以下各節將說明如何使用 Glance 建立簡易的應用程式小工具。
在資訊清單中宣告 AppWidget
- 在
AndroidManifest.xml
檔案中註冊應用程式小工具的供應商 相關的中繼資料檔案:
<receiver android:name=".glance.MyReceiver"
android:exported="true">
<intent-filter>
<action android:name="android.appwidget.action.APPWIDGET_UPDATE" />
</intent-filter>
<meta-data
android:name="android.appwidget.provider"
android:resource="@xml/my_app_widget_info" />
</receiver>
- 從
GlanceAppWidgetReceiver
擴充AppWidget
接收器:
class MyAppWidgetReceiver : GlanceAppWidgetReceiver() { override val glanceAppWidget: GlanceAppWidget = TODO("Create GlanceAppWidget") }
新增 AppWidgetProviderInfo
中繼資料
接下來,請按照下列步驟新增 AppWidgetProviderInfo
中繼資料:
按照「建立簡易小工具」指南的說明建立及定義應用程式
@xml/my_app_widget_info
檔案中的小工具資訊。Glance 的唯一差別在於,沒有
initialLayout
XML,但 您必須先定義一個您可以使用 程式庫:
<appwidget-provider xmlns:android="http://schemas.android.com/apk/res/android"
android:initialLayout="@layout/glance_default_loading_layout">
</appwidget-provider>
定義「GlanceAppWidget
」
建立從
GlanceAppWidget
擴充的新類別,並覆寫provideGlance
方法。您可以使用這個方法載入 轉譯小工具所需的資源:
class MyAppWidget : GlanceAppWidget() { override suspend fun provideGlance(context: Context, id: GlanceId) { // In this method, load data needed to render the AppWidget. // Use `withContext` to switch to another thread for long running // operations. provideContent { // create your AppWidget here Text("Hello World") } } }
- 在
GlanceAppWidgetReceiver
的glanceAppWidget
中執行個體化:
class MyAppWidgetReceiver : GlanceAppWidgetReceiver() { // Let MyAppWidgetReceiver know which GlanceAppWidget to use override val glanceAppWidget: GlanceAppWidget = MyAppWidget() }
您現已使用「資訊一覽」設定 AppWidget
。
建立使用者介面
下列程式碼片段說明如何建立 UI:
/* Import Glance Composables In the event there is a name clash with the Compose classes of the same name, you may rename the imports per https://kotlinlang.org/docs/packages.html#imports using the `as` keyword. import androidx.glance.Button import androidx.glance.layout.Column import androidx.glance.layout.Row import androidx.glance.text.Text */ class MyAppWidget : GlanceAppWidget() { override suspend fun provideGlance(context: Context, id: GlanceId) { // Load data needed to render the AppWidget. // Use `withContext` to switch to another thread for long running // operations. provideContent { // create your AppWidget here GlanceTheme { MyContent() } } } @Composable private fun MyContent() { Column( modifier = GlanceModifier.fillMaxSize() .background(GlanceTheme.colors.background), verticalAlignment = Alignment.Top, horizontalAlignment = Alignment.CenterHorizontally ) { Text(text = "Where to?", modifier = GlanceModifier.padding(12.dp)) Row(horizontalAlignment = Alignment.CenterHorizontally) { Button( text = "Home", onClick = actionStartActivity<MyActivity>() ) Button( text = "Work", onClick = actionStartActivity<MyActivity>() ) } } } }
上述程式碼範例會執行以下操作:
- 在頂層
Column
中,各個項目會依序放置在垂直排列一個項目 其他。 Column
會展開大小以符合可用空間 (透過GlanceModifier
,並將內容對齊頂端 (verticalAlignment
) 然後水平置中 (horizontalAlignment
)。- 系統會使用 lambda 定義
Column
的內容。這些順序十分重要。
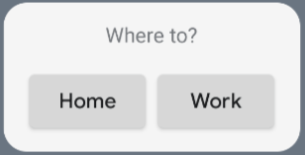
您可以變更對齊值,或套用不同的修飾符值 (例如 邊框間距),即可變更元件的位置和大小。詳情請參閱參考資料 說明文件,內含元件、參數和可用物件的完整清單 每個類別的修飾符