如果您有基于 View 的现有应用,您可能不想重写整个应用 所有操作本页可帮助您将新的 Compose 组件添加到 现有应用如需开始在应用中使用 Compose,请参阅设置 Compose 现有应用的建议。
Jetpack Compose 从设计之初就考虑到了 View 互操作性。此功能意味着,您可以将现有的基于 View 的应用迁移到 Compose,同时仍然能够构建新功能。如需迁移到 Compose,我们建议您执行增量迁移(Compose 和 View 在代码库中共存),直到应用完全迁移至 Compose 为止。
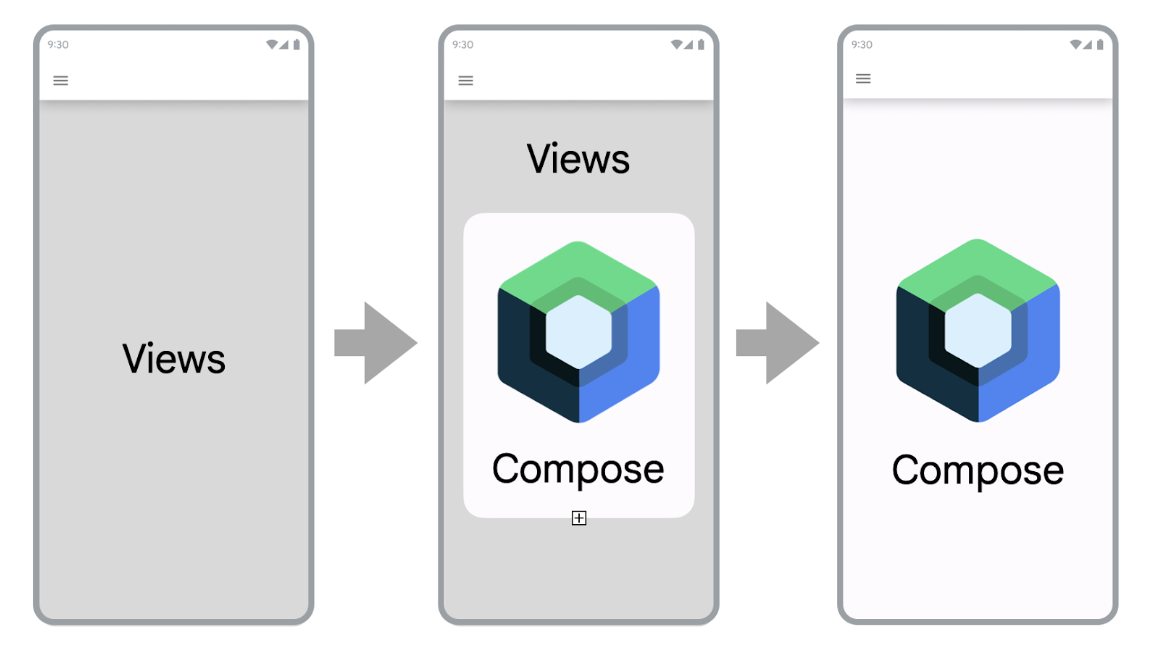
如需将应用迁移到 Compose,请按以下步骤操作:
- 使用 Compose 构建新界面。
- 在构建功能时,确定可重复使用的元素,并开始创建常见界面组件库。
- 一次替换一个界面的现有功能。
使用 Compose 构建新界面
最好使用 Compose 构建覆盖整个界面的新功能 来推动 Compose 的采用。借助此策略,您可以 并利用 Compose 的优势,同时仍然满足您的需求 业务需求。
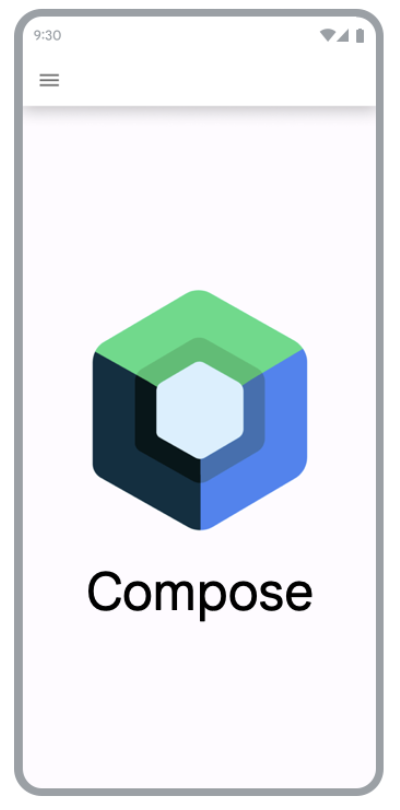
在使用 Compose 在现有应用中构建新界面时 能够在应用架构限制条件下正常运行。如果您使用的是 fragment 和 Navigation 组件,那么您必须创建新的 fragment,并在 Compose 中添加其内容。
如需在 fragment 中使用 Compose,请在 fragment 的 onCreateView()
生命周期方法中返回 ComposeView
。ComposeView
具有 setContent()
方法,您可以在该方法中提供一个可组合函数。
class NewFeatureFragment : Fragment() { override fun onCreateView( inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle? ): View { return ComposeView(requireContext()).apply { setViewCompositionStrategy(ViewCompositionStrategy.DisposeOnViewTreeLifecycleDestroyed) setContent { NewFeatureScreen() } } } }
如需了解详情,请参阅 fragment 中的 ComposeView。
在现有屏幕中添加新功能
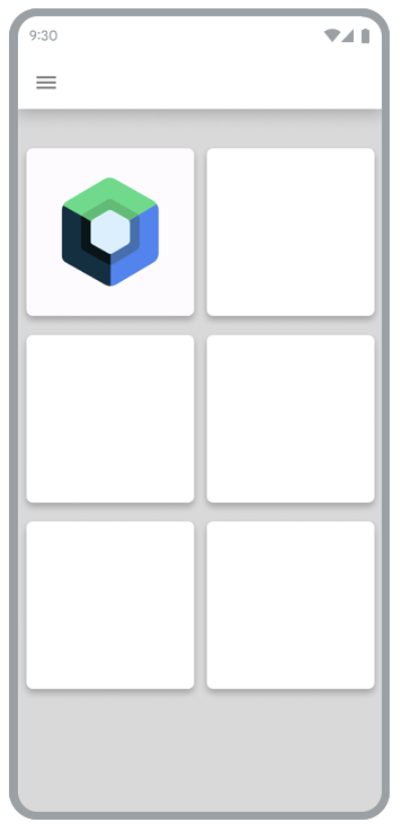
如果您要添加的新功能是现有界面的一部分,您还可以在基于 View 的现有界面中使用 Compose。为此,请像添加任何其他 View 一样,将 ComposeView
添加到 View 层次结构中。
例如,假设您想向 LinearLayout
添加子视图。您可以在 XML 中执行此操作,如下所示:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/text" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <androidx.compose.ui.platform.ComposeView android:id="@+id/compose_view" android:layout_width="match_parent" android:layout_height="match_parent" /> </LinearLayout>
该视图经过扩充后,您可以稍后引用层次结构中的 ComposeView
并调用 setContent()
。
如需详细了解 ComposeView
,请参阅 Interoperability API。
构建常见界面组件库
使用 Compose 构建功能时,您很快就会意识到,您最终会构建组件库。通过创建常见界面组件库,您可以在应用中为这些组件提供单一可信来源,并提高可重用性。您构建的功能随后可以依赖于这个库。如果您要在 Compose 中构建自定义设计系统,此方法会特别有用。
此库可以是单独的软件包、模块或库模块,具体取决于应用的大小。如需详细了解如何整理应用中的各个模块,请参阅 Android 应用模块化指南。
使用 Compose 替换现有功能
除了使用 Compose 构建新功能之外,您还需要逐步迁移应用中的现有功能,以便利用 Compose。
将应用设为仅处理 Compose 可以加快开发速度, 缩减应用的 APK 大小和构建时间。请参阅比较 Compose 和 View 性能。
简单界面
将现有功能迁移到 Compose 时,应首先查看简单的界面。简单的界面可以是欢迎界面、确认界面或设置界面,这些界面中显示的数据是相对静态的。
接受以下 XML 文件:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/title_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/title" android:textAppearance="?attr/textAppearanceHeadline2" /> <TextView android:id="@+id/subtitle_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/subtitle" android:textAppearance="?attr/textAppearanceHeadline6" /> <TextView android:id="@+id/body_text" android:layout_width="wrap_content" android:layout_height="0dp" android:layout_weight="1" android:text="@string/body" android:textAppearance="?attr/textAppearanceBody1" /> <Button android:id="@+id/confirm_button" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="@string/confirm"/> </LinearLayout>
您可以在 XML 中用几行代码重新编写 XML 文件:
@Composable fun SimpleScreen() { Column(Modifier.fillMaxSize()) { Text( text = stringResource(R.string.title), style = MaterialTheme.typography.headlineMedium ) Text( text = stringResource(R.string.subtitle), style = MaterialTheme.typography.headlineSmall ) Text( text = stringResource(R.string.body), style = MaterialTheme.typography.bodyMedium ) Spacer(modifier = Modifier.weight(1f)) Button(onClick = { /* Handle click */ }, Modifier.fillMaxWidth()) { Text(text = stringResource(R.string.confirm)) } } }
混合视图和 Compose 界面
已包含少量 Compose 代码的界面非常适合完全迁移到 Compose。根据界面的复杂性,您可以将其完全迁移到 Compose,也可以逐段迁移。如果界面最初包含以界面层次结构的子树状显示的 Compose,您需继续迁移界面元素,直到整个界面都显示在 Compose 中。此方法也称为“自下而上”方法。
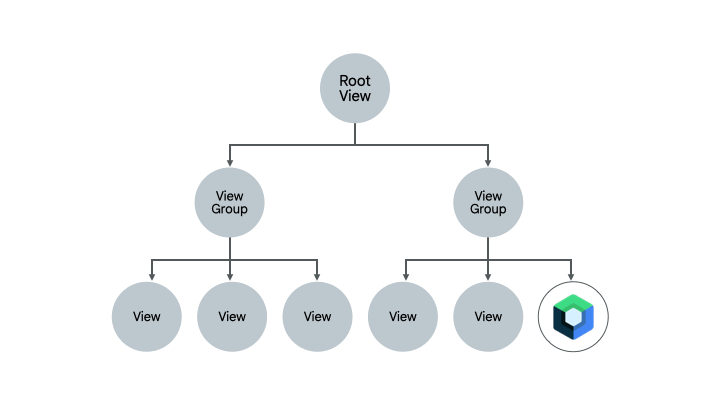
移除 fragment 和 Navigation 组件
移除所有 fragment 并将其替换为相应的界面级可组合项后,您就可以迁移到 Navigation Compose 了。屏幕级可组合项可以包含 Compose 和 View 内容的混合,但所有导航目的地都必须是可组合项,才能启用 Navigation Compose 迁移。在此之前,您应继续在混合 View 和 Compose 代码库中使用基于 fragment 的 Navigation 组件。如需了解详情,请参阅将 Jetpack Navigation 迁移到 Navigation Compose。
其他资源
如需详细了解如何将现有的基于 View 的应用迁移到 Compose,请参阅下面列出的其他资源:
- Codelab
- 迁移到 Jetpack Compose:在此 Codelab 中,了解如何将 Sunflower 应用的部分界面迁移到 Compose。
- 博文
- 将 Sunflower 迁移到 Jetpack Compose:了解如何使用本页所述的策略将 Sunflower 迁移到 Compose。
- Jetpack Compose 互操作性:在 RecyclerView 中使用 Compose:了解如何在
RecyclerView
中高效使用 Compose。
后续步骤
现在,您已经了解迁移现有的基于 View 的应用可采取的策略,如需了解更多内容,可以探索互操作性 API。
为您推荐
- 注意:当 JavaScript 处于关闭状态时,系统会显示链接文字
- 在 View 中使用 Compose
- 滚动
- 将
RecyclerView
迁移到延迟列表