialValue = Color(0xFF60DDAD), targetValue = Color(0xFF4285F4), animationSpec = infiniteRepeatable(tween(1000), RepeatMode.Reverse), label = "color" ) BasicText( text = "Hello Compose", color = { animatedColor }, // ... )
สลับระหว่างเนื้อหาประเภทต่างๆ
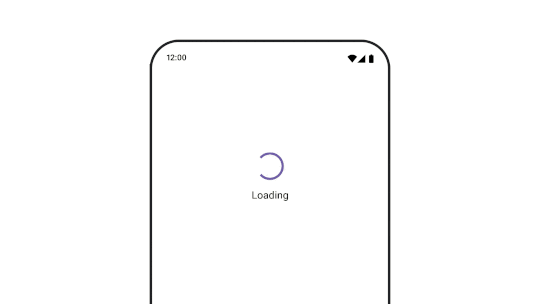
ใช้ AnimatedContent
เพื่อสร้างภาพเคลื่อนไหวระหว่าง Composable ต่างๆ หากคุณ
แค่ต้องการให้ Composable มาตรฐานจางลง ให้ใช้ Crossfade
var state by remember { mutableStateOf(UiState.Loading) } AnimatedContent( state, transitionSpec = { fadeIn( animationSpec = tween(3000) ) togetherWith fadeOut(animationSpec = tween(3000)) }, modifier = Modifier.clickable( interactionSource = remember { MutableInteractionSource() }, indication = null ) { state = when (state) { UiState.Loading -> UiState.Loaded UiState.Loaded -> UiState.Error UiState.Error -> UiState.Loading } }, label = "Animated Content" ) { targetState -> when (targetState) { UiState.Loading -> { LoadingScreen() } UiState.Loaded -> { LoadedScreen() } UiState.Error -> { ErrorScreen() } } }
สามารถปรับแต่ง AnimatedContent
เพื่อแสดงการป้อนและ
ออกจากทรานซิชัน อ่านข้อมูลเพิ่มเติมได้ในเอกสารประกอบเกี่ยวกับ
AnimatedContent
หรืออ่านบล็อกโพสต์นี้เกี่ยวกับ
AnimatedContent
ภาพเคลื่อนไหวขณะไปยังปลายทางต่างๆ
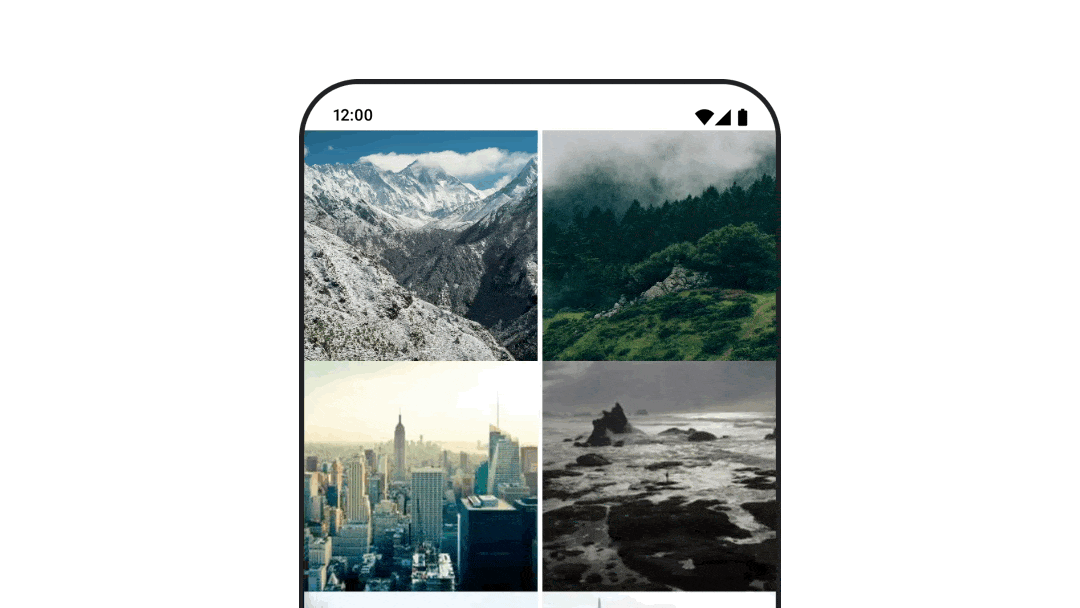
สร้างภาพเคลื่อนไหวการเปลี่ยนระหว่าง Composable เมื่อใช้
navigation-compose จะระบุ enterTransition
และ
exitTransition
ใน Composable นอกจากนี้ คุณยังตั้งค่าภาพเคลื่อนไหวเริ่มต้นให้ใช้กับปลายทางทั้งหมดที่ระดับบนสุด NavHost
ได้ด้วย โดยทำดังนี้
val navController = rememberNavController() NavHost( navController = navController, startDestination = "landing", enterTransition = { EnterTransition.None }, exitTransition = { ExitTransition.None } ) { composable("landing") { ScreenLanding( // ... ) } composable( "detail/{photoUrl}", arguments = listOf(navArgument("photoUrl") { type = NavType.StringType }), enterTransition = { fadeIn( animationSpec = tween( 300, easing = LinearEasing ) ) + slideIntoContainer( animationSpec = tween(300, easing = EaseIn), towards = AnimatedContentTransitionScope.SlideDirection.Start ) }, exitTransition = { fadeOut( animationSpec = tween( 300, easing = LinearEasing ) ) + slideOutOfContainer( animationSpec = tween(300, easing = EaseOut), towards = AnimatedContentTransitionScope.SlideDirection.End ) } ) { backStackEntry -> ScreenDetails( // ... ) } }
ทรานซิชันของช่วงเข้าและช่วงออกมีหลายประเภทที่ใช้เอฟเฟกต์ที่แตกต่างกันกับเนื้อหาขาเข้าและขาออก โปรดดูข้อมูลเพิ่มเติมในเอกสารประกอบ
เล่นภาพเคลื่อนไหวซ้ำ
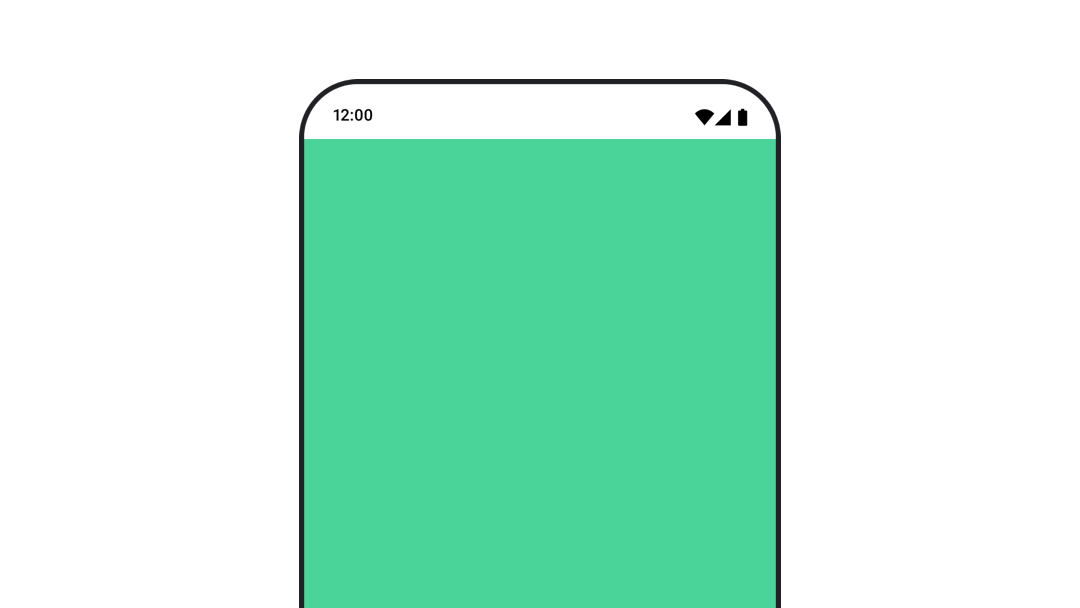
ใช้ rememberInfiniteTransition
ด้วย infiniteRepeatable
animationSpec
เพื่อแสดงภาพเคลื่อนไหวซ้ำไปเรื่อยๆ เปลี่ยน RepeatModes
เพื่อระบุวิธีเลื่อนไปมา
ใช้ finiteRepeatable
เพื่อทำซ้ำตามจำนวนครั้งที่กำหนด
val infiniteTransition = rememberInfiniteTransition(label = "infinite") val color by infiniteTransition.animateColor( initialValue = Color.Green, targetValue = Color.Blue, animationSpec = infiniteRepeatable( animation = tween(1000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "color" ) Column( modifier = Modifier.drawBehind { drawRect(color) } ) { // your composable here }
เริ่มภาพเคลื่อนไหวเมื่อเปิดใช้งานคอมโพสิเบิล
LaunchedEffect
จะทำงานเมื่อคอมโพสิเบิลเข้าสู่การคอมโพสิชัน ฟีเจอร์นี้จะเริ่มต้นภาพเคลื่อนไหวเมื่อเปิดใช้งานคอมโพสิเบิล คุณสามารถใช้ฟีเจอร์นี้เพื่อขับเคลื่อนการเปลี่ยนแปลงสถานะของภาพเคลื่อนไหวได้ การใช้ Animatable
กับเมธอด animateTo
เพื่อเริ่มภาพเคลื่อนไหวเมื่อเปิด
val alphaAnimation = remember { Animatable(0f) } LaunchedEffect(Unit) { alphaAnimation.animateTo(1f) } Box( modifier = Modifier.graphicsLayer { alpha = alphaAnimation.value } )
สร้างภาพเคลื่อนไหวตามลำดับ
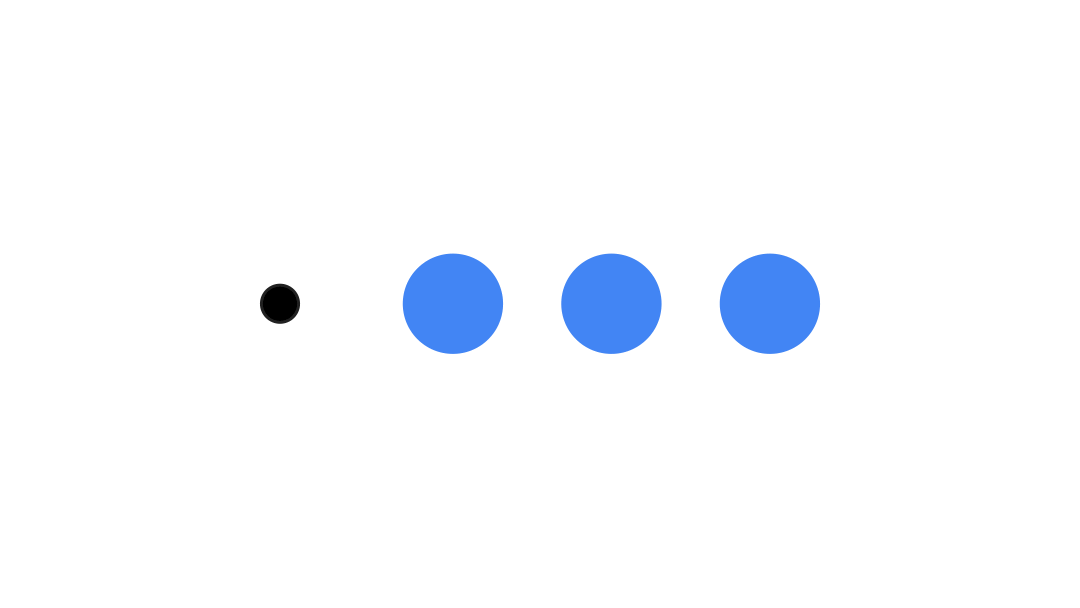
ใช้ coroutine API ของ Animatable
เพื่อดำเนินการตามลำดับหรือพร้อมกัน
ภาพเคลื่อนไหว กำลังโทรหา animateTo
บน Animatable
หลังเกิดเหตุการณ์อื่นๆ
ภาพเคลื่อนไหวแต่ละภาพเพื่อรอให้ภาพเคลื่อนไหวก่อนหน้าจบก่อน จึงดำเนินการต่อ
เพราะเป็นฟังก์ชันระงับ
val alphaAnimation = remember { Animatable(0f) } val yAnimation = remember { Animatable(0f) } LaunchedEffect("animationKey") { alphaAnimation.animateTo(1f) yAnimation.animateTo(100f) yAnimation.animateTo(500f, animationSpec = tween(100)) }
สร้างภาพเคลื่อนไหวพร้อมกัน
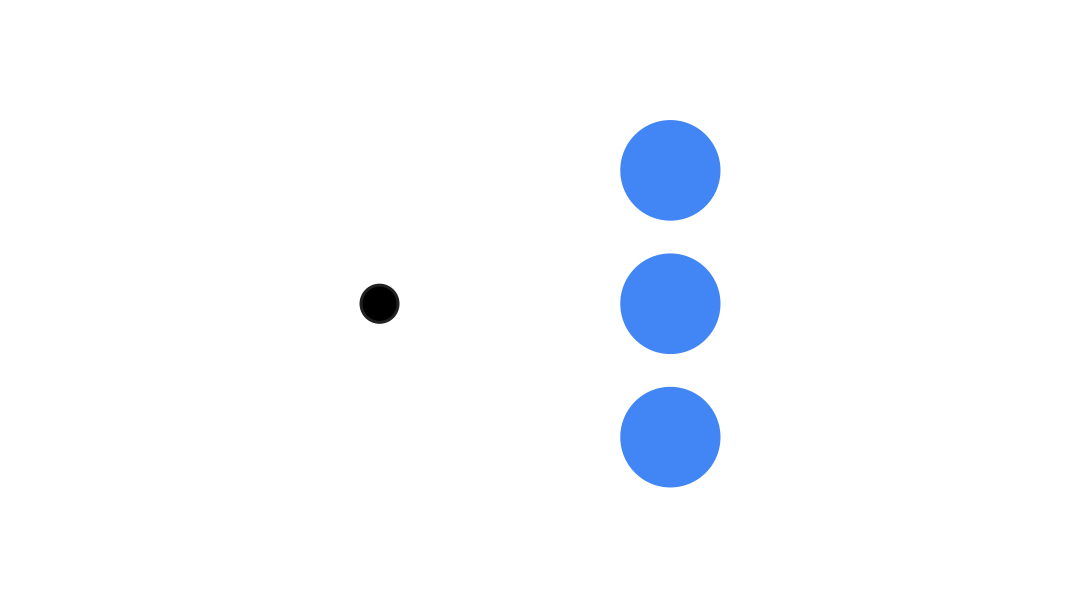
ใช้ coroutine API (Animatable#animateTo()
หรือ animate
) หรือ Transition
API เพื่อให้ได้ภาพเคลื่อนไหวพร้อมกัน หากคุณใช้หลายอุปกรณ์
เปิดใช้งานฟังก์ชันในบริบทโครูทีน
เปิดตัวภาพเคลื่อนไหวพร้อมกับ
เวลา:
val alphaAnimation = remember { Animatable(0f) } val yAnimation = remember { Animatable(0f) } LaunchedEffect("animationKey") { launch { alphaAnimation.animateTo(1f) } launch { yAnimation.animateTo(100f) } }
คุณสามารถใช้ updateTransition
API เพื่อใช้สถานะเดียวกันในการขับรถได้
ภาพเคลื่อนไหวของคุณสมบัติที่แตกต่างกันได้หลายตัวในเวลาเดียวกัน ตัวอย่างด้านล่างเป็นภาพเคลื่อนไหว
มีพร็อพเพอร์ตี้ 2 รายการที่ถูกควบคุมโดยการเปลี่ยนแปลงสถานะ ได้แก่ rect
และ borderWidth
var currentState by remember { mutableStateOf(BoxState.Collapsed) } val transition = updateTransition(currentState, label = "transition") val rect by transition.animateRect(label = "rect") { state -> when (state) { BoxState.Collapsed -> Rect(0f, 0f, 100f, 100f) BoxState.Expanded -> Rect(100f, 100f, 300f, 300f) } } val borderWidth by transition.animateDp(label = "borderWidth") { state -> when (state) { BoxState.Collapsed -> 1.dp BoxState.Expanded -> 0.dp } }
เพิ่มประสิทธิภาพของภาพเคลื่อนไหว
ภาพเคลื่อนไหวในเครื่องมือเขียนอาจทำให้เกิดปัญหาด้านประสิทธิภาพ เนื่องจากลักษณะ ภาพเคลื่อนไหวเป็นอย่างไร ทั้งการเคลื่อนไหวหรือเปลี่ยนพิกเซลบนหน้าจออย่างรวดเร็ว ทีละเฟรมเพื่อสร้างภาพลวงตาของการเคลื่อนไหว
ลองพิจารณาระยะต่างๆ ขององค์ประกอบ ได้แก่ การจัดองค์ประกอบ เลย์เอาต์ และการวาด ถ้า ภาพเคลื่อนไหวจะเปลี่ยนระยะของเลย์เอาต์ต้องใช้ Composable ที่ได้รับผลกระทบทั้งหมด การส่งต่อและวาดใหม่ หากภาพเคลื่อนไหวของคุณเกิดขึ้นในระยะการวาด ค่าเริ่มต้นจะมีประสิทธิภาพมากกว่ากรณีที่คุณเรียกใช้ภาพเคลื่อนไหวในเลย์เอาต์ เพราะจะทำให้มีงานทำในภาพรวมน้อยลง
เลือก lambda เพื่อให้แอปทำงานน้อยที่สุดขณะแสดงภาพเคลื่อนไหว
Modifier
หากเป็นไปได้ ซึ่งจะข้ามการจัดองค์ประกอบใหม่และแสดงภาพเคลื่อนไหวนอกระยะการจัดองค์ประกอบ หรือใช้ Modifier.graphicsLayer{ }
เนื่องจากตัวแก้ไขนี้จะทำงานในระยะวาดเสมอ สำหรับข้อมูลเพิ่มเติมเกี่ยวกับเรื่องนี้ โปรดดูส่วนการเลื่อนการอ่านใน
เอกสารประกอบเกี่ยวกับประสิทธิภาพ
เปลี่ยนเวลาของภาพเคลื่อนไหว
โดยค่าเริ่มต้น การเขียนจะใช้ภาพเคลื่อนไหวฤดูใบไม้ผลิสำหรับภาพเคลื่อนไหวส่วนใหญ่ Springs หรือ
ภาพเคลื่อนไหวที่อิงตามฟิสิกส์ ให้ความรู้สึกเป็นธรรมชาติมากขึ้น และยังรบกวนผู้ใช้ได้ด้วย
จะคำนึงถึงความเร็วปัจจุบันของวัตถุ ไม่ใช่เวลาคงที่
หากต้องการลบล้างค่าเริ่มต้น API ภาพเคลื่อนไหวทั้งหมดที่แสดงข้างต้น
สามารถตั้งค่า animationSpec
เพื่อปรับแต่ง
วิธีแสดงภาพเคลื่อนไหวได้
คุณต้องการให้ทำงาน
เป็นระยะเวลาหนึ่งหรือให้เด้งดึ๋งขึ้น
ต่อไปนี้เป็นข้อมูลสรุปเกี่ยวกับตัวเลือกต่างๆ ของ animationSpec
:
spring
: ภาพเคลื่อนไหวตามฟิสิกส์ ซึ่งเป็นค่าเริ่มต้นสำหรับภาพเคลื่อนไหวทั้งหมด คุณ เปลี่ยนความแข็งหรืออัตราส่วนการหน่วงได้เพื่อให้ได้ภาพเคลื่อนไหวแบบอื่น รูปลักษณ์และความรู้สึกtween
(ย่อมาจาก between): ภาพเคลื่อนไหวตามระยะเวลา จะแสดงภาพเคลื่อนไหวระหว่าง 2 ค่าด้วยฟังก์ชันEasing
keyframes
: ข้อกำหนดสำหรับการระบุค่าที่จุดสำคัญบางจุดใน ภาพเคลื่อนไหวrepeatable
: ข้อมูลจำเพาะตามระยะเวลาที่ทำงานเป็นจํานวนครั้งที่ระบุโดยRepeatMode
infiniteRepeatable
: ข้อกำหนดตามระยะเวลาที่ทำงานตลอดไปsnap
: สแนปไปยังค่าสิ้นสุดทันทีโดยไม่ต้องมีภาพเคลื่อนไหว
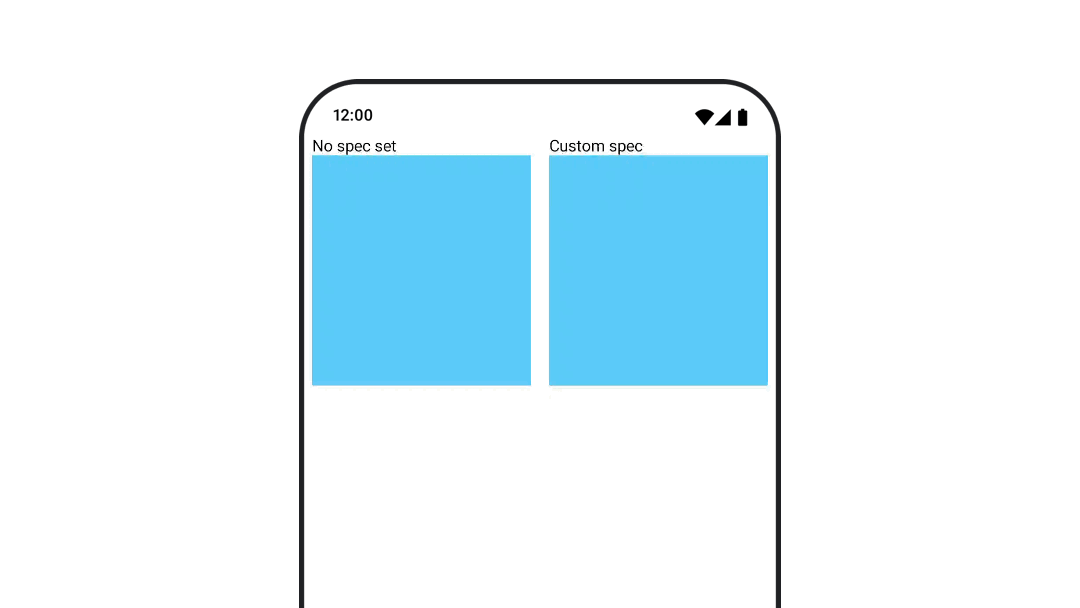
อ่านเอกสารประกอบฉบับเต็มเพื่อดูข้อมูลเพิ่มเติมเกี่ยวกับ animationSpecs
แหล่งข้อมูลเพิ่มเติม
ดูตัวอย่างภาพเคลื่อนไหวสนุกๆ เพิ่มเติมในเครื่องมือเขียนได้ที่ด้านล่าง
- ภาพเคลื่อนไหวสั้นๆ 5 แบบใน Compose
- การทำให้แมงกะพรุนเคลื่อนไหวใน Compose
- การปรับแต่ง
AnimatedContent
ใน Compose - ทำความรู้จักกับฟังก์ชัน Easing ในเครื่องมือเขียน